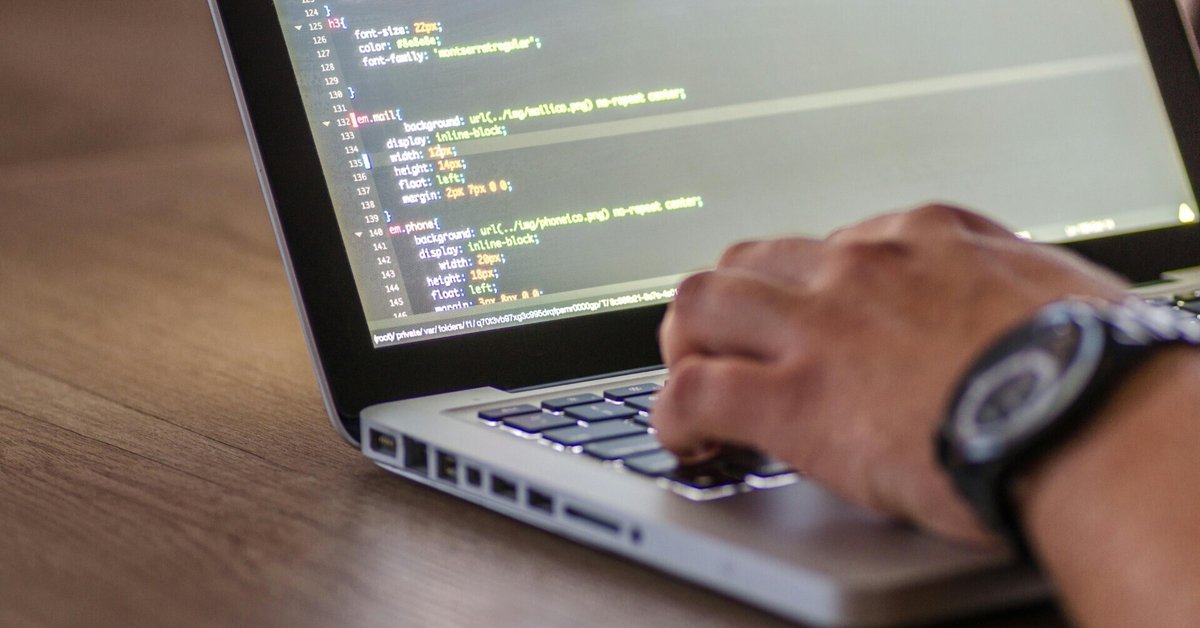
Leetcode を英語で説明する①(Java)
https://leetcode.com/problems/two-sum/description/
説明順序
define the problem
identify parameters and return type
identify unique attributes
explain constraints
explain algorithms
explain the code you wrote
answer time complexity
1. define the problem ( name of problem)
The given problem which is two sum.
2. identify parameters and return type
There are two given parameters, nums and target.
Nums is an array of integer and target is an integer.
Return type is an array of integer which is the indexes of the two numbers who's sum equals target.
3. identify unique attributes
Nums isn't sorted and there is only one solution.
4. explain constraints
Nums cant be 1 or 0. Nums length is minimum 2 and max 10 to the power of 4. Numbers in nums and target is minimum -10 to the power of 9 and max 10 to the power of 9.
5. explain algorithms (how to solve the solution)
we have to check every number to see if the sum of the two numbers equals target because nums isn't sorted.
6. explain the code you wrote
class Solution {
public int[] twoSum(int[] nums, int target) {
int first = 0;
int comp = 0;
int[] res = new int[2];
for (int i = 0; i < nums.length - 1; i++){
first = nums[i];
for(int k = i+1; k < nums.length; k++){
comp = nums[k];
if(first + comp == target){
res[0] = i;
res[1] = k;
return res;
}
}
}
return res;
}
}
Make three variables ( have to explain the data type and what's the purpose of the variables)
two of them are ints, which hold the number values we're comparing.
third is int array of size two that hold solution
parent for loop
this goes through length of nums -1 for each iteration and we're going to save value at the index in first variable we made earlier.
And then also each iteration we're going to check all the other remaining values of nums. We do it with for loop that starts at k = i+1 and goes till full lengths of nums.
child for loop
During this for loop, we'll store the value of nums at k in the another variable we made earlier.
finally if two stored values sum adds up the target, we'll store the indexes in the int array we made earlier.
Since there's only one solution, we can short circuit the method by retuning the array.
7. answer time complexity
This algorithm is big O of n square because of the nested for loop who in the worst case we need to iterate n times n.
この記事が気に入ったらサポートをしてみませんか?