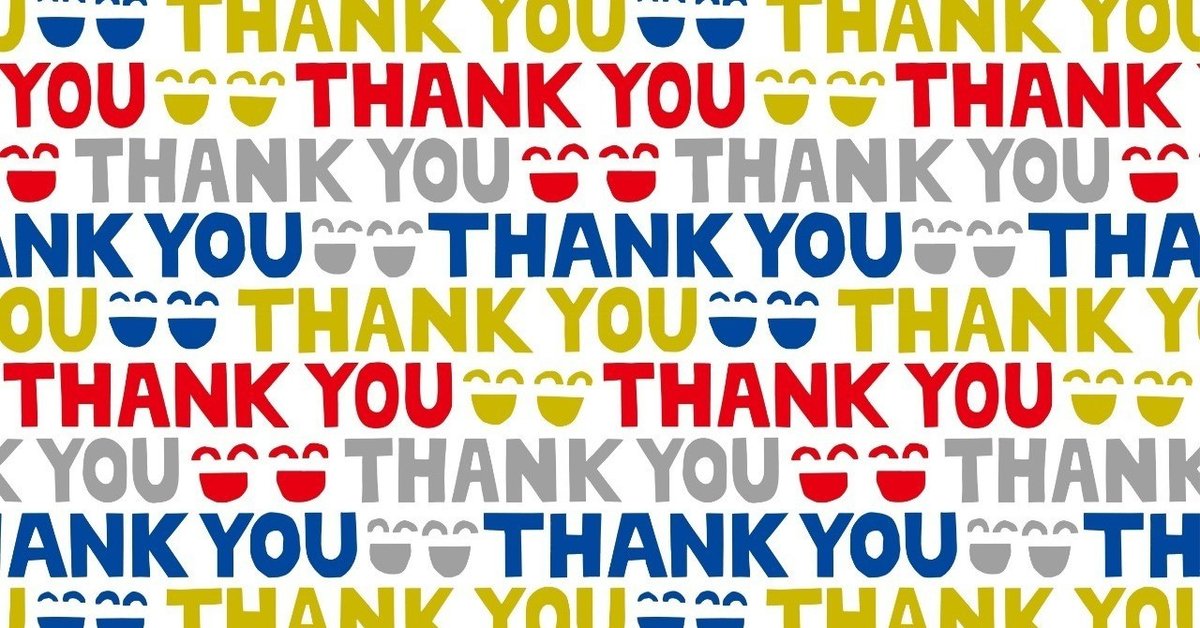
Photo by
turbo1019
[Python]Flaskで作る生誕日数計算アプリ
1.生誕日数アプリ
生年月日を入力し現在までの生誕日数を計算するアプリを作ります。フレームワークにFlaskを用います。
2.アプリ構成
アプリの構成は以下になります。
helloworld/
├── app.py
├── days_from_birth.py
├── static
│ ├── img
│ │ ├── baby.png
│ │ └── parents.png
│ ├── sanitize.css
│ └── style.css
└── templates
├── form.html
└── result.html
3.フロント部分作成
アプリケーションのフロント部分をtemplatesディレクトリに配下します。作成するのは入力を受け付けるform.htmlと結果を表示するresult.htmlの2つです。
3.1 フォーム
form.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>生誕〇〇日</title>
</head>
<link rel="stylesheet" href="../static/style.css">
<body>
<div class="content">
<header>
<h1>あなたが生まれて〇〇日</h1>
<img src="../static/img/baby.png" alt="赤ちゃんの画像" width="50%" height="50%">
</header>
<form action="/calc" method="POST">
<div class="control">
<label for="birth">生年月日(例.〇〇/〇〇/〇〇)</label>
<input type="text" id="birth" name="birth">
</div>
<div class="control">
<button type="submit">計算する</button>
</div>
</form>
</div>
</body>
</html>
3.2 結果画面
result.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>生誕{{ days }} 日</title>
</head>
<link rel="stylesheet" href="../static/style.css">
<body>
<div class="content">
<header>
<h1>あなたが生まれて{{ days }}日たちました</h1>
<img src="../static/img/parents.png" alt="親の画像" width="50%" height="50%">
</header>
<div class="control">
{% if days > 7000 %}
<p>両親、友人、普段意識することのない人たちによってここまで生かされてここまで生きて来れました</p>
{% else %}
<p>この日数を見て何を感じましたか?</p>
{% endif %}
</div>
</div>
</body>
</html>
4.スタイル
HTMLに装飾を当てるためCSSを記述します。
style.css
@import url("sanitize.css");
@import url('https://fonts.googleapis.com/css?family=M+PLUS+Rounded+1c');
* {
font-family: 'M PLUS Rounded 1c', sans-serif;
}
.content {
width: 600px;
border: 1px solid #d1d1d1;
margin: 15px auto;
padding: 20 30 30px;
background-color:#fff;
}
header {
text-align:center;
color: #666;
}
input {
display: block;
border-top: none;
border-left: none;
border-right: none;
border-bottom: 1px solid #666;
width: 30%;
font-size: 1.5em;
padding: 8px;
margin-left: 210px;
margin-top: 20px;
}
.control {
margin-bottom: 15px;
text-align: center
}
button {
color:#fff;
background-color: #1da1f2;
padding: 15px;
border-radius: 10px;
box-shadow: 0 0 5px rgba(0, 0, 0, .3);
width: 30%;
}
button:hover {
color: #1da1f2;
background-color: #fff;
padding: 15px;
border-radius: 10px;
box-shadow: 0 0 5px rgba(0, 0, 0, .3);
width: 30%;
}
5.誕生日計算処理
誕生日計算処理モジュールを作成します。
days_from_birth.py
from datetime import date
def calc(birth_date):
now_date = date.today()
bth_date_list = [int(x) for x in birth_date.split("/")]
year = bth_date_list[0]
month = bth_date_list[1]
day = bth_date_list[2]
birth_date = date(year, month, day)
diff_day = (now_date - birth_date).days
return diff_day
if __name__ == "__main__":
print(calc("1998/9/25"))
6.ロジック部分の実装
app.py
上記よりロジック部分の実装を行なっていきます。
from flask import Flask
from flask import render_template
from flask import request
from days_from_birth import calc
app = Flask(__name__)
@app.route("/calc", methods=["POST", "GET"])
def index():
if request.method == "POST":
birth_date = request.form["birth"]
days = calc(birth_date)
return render_template("result.html", days=days)
else:
return render_template("form.html")
7.実行結果
この記事が気に入ったらサポートをしてみませんか?