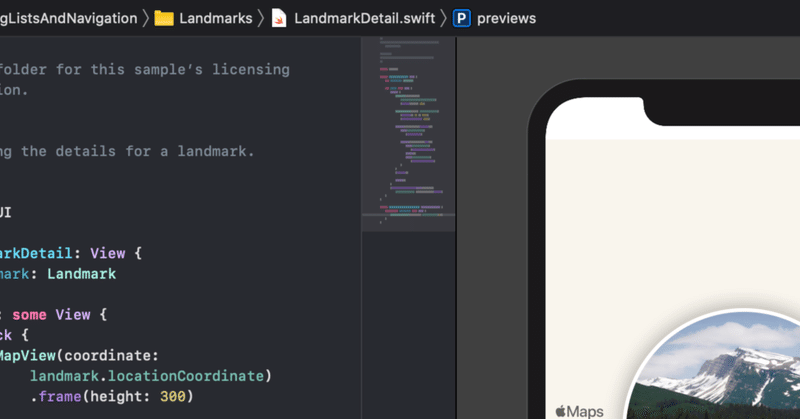
SwiftUIちょっとチップス - forEach
ForEachは繰り返し処理の中で、Viewを生成する仕組みです。
ForEachは複数のViewを生成しますので、FormやList、VStackなどのコンテナ内に記述するのが前提となります。また、Pickerの選択肢設定などにも使えます。
"Form"または"List"で使うことができます。その違いはというと、"Form" は、データをグループ化してリスト表示することができ、"Listは"単純にデータを順番に並べることができます。
インデックスを利用
ForEach、Formを使って簡単に実装。基本的な使い方としては、配列のインデックスを指定して取り出すやり方。
struct ContentView: View {
var fruits = ["apple","banana","peach","grape","strawberry"]
var body: some View {
NavigationView {
Form {
ForEach(0..<fruits.count){ index in
Text(fruits[index])
}
}
}
}
}
ForEach(0..<fruits.count)
これで配列のインデックス順番に取り出します。取り出したインデックスを
Text(fruits[index])
で画面表示させます。
"Form"を"List"に変更しても同じになります。
配列から直接
次に配列から直接値を取って表示させる方法として、"id:\.self"を使った使い方。
struct ContentView: View {
var fruits = ["apple","banana","peach","grape","strawberry"]
var body: some View {
NavigationView {
Form{
ForEach(fruits,id:\.self){ name in
Text(name)
}
}
}
}
}
ForEach(fruits,id:\.self)
とすると、配列fruitsの各要素を取り出して、その要素を"name"というキーワード(自分で自由に決めることができます)で取り出してText()で表示させています。
辞書型の配列
辞書型の配列を取り出し表示させます。構造体を作ってプロトコル
Identifiableに適合させるやり方
struct FurutsId:Identifiable{
var id = UUID()
var name:String
}
辞書は構造体を使って定義します。
var fruits = [ FurutsId(name:"apple"),FurutsId(name:"banana"),FurutsId(name:"peach"),FurutsId(name:"grape"),FurutsId(name:"strawberry")]
あとは取り出したい変数を指定して ForEachでループします。
ForEach(fruits){ fl in
Text(fl.name)
}
全体です。
struct FurutsId:Identifiable{
var id = UUID()
var name:String
}
struct ContentView: View {
var fruits = [ FurutsId(name:"apple"),FurutsId(name:"banana"),FurutsId(name:"peach"),FurutsId(name:"grape"),FurutsId(name:"strawberry")]
var body: some View {
NavigationView {
Form{
ForEach(fruits){ fl in
Text(fl.name)
}
}
}
}
}
Identifiableに準拠した構造体を作ってそれに適応した要素を取り出します。
struct FurutsId:Identifiable{
var id = UUID()
var name:String
}
Identifiableに準拠させないやり方
ForEach(fruits,id:\.id)
という感じので"id"を取り出し同じことができます。
この場合は"\.idと"して" var id = UUID()"と定義したものを取り出しに使います。
この記事が気に入ったらサポートをしてみませんか?