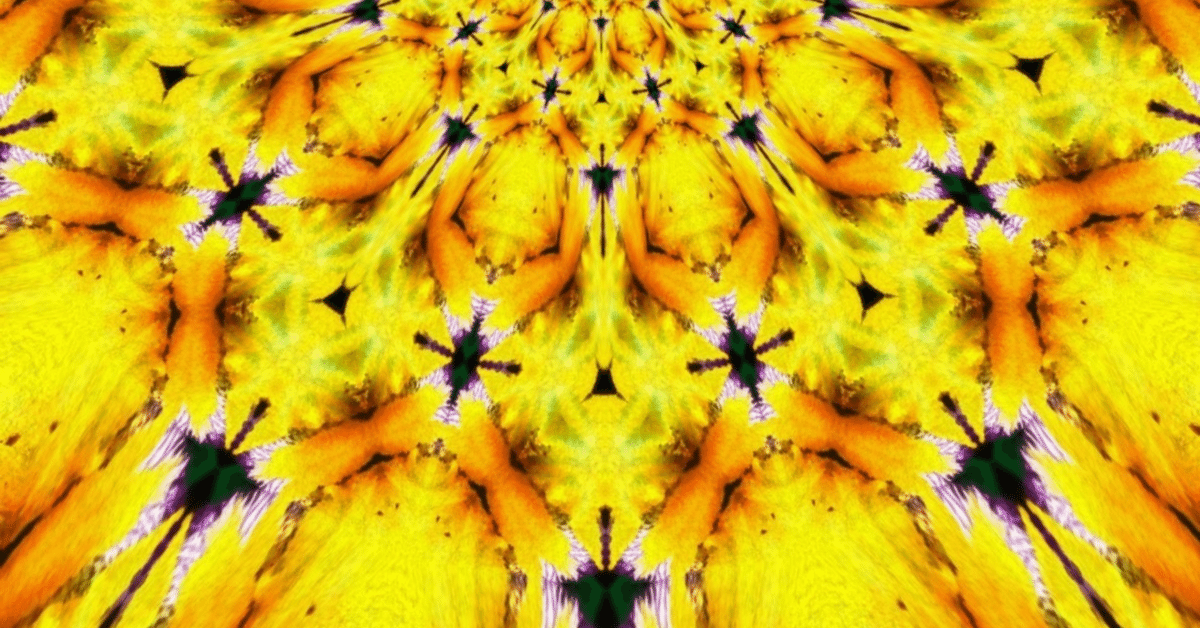
Photo by
cinemakicks
久々の電子工作
久々の電子工作
夏の暑い中、家で過ごす日が多くなり、中断していた電子工作を再開することにしました。
とりあえず、あつい日が続くので、手持ちの部品を利用して温度、気圧を測定してみよう。
とりあえず、センサーから温度、気圧を取得し、ディスプレイに表示。
次に、Ambient(IoTデータの可視化サービスです)を利用して可視化をためす。
ネットの記事を見ながら、なんとかできたかな。覚書として残しておこう。
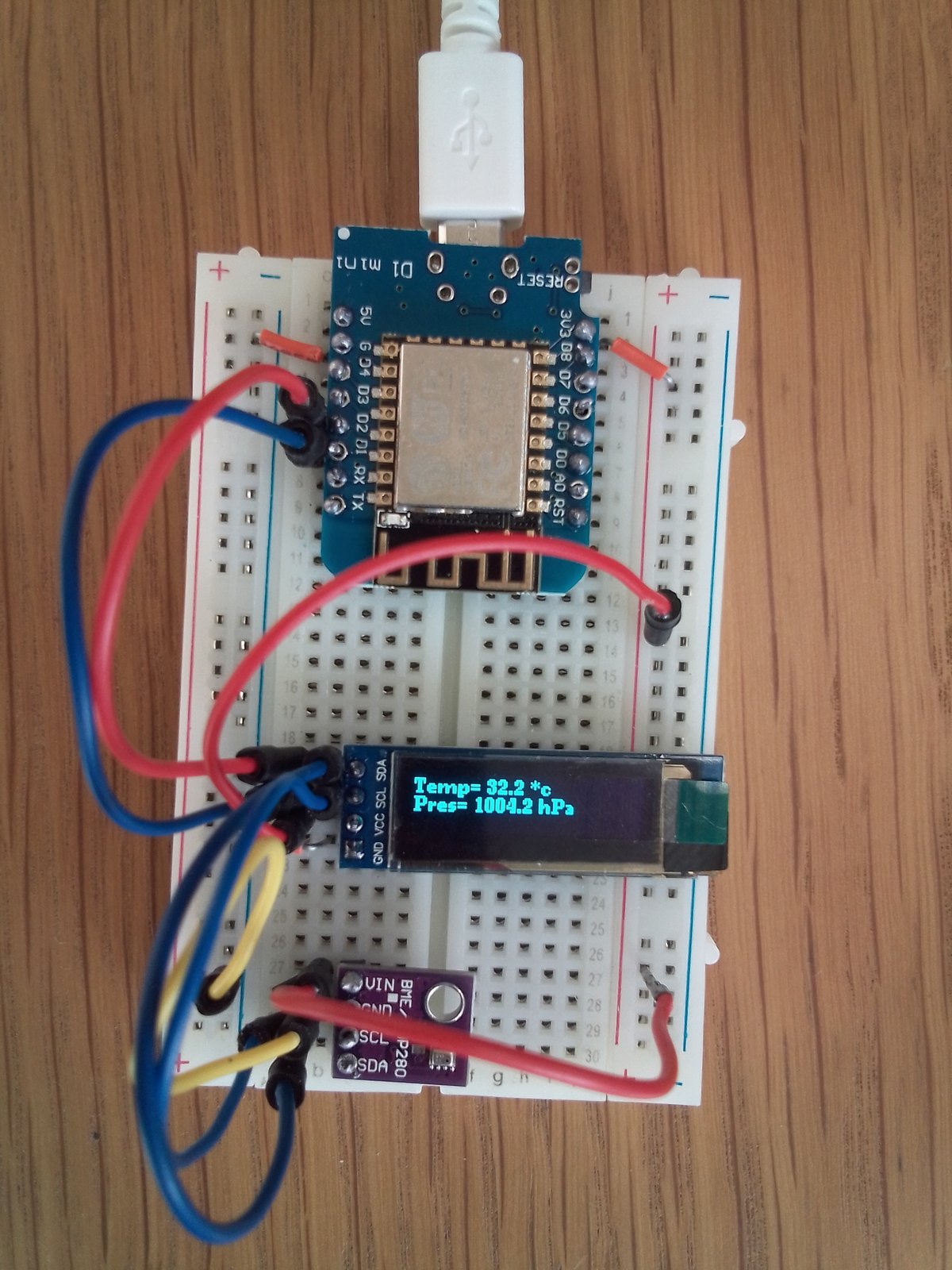
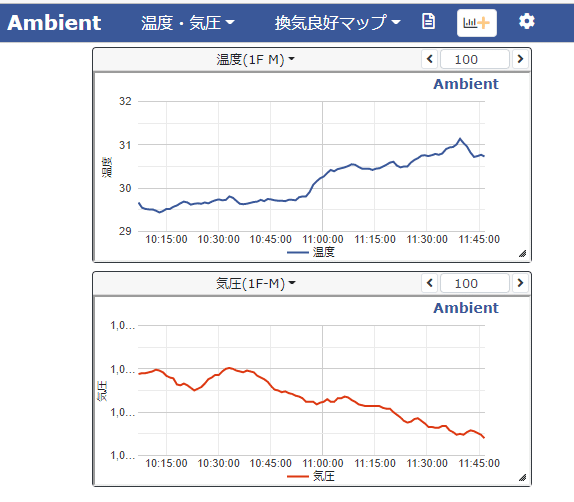
Aideepen WeMos D1 Mini NodeMcu WiFi Development Board ESP8266 CH340G Module with Micro USB, ESP-12F 3.3V 500mA for 4M Byte
BMP280
U8G2_SSD1306_128X32
/***************************************************************************
This is a library for the BMP280 humidity, temperature & pressure sensor
Designed specifically to work with the Adafruit BMP280 Breakout
----> http://www.adafruit.com/products/2651
These sensors use I2C or SPI to communicate, 2 or 4 pins are required
to interface.
Adafruit invests time and resources providing this open source code,
please support Adafruit andopen-source hardware by purchasing products
from Adafruit!
Written by Limor Fried & Kevin Townsend for Adafruit Industries.
BSD license, all text above must be included in any redistribution
***************************************************************************/
#include <Wire.h>
#include <SPI.h>
#include <U8g2lib.h>
#include <ESP8266WiFi.h>
#include "Ambient.h"
#include <Adafruit_BMP280.h>
#define BMP_SCK (13)
#define BMP_MISO (12)
#define BMP_MOSI (11)
#define BMP_CS (10)
Adafruit_BMP280 bmp; // I2C
//Adafruit_BMP280 bmp(BMP_CS); // hardware SPI
//Adafruit_BMP280 bmp(BMP_CS, BMP_MOSI, BMP_MISO, BMP_SCK);
int Alt = 22.7; //標高
float temp = 0.0;
float pres = 0.0;
unsigned long delayTime;
U8G2_SSD1306_128X32_UNIVISION_F_HW_I2C u8g2(U8G2_R0, /* reset=*/ U8X8_PIN_NONE);
//----------------------------------------------
//WiFi
const char* ssid = "**********";
const char* password = "**********";
//Ambient
unsigned int channelId = **********;
const char* writeKey = "**********";
WiFiClient client;
Ambient ambient;
//----------------------------------------------
void setup() {
Serial.begin(9600);
// wifi初期化
WiFi.begin(ssid, password);
Serial.println("WiFi start.");
// Wait for connection
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
// チャネルIDとライトキーを指定してAmbientの初期化
ambient.begin(channelId, writeKey, &client);
Serial.println("Ambient start.");
while ( !Serial ) delay(100); // wait for native usb
Serial.println(F("BMP280 measure"));
unsigned status;
//status = bmp.begin(BMP280_ADDRESS_ALT, BMP280_CHIPID);
status = bmp.begin(0x76);
if (!status) {
Serial.println(F("Could not find a valid BMP280 sensor, check wiring or "
"try a different address!"));
Serial.print("SensorID was: 0x"); Serial.println(bmp.sensorID(),16);
Serial.print(" ID of 0xFF probably means a bad address, a BMP 180 or BMP 085\n");
Serial.print(" ID of 0x56-0x58 represents a BMP 280,\n");
Serial.print(" ID of 0x60 represents a BME 280.\n");
Serial.print(" ID of 0x61 represents a BME 680.\n");
while (1) delay(10);
}
u8g2.begin();
delayTime = 1000*60;
}
//void draw(void) {
// /* Default settings from datasheet. */
// bmp.setSampling(Adafruit_BMP280::MODE_NORMAL, /* Operating Mode. */
// Adafruit_BMP280::SAMPLING_X2, /* Temp. oversampling */
// Adafruit_BMP280::SAMPLING_X16, /* Pressure oversampling */
// Adafruit_BMP280::FILTER_X16, /* Filtering. */
// Adafruit_BMP280::STANDBY_MS_500); /* Standby time. */
//}
void loop() {
// BMP280 Read
measure ();
//値をシリアルにプリント
printValues();
//値をDSP
printScreenValues();
// Ambientに連携
sendAmbient ();
// 送信間隔
delay(delayTime);
}
void printScreenValues() {
char result1[8];
char result2[8];
char result3[8];
char str1[20]="Temp= ";
char str2[20]="Pres= ";
char str3[20]="Humi= ";
char unit1[4]=" *c";
char unit2[5]=" hPa";
char unit3[3]=" %";
u8g2.clearBuffer(); // clear the internal memory
u8g2.setFont(u8g2_font_ncenB08_tr); // choose a suitable font
//u8g2.drawStr(0,10,"Hello World!");
dtostrf(temp, 1, 1, result1);
strcat(str1, result1);
strcat(str1, unit1);
u8g2.drawStr(0,10,str1);
dtostrf(pres, 2, 1, result2);
strcat(str2, result2);
strcat(str2, unit2);
u8g2.drawStr(0,20,str2);
// dtostrf(bme.readHumidity(), 3, 1, result3);
// strcat(str3, result3);
// strcat(str3, unit3);
// u8g2.drawStr(0,30,str3);
u8g2.sendBuffer(); // transfer internal memory to the display
}
void printValues() {
Serial.print("Temperature = ");
Serial.print(temp);
Serial.println(" *C");
Serial.print("Pressure = ");
Serial.print(pres);
Serial.println(" hPa");
// Serial.print("Humidity = ");
// Serial.print(bme.readHumidity());
// Serial.println(" %");
Serial.println();
}
void measure (){
pres = bmp.readPressure() / 100;
pres = pres/(pow((1-((0.0065*Alt)/(bmp.readTemperature()+0.0065*Alt+273.15))),5.257)); // 補正
temp = bmp.readTemperature();
}
void sendAmbient (){
ambient.set(1, temp);
ambient.set(2, pres);
ambient.send();
}
参考ページ
この記事が気に入ったらサポートをしてみませんか?