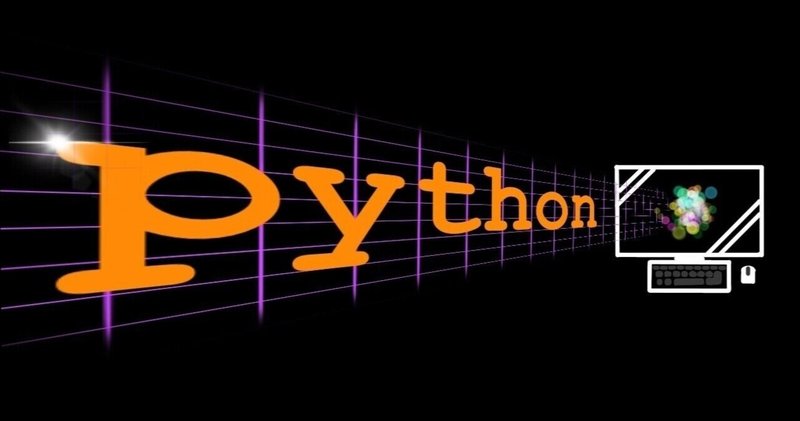
言語処理100本ノックから自然言語処理を始める 第1章 解答例参照
はじめに
言語処理100本ノックから自然言語処理を始める 第1章の解答例参照をしていきたいと思う。
00. 文字列の逆順
x = 'stressed'
print(x[::-1])
Output: desserts
ex[start: end: step]でやれたのに…自分はめっちゃ面倒くさい手順でやっちまった。
01. 「パタトクカシーー」
x = "パタトクカシーー"
print(x[::2])
Output: パトカー
00と同じく、ちなみにx[1::2]とするとタクシーが出てくる。start pointを1にずらしたからだ。
02. 「パトカー」+「タクシー」=「パタトクカシーー」
x = "パトカー"
y = "タクシー"
print(''.join([i + j for i, j in zip(x, y)]))
Output: パタトクカシーー
' '.join は単純連結を示す。例えば、 x = ['aaa','bbb','ccc']に' '.join(x)とするとaaabbbcccを返す。ちなみに','.join(x)だとaaa,bbb,cccを返す。
zip()関数は要素をまとめる関数。
03. 円周率
x = "Now I need a drink, alcoholic of course, after the heavy lectures involving quantum mechanics."
x = x.replace(',','').replace('.','')
print([len(w) for w in x.split()])
Output: [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5, 8, 9, 7, 9]
len(w) for w in x.split()とできるのか、自分のコードはfor文とappendで割と長くしてしまった…
04. 元素記号
s = "Hi He Lied Because Boron Could Not Oxidize Fluorine. New Nations Might Also Sign Peace Security Clause. Arthur King Can."
s = s.replace('.','')
idx = [1, 5, 6, 7, 8, 9, 15, 16, 19]
mp = {}
for i,w in enumerate(s.split()):
if (i+1) in idx:
v = w[:1]
else:
v = w[:2]
mp[v] = i+1
print (mp)
これは自分のコード大差なし。
05. n-gram
def ngram(S, n):
r = []
for i in range(len(S) - n + 1):
r.append(S[i:i+n])
return r
s = 'I am an NLPer'
print (ngram(s.split(),2))
print (ngram(s,2))
Output:
[['I', 'am'], ['am', 'an'], ['an', 'NLPer']]
['I ', ' a', 'am', 'm ', ' a', 'an', 'n ', ' N', 'NL', 'LP', 'Pe', 'er']
単語のbi-gramはスペース抜きだと思ったがそのままでいいらしい。
06. 集合
ef ngram(S, n):
r = []
for i in range(len(S) - n + 1):
r.append(S[i:i+n])
return r
s1 = 'paraparaparadise'
s2 = 'paragraph'
st1 = set(ngram(s1, 2))
st2 = set(ngram(s2, 2))
print(st1 | st2)
print(st1 & st2)
print(st1 - st2)
print('se' in st1)
print('se' in st2)
さらに,’se’というbi-gramがXおよびYに含まれるかどうかを調べよ。という部分を忘れていた。。。
07. テンプレートによる文生成
def sentence(x, y, z):
print(str(x)+"時の"+str(y)+"は"+str(z))
x=12
y="気温"
z=22.4
sentence(x,y,z)
これは特になし。
08. 暗号文
def cipher(S):
new = []
for s in S:
if 97 <= ord(s) <= 122:
s = chr(219 - ord(s))
new.append(s)
return ''.join(new)
s = 'I am an NLPer'
new = cipher(s)
print (new)
print (cipher(new))
これは、前回わからずに確認したところ。
09. Typoglycemia
import random
s = 'I couldn’t believe that I could actually understand what I was reading : the phenomenal power of the human mind .'
ans = []
text = s.split()
for word in text:
if (len(word)>4):
mid = list(word[1:-1])
random.shuffle(mid)
word = word[0] + ''.join(mid) + word[-1]
ans.append(word)
else:
ans.append(word)
print (' '.join(ans))
random.shuffle使えば楽だった、わざわざ重複なしのランダムプログラムを作る必要性はなかった。
最後に
自分が20行くらいで書いたコードが5行とかで書かれてるとなんか悲しいよね。、と同時にこうすれば良いのかってことがわかって学んだことは多い。次は、第二章のUNIXコマンド。これも楽しみ、どんな問題がでるんだろう。
参照したサイト:
https://kakedashi-engineer.appspot.com/2020/05/09/nlp100-ch1/
https://qiita.com/yamaru/items/6d66445dbd5e7cef8640
この記事が気に入ったらサポートをしてみませんか?