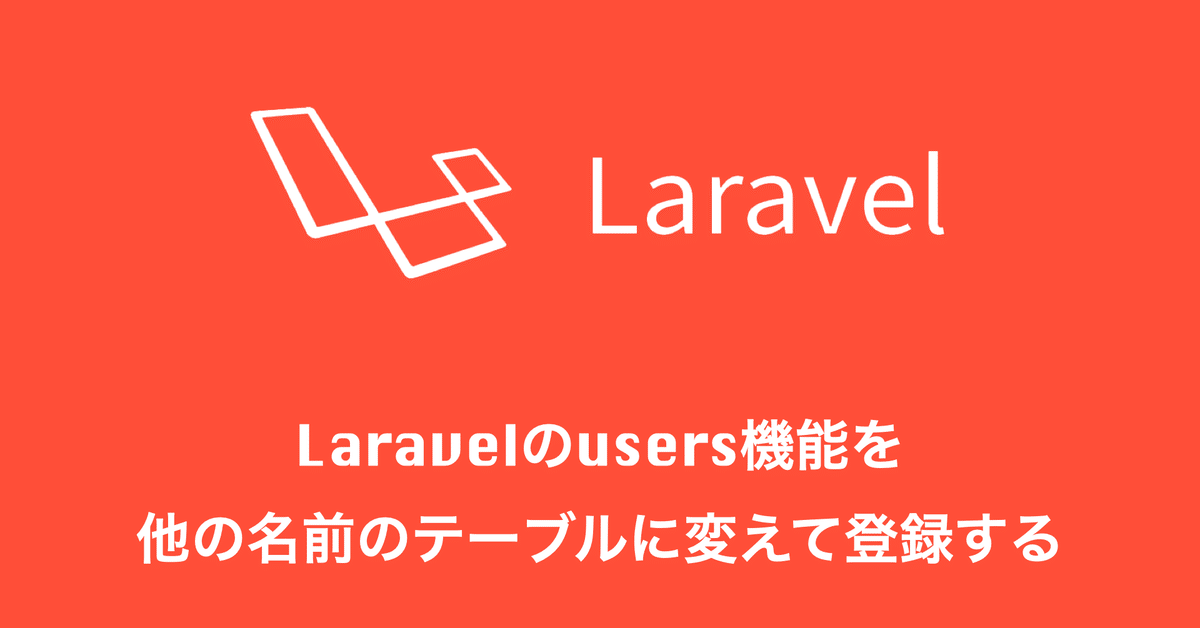
【Laravel 7.x】Laravelのusers機能を他の名前のテーブルに変えて登録する
はじめに
Laravelではデフォルトでマイグレーションファイルにusersテーブルが作成されるように出来ていますが、今回、usersテーブルではなくstaffにしたいと思ったので、備忘録として記しておこうと思います。
・新規ユーザーをusersテーブルではなく、staffテーブルに格納する
・Authの機能もstaffにする
やっていきます
マイグレーションファイルの作成(今回はstaffとします)
php artisan make:model Staff -m -c -r
// オプション説明
-m マイグレーションファイル
-c コントローラー
-r CRUD ルートをコントローラへ割り付け
マイグレーションファイル・モデル・コントローラを一気に作る時はこれが便利です。
出来上がったマイグレーションファイルを編集します。
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateStaffTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('staff', function (Blueprint $table) {
$table->id();
// ここから
$table->string('name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->rememberToken();
// ここまで追加
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('staffs');
}
}
既にあるusersテーブルを作成するマイグレーションファイルからコピーしてきても良いと思います。
モデルの編集(app/Models/staff.php)
<?php
namespace App\Models;
use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Notifications\Notifiable;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable; // 追加
class Staff extends Authenticatable // 変更
{
use HasFactory, Notifiable;
/**
* The attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'name',
'email',
'password',
];
/**
* The attributes that should be hidden for arrays.
*
* @var array
*/
protected $hidden = [
'password',
'remember_token',
];
/**
* The attributes that should be cast to native types.
*
* @var array
*/
protected $casts = [
'email_verified_at' => 'datetime',
];
}
extends Authenticatable の Authenticatable を使うために
use Illuminate\Foundation\Auth\User as Authenticatable; // 追加
が必要です。
認証機能の変更(config/auth.php)
<?php
return [
/*
|--------------------------------------------------------------------------
| Authentication Defaults
|--------------------------------------------------------------------------
|
| This option controls the default authentication "guard" and password
| reset options for your application. You may change these defaults
| as required, but they're a perfect start for most applications.
|
*/
'defaults' => [
'guard' => 'web',
'passwords' => 'staff', // 変更
],
/*
|--------------------------------------------------------------------------
| Authentication Guards
|--------------------------------------------------------------------------
|
| Next, you may define every authentication guard for your application.
| Of course, a great default configuration has been defined for you
| here which uses session storage and the Eloquent user provider.
|
| All authentication drivers have a user provider. This defines how the
| users are actually retrieved out of your database or other storage
| mechanisms used by this application to persist your user's data.
|
| Supported: "session", "token"
|
*/
'guards' => [
'web' => [
'driver' => 'session',
'provider' => 'staff', // 変更
],
'api' => [
'driver' => 'token',
'provider' => 'staff', // 変更
'hash' => false,
],
],
/*
|--------------------------------------------------------------------------
| User Providers
|--------------------------------------------------------------------------
|
| All authentication drivers have a user provider. This defines how the
| users are actually retrieved out of your database or other storage
| mechanisms used by this application to persist your user's data.
|
| If you have multiple user tables or models you may configure multiple
| sources which represent each model / table. These sources may then
| be assigned to any extra authentication guards you have defined.
|
| Supported: "database", "eloquent"
|
*/
'providers' => [
'users' => [
'driver' => 'eloquent',
'model' => App\Models\User::class,
],
// ここから
'staff' => [
'driver' => 'eloquent',
'model' => App\Models\Staff::class,
],
// ここまで追加
// 'users' => [
// 'driver' => 'database',
// 'table' => 'users',
// ],
],
/*
|--------------------------------------------------------------------------
| Resetting Passwords
|--------------------------------------------------------------------------
|
| You may specify multiple password reset configurations if you have more
| than one user table or model in the application and you want to have
| separate password reset settings based on the specific user types.
|
| The expire time is the number of minutes that the reset token should be
| considered valid. This security feature keeps tokens short-lived so
| they have less time to be guessed. You may change this as needed.
|
*/
'passwords' => [
'users' => [
'provider' => 'users',
'table' => 'password_resets',
'expire' => 60,
'throttle' => 60,
],
// ここから
'staff' => [
'provider' => 'staff',
'table' => 'password_resets',
'expire' => 60,
'throttle' => 60,
],
// ここまで追加
],
/*
|--------------------------------------------------------------------------
| Password Confirmation Timeout
|--------------------------------------------------------------------------
|
| Here you may define the amount of seconds before a password confirmation
| times out and the user is prompted to re-enter their password via the
| confirmation screen. By default, the timeout lasts for three hours.
|
*/
'password_timeout' => 10800,
];
ユーザーの新規登録の変更(app/Http/Controllers/Auth/RegisterController.php)
<?php
namespace App\Http\Controllers\Auth;
use App\Http\Controllers\Controller;
use App\Providers\RouteServiceProvider;
use App\Models\User;
use App\Models\Staff; // 追加
use Illuminate\Foundation\Auth\RegistersUsers;
use Illuminate\Support\Facades\Hash;
use Illuminate\Support\Facades\Validator;
class RegisterController extends Controller
{
/*
|--------------------------------------------------------------------------
| Register Controller
|--------------------------------------------------------------------------
|
| This controller handles the registration of new users as well as their
| validation and creation. By default this controller uses a trait to
| provide this functionality without requiring any additional code.
|
*/
use RegistersUsers;
/**
* Where to redirect users after registration.
*
* @var string
*/
// protected $redirectTo = RouteServiceProvider::HOME;
protected $redirectTo = ('/staff'); // 新規登録後の遷移先はここで変更
/**
* Create a new controller instance.
*
* @return void
*/
public function __construct()
{
$this->middleware('guest');
}
/**
* Get a validator for an incoming registration request.
*
* @param array $data
* @return \Illuminate\Contracts\Validation\Validator
*/
protected function validator(array $data)
{
return Validator::make($data, [
'name' => ['required', 'string', 'max:255'],
// この下の行を変更
'email' => ['required', 'string', 'email', 'max:255', 'unique:staff'],
'password' => ['required', 'string', 'min:8', 'confirmed'],
]);
}
/**
* Create a new user instance after a valid registration.
*
* @param array $data
* @return \App\Models\User
*/
protected function create(array $data)
{
return Staff::create([ // ここを変更
'name' => $data['name'],
'email' => $data['email'],
'password' => Hash::make($data['password']),
]);
}
}
これですべての設定が完了。
確認
php artisan ser
で立ち上げて、実際に新規作成を行って確認します
select * from staff;
usersに作成されていないかも確認
select * from users;
何も入っていないので、完了。
参考記事
ありがとうございました。
この記事が気に入ったらサポートをしてみませんか?