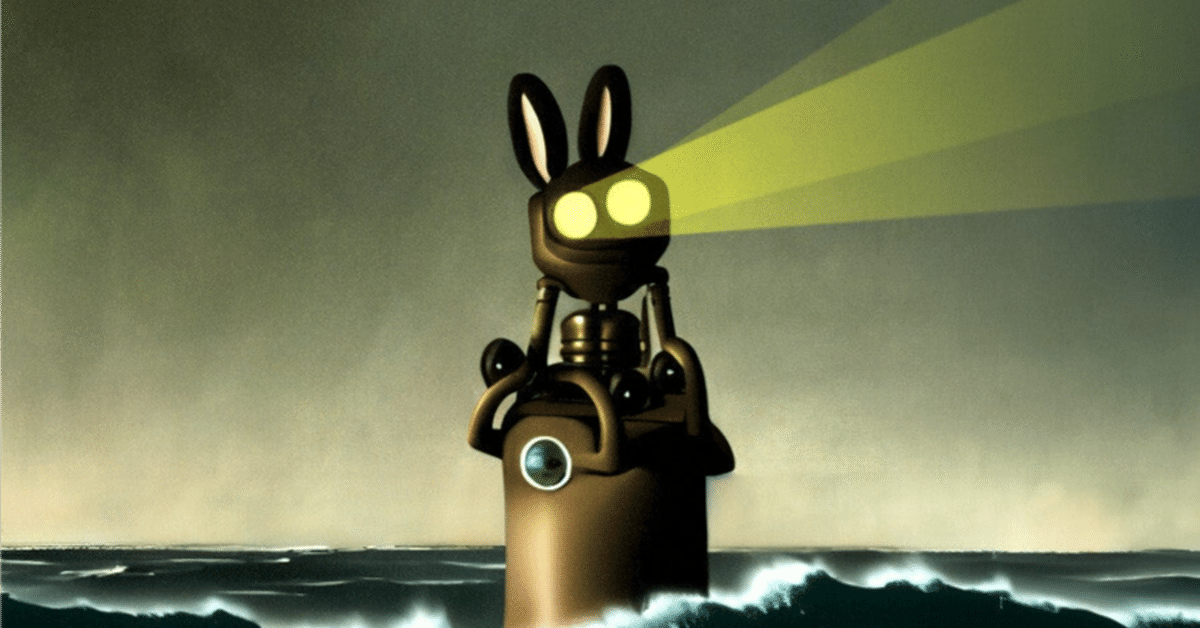
TypeScript 5.3がリリースされました!
本記事は Announcing TypeScript 5.3 のChatGPTによる要約です。
TypeScript 5.3がリリースされ、JavaScriptに型構文を追加し、型チェックを可能にするTypeScriptの機能が強化されました。
1. Import Attributes
最新のimport属性提案をサポートし、モジュールのフォーマット情報をランタイムに提供することが可能になりました。これにより、より具体的なモジュール解決が可能になります。
// We only want this to be interpreted as JSON,
// not a runnable/malicious JavaScript file with a `.json` extension.
import obj from "./something.json" with { type: "json" };
// TypeScript is fine with this.
// But your browser? Probably not.
import * as foo from "./foo.js" with { type: "fluffy bunny" };
// Dynamic import() calls can also use import attributes through a second argument.
const obj = await import("./something.json", {
with: { type: "json" }
});
2. resolution-modeの安定したサポート
import typeでresolution-mode属性をサポートし、requireまたはimportの意味論に基づいて特定の指定子が解決されるようになりました。
/// <reference types="pkg" resolution-mode="require" />
// or
/// <reference types="pkg" resolution-mode="import" />
// Resolve `pkg` as if we were importing with a `require()`
import type { TypeFromRequire } from "pkg" with {
"resolution-mode": "require"
};
// Resolve `pkg` as if we were importing with an `import`
import type { TypeFromImport } from "pkg" with {
"resolution-mode": "import"
};
export interface MergedType extends TypeFromRequire, TypeFromImport {}
// These import attributes can also be used on import() types.
export type TypeFromRequire =
import("pkg", { with: { "resolution-mode": "require" } }).TypeFromRequire;
export type TypeFromImport =
import("pkg", { with: { "resolution-mode": "import" } }).TypeFromImport;
export interface MergedType extends TypeFromRequire, TypeFromImport {}
3. resolution-modeの全モジュールモードでのサポート
resolution-modeはすべてのmoduleResolutionオプションで適切に動作し、型目的でモジュールを簡単に検索できるようになりました。
4. switch (true) Narrowing
switch (true)内の各case句に基づいて条件の絞り込みを行うことができるようになりました。
function f(x: unknown) {
switch (true) {
case typeof x === "string":
// 'x' is a 'string' here
console.log(x.toUpperCase());
// falls through...
case Array.isArray(x):
// 'x' is a 'string | any[]' here.
console.log(x.length);
// falls through...
default:
// 'x' is 'unknown' here.
// ...
}
}
5. ブール値との比較による絞り込み
trueまたはfalseとの直接的な比較を行う際、TypeScriptはこれらの表現を理解し、変数の絞り込みを行います。
interface A {
a: string;
}
interface B {
b: string;
}
type MyType = A | B;
function isA(x: MyType): x is A {
return "a" in x;
}
function someFn(x: MyType) {
if (isA(x) === true) {
console.log(x.a); // works!
}
}
6. instanceof Narrowing Through Symbol.hasInstance
JavaScriptのinstanceofオペレータの動作を上書きする機能をサポートし、型述語関数として宣言された[Symbol.hasInstance]メソッドが存在する場合、instanceofの左側のテスト値を適切に絞り込むようになりました。
class Weirdo {
static [Symbol.hasInstance](testedValue) {
// wait, what?
return testedValue === undefined;
}
}
// false
console.log(new Thing() instanceof Weirdo);
// true
console.log(undefined instanceof Weirdo);
interface PointLike {
x: number;
y: number;
}
class Point implements PointLike {
x: number;
y: number;
constructor(x: number, y: number) {
this.x = x;
this.y = y;
}
distanceFromOrigin() {
return Math.sqrt(this.x ** 2 + this.y ** 2);
}
static [Symbol.hasInstance](val: unknown): val is PointLike {
return !!val && typeof val === "object" &&
"x" in val && "y" in val &&
typeof val.x === "number" &&
typeof val.y === "number";
}
}
function f(value: unknown) {
if (value instanceof Point) {
// Can access both of these - correct!
value.x;
value.y;
// Can't access this - we have a 'PointLike',
// but we don't *actually* have a 'Point'.
value.distanceFromOrigin();
}
}
7. superプロパティアクセスに対するチェック
superキーワードを使用して基底クラスの宣言にアクセスする際、インスタンスフィールドに対するsuperアクセスがクラスフィールドに対応しているかどうかをTypeScriptがより詳細に検査するようになりました。
class Base {
someMethod() {
console.log("Base method called!");
}
}
class Derived extends Base {
someMethod() {
console.log("Derived method called!");
super.someMethod();
}
}
new Derived().someMethod();
// Prints:
// Derived method called!
// Base method called!
class Base {
someMethod() {
console.log("someMethod called!");
}
}
class Derived extends Base {
someOtherMethod() {
// These act identically.
this.someMethod();
super.someMethod();
}
}
new Derived().someOtherMethod();
// Prints:
// someMethod called!
// someMethod called!
class Base {
someMethod = () => {
console.log("someMethod called!");
}
}
class Derived extends Base {
someOtherMethod() {
super.someMethod();
}
}
new Derived().someOtherMethod();
// 💥
// Doesn't work because 'super.someMethod' is 'undefined'.
8. インタラクティブなInlay Hints
タイプの定義にジャンプするInlay Hintsをサポートし、コードのナビゲーションを容易にしました。
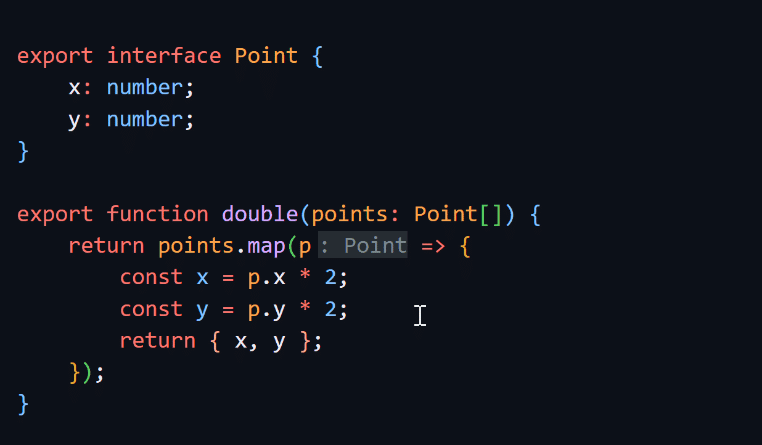
9. typeの自動インポートを好む設定
タイプ位置での自動インポートを生成する際に、type修飾子を追加する設定をエディタ固有のオプションとして可能にしました。
export let p: Person
import { Person } from "./types";
export let p: Person
import { type Person } from "./types";
export let p: Person
10. JSDoc解析のスキップによる最適化
tscを介してTypeScriptを実行する際にJSDocの解析を避け、パース時間の短縮、コメントの記憶によるメモリ使用量の削減、ガベージコレクションでの時間節約が可能になりました。
11. 正規化されていない交差による比較の最適化
交差と統合の特定形式を保ちつつ、タイプ間で交差と統合の特定形式を保ちつつ、タイプ間での比較をより効率的に行うことができるようになりました。
11. tsserverlibrary.jsとtypescript.jsの統合
TypeScriptはこれまでtsserverlibrary.jsとtypescript.jsの2つのライブラリファイルを提供していましたが、これらを統合し、typescript.jsがtsserverlibrary.jsの内容を含むようになり、パッケージサイズが約20.5%削減されました。
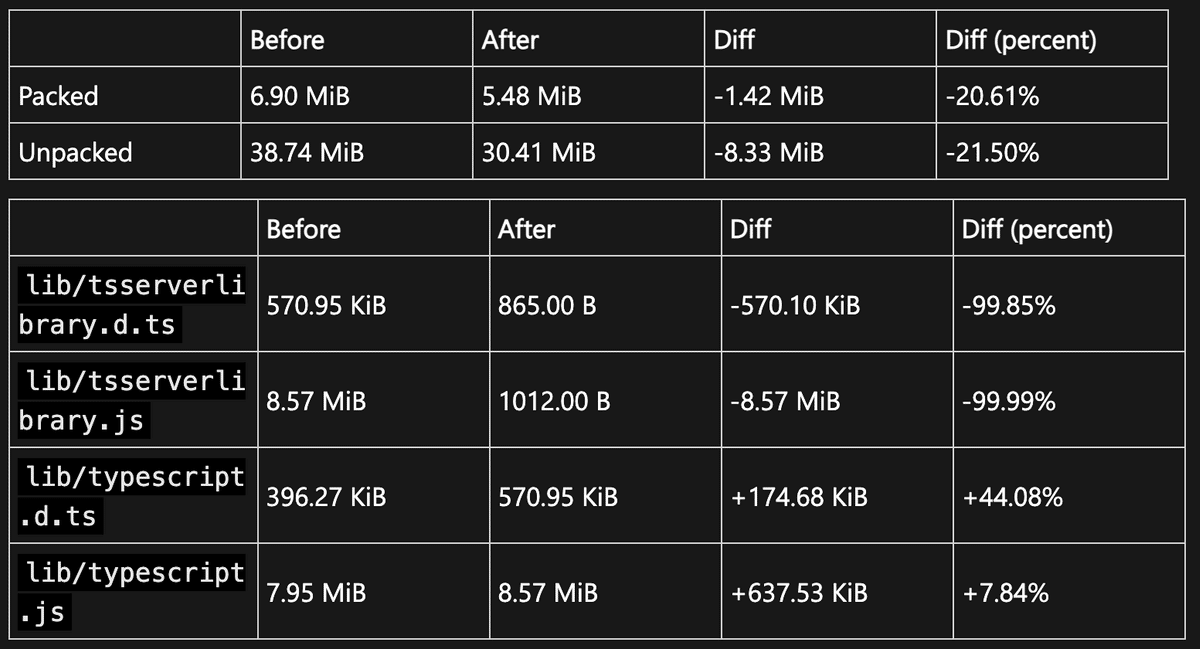
12. 破壊的変更と正確性の向上
DOMのために生成されたタイプがコードベースに影響を与える可能性があるため、superプロパティアクセスのチェックが改善され、クラスフィールドに対応するsuper.プロパティアクセスを参照する際にエラーを発生させるようになりました。
出典: https://devblogs.microsoft.com/typescript/announcing-typescript-5-3/
この記事が気に入ったらサポートをしてみませんか?