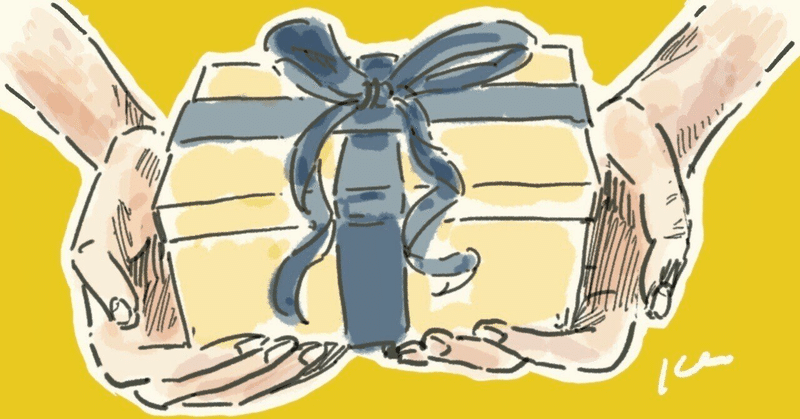
🚀 Tableauユーザー管理を自動化!Slack通知で効率的に古いユーザーをクリーンアップする方法
こんにちは、Tableauユーザーの皆さん。Tableau ServerやTableau Cloudを使用していると、ユーザーの管理が煩雑になりがちです。特に、長期間ログインしていないユーザーを特定し、適切に削除する作業は手間がかかります。そこで今回は、Pythonを使ってTableauのユーザー管理を自動化し、Slackで通知を行う方法をご紹介します。このスクリプトを使えば、古いユーザーを効率的にクリーンアップできるようになります。
1. スクリプトの概要
このスクリプトは、Tableau ServerまたはTableau Cloud上のユーザーのアクセス権をレビューし、古いユーザーを削除します。また、削除予定のユーザーには1週間前にSlackで通知し、削除後にも再度通知を行います。これにより、ユーザー管理が簡素化され、セキュリティリスクを低減できます。
2. 必要な環境と準備
まず、このスクリプトを実行するための環境を整えましょう。以下の環境が必要です。
必要な環境
Python 3.6以上
インターネット接続
Tableau ServerまたはTableau Cloudのアクセス権
SlackワークスペースとBotトークン
ファイル構成
プロジェクトは以下のファイルで構成されます。
config.json: 設定情報を含むファイル
main.py: メインのPythonスクリプト
requirements.txt: 必要なライブラリを記載したファイル
3. スクリプトの詳細
このスクリプトのメイン部分は、ユーザーのアクセス情報を取得し、削除予定のユーザーと削除するユーザーを特定する処理です。それでは、各部分の詳細を見ていきましょう。
config.json
まず、`config.json`ファイルにTableau ServerまたはTableau CloudとSlackの認証情報を入力します。
#Json
{
"tableau": {
"server_url": "your_tableau_server_or_cloud_url",
"personal_access_token_name": "your_personal_access_token_name",
"personal_access_token": "your_personal_access_token",
"site_id": "your_site_id",
"project_name": "your_tableau_project_name"
},
"slack": {
"slack_bot_token": "your_slack_bot_token",
"slack_channel_id": "your_slack_channel_id"
}
}
main.py
続いて、メインのPythonスクリプトです。このスクリプトは、ユーザー情報を取得し、削除予定のユーザーを1週間前に通知し、削除後に再度通知を行います。
#Python
import tableauserverclient as TSC
from datetime import datetime, timedelta
from slack_sdk import WebClient
from slack_sdk.errors import SlackApiError
import pytz
import json
# JSONファイルから設定情報を読み込む関数
def load_config(config_file='config.json'):
with open(config_file, 'r') as f:
config = json.load(f)
return config
# Slackにメッセージを送信する関数
def send_slack_message(client, channel_id, blocks):
try:
response = client.chat_postMessage(channel=channel_id, blocks=blocks)
except SlackApiError as e:
print(f"Error sending message to Slack: {e.response['error']}")
# 古いユーザーや不要なアクセス権を削除する関数
def review_and_cleanup_users(server, slack_client, channel_id, threshold_days=90, notify_days=7):
with server.auth.sign_in(server_auth):
all_users, pagination_item = server.users.get()
now = datetime.now(pytz.utc) # 現在時刻をUTCに設定
threshold_date = now - timedelta(days=threshold_days)
notify_date = now - timedelta(days=threshold_days - notify_days)
removed_users = []
users_to_notify = []
users_to_remove = []
for user in all_users:
if user.last_login:
last_login_utc = user.last_login.astimezone(pytz.utc)
if last_login_utc < notify_date and last_login_utc >= threshold_date:
users_to_notify.append(user)
elif last_login_utc < threshold_date:
users_to_remove.append(user)
if users_to_notify:
pre_removal_message_blocks = [
{
"type": "section",
"text": {
"type": "mrkdwn",
"text": f"*以下のユーザーは、{threshold_days}日以上アクティブではなかったため、1週間後に削除される予定です:*"
}
},
{
"type": "section",
"text": {
"type": "mrkdwn",
"text": "\n".join([f"- {user.name} (最終ログイン: {user.last_login})" for user in users_to_notify])
}
}
]
send_slack_message(slack_client, channel_id, pre_removal_message_blocks)
if users_to_remove:
for user in users_to_remove:
print(f"Removing user: {user.name}, last login: {user.last_login}")
server.users.remove(user.id)
removed_users.append(user.name)
post_removal_message_blocks = [
{
"type": "section",
"text": {
"type": "mrkdwn",
"text": "*以下のユーザーが削除されました:*"
}
},
{
"type": "section",
"text": {
"type": "mrkdwn",
"text": "\n".join([f"- {user}" for user in removed_users])
}
}
]
send_slack_message(slack_client, channel_id, post_removal_message_blocks)
else:
no_removal_message_blocks = [
{
"type": "section",
"text": {
"type": "mrkdwn",
"text": f"*{threshold_days}日以内にすべてのユーザーがログインしています。削除されるユーザーはいません。*"
}
}
]
send_slack_message(slack_client, channel_id, no_removal_message_blocks)
print("User review and cleanup completed.")
if __name__ == "__main__":
config = load_config()
tableau_config = config['tableau']
slack_config = config['slack']
server = TSC.Server(tableau_config['server_url'], use_server_version=True)
server_auth = TSC.PersonalAccessTokenAuth(
tableau_config['personal_access_token_name'],
tableau_config['personal_access_token'],
tableau_config['site_id']
)
slack_client = WebClient(token=slack_config['slack_bot_token'])
slack_channel_id = slack_config['slack_channel_id']
print(f"Starting user review and cleanup at {datetime.now()}")
review_and_cleanup_users(server, slack_client, slack_channel_id, threshold_days=90, notify_days=7)
print("Completed user review and cleanup.")
requirements.txt
依存関係を管理するために`requirements.txt`ファイルを作成します。
tableauserverclient
slack_sdk
pytz
4. 実行方法
必要なライブラリをインストールし、スクリプトを実行します。以下のコマンドを実行してください:
pip install -r requirements.txt
python main.py
5. ユースケース
このスクリプトを使用することで、さまざまなユースケースに対応できます。
定期的なユーザー管理
定期的にスクリプトを実行することで、長期間ログインしていないユーザーを自動的に削除し、ユーザー管理の効率を向上させます。
セキュリティ強化
古いユーザーアカウントを削除することで、不要なアクセス権限を排除し、システムのセキュリティを強化できます。
Slack通知による可視化
削除予定のユーザーや削除されたユーザーの情報をSlackで通知することで、管理者がリアルタイムで状況を把握でき、チーム全体での情報共有が容易になります。
Tableau CloudとTableau Serverの両方に対応
このスクリプトは、Tableau CloudとTableau Serverの両方に対応しているため、どちらの環境でも利用できます。
6. 結果の確認と活用
スクリプトを実行すると、削除予定のユーザーが1週間前にSlackで通知され、実際に削除された際にも通知が行われます。これにより、ユーザー管理が容易になり、セキュリティが向上します。
7. おわりに
Tableauのユーザー管理は多くの企業にとって重要な課題です。このスクリプトを活用することで、効率的かつ安全にユーザーを管理し、システムのパフォーマンスを最適化できます。ぜひ、このスクリプトを試してみてください!
このスクリプトの詳細については、以下のGitHubリポジトリをご参照ください。
ご質問やご意見がありましたら、コメントでお知らせください。
この記事が気に入ったらサポートをしてみませんか?