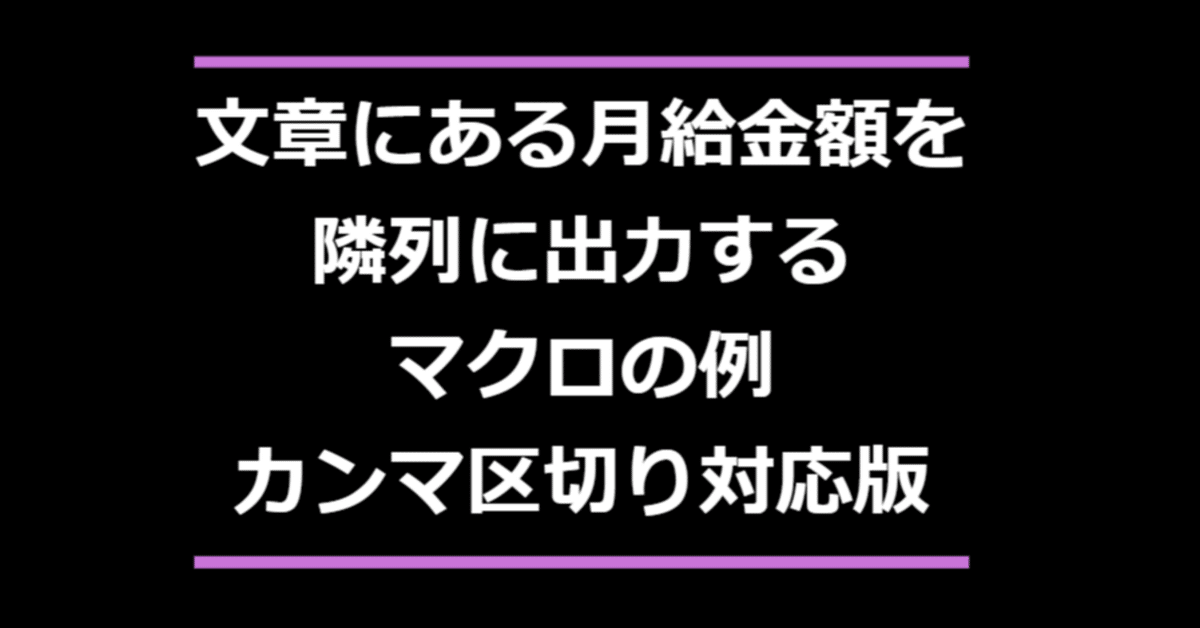
文章内にある月給をすべて隣列にだすよカンマ区切り3種対応
この説明は、ChatGPTで作成しています。
プロシージャの概要
このVBAプロシージャは、選択したセル範囲内にあるテキストの中から「月給」と記載された金額を探し出し、それを隣のセルに表示するものです。金額の書き方には3つのパターン(例:月給100000円、月給100,000円、月給10,000円)に対応しています。金額の横には「円」という文字がついていることを前提としています。
仕組みの説明
1. プロシージャの開始
最初に、`Application.ScreenUpdating = False`としています。これにより、このプログラムが動いている間は画面更新を一時的に止めて、動作をスムーズにし、処理速度を速めることができます。
2. 正規表現オブジェクトの作成
次に、`reg` と `regClean` という正規表現(特定のパターンに当てはまる文字列を探す方法)のオブジェクトを作成しています。これらは「VBScript.RegExp」という名前のツールを使って設定しています。
`reg` は月給のパターンを探すために使われます。
`regClean` は見つけた月給の中から数字以外の文字(例えば「円」やカンマ)を取り除くために使われます。
3. 月給パターンの設定
`With reg` の部分で、月給を見つけるためのルールを設定しています。
`.Pattern = "月給\d{6,}円|月給(\d{1,3}([,、.]\d{3})*)円"`
月給の後に6桁以上の数字が続くもの(例:月給1000000円)
または、月給の後にカンマやピリオドで区切られた数字が続くもの(例:月給1,000,000円)
このパターンに合うものが見つかると、その金額を取得することができます。
4. クリーニング用の設定
`With regClean` の部分では、数字以外の文字を取り除くように設定しています。
5. セルの選択範囲をループ
次に、`For Each myRng In Selection` で、選択したセルを一つずつチェックしていきます。
`txt = myRng.Value` で、セルの内容を `txt` という変数に入れます。
`Set match = reg.Execute(txt)` で、その内容に月給が含まれているかを確認します。
もし該当する月給があった場合、次のループ (`For i = 1 To match.count`) で見つかった月給を隣のセルに出力します。
6. 結果を隣のセルに出力
`myRng.Offset(0, i).Value = regClean.Replace(match(i - 1).Value, "")` で、見つかった月給を数字だけにして、選択したセルの右隣のセルに表示します。
7. 画面更新の再開
最後に、`Application.ScreenUpdating = True`で画面更新を再開します。これで、処理が完了したことがわかりやすくなります。
まとめ
このプログラムは、文章の中に「月給」という単語とそれに続く金額を探して、数字だけを隣のセルに表示してくれる便利なものです。例えば、求人情報がたくさん並んだリストから、月給の金額だけを簡単に抽出するのに使えます。
Sub 文章内にある月給をすべて隣列にだすよカンマ区切り3種対応()
Application.ScreenUpdating = False
Dim reg As Object, regClean As Object
Set reg = CreateObject("VBScript.RegExp") 'オブジェクト作成
Set regClean = CreateObject("VBScript.RegExp") ' クリーニング用のオブジェクト作成
Dim myRng As Range
Dim txt As String
Dim match As Object
Dim i As Long
' 時給パターンの正規表現
With reg
.Pattern = "月給\d{6,}円|月給(\d{1,3}([,、.]\d{3})*)円"
.IgnoreCase = True
.Global = True
End With
' クリーニング用の正規表現パターン
With regClean
.Pattern = "[^0-9]" ' 数字以外の文字をすべて取り除く
.IgnoreCase = True
.Global = True
End With
On Error Resume Next
For Each myRng In Selection
txt = myRng.Value
Set match = reg.Execute(txt)
For i = 1 To match.count
' matchの結果をクリーニングして隣のセルに出力
myRng.Offset(0, i).Value = regClean.Replace(match(i - 1).Value, "")
Next i
Next myRng
Application.ScreenUpdating = True
End Sub
このマクロの「メリット」
手作業の効率化: 大量のテキストデータから「月給」に関する情報を簡単に抜き出し、隣のセルに自動的に表示するため、手動で探す手間を省けます。例えば、求人情報などから給与情報を抽出する際に非常に便利です。
多様なフォーマットに対応: このマクロは「月給100000円」や「月給100,000円」、「月給10,000円」など、カンマや小数点を含む3種類のフォーマットに対応しているため、さまざまなデータ形式に対して柔軟に対応できます。
正規表現を活用: 正規表現を使っているため、金額のパターンを幅広く探すことができます。特定の文字列パターンに対して非常に強力であり、カンマの位置や数値の並びが異なるケースも正確に抽出できます。
Excel初心者でも使いやすい: 一度セットアップしておけば、Excelの基本的な操作ができる人でも簡単に利用できるため、プログラミング経験がなくても使えるのは大きなメリットです。
動作速度の向上: `Application.ScreenUpdating = False` により、処理中の画面更新を一時停止することで、動作がスムーズに行われます。これにより、大量のデータを処理する際にも高速に動作します。
このマクロの「デメリット」
特定の表現に依存: このマクロは「月給〇〇円」というフォーマットに依存しています。もし「月給」以外の言葉や、他の通貨記号・単位が使われている場合には抽出できません。
選択範囲に制限: このマクロは選択したセル範囲内のみを対象とします。データ範囲が広い場合、都度範囲を選択する必要があるため、特定の範囲に自動で適用するような工夫はされていません。
全角・半角の区別に注意: 正規表現のパターンによっては、全角や半角のカンマや数字などの違いに対応できないケースもあります。もしデータに全角・半角が混在していると、抽出できない部分が出るかもしれません。
エラーハンドリングが不十分: `On Error Resume Next` が使われているため、エラーが発生しても無視されて処理が進んでしまいます。このため、予期しない動作をする場合でも気づかない可能性があります。
初心者には正規表現が難しい: プログラミング初心者にとっては、正規表現の内容がやや難解かもしれません。パターンの修正や新しいフォーマットへの対応をする際に、正規表現の知識が必要となります。
まとめ
このマクロは、特定のフォーマットに沿った「月給」のデータを効率的に抽出するのに優れている一方で、フォーマットが異なるデータやエラー処理などには注意が必要です。
メリット・デメリットを踏まえた活用
メリットを最大限活かすためには、対象データのフォーマットが一定であることや、適切なセル範囲を選択することが重要です。デメリットに対しては、正規表現の知識を少し学ぶことで対処できる部分も多いでしょう。
キーワード
#excel #できること #vba #正規表現 #月給 #テキスト抽出 #データ解析 #データクリーニング #求人情報 #給与情報 #データ処理 #マクロ #VBAプログラム #オートメーション #VBScript #スクリーン更新 #エクセルマクロ #金額抽出 #文字列処理 #オフィス業務効率化
Extract Monthly Salary in Adjacent Cells - Comma-Separated 3 Types Supported
This explanation is created by ChatGPT.
Overview of the Procedure
This VBA procedure extracts all instances of the phrase "monthly salary" along with the corresponding amount from a selected range of cells and outputs them into the adjacent cell. It supports three patterns of salary formats (e.g., "monthly salary 100000 yen", "monthly salary 100,000 yen", "monthly salary 10,000 yen").
How It Works
1. Starting the Procedure
The procedure starts with `Application.ScreenUpdating = False` to temporarily pause screen updates while the macro runs, making the process more efficient and faster.
2. Creating Regular Expression Objects
Two regular expression objects, `reg` and `regClean`, are created using `CreateObject("VBScript.RegExp")`.
`reg` is used to detect patterns related to the monthly salary.
`regClean` is used to clean the extracted values, removing any non-numeric characters.
3. Setting Up the Monthly Salary Pattern
Within `With reg`, the pattern for identifying the salary is set up:
`.Pattern = "月給\d{6,}円|月給(\d{1,3}([,、.]\d{3})*)円"`
This detects amounts that have six or more digits following "monthly salary" (e.g., "monthly salary 1000000 yen").
It also matches amounts with commas or periods (e.g., "monthly salary 1,000,000 yen").
4. Setting Up the Cleaning Pattern
In `With regClean`, the setup removes any non-numeric characters from the extracted values.
5. Looping Through the Selected Cells
The loop `For Each myRng In Selection` iterates over each selected cell:
`txt = myRng.Value` extracts the cell's text.
`Set match = reg.Execute(txt)` searches for the "monthly salary" pattern in the text.
If any match is found, the next loop (`For i = 1 To match.count`) outputs the cleaned value into the adjacent cell.
6. Outputting Results to Adjacent Cells
The line `myRng.Offset(0, i).Value = regClean.Replace(match(i - 1).Value, "")` places the cleaned salary values into the adjacent cell.
7. Resuming Screen Updates
`Application.ScreenUpdating = True` resumes screen updates, indicating the completion of the process.
Summary
This procedure is handy for extracting "monthly salary" figures from blocks of text and placing only the numeric values in adjacent cells, making it useful for analyzing job listings or salary reports.
Keywords
#excel #capabilities #vba #regex #monthlysalary #textdataextraction #dataanalysis #datacleaning #joblisting #salaryinformation #dataprocessing #macros #VBAprogramming #automation #VBScript #screenupdating #excelmacros #salaryextraction #textprocessing #officeefficiency
この記事が気に入ったらサポートをしてみませんか?