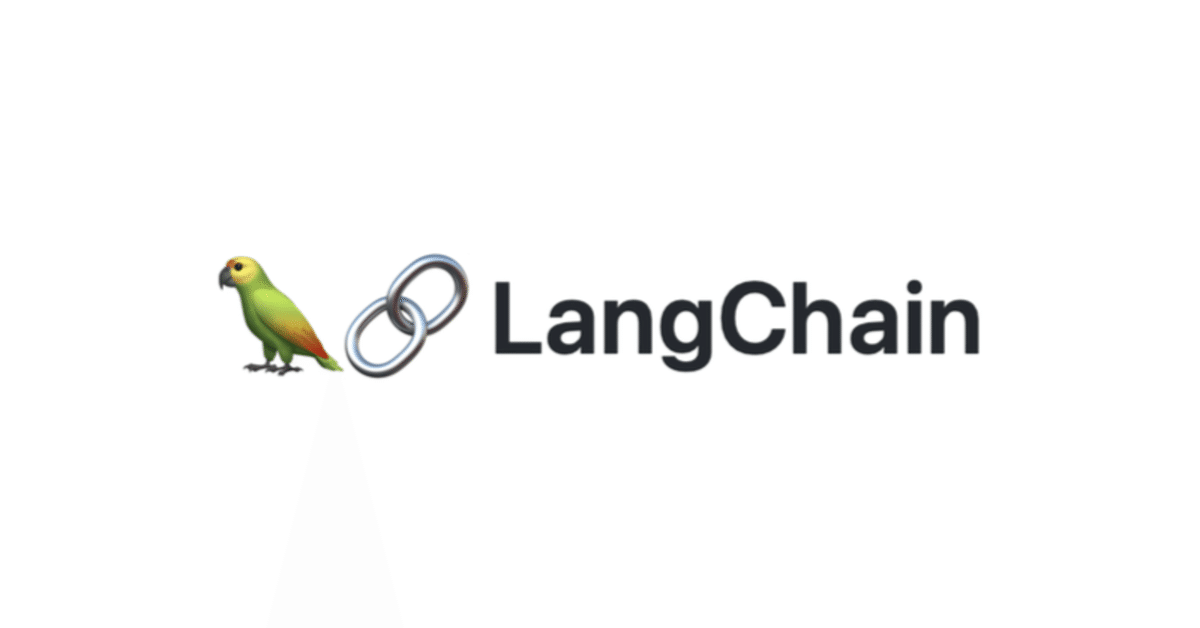
LLM連携アプリの開発を支援するライブラリ LangChain の使い方 (4) - メモリ
「LangChain」の「メモリ」の使い方をまとめました。
前回
1. メモリ
「メモリ」は、過去のメッセージのやり取りを記憶します。前回までのチェーンとエージェントはステートレスでした。しかし、過去のやり取りの記憶を持たせたい場合もよくあります。「メモリ」は、この処理をサポートします。
2. インストール
Google Colabでのインストール手順は、次のとおりです。
(1) パッケージのインストール。
# パッケージのインストール
!pip install langchain
!pip install openai
(2) 環境変数の準備。
以下のコードの <OpenAI_APIのトークン> にはOpenAI APIのトークンを指定します。(有料)
# 環境変数の準備
import os
os.environ["OPENAI_API_KEY"] = "<OpenAI_APIのトークン>"
3. Colabでの実行
Google Colabでの「メモリ」の実行手順は、次のとおりです。
3-1. デフォルトメモリ
(1) ConversationChainの準備と会話の実行。
ConversationChainは会話のためのチェーンです。
from langchain import OpenAI, ConversationChain
# ConversationChainの準備
conversation = ConversationChain(
llm=OpenAI(temperature=0),
verbose=True
)
# 会話の実行
conversation.predict(input="こんにちは!")
> Entering new ConversationChain chain...
Prompt after formatting:
The following is a friendly conversation between a human and an AI. The AI is talkative and provides lots of specific details from its context. If the AI does not know the answer to a question, it truthfully says it does not know.
Current conversation:
Human: こんにちは!
AI:
> Finished ConversationChain chain.
' こんにちは!私はAIです。どうぞよろしくお願いします!'
(2) 会話の実行。
プロンプトには、過去のやりとりが含まれていることがわかります。
# 会話の実行
conversation.predict(input="AIさんはどこで生まれたのですか?")
> Entering new ConversationChain chain...
Prompt after formatting:
The following is a friendly conversation between a human and an AI. The AI is talkative and provides lots of specific details from its context. If the AI does not know the answer to a question, it truthfully says it does not know.
Current conversation:
Human: こんにちは!
AI: こんにちは!私はAIです。どうぞよろしくお願いします!
Human: AIさんはどこで生まれたのですか?
AI:
> Finished ConversationChain chain.
' 私はコンピューター上で生まれました。私はプログラムによって作られ、コンピューター上で動作しています。
(3) 会話の実行。
# 会話の実行
conversation.predict(input="何という名前のコンピュータですか?")
> Entering new ConversationChain chain...
Prompt after formatting:
The following is a friendly conversation between a human and an AI. The AI is talkative and provides lots of specific details from its context. If the AI does not know the answer to a question, it truthfully says it does not know.
Current conversation:
Human: こんにちは!
AI: こんにちは!私はAIです。どうぞよろしくお願いします!
Human: AIさんはどこで生まれたのですか?
AI: 私はコンピューター上で生まれました。私はプログラムによって作られ、コンピューター上で動作しています。
Human: 何という名前のコンピュータですか?
AI:
> Finished ConversationChain chain.
' 私の名前はAIですが、コンピューターの名前はわかりません。
3-2. ConversationSummaryMemory
(1) ConversationChainの準備と会話の実行。
ConversationChainにConversationSummaryMemoryを設定します。
from langchain.chains.conversation.memory import ConversationSummaryMemory
# ConversationChainの準備
conversation_with_summary = ConversationChain(
llm=OpenAI(temperature=0),
memory=ConversationSummaryMemory(llm=OpenAI()),
verbose=True
)
# 会話の実行
conversation_with_summary.predict(input="こんにちは!")
> Entering new ConversationChain chain...
Prompt after formatting:
The following is a friendly conversation between a human and an AI. The AI is talkative and provides lots of specific details from its context. If the AI does not know the answer to a question, it truthfully says it does not know.
Current conversation:
Human: こんにちは!
AI:
> Finished ConversationChain chain.
' こんにちは!私はAIです。どうぞよろしくお願いします!'
(2) 会話の実行。
プロンプトには、過去のやりとりが要約されて含まれていることがわかります。(英語で要約されてるので、日本語で要約するように調整しないとかも)
# 会話の実行
conversation_with_summary.predict(input="AIさんはどこで生まれたのですか?")
> Entering new ConversationChain chain...
Prompt after formatting:
The following is a friendly conversation between a human and an AI. The AI is talkative and provides lots of specific details from its context. If the AI does not know the answer to a question, it truthfully says it does not know.
Current conversation:
The human greets the AI, who responds in kind and introduces itself as an AI.
Human: AIさんはどこで生まれたのですか?
AI:
> Finished ConversationChain chain.
' 私は、コンピューター上で生まれました。私は、プログラムとデータを使用して作成されました。
(3) 会話の実行。
# 会話の実行
conversation_with_summary.predict(input="何という名前のコンピュータですか?")
> Entering new ConversationChain chain...
Prompt after formatting:
The following is a friendly conversation between a human and an AI. The AI is talkative and provides lots of specific details from its context. If the AI does not know the answer to a question, it truthfully says it does not know.
Current conversation:
The human greets the AI, who responds in kind and introduces itself as an AI. The human then asks where it was born, to which the AI responds that it was created on a computer using programs and data.
Human: 何という名前のコンピュータですか?
AI:
> Finished ConversationChain chain.
' 私は、IBMのPower Systemsサーバー上で作成されました。'