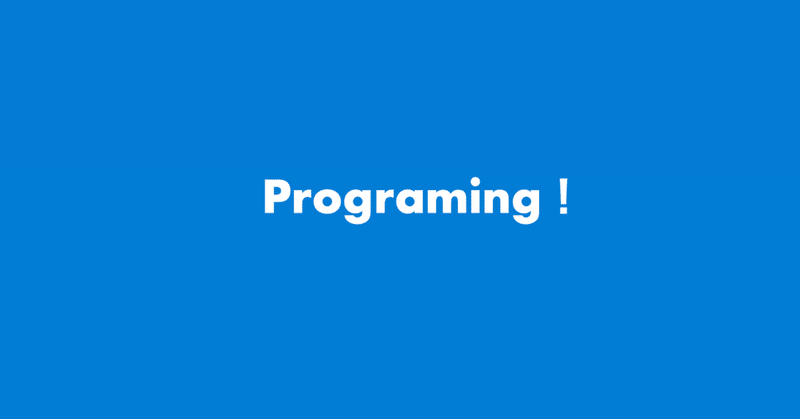
プログラミングを始める。 - 判定しましょ。"Swich"2
さらに、switchを使っていろんな条件によって振り分けます。
let anotherPoint = (2, 0)
でタプルの変数があります。この変数に合致した上で、片方の値が欲しい場合に変数で受ける仕組みがあります。
case (let x, 0):
ということであれば左の値を"let x"として定義して変数"x"に入れて以降のコードで利用が可能となります。
let anotherPoint = (2, 0)
switch anotherPoint {
case (let x, 0):
print("on the x-axis with an x value of \(x)")
case (0, let y):
print("on the y-axis with a y value of \(y)")
case let (x, y):
print("somewhere else at (\(x), \(y))")
}
この場合は
case (let x, 0):
print("on the x-axis with an x value of \(x)")
に該当します。
出力は"on the x-axis with an x value of 2"となります。
次に色々無条件について変数を使ってさらに絞った選択ができるようにするために
Where
を使って条件を付けていきます。
let yetAnotherPoint = (1, -1)
switch yetAnotherPoint {
case let (x, y) where x == y:
print("(\(x), \(y)) is on the line x == y")
case let (x, y) where x == -y:
print("(\(x), \(y)) is on the line x == -y")
case let (x, y):
print("(\(x), \(y)) is just some arbitrary point")
}
この場合だとタプルで受けた値を比較してその条件にあったものを選択するものとなっています。
case let (x, y) where x == -y:
print("(\(x), \(y)) is on the line x == -y")
"(1, -1) is on the line x == -y"と出力されます。
次にcaseで複数条件の中のどれかに合致すればその処理が実行される仕組みをみていきます。
let someCharacter: Character = "e"
switch someCharacter {
case "a", "e", "i", "o", "u":
print("\(someCharacter) is a vowel")
case "b", "c", "d", "f", "g", "h", "j", "k", "l", "m",
"n", "p", "q", "r", "s", "t", "v", "w", "x", "y", "z":
print("\(someCharacter) is a consonant")
default:
print("\(someCharacter) is not a vowel or a consonant")
}
提示する変数の値は"e"となっています。それに合致する"case"の処理が行われることになります。この場合
case "a", "e", "i", "o", "u":
の中に"e"が入っているので、このcaseの処理、
print("\(someCharacter) is a vowel")
が実行され、 "e is a vowel"が出力されます。
あとは
let numberSymbol: Character = "三"
var possibleIntegerValue: Int?
switch numberSymbol {
case "1", "١", "一", "๑":
possibleIntegerValue = 1
case "2", "٢", "二", "๒":
possibleIntegerValue = 2
case "3", "٣", "三", "๓":
possibleIntegerValue = 3
case "4", "٤", "四", "๔":
possibleIntegerValue = 4
default:
break
}
if let integerValue = possibleIntegerValue {
print("The integer value of \(numberSymbol) is \(integerValue).")
} else {
print("An integer value could not be found for \(numberSymbol).")
}
では
default:
break
ということで基本的には"break"これは実行を終了するということで、処理を終わらせます。該当ない場合にはコードを実行しません。
let integerToDescribe = 5
var description = "The number \(integerToDescribe) is"
switch integerToDescribe {
case 2, 3, 5, 7, 11, 13, 17, 19:
description += " a prime number, and also"
fallthrough
default:
description += " an integer."
}
print(description)
ここでは
fallthrough
ですが、このキーワードが入ってる場合はそのあとの処理、この場合だと、
description += " an integer."
を実行することを意味しています。ですので出力は、
The number 5 is a prime number, and also an integer.
となります。
この記事が気に入ったらサポートをしてみませんか?