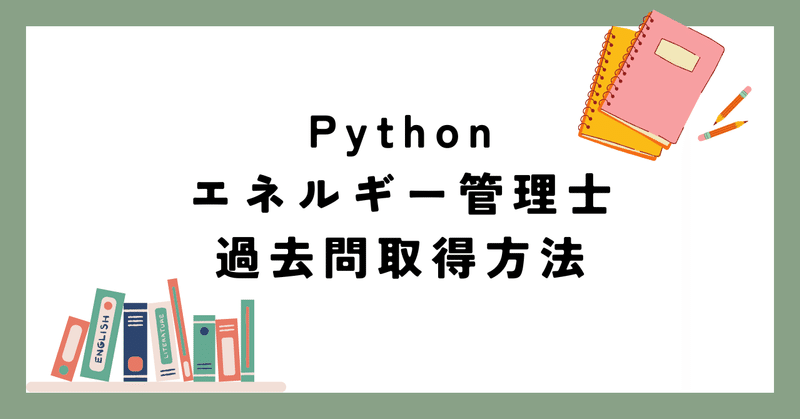
エネルギー管理士 Pythonを用いた過去問取得方法
注意事項
Pythonを用いて、省エネルギーセンターのエネルギー管理士試験過去問の内、電気分野の過去問を一括取得する方法を解説する。
過去問の取り扱いについては、個人利用のみ認められている。
試験問題は、個人的な利用を目的としたものです。これらの全部又は一部を無断で複製・転写することを固く禁じます。個人利用の範囲を超えて利用される方は、当センター試験部までご一報ください。
必要な外部ライブラリ
今回は外部ライブラリとしてBeautifulsoup4というライブラリを使用する。そのため、インストールしていない場合は、以下のコマンドでインストールする必要がる。
pip用
pip install beautifulsoup4
Anaconda用
conda install beautifulsoup4
プログラム
必要ライブラリのimport
Beautifulsoup4以外は標準ライブラリなので、インストールしなくても使用できる。
from bs4 import BeautifulSoup
import urllib.request as req
import urllib
import os
import time
from urllib.parse import urljoin
aタグを取得
urlにエネルギー管理士の過去問掲載ページのurlを指定する。
url = "https://www.eccj.or.jp/mgr1/test_past/index.html" # 過去問
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
result = soup.select("a[href]")
# 問題のurl
testurl = "https://www.eccj.or.jp/mgr1/test_past/pdf/"
リンクのみ取得
link_list =[]
for link in result:
href = link.get("href")
link_list.append(href)
PDFのみ取得
# pdfのみ取得
pdf_list = [temp for temp in link_list if temp.endswith(('pdf', 'PDF'))]
test_pdf_list = [os.path.basename(temp) for temp in pdf_list]
電気分野の過去問を平成と令和に分ける
pdfファイル名の末尾は以下のようになっている。
1:エネルギー総合管理及び法規
2:熱と流体の流れの基礎
3:燃料と燃焼
4:熱利用設備及びその管理
5:電気の基礎
6:電気設備及び機器
7:電力応用
今回は、電気分野と共通のもののみ取得したいので、pdfファイル名の末尾が1,5,6,7となっているか判断している。また、平成の場合は、h、令和の場合は、rがファイル名に含まれている。
htest_list = []
rtest_list = []
for testpdf in test_pdf_list:
if ("_1" in testpdf or "_5" in testpdf or "_6" in testpdf or "_7" in testpdf) and "h" in testpdf:
htest_list.append(testpdf)
elif ("_1" in testpdf or "_5" in testpdf or "_6" in testpdf or "_7" in testpdf) and "r" in testpdf:
rtest_list.append(testpdf)
平成の過去問を取得
substr = ["エネルギー総合管理及び法規", "エネルギー総合管理及び法規_解答", "電気の基礎", "電気の基礎_解答", "電気設備及び機器", "電気設備及び機器_解答", "電力応用", "電力応用_解答"]
# 平成22年度から平成30年度まで
for y in range(22,31):
k = 0
for tpdf in htest_list:
if str(y) in tpdf:
target_dir = "保存先ファイル名のパス"
filename = f'平成{y}年度{substr[k]}.pdf'
savepath = os.path.join(target_dir, filename)
if y <= 27:
pdflink = urljoin(testurl, tpdf)
else:
pdflink = urljoin(testurl, f'h{y}/{tpdf}')
urllib.request.urlretrieve(pdflink, savepath)
time.sleep(2)
k = k + 1
令和の過去問を取得
# 令和元年以降
for y in range(1,5):
k = 0
for tpdf in rtest_list:
if f'0{str(y)}' in tpdf:
target_dir = "保存先ファイル名のパス"
filename = f'令和{y}年度{substr[k]}.pdf'
savepath = os.path.join(target_dir, filename)
pdflink = urljoin(testurl, f'r0{y}/{tpdf}')
urllib.request.urlretrieve(pdflink, savepath)
time.sleep(2)
k = k + 1
コード全体
from bs4 import BeautifulSoup
import urllib.request as req
import urllib
import os
import time
from urllib.parse import urljoin
# <a>タグを取得
url = "https://www.eccj.or.jp/mgr1/test_past/index.html" # 過去問
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
result = soup.select("a[href]")
# 問題のurl
testurl = "https://www.eccj.or.jp/mgr1/test_past/pdf/"
# リンクのみ取得
link_list =[]
for link in result:
href = link.get("href")
link_list.append(href)
# pdfのみ取得
pdf_list = [temp for temp in link_list if temp.endswith(('pdf', 'PDF'))]
test_pdf_list = [os.path.basename(temp) for temp in pdf_list]
htest_list = []
rtest_list = []
for testpdf in test_pdf_list:
if ("_1" in testpdf or "_5" in testpdf or "_6" in testpdf or "_7" in testpdf) and "h" in testpdf:
htest_list.append(testpdf)
elif ("_1" in testpdf or "_5" in testpdf or "_6" in testpdf or "_7" in testpdf) and "r" in testpdf:
rtest_list.append(testpdf)
substr = ["エネルギー総合管理及び法規", "エネルギー総合管理及び法規_解答", "電気の基礎", "電気の基礎_解答", "電気設備及び機器", "電気設備及び機器_解答", "電力応用", "電力応用_解答"]
# 平成22年度から平成30年度まで
for y in range(22,31):
k = 0
for tpdf in htest_list:
if str(y) in tpdf:
target_dir = "保存先ファイル名のパス"
filename = f'平成{y}年度{substr[k]}.pdf'
savepath = os.path.join(target_dir, filename)
if y <= 27:
pdflink = urljoin(testurl, tpdf)
else:
pdflink = urljoin(testurl, f'h{y}/{tpdf}')
urllib.request.urlretrieve(pdflink, savepath)
time.sleep(2)
k = k + 1
# 令和元年以降
for y in range(1,5):
k = 0
for tpdf in rtest_list:
if f'0{str(y)}' in tpdf:
target_dir = "保存先ファイル名のパス"
filename = f'令和{y}年度{substr[k]}.pdf'
savepath = os.path.join(target_dir, filename)
pdflink = urljoin(testurl, f'r0{y}/{tpdf}')
urllib.request.urlretrieve(pdflink, savepath)
time.sleep(2)
k = k + 1
関連記事
電験一種の過去問をPythonで取得する方法
https://note.com/elemag/n/n9f86c492dcc9?sub_rt=share_pw
電験二種の過去問をPythonで取得する方法
https://note.com/elemag/n/ne296a22a871f?sub_rt=share_pw
電験三種の過去問をPythonで取得する方法
https://note.com/elemag/n/nfdba4ff3112d?sub_rt=share_pw
サイト
https://sites.google.com/view/elemagscience/%E3%83%9B%E3%83%BC%E3%83%A0
この記事が気に入ったらサポートをしてみませんか?