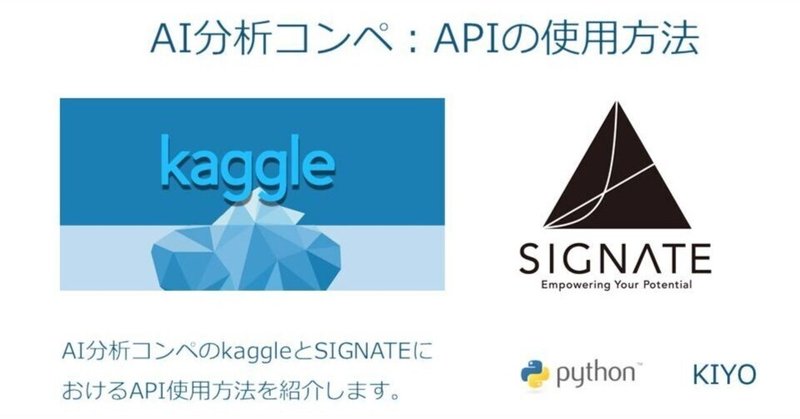
AI分析コンペ:APIの使用方法
1.概要
分析コンペとは与えられた課題に対してAI・機械学習を用いて問題を解くコンペであり下記のようなものがあります。
【AI分析コンペのプラットフォーム】
●Kaggle:一番有名であり世界中の企業や研究機関がコンペを開催している。
●SIGNATE:国産プラットフォームであり参加者は日本人がメイン
●Numerai:株式市場の予測モデルがメイン(2015年設立)
●TopCoder:基本的はプログラミングコンテストがメイン
今回はKaggleとSIGNATEのAPIを使用して情報確認やデータDLを説明します。注意点は下記の通りです。
【注意点】
●ブラウザから各情報は取得できるため覚えなくてもコンペは参加できます。
●APIの使用前にアカウント取得が必要となります。
2.自作モジュールのまとめ(自分用)
3章以降で説明する処理をモジュール化して自分が使用しやすいようにまとめました。
2-1.Kaggle用
フォルダ構成および機能一覧(kaggleAPI.pyの中身は別添参照)※kaggleモジュールのversionは最新のやつ
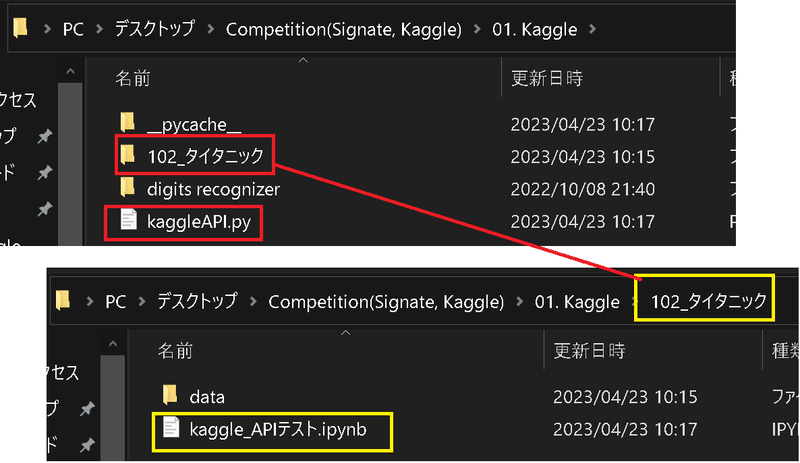
作業ディレクトリの親フォルダにモジュール(kaggleAPI.py)を保存しているためsysで環境変数を追加
kaggle APIの①コンペ一覧取得、②ファイル一覧取得、③ファイルのDL、④提出ファイルの確認機能をモジュールに追加(提出は手動予定)
得られた結果をDataFrameにして出力
参考としてTitanicで実行してみました。
[IN]
import sys
import os
import pandas as pd
class HorizontalDisplay:
def __init__(self, *args):
self.args = args
def _repr_html_(self):
template = '<div style="float: left; padding: 10px;">{}</div>'
return '\n'.join(template.format(a._repr_html_()) for a in self.args)
def add_parent_directory_to_path():
parent_directory = os.path.dirname(os.getcwd()) # 親ディレクトリのパスを取得
if parent_directory not in sys.path: # 既に追加されているかどうかを確認
sys.path.append(parent_directory) # Pathに追加
print(f'Path追加:{parent_directory}')
add_parent_directory_to_path()
import kaggleAPI
df_competitions = kaggleAPI.compList()
PJname = df_competitions.loc[12, 'PJname'] #Titanicを文字列で抽出 ※手打ちもOK
print(f'PJname:{PJname}')
df_filenames = kaggleAPI.get_filenames(PJname) #PJで使用するファイル名の取得
kaggleAPI.DL_files(PJname) # ファイルのダウンロード
df_kaggleresults = kaggleAPI.get_PJresults(PJname) # kaggleの結果を取得
display(HorizontalDisplay(df_competitions, df_filenames, df_kaggleresults))
[OUT]
Path追加:c:\Users\KIYO\Desktop\Competition(Signate, Kaggle)\01. Kaggle
PJname:titanic
Files for titanic already exist. No download needed.
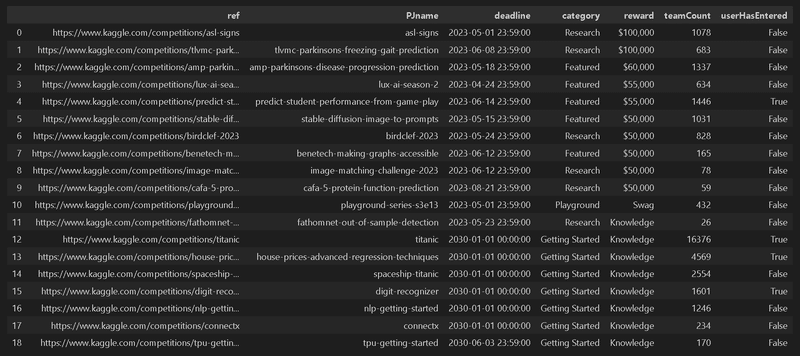
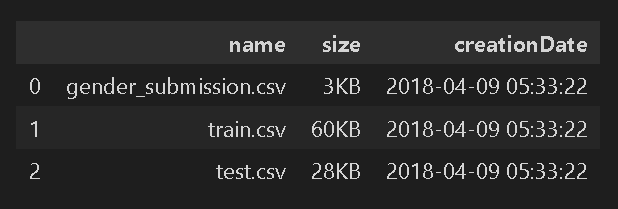

3.Kaggle API
基本的に公式に従ってやっていきます。
pip install kaggle
3-1.環境構築
まずは「APIトークン」を発行します。「アカウント設定」->「Create New API Token」を押すとkaggle.jsonがダウンロードされます。

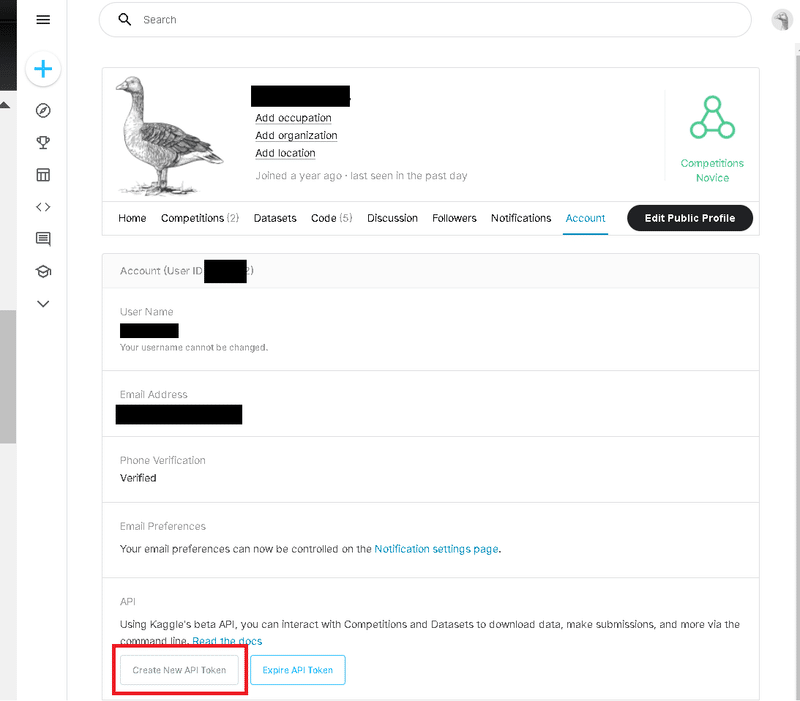
ホームディレクトリに".kaggleフォルダ"を作成して、その中にjsonファイルを入れたら完了です。

気になる人はchmodコマンドによる権限設定でファイルを触れる人を制限します。
3-2.コンペ一覧取得:kaggle competitions list
コンペの終了日が遅い順に20件分の一覧を取得するには”kaggle competitions list”を使用します。(Jupyter使用時は頭に!をつけます)
[In]
!kaggle competitions list
[Out]
ref deadline category reward teamCount userHasEntered
--------------------------------------------- ------------------- --------------- --------- --------- --------------
contradictory-my-dear-watson 2030-07-01 23:59:00 Getting Started Prizes 76 False
gan-getting-started 2030-07-01 23:59:00 Getting Started Prizes 116 False
store-sales-time-series-forecasting 2030-06-30 23:59:00 Getting Started Knowledge 851 False
tpu-getting-started 2030-06-03 23:59:00 Getting Started Knowledge 171 False
digit-recognizer 2030-01-01 00:00:00 Getting Started Knowledge 1831 False
titanic 2030-01-01 00:00:00 Getting Started Knowledge 14044 True
house-prices-advanced-regression-techniques 2030-01-01 00:00:00 Getting Started Knowledge 4876 True
connectx 2030-01-01 00:00:00 Getting Started Knowledge 225 False
nlp-getting-started 2030-01-01 00:00:00 Getting Started Knowledge 872 False
competitive-data-science-predict-future-sales 2022-12-31 23:59:00 Playground Kudos 13468 True
ubiquant-market-prediction 2022-04-18 23:59:00 Featured $100,000 780 False
feedback-prize-2021 2022-03-15 23:59:00 Featured $160,000 1059 False
tensorflow-great-barrier-reef 2022-02-14 23:59:00 Research $150,000 1856 False
jigsaw-toxic-severity-rating 2022-02-07 23:59:00 Featured $50,000 2217 False
g-research-crypto-forecasting 2022-02-01 23:59:00 Featured $125,000 2100 True
tabular-playground-series-jan-2022 2022-01-31 23:59:00 Playground Kudos 1523 False
petfinder-pawpularity-score 2022-01-14 23:59:00 Research $25,000 3537 False
santa-2021 2022-01-12 23:59:00 Featured $25,000 867 False
optiver-realized-volatility-prediction 2022-01-10 18:36:00 Featured $100,000 3852 False
nfl-big-data-bowl-2022 2022-01-06 23:59:00 Analytics $100,000 0 False
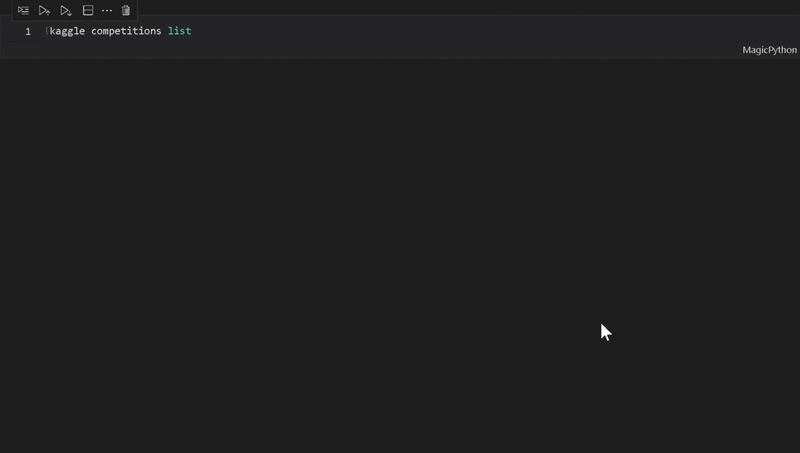
3-3.コンペのファイル一覧を取得:files -c ref
参加したいコンペのファイル一覧(学習用・テストデータセット、提出サンプル)の取得は”kaggle competitions files -c コンペ名”です。
[In]
!kaggle competitions files -c titanic
[Out]
name size creationDate
--------------------- ---- -------------------
test.csv 28KB 2018-04-09 05:33:22
gender_submission.csv 3KB 2018-04-09 05:33:22
train.csv 60KB 2018-04-09 05:33:22
3-4.ファイルのダウンロード:download -c ref
ファイルのダウンロードは”kaggle competitions download -c コンペ名”です。参考までにzip解凍のコードもつけました。
[In]
!kaggle competitions download -c titanic
import glob
import os
import zipfile
zipfiles = glob.glob('*.zip')
for file in zipfiles:
with zipfile.ZipFile(file, 'r') as f:
f.extractall(f'./{file[:-4]}')
print(f'{file}を解凍しました')
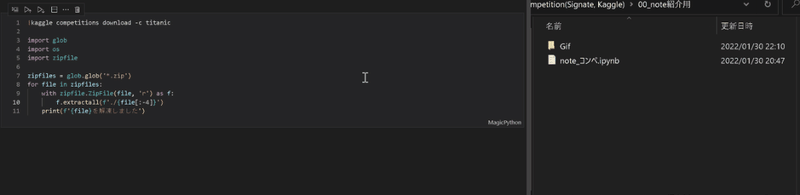
3-5.ファイルの提出
作成したファイルの提出は”!kaggle competitions submit -c コンペ名 -f 提出ファイルパス -m コメント”でできます。
[In]
!kaggle competitions submit -c titanic -f titanic/gender_submission.csv -m note用として提出

3-6.提出ファイルの確認
過去に提出した結果の一覧は”!kaggle competitions submissions -c コンペ名”で確認できます。
[In]
!kaggle competitions submissions -c titanic
[Out]
fileName date description status publicScore privateScore
--------------------- ------------------- ---------------------------------------------- -------- ----------- ------------
gender_submission.csv 2022-01-30 13:34:27 note用として提出 complete 0.76555 None
4.SIGNATE API
基本的に公式に従ってやっていきます。
pip install signate
4-1.環境構築
公式通り下記手順で実施します。
【手順】
1.SIGNATE でアカウント登録
2.アカウント設定 画面の "API Token" の "作成" をクリック(下図参照)
3."新規作成" をクリックしてAPI Token(signate.json)を取得
4.取得したAPI Tokenを ~/.signate直下に配置(下図参照)
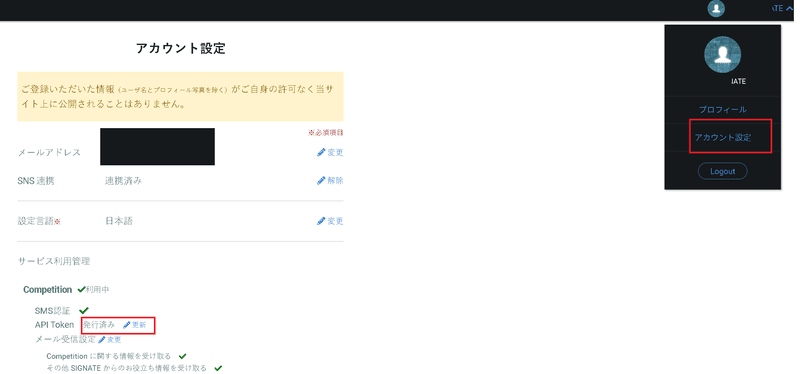
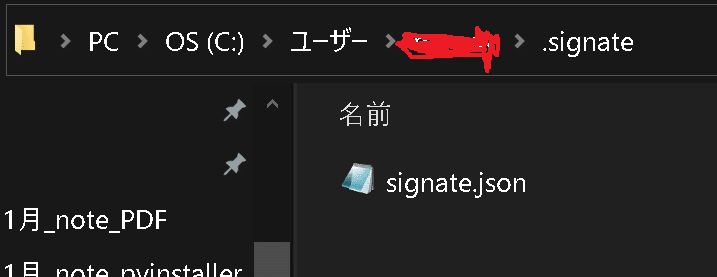
【動作チェック】
インストール後に"signate --help"でヘルプ表示を確認します。(ターミナルではそのままですがJupyter使用時は頭に!をつけます)
[In]※ターミナルでの記法:以下はJupyter記法で記載します。
signate --help
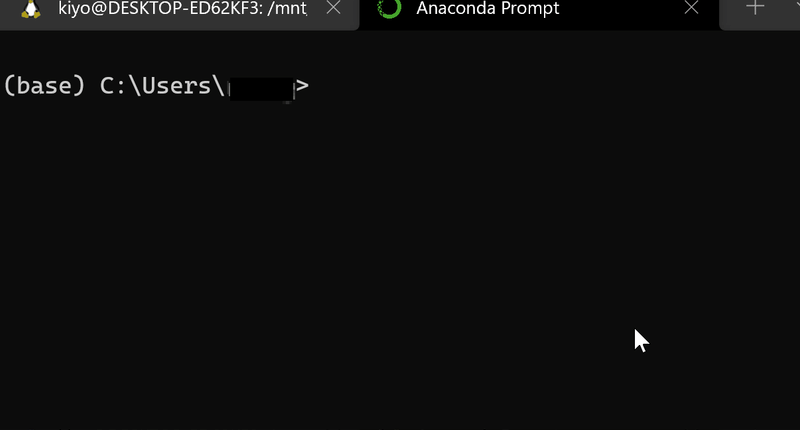
4-2.コンペ一覧の取得:signate list
”signate list”でid, タイトル、終了日、賞金、提出者数が確認できます。
[In]
!signate list
[Out]
competitionId title closing prize submitters
--------------- ---------------------------------------------------------------------------------------- ---------- ------------------- ------------
1 【練習問題】銀行の顧客ターゲティング - 5423
24 【練習問題】お弁当の需要予測 - 6716
27 【練習問題】Jリーグの観客動員数予測 - 1652
100 【練習問題】手書き文字認識 - Knowledge 205
102 【練習問題】タイタニックの生存予測 - Knowledge 1284
103 【練習問題】音楽ラベリング - Knowledge 62
104 【練習問題】スパムメール分類 - Knowledge 139
105 【練習問題】毒キノコの分類 - Knowledge 271
106 【練習問題】アワビの年齢予測 - Knowledge 368
107 【練習問題】国勢調査からの収入予測 - Knowledge 548
108 【練習問題】画像ラベリング(20種類) - Knowledge 226
112 【練習問題】ワインの品種の予測 - Knowledge 318
113 【練習問題】山火事の消失面積予測 - Knowledge 206
114 【練習問題】レンタル自転車の利用者数予測 - Knowledge 388
115 【練習問題】アヤメの分類 - Knowledge 355
116 【練習問題】活動センサーログからの動作予測 - Knowledge 52
118 【練習問題】テニスの試合結果の予測 - Knowledge 127
121 【練習問題】自動車の走行距離予測 - Knowledge 1481
122 【練習問題】自動車の評価 - Knowledge 315
123 【練習問題】オゾンレベルの分類 - Knowledge 48
124 【練習問題】ボットの判別 - Knowledge 309
125 【練習問題】ガラスの分類 - Knowledge 163
126 【練習問題】林型の分類 - Knowledge 41
127 【練習問題】ゲーム選手のリーグ分類 - Knowledge 60
128 【練習問題】ステンレス板の欠陥分類 - Knowledge 68
129 【練習問題】都市サイクルの燃料消費量予測 - Knowledge 130
130 【練習問題】天秤のバランス分類 - Knowledge 105
132 【練習問題】ネット広告のクリック予測 - Knowledge 145
133 【練習問題】画像ラベリング(10種類) - 352
135 【練習問題】ネット画像の分類 - 74
262 【SOTA】国立国会図書館の画像データレイアウト認識 2100-12-31 - 74
263 【SOTA】産業技術総合研究所 衛星画像分析コンテスト 2100-12-31 - 45
264 【SOTA】マイナビ × SIGNATE Student Cup 2019: 賃貸物件の家賃予測 2100-12-31 - 373
265 【練習問題】健診データによる肝疾患判定 - 516
266 【練習問題】民泊サービスの宿泊価格予測 - 577
267 【SOTA】海洋研究開発機構 熱帯低気圧(台風等)検出アルゴリズム作成 2100-12-31 - 17
268 【SOTA】オプト レコメンドエンジン作成 2100-12-31 39
269 【SOTA】アップル 引越し需要予測 2100-12-31 - 334
270 【SOTA】Weather Challenge:雲画像予測 2100-12-31 - 8
271 【SOTA】JR西日本 走行中の北陸新幹線車両台車部の着雪量予測 2100-12-31 - 21
288 【SOTA】Sansan 名刺の項目予測 2100-12-31 - 30
294 【練習問題】債務不履行リスクの低減 - 192
358 【練習問題】機械稼働音の異常検知 - 73
404 【練習問題】モノクロ顔画像の感情分類 - 78
406 【練習問題】鋳造製品の欠陥検出 - 194
409 【練習問題】株価の推移予測 - 177
537 第5回AIエッジコンテスト(実装コンテスト③) 2022-02-15 total 1,600,000 yen 16
563 SUBARU 画像認識チャレンジ 2022-01-31 総額¥2,000,000 146
565 【SOTA】SIGNATE Student Cup 2021春:楽曲のジャンル推定チャレンジ!! 2100-12-31 - 36
567 【SOTA】SIGNATE Student Cup 2021秋:オペレーション最適化に向けたシェアサイクルの利用予測 2100-12-31 - 29
575 【第18回_Beginner限定コンペ】PCゲームの勝敗予測 2022-01-31 130
4-3.ファイル一覧を取得(確認のみ):signate files
見たいコンペにどのようなファイルがあるかを"signate files --competition-id=<competition-id> "で確認します。
[In]
!signate files --competition-id=575
[Out]
fileId name title size updated_at
-------- ----------------- -------------------------------- ------ -------------------
2510 train.csv 学習用データ 158733 2021-12-23 10:05:37
2511 test.csv 評価用データ 149993 2021-12-23 10:05:55
2512 sample_submit.csv サンプルサブミッション用ファイル 39398 2021-12-23 10:06:32
4-4.ファイルのダウンロード:signate download
参加するコンペで使用するファイルは”signate download --competition-id=<competition-id> ”で取得可能です。
※本機能は事前にコンペへの参加同意が必要
[In]
import os
print(os.getcwd()) #ファイルが作成されるディレクトリを確認
!signate download --competition-id=575
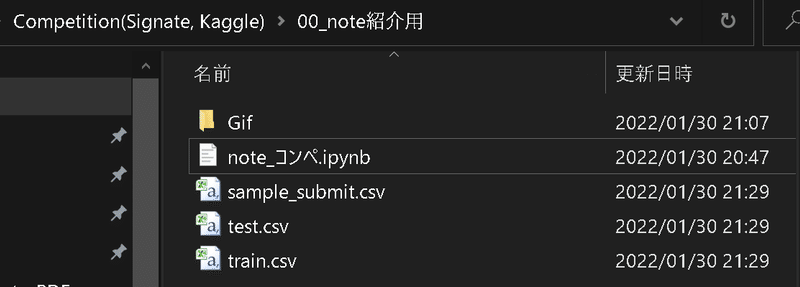
4-5.ファイルの提出:signate submit
予測結果をコンペに提出する時は”signate submit --competition-id=<competition-id> <結果ファイルのパス>”です。フラグとして"--note コメント"とすることでコメントも残すことが出来ます。
[In]
!signate submit --competition-id=575 sample_submit.csv --note note記事用として投稿
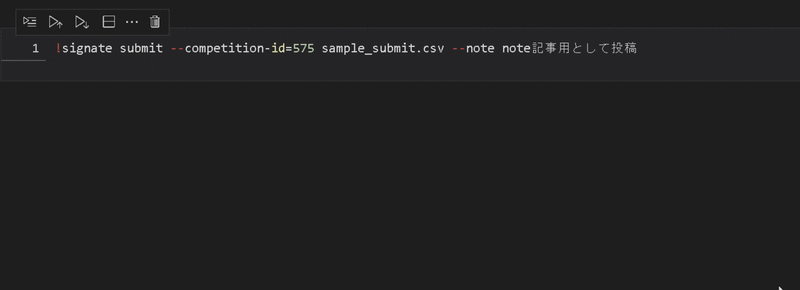
提出できたら結果はメールで飛んできますしアカウント画面からも確認できます。
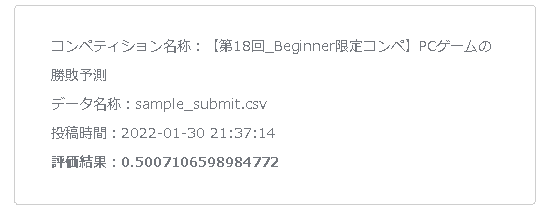
5.Nishika
APIは多分なし(あれば追記)
6.別添:自作モジュール
2章で使用した自作モジュールの紹介
6-1.Kaggle
[kaggleAPI.py]
import subprocess
import pandas as pd
from io import StringIO
import os
import shutil
import zipfile
'''
kaggle competitions list:コンペ一覧を取得
kaggle competitions files -c <competition>:コンペのファイル一覧を取得
kaggle competitions download -c <competition>:コンペのファイルをダウンロード
'''
#kaggle competitions listの取得
def compList(verbose=False):
result = subprocess.run(["kaggle", "competitions", "list"], capture_output=True, text=True)
output = result.stdout
# Warningメッセージと表の部分を分離
output_lines = output.split("\n")
warning_msg = output_lines[0]
table_data = "\n".join(output_lines[2:])
# Warningメッセージの出力
if verbose:
print(warning_msg)
# Pandasで表の部分を表示
df = pd.read_csv(StringIO(table_data), sep='\s\s+', engine='python')
# カラム名の調整
df.columns = ['ref', 'deadline', 'category', 'reward', 'teamCount', 'userHasEntered']
# refの最後の文字列を取得し、新たなカラムPJnameを作成
df['PJname'] = df['ref'].apply(lambda x: x.split('/')[-1])
# PJname列をrefの隣に追加
cols = df.columns.tolist()
cols.insert(1, cols.pop(cols.index('PJname')))
df = df[cols]
return df
#kaggle competitions files -c <competition>の取得
def get_filenames(competition_name):
result = subprocess.run(["kaggle", "competitions", "files", "-c", competition_name], capture_output=True, text=True)
output = result.stdout
# 線を除外した表の部分を取得
output_lines = output.split("\n")
table_data = "\n".join(line for line in output_lines if not line.startswith('-'))
# Pandasで表の部分を表示
df = pd.read_csv(StringIO(table_data), sep='\s\s+', engine='python')
# カラム名の調整
df.columns = ['name', 'size', 'creationDate']
return df
def DL_files(competition_name):
# 1. "data"フォルダがあるか確認して、なければフォルダ作成
if not os.path.exists("data"):
os.makedirs("data")
# 2. "data"フォルダ内にget_filenamesで取得したファイルが存在するか確認
existing_files = os.listdir("data")
competition_files_df = get_filenames(competition_name)
competition_files = competition_files_df['name'].tolist() # ファイル名のみのリストを作成
missing_files = [file for file in competition_files if file not in existing_files]
# 3. ファイルがなければdataフォルダにファイルを保存
if missing_files:
subprocess.run(["kaggle", "competitions", "download", "-c", competition_name])
# 4. 処理後に作業ディレクトリにプロジェクト名と同じ名前のzipフォルダが生成される。
# zipでファイルの中身をdataフォルダに移動させて、ダウンロードしたzipフォルダを削除
with zipfile.ZipFile(f"{competition_name}.zip", "r") as zip_ref:
zip_ref.extractall("data")
os.remove(f"{competition_name}.zip")
print(f"Downloaded:{competition_name}.")
else:
print(f"Files for {competition_name} already exist. No download needed.")
def get_PJresults(competition_name):
result = subprocess.run(["kaggle", "competitions", "submissions", "-c", competition_name],
capture_output=True, text=True, encoding='utf-8')
output = result.stdout
# 表の部分を取得
output_lines = output.split("\n")
table_data = "\n".join(line for line in output_lines if not line.startswith('-'))
# Pandasで表の部分を表示
df = pd.read_csv(StringIO(table_data), sep='\s\s+', engine='python')
# カラム名の調整
df.columns = ['fileName', 'date', 'description', 'status', 'publicScore', 'privateScore']
return df
あとがき
メインは提出用に使いたいので他はおまけ。
とりあえずコンペ参加の準備できたけど再度優先順位を決めないと何もできなくなりそう・・・・
この記事が気に入ったらサポートをしてみませんか?