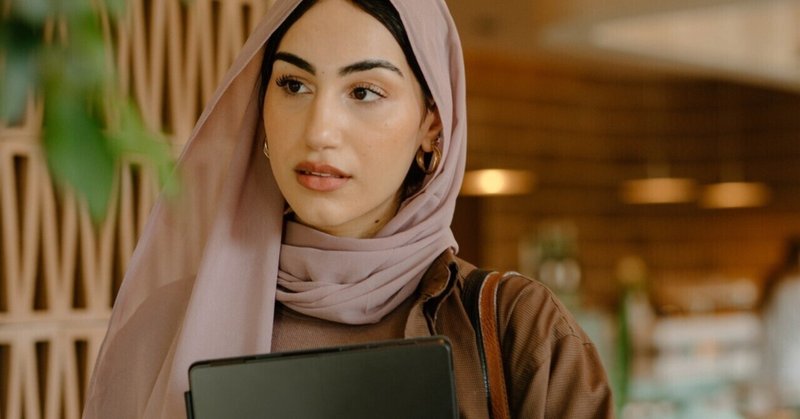
【GAS】Google Apps Script 活用事例 spliceメソッドの挙動を勉強してみた。
値の削除、追加、置換何でも出来るけども、苦手意識があったspliceメソッドの挙動を勉強してみました。
これまでスクリプトを書く際は、主にpush、先頭に加えたい場合のみunshiftを使用するだけで事足りました。値の削除は、reduceを使う事が多いでしょうか。
自分が知っているものしか使わないと引き出しが、
いつまでも増えないので、知っているものを増やすという
方向性も時に重要かなと思っています。
挙動を確認するサンプル
function sampleFunction3(){
//配列の最初の要素から2つを削除する
const array1 = [ '1', '2', '3', '4', '5', '6' ];
array1.splice(0, 2);
console.log('array1.splice(0, 2)', array1);
//expected output [ '3', '4', '5', '6' ]
//最後の値を削除する
const array3 = [ '1', '2', '3', '4', '5', '6' ];
array3.splice(-1, 1);
console.log('array3.splice(-1, 1)', array3);
//expected output [ '1', '2', '3', '4', '5']
//置換する
const array2 = [ '1', '2', '3', '4', '5', '6' ];
array2.splice(0, 1, '1.5');//開始位置, 変化量, 置換後の値
console.log(`array2.splice(0, 1, '1.5')`, array2);
console.log('└ 開始位置, 変化量, 置換後の値');
console.warn('└ 2番目の値が1の時、かつ引数が3つあると置換系メソッド');
//expected output [ '1.5', '2', '3', '4', '5', '6' ]
//2番目の引数を0にすると値を追加する
const array4 = [ '1', '2', '3', '4', '5', '6' ];
array4.splice(1, 0, '1.5');
console.log(`array4.splice(1, 0, '1.5')`, array4);
console.log('└ 開始位置, 変化量, 追加したい値');
console.warn('└ 2番目の値が0の時、追加系メソッド');
//[ '1', '1.5', '2', '3', '4', '5', '6' ]
//2と3を1.5で置き換える
const array5 = [ '1', '2', '3', '4', '5', '6' ];
array5.splice(1, 2, '1.5');//開始位置, 変化量, 置換後の値
console.log(`array5.splice(1, 2, '1.5')`, array5);
console.log('└ 開始位置, 変化量, 置換後の値');
console.warn('└ 2番目の値が1の時、かつ引数が3つあると置換系メソッド');
//[ '1', '1.5', '4', '5', '6' ]
}
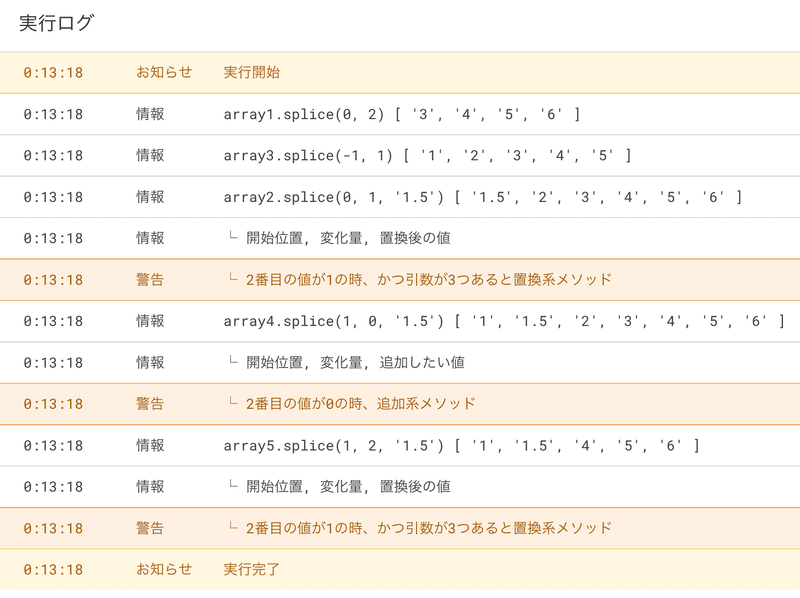
分かりやすいように関数化してみました。
function myFunction(){
const array = [ '1', '2', '3', '4', '5', '6' ];
const newArray = splice_(array, 1, 0, '1.5');
}
/**
* spliceメソッドを使用時に分かりやすくログを表示する
*
* @param {Array.<string>} array - 1次元配列
* @param {number} start - 処理の開始位置
* @param {number} quantity - 変化量 0の時は値を追加、それ以外は置換
* @param {string|number} keyword - 文字列、値いずれも可。2番目の引数によって役割が変化する
*
*/
function splice_(array, start, quantity, keyword){
if(!keyword){
console.warn(`配列の${start}番目から値を${quantity}つ削除します。`);
const deleted = array.splice(start, quantity);
console.log(`削除された値:${deleted}`);
}else if(quantity === 0 && keyword){
console.warn(`配列の${start}番目に値、${keyword}を追加する`);
array.splice(start, quantity, keyword);
}else if(quantity !== 0 && keyword){
console.warn(`配列の${start}番目の値${array[start]}を${keyword}で置換する`);
array.splice(start, quantity, keyword);
}
console.log(array);
return array
}
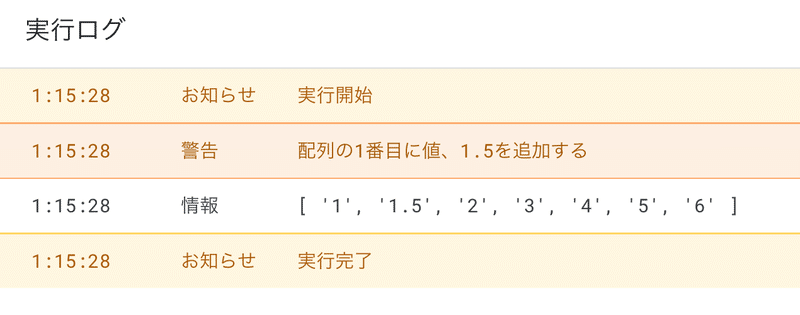
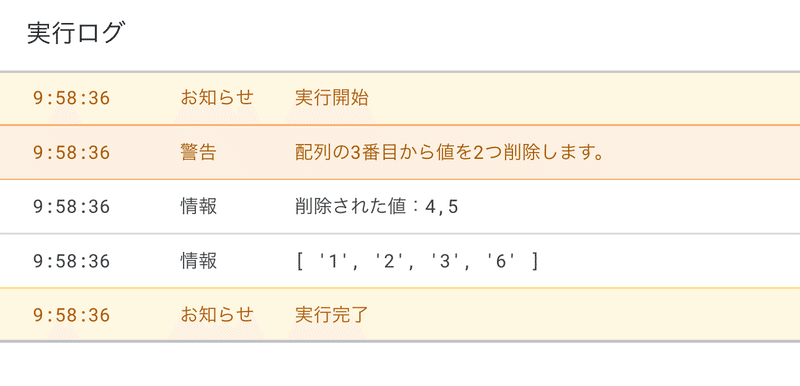
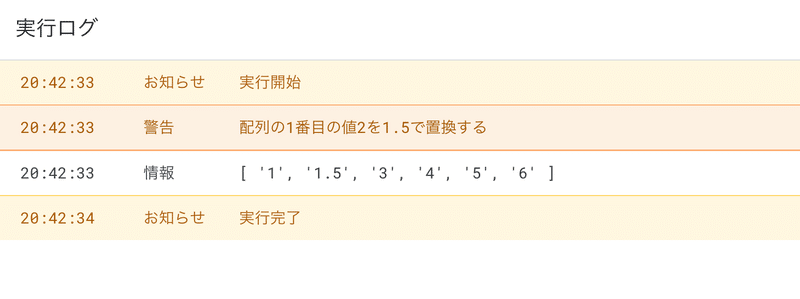
こんな時に使えるかもSpliceメソッド
最近実務では、面接官リストの中から重複せずに二人を選び、カレンダーに登録するという処理を書いた時に使いました。
高校数学の確立で、なんかこんなのやったなぁ〜と。
/**
* 赤、青、白、黄の玉が入った袋の中から重複せずに2個取り出す
*
*/
function myFunction(){
const originalList = ['Red', 'White', 'Blue', 'Red', 'White', 'Yellow', 'Blue', 'Red', 'Red', 'Blue', 'Yellow'];
//次に取り出す玉の重複を避けるために重複を削除する
const random = (number) => { return Math.floor(Math.random() * number)};
const first = originalList[random(originalList.length)];
//最初に取り出した玉を除いた重複なしの配列を作成する
const newList = Array.from(new Set(originalList));
const index = newList.indexOf(first);
newList.splice(index, 1);
const second = newList[random(newList.length)];
console.log(`1番目: ${first} \n2番目: ${second}`);
}
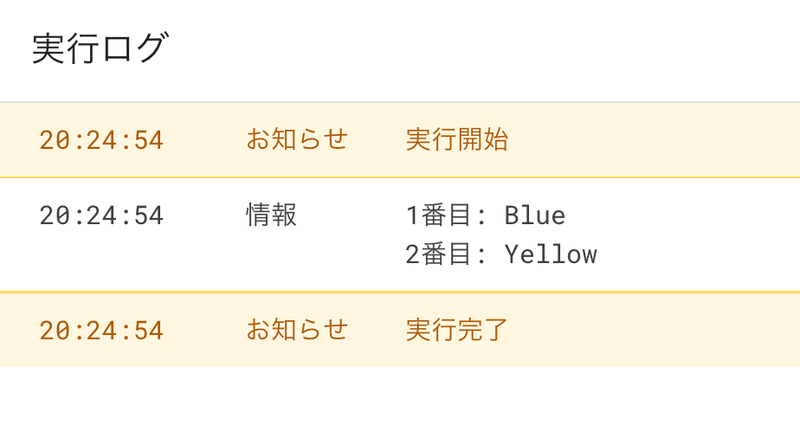
配列を結合する
/**
* 配列を結合する
* 最終更新日: 2022/11/12
*/
function sampleFunction2(){
const array1 = ['abc', 'edf', 'ghj'];
const array2 = ['jkg', 'asd', 'efr'];
const temp = array1.concat(array2);
console.log(temp);
//expeted output [ 'abc', 'edf', 'ghj', 'jkg', 'asd', 'efr' ]
}
オブジェクトを結合する
/**
* オブジェクトを結合する
* 最終更新日: 2022/11/12
*/
function sampleFunction() {
const target = { a: 1, b: 2 };
const source = { c: 5, d: 6 };
const returnedTarget = Object.assign(target, source);
console.log(returnedTarget);
//expeted output { a: 1, b: 2, c: 5, d: 6 }
}
filterメソッドについてのまとめ
この記事が気に入ったらサポートをしてみませんか?