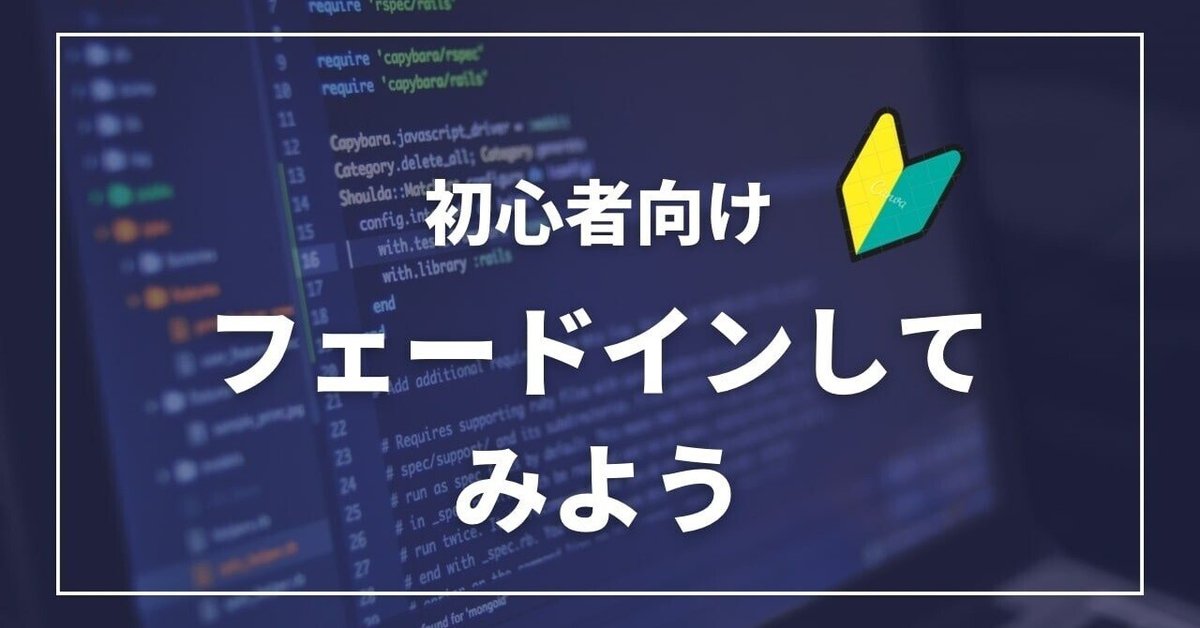
40代高卒者が製造業からIT転職する【フェードイン】
こんにちは、フローです。
今回は、カードデザインが下からふわっと出てくるアニメーションを、
HTML,CSS,jQueryを使用して書いていきましょう。
目次
ふわっとアニメーション
こんな感じです。
テキストは英語ですが、何語でも構いません。
レスポンシブにも対応しています。
しかし、iPhone等の小さな画面だとコードペンの画面が小さすぎて、
うまく表示されない場合があります。
その時は、pcで確認して見て下さい。
それでは記述を見ていきましょう。
HTML
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>ふわっとアニメーション</title>
</head>
<body>
<div class="card-row">
<div class="card">
<div class="card-content">
<h2>タイトル1</h2>
<p>テキスト1</p>
</div>
</div>
<div class="card">
<div class="card-content">
<h2>タイトル2</h2>
<p>テキスト2</p>
</div>
</div>
<div class="card">
<div class="card-content">
<h2>タイトル3</h2>
<p>テキスト3</p>
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
jQuery読み込む CDN
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
jQueryの記述が書いてあるファイルを読み込む為の記述
<script src="script.js"></script>
CSS
.card-row {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
min-height: 100vh;
overflow: hidden;
background-color: #f5f5f5;
}
.card {
width: 100%;
max-width: 300px;
margin: 0 10px 20px 10px;
position: relative;
overflow: hidden;
height: 0;
opacity: 0;
transform: translateY(50%);
background-color: #FFE0B2; /* 背景色 */
border-radius: 10px; /* 角丸 */
box-shadow: 0px 5px 15px rgba(0,0,0,0.2); /* 影 */
transition: transform 0.5s ease-in-out, opacity 0.5s ease-in-out;
}
.card.show {
height: auto;
opacity: 1;
transform: translateY(0%);
transition-delay: 0.5s;
}
.card:nth-child(1) {
transition-delay: 0.5s;
}
.card:nth-child(2) {
transition-delay: 1s;
}
.card:nth-child(3) {
transition-delay: 1.5s;
}
card .card-content {
padding: 20px;
text-align: center;
}
.card h2 {
font-size: 24px;
margin-bottom: 10px;
color: #FF9800; /* タイトルの色 */
}
.card p {
font-size: 16px;
line-height: 1.5;
color: #4C2600; /* テキストの色 */
}
body::-webkit-scrollbar {
width: 10px;
}
body::-webkit-scrollbar-thumb {
background-color: #FF9800;
border-radius: 10px;
}
@media only screen and (min-width: 768px) {
.card-row {
flex-direction: row;
}
.card {
margin: 0 10px;
}
}
/* iPhone など小さい画面でのカード表示アニメーション */
@media only screen and (max-width: 767px) {
.card {
transition: transform 1s ease-in-out, opacity 1s ease-in-out;
}
.card.show {
height: auto;
opacity: 1s;
transform: translateY(0%);
}
}
カードデザインは3つ用意して見ました。
色は適当に付けましたので、お好きな色などに変更してください。
pcでは横並びに、iPhoneなどのレスポンシブでは、縦並びになるように設計しています。
またスピードもわかりやすいように、気持ち遅めに設定しているので、
その辺も好みで変えてみて下さい。
jQuery
let ticking = false;
window.addEventListener('scroll', function() {
if (!ticking) {
window.requestAnimationFrame(function() {
let cards = document.querySelectorAll('.card');
for (let i = 0; i < cards.length; i++) {
if (cards[i].getBoundingClientRect().top + 100 < window.innerHeight) {
cards[i].classList.add('show');
}
}
ticking = false;
});
ticking = true;
}
});
このjQueryの記述を、HTMLで書いた
“script.js”というファイルを用意して、その中に保存して使って下さい。
まとめ
今回のカードデザインも、よく使われているデザインなので、
色々調整しながら、自分好みのデザインに変化させて使ってみて下さい。
次回は、これらの記述を詳しい解説していきます。
レッツチャレンジ!
この記事が気に入ったらサポートをしてみませんか?