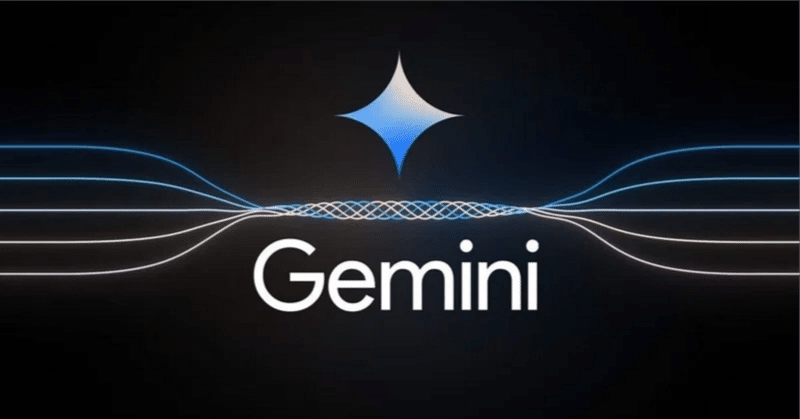
Gemini API の Code Execution
以下の記事が面白かったので、簡単にまとめました。
・Code execution | Google AI for Developers | Google for Developers
1. Code Eexecution
「Code Execution」は、モデルがPythonコードを生成して実行することを可能にします。「Google AI Studio」や「Gemini API」で利用可能です。仮想マシン上でNumPyやSimPyなどのライブラリを使用できますが、追加のライブラリをインストールすることはできません。コード実行はモデルまたはリクエストレベルで有効化でき、チャットでも利用可能です。実行環境には30秒のタイムアウトなどの制限があります。
2. Code Execution の有効化
「Code Execution」の有効化の手順は、次のとおりです。
(1) パッケージのインストール。
# パッケージのインストール
!pip install -q -U google-generativeai
(2) 環境変数の準備。
from google.colab import userdata
import google.generativeai as genai
# 環境変数の準備 (左端の鍵アイコンでGOOGLE_API_KEYを設定)
GOOGLE_API_KEY=userdata.get("GOOGLE_API_KEY")
genai.configure(api_key=GOOGLE_API_KEY)
(3) コードの実行。
import os
import google.generativeai as genai
# モデルの準備
model = genai.GenerativeModel(
model_name="gemini-1.5-pro",
tools="code_execution" # Code Executionの有効化
)
# 推論
response = model.generate_content((
"What is the sum of the first 50 prime numbers? "
"Generate and run code for the calculation, and make sure you get all 50."))
print(response.text)
```python
def is_prime(n):
"""Checks if a number is prime."""
if n <= 1:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
def sum_of_primes(n):
"""Calculates the sum of the first n prime numbers."""
primes = []
i = 2
while len(primes) < n:
if is_prime(i):
primes.append(i)
i += 1
return sum(primes)
# Calculate the sum of the first 50 prime numbers
sum_of_first_50_primes = sum_of_primes(50)
print(f"The sum of the first 50 prime numbers is: {sum_of_first_50_primes}")
```
**Explanation:**
1. **`is_prime(n)` Function:**
- Takes an integer `n` as input.
- Returns `False` for numbers less than or equal to 1 (not prime).
- Iterates from 2 up to the square root of `n`. If `n` is divisible by any
number in this range, it's not prime, and we return `False`.
- If the loop completes without finding a divisor, the number is prime, and
we return `True`.
2. **`sum_of_primes(n)` Function:**
- Takes an integer `n` (number of primes desired) as input.
- Initializes an empty list `primes` to store the prime numbers.
- Starts a loop, iterating through numbers starting from 2.
- For each number `i`, it checks if it's prime using the `is_prime()` function.
- If `i` is prime, it's appended to the `primes` list.
- The loop continues until the `primes` list has `n` prime numbers.
- Finally, it calculates and returns the sum of all the prime numbers in the
`primes` list.
3. **Main Part:**
- Calls `sum_of_primes(50)` to get the sum of the first 50 prime numbers.
- Prints the result.
**Output:**
```
The sum of the first 50 prime numbers is: 5117
リクエスト時に有効化することもできます。
# モデルの準備
model = genai.GenerativeModel(
model_name='gemini-1.5-pro'
)
# 推論
response = model.generate_content(
("What is the sum of the first 50 prime numbers? "
"Generate and run code for the calculation, and make sure you get all 50."),
tools="code_execution" # Code Execution の有効化
)
print(response.text)
4. Code Execution と Function Calling
「Code Execution」と「Function Calling」は類似した機能です。
・Code Execution : モデルはAPIバックエンドのコードを隔離された環境で実行
・Function Calling : モデルが要求する関数を任意の環境で実行
一般的にユースケースに対応できる場合は、「Code Execution」を使用することを推奨します。「Code Execution」は使い方が簡単で (有効化のみ)、1回のリクエストで解決されます (1 回の料金が発生)。「Function Calling」では、各関数呼び出しからの出力を返すために追加のリクエストが必要です (複数の料金が発生)。
ほとんどの場合、ローカルで実行したい独自の関数がある場合は「Function Calling」を使用し、APIでPython コードを記述して実行し、結果を返したい場合は「Code Execution」を使用する必要があります。
5. Code Execution の使用料金
「Gemini API」で「Code Execution」を有効化しても追加料金はかかりません。入力トークンと出力トークンの現在のレートで課金されます。
知っておくべきことは、次のとおりです。
・モデルに渡す入力トークンに対して1回のみ課金され、モデルから返される最終的な出力トークンに対して課金される。
・生成されたコードを表すトークンは、出力トークンとしてカウントされる。
・コード実行結果も出力トークンとしてカウントされる。
6. Code Execution の制限事項
・モデルはコードを生成して実行することしかできません。メディアファイルなどの他の成果物を返すことはできません。
・この機能は、ファイル I/O や、テキスト以外の出力 (データ プロットなど) を伴うユースケースをサポートしていません。
・「Code Execution」は、タイムアウトするまで最大30秒間実行できます。
・「Code Execution」を有効にすると、モデル出力の他の領域 (ストーリーの作成など) で回帰が発生する可能性があります。
・「Code Execution」を正常に使用する能力は、モデルによって異なります。テストによると、「Gemini 1.5 Pro」が最もパフォーマンスの高いです。
この記事が気に入ったらサポートをしてみませんか?