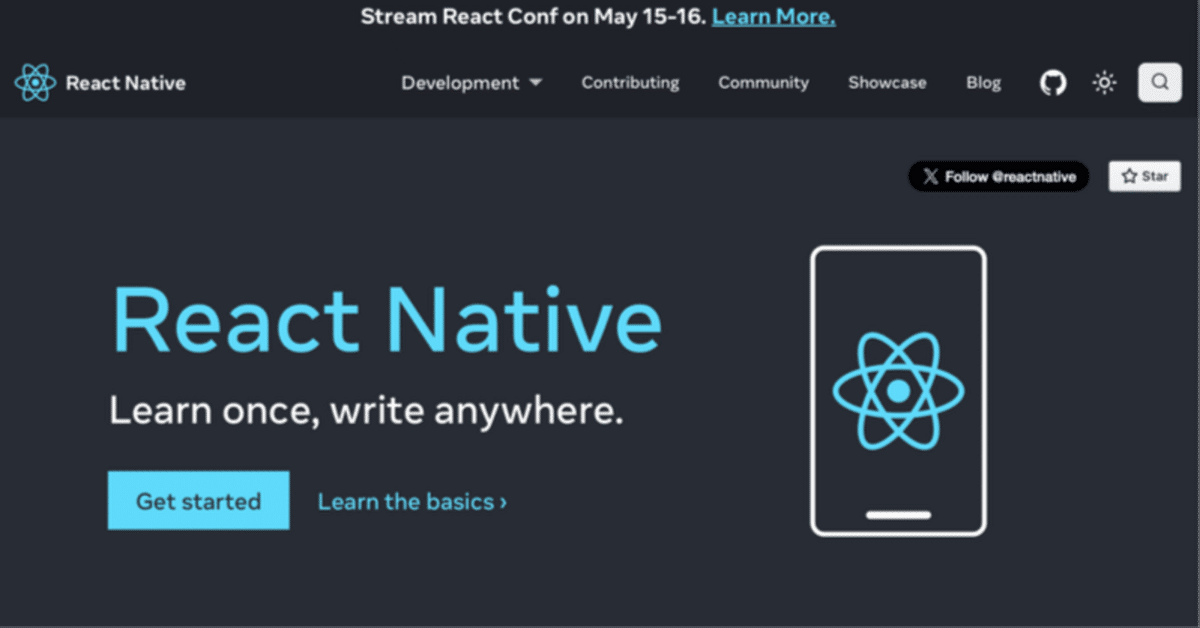
react-native-fs の使い方
「react-native-fs」の使い方をまとめました。
前回
1. react-native-fs
「react-native-fs」は、React Nativeアプリからネイティブファイルシステムにアクセスするためのライブラリです。
2. ファイルの読み書き
(1) React Nativeプロジェクトの生成。
npx react-native init my_app
cd my_app
(2) パッケージのインストール。
npm install react-native-fs
(3) コードの編集と実行。
import React from 'react';
import { Button, View } from 'react-native';
import RNFS from 'react-native-fs';
// アプリ
const App = () => {
// ファイルの書き込み
const onWriteFile = async () => {
const path = RNFS.DocumentDirectoryPath + '/test.txt';
RNFS.writeFile(path, 'This is a test file', 'utf8')
.then(() => {
console.log('File written successfully!');
})
.catch((err) => {
console.log(err.message);
});
};
// ファイルの読み込み
const onReadFile = async () => {
const path = RNFS.DocumentDirectoryPath + '/test.txt';
RNFS.readFile(path, 'utf8')
.then((contents) => {
console.log(contents);
})
.catch((err) => {
console.log(err.message);
});
};
// ファイルの削除
const onDeleteFile = async () => {
const path = RNFS.DocumentDirectoryPath + '/test.txt';
RNFS.unlink(path)
.then(() => {
console.log('File deleted successfully!');
})
.catch((err) => {
console.log(err.message);
});
};
// ディレクトリの読み込み
const onReadDir = async () => {
const dirPath = RNFS.DocumentDirectoryPath;
RNFS.readDir(dirPath)
.then((result) => {
console.log(result);
})
.catch((err) => {
console.log(err.message);
});
};
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<View style={{marginVertical: 10}}>
<Button title="Write File" onPress={onWriteFile} />
</View>
<View style={{marginVertical: 10}}>
<Button title="Read File" onPress={onReadFile} />
</View>
<View style={{marginVertical: 10}}>
<Button title="Delete File" onPress={onDeleteFile} />
</View>
<View style={{marginVertical: 10}}>
<Button title="Read Directory" onPress={onReadDir} />
</View>
</View>
);
};
export default App;
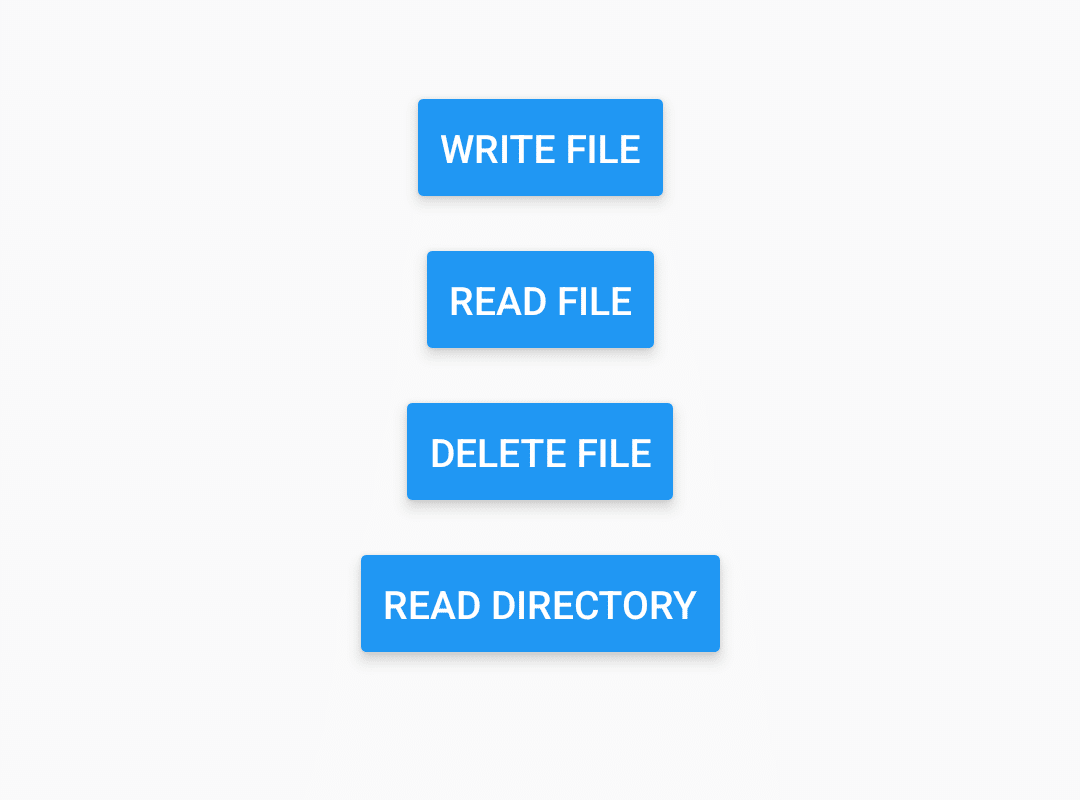
次回
この記事が気に入ったらサポートをしてみませんか?