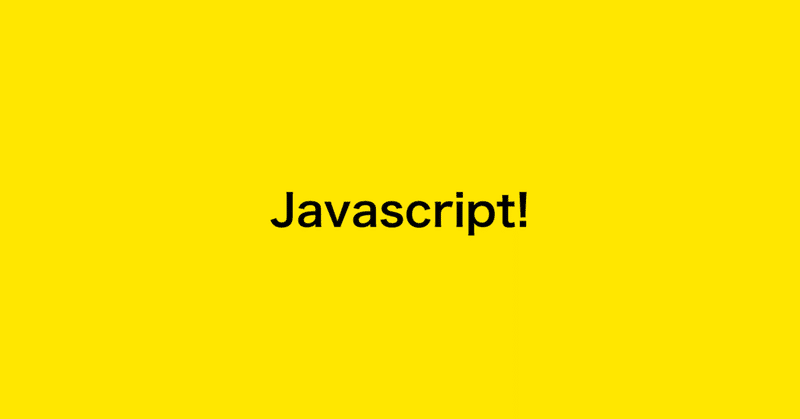
やっぱり。JavaScript!-カレンダーを作る!
まずHTMLの構造から。HTML5で<head></head>には、meta要素、スタイル、タイトル、そのあとに<body></body>として具体的に内容、JavaScriptを書きます。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
body{
font-family: sans-serif;
}
</style>
<title>カレンダー</title>
</head>
<body>
</body>
</html>
JavaScriptを適応させて表示部分を作ります。
<div id = "cal"> </div>
"id"で名前をつけておきます。今回は"cal"としています。表示部分はこれだけです。
あとはJavaScript本体を書いていきます。<script></script>を作ってその中に書きます。日付の取り方は以下のサイトが参考になります。
const date = new Date();
これでそのときの日付を取得します。ブラウザのコンソールで試してみると、
Sun Jul 04 2021 05:42:46 GMT+0900
と取得が確認できます。この"date"を使って欲しいデータを取り出して変数に入れていきます。
const year = date.getFullYear(); ・・・・ 年を取得
// 月表示のために"+1"をする
const month = date.getMonth() + 1; ・・・・ 月を取得
// monthは表示のために"+1"していたため曜日を取得するためには"-1"してやる
const firstDate = new Date(year,month-1,1); ・・・・ 月の最初の日を上記で取得した年、月から取得します。
const firstDay = firstDate.getDay(); ・・・・ 取得した最初の日から曜日を取得します。
const lastDate = new Date(year,month,0); ・・・・ 月の最後の日を上記で取得した年、月から取得します。
const lastDay = lastDate.getDate(); ・・・・ 取得した最後の日からその数字(30,31,29,28)を取得します。
次に日にちをカウントする数字を定義します。
let dayCount = "1"
そして、カレンダーを作ってそれを表示させるためのHTMLを書くための変数を定義します。
let createHtml = ""
これで変数の準備ができました。それではまず、カレンダーの一番上の曜日を作っていきます。変数createHtmlにタグと数字をfor文で繰り返して、リストweeksからweeks[i]として選び出して表示させています。"+="で追加していきます。
const weeks = ["日","月","火","水","木","金","土"];
createHtml = "<h3>" + year + " / " + month + "</h3>"
createHtml += "<table>" + "<tr>"
const weeks = ["日","月","火","水","木","金","土"];
for (let i = 0;i < weeks.length;i++){
createHtml += "<td>" + weeks[i] + "</td>"
}
createHtml += "</tr>"
createHtml += "</table>"
基本はテーブルタグを使って表を作ってやります。横一列になります。
"<table></table>" の中に "<tr></tr>"を作りさらにその中に"<td></td>"を入れてやります。
そして次にメインのカレンダーの表示部分になります。"<table></table>"の中に曜日とは別に"<tr></tr>"を作りその中にfor文で繰り返して作ります。
for (let w = 0; w < 6; w++) {
createHtml += "<tr>"
for (let d = 0; d < 7; d++) {
if (w == 0 && d < firstDay) {
createHtml += "<td></td>"
} else if (dayCount > lastDay) {
createHtml += "<td></td>"
} else {
if (dayCount < 10){
createHtml += "<td>" + " " + dayCount + "</td>"
}else{
createHtml += "<td>" + dayCount + "</td>"
}
dayCount++
}
}
createHtml += "</tr>"
}
if (w == 0 && d < firstDay) {
createHtml += "<td></td>"
} else if (dayCount > lastDay) {
createHtml += "<td></td>"
}
この部分でカレンダー空白部分を作っています。空白部分は"<td></td>"を入れて埋めていきます。カレンダー上部は"firstDay"で取得した曜日の数字を使っています。
if (w == 0 && d < firstDay) {
createHtml += "<td></td>"
}
1行目(w==0)で、最初の日の曜日より"d"が小さい時(d < firstDay)に空白を入れます。
最後の日も同じように、
else if (dayCount > lastDay) {
createHtml += "<td></td>
}
dayCountはカレンダーの日付にあたる部分で上から順番の続いてきている数字。これが"lastDay"を超えれば空白を入れます。
上記以外の場合は
createHtml += '<td>' + dayCount + "</td>"
で埋めていけば良いのですが、ここでは、1,2 ・・は10,11と比べて半角なのでこれをスペースで埋めて揃えています。
if (dayCount < 10){
createHtml += "<td>" + " " + dayCount + "</td>"
}else{
createHtml += "<td>" + dayCount + "</td>"
}
dayCount++
dayCount++
で数字をカウントして1 から月最後まで数字を入れます。
この命令が終わればあとほ表示させるのみなので
document.querySelector("#cal").innerHTML = createHtml
今まで変数createHtmlにタグ、データを追加してきたものを表示させる命令です。これで表示されます。
全体です。
<script>
const date = new Date();
const year = date.getFullYear();
const month = date.getMonth() + 1;
const firstDate = new Date(year,month-1,1);
const firstDay = firstDate.getDay();
const lastDate = new Date(year,month,0);
const lastDay = lastDate.getDate();
let dayCount = "1"
let createHtml = ""
createHtml = "<h3>" + year + " / " + month + "</h3>"
createHtml += "<table>" + "<tr>"
const weeks = ["日","月","火","水","木","金","土"];
for (let i = 0;i < weeks.length;i++){
createHtml += "<td>" + weeks[i] + "</td>"
}
for (let w = 0; w < 6; w++) {
createHtml += "<tr>"
for (let d = 0; d < 7; d++) {
if (w == 0 && d < firstDay) {
createHtml += "<td></td>"
} else if (dayCount > lastDay) {
createHtml += "<td></td>"
} else {
if (dayCount < 10){
createHtml += "<td>" + " " + dayCount + "</td>"
}else{
createHtml += "<td>" + dayCount + "</td>"
}
dayCount++
}
}
createHtml += "</tr>"
}
createHtml += "</tr>"
createHtml += "</table>"
document.querySelector("#cal").innerHTML = createHtml
</script>
この記事が気に入ったらサポートをしてみませんか?