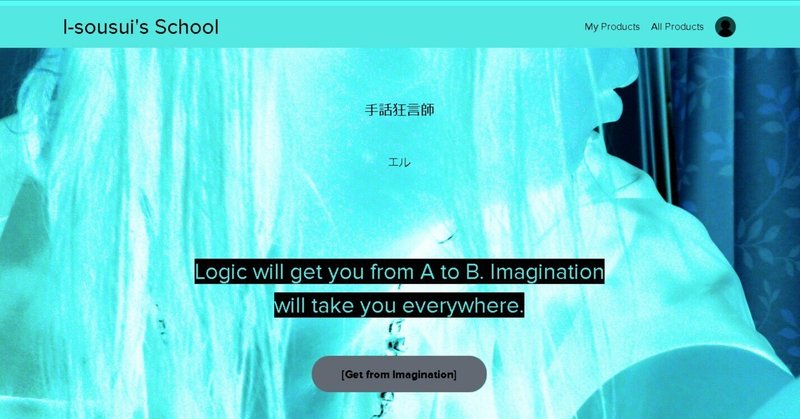
Write a weather usage programme in python.
pythonCopy code
import requests def get_weather(api_key, city): base_url = "https://api.openweathermap.org/data/2.5/weather" params = { "q": city, "appid": api_key, "units": "metric" } response = requests.get(base_url, params=params) weather_data = response.json() return weather_data def display_weather(weather_data): if weather_data["cod"] != "404": main_info = weather_data["weather"][0]["main"] description = weather_data["weather"][0]["description"] temperature = weather_data["main"]["temp"] humidity = weather_data["main"]["humidity"] wind_speed = weather_data["wind"]["speed"] city = weather_data["name"] country = weather_data["sys"]["country"] print(f"Weather in {city}, {country}:") print(f"Conditions: {main_info} ({description})") print(f"Temperature: {temperature}°C") print(f"Humidity: {humidity}%") print(f"Wind Speed: {wind_speed} m/s") else: print("City not found.") def main(): api_key = "YOUR_API_KEY" # Replace with your OpenWeatherMap API key city = input("Enter city name: ") weather_data = get_weather(api_key, city) display_weather(weather_data) if __name__ == "__main__": main()
追記
To use this program, you need to have an API key from OpenWeatherMap (https://openweathermap.org/api) to access their weather API. Replace "YOUR_API_KEY" in the main function with your actual API key.
When you run the program, it will prompt you to enter the name of a city. It will then retrieve the weather data for that city using the OpenWeatherMap API and display the current weather conditions, including the weather description, temperature, humidity, and wind speed.
Note: Remember to install the requests module if you haven't already by running pip install requests in your terminal or command prompt.
日本語訳
このプログラムを使用するには、OpenWeatherMap (https://openweathermap.org/api) の天気APIにアクセスするためのAPIキーが必要です。メイン関数の「YOUR_API_KEY」を実際のAPIキーに置き換えてください。
プログラムを実行すると、都市名を入力するよう促されます。そして、OpenWeatherMap APIを使ってその都市の天気データを取得し、天気の説明、気温、湿度、風速を含む現在の天気を表示します。
注:まだインストールされていない場合は、ターミナルまたはコマンドプロンプトで pip install requests を実行して、requests モジュールをインストールすることを忘れないでください。
この記事が気に入ったらサポートをしてみませんか?