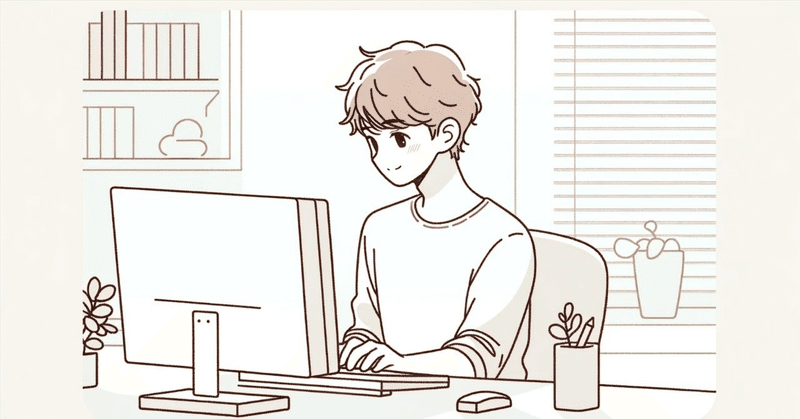
非エンジニアでも水道局のホームページを自作できるコードを公開します
※ 水道局(架空)です!
プラン
HTML構造:
基本的なHTML5の構造を作成します。
ヘッダーにはナビゲーションメニューを含めます。
メインセクションにはウェルカムメッセージと簡単な説明を追加します。
料金ページのリンクを追加します。
フッターには連絡先情報とソーシャルメディアのリンクを含めます。
CSSスタイリング:
ヘッダー、メインコンテンツ、フッターをスタイルします。
レイアウトが異なる画面サイズに対応するようにします。
JavaScript:
必要に応じてインタラクティブな要素を追加します(例:レスポンシブナビゲーションメニュー)。
HTML (ホームページ)
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>水道局ホームページ</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<nav>
<ul>
<li><a href="#home">ホーム</a></li>
<li><a href="#services">サービス</a></li>
<li><a href="rates.html">料金</a></li>
<li><a href="#contact">お問い合わせ</a></li>
</ul>
</nav>
<h1>ようこそ、水道局へ</h1>
</header>
<main>
<section id="home">
<h2>清潔で安全な水をお届けします</h2>
<p>私たちは地域社会に高品質の水を供給しています。</p>
</section>
<section id="services">
<h2>サービス内容</h2>
<ul>
<li>定期的な水質検査</li>
<li>水道の設置と修理</li>
<li>水道料金の管理</li>
</ul>
</section>
</main>
<footer>
<p>お問い合わせ: <a href="mailto:info@waterbureau.jp">info@waterbureau.jp</a></p>
<p>フォローしてください:
<a href="https://twitter.com/waterbureau">Twitter</a> |
<a href="https://facebook.com/waterbureau">Facebook</a>
</p>
</footer>
</body>
</html>
HTML (料金ページ)
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>料金 - 水道局</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<nav>
<ul>
<li><a href="index.html">ホーム</a></li>
<li><a href="index.html#services">サービス</a></li>
<li><a href="rates.html">料金</a></li>
<li><a href="index.html#contact">お問い合わせ</a></li>
</ul>
</nav>
<h1>料金</h1>
</header>
<main>
<section id="rates">
<h2>料金体系</h2>
<table>
<thead>
<tr>
<th>プラン</th>
<th>月額料金</th>
<th>詳細</th>
</tr>
</thead>
<tbody>
<tr>
<td>基本プラン</td>
<td>3000円</td>
<td>20立方メートルまで</td>
</tr>
<tr>
<td>標準プラン</td>
<td>5000円</td>
<td>50立方メートルまで</td>
</tr>
<tr>
<td>プレミアムプラン</td>
<td>8000円</td>
<td>100立方メートルまで</td>
</tr>
</tbody>
</table>
</section>
</main>
<footer>
<p>お問い合わせ: <a href="mailto:info@waterbureau.jp">info@waterbureau.jp</a></p>
<p>フォローしてください:
<a href="https://twitter.com/waterbureau">Twitter</a> |
<a href="https://facebook.com/waterbureau">Facebook</a>
</p>
</footer>
</body>
</html>
CSS (共通)
/* styles.css */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
box-sizing: border-box;
}
header {
background-color: #4CAF50;
color: white;
padding: 1em 0;
text-align: center;
}
nav ul {
list-style-type: none;
margin: 0;
padding: 0;
overflow: hidden;
background-color: #333;
}
nav ul li {
float: left;
}
nav ul li a {
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
nav ul li a:hover {
background-color: #111;
}
main {
padding: 1em;
}
section {
margin: 2em 0;
}
footer {
background-color: #333;
color: white;
text-align: center;
padding: 1em 0;
position: fixed;
bottom: 0;
width: 100%;
}
footer a {
color: #4CAF50;
text-decoration: none;
}
footer a:hover {
text-decoration: underline;
}
table {
width: 100%;
border-collapse: collapse;
margin: 1em 0;
}
table, th, td {
border: 1px solid #ddd;
}
th, td {
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
このコードを使って、水道局のホームページと料金ページを作成できます。
次のステップ
a. 料金ページに計算機能を追加するためのJavaScriptを追加します。
b. サイトのデザインを強化するために追加のCSSスタイルを追加します。
次のステップとして、料金ページに計算機能を追加するためのJavaScriptを実装します。ユーザーが使用量を入力すると、対応する料金プランと合計料金が表示されるようにします。
HTML (料金ページ) 更新版
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>料金 - 水道局</title>
<link rel="stylesheet" href="styles.css">
<script src="script.js" defer></script>
</head>
<body>
<header>
<nav>
<ul>
<li><a href="index.html">ホーム</a></li>
<li><a href="index.html#services">サービス</a></li>
<li><a href="rates.html">料金</a></li>
<li><a href="index.html#contact">お問い合わせ</a></li>
</ul>
</nav>
<h1>料金</h1>
</header>
<main>
<section id="rates">
<h2>料金体系</h2>
<table>
<thead>
<tr>
<th>プラン</th>
<th>月額料金</th>
<th>詳細</th>
</tr>
</thead>
<tbody>
<tr>
<td>基本プラン</td>
<td>3000円</td>
<td>20立方メートルまで</td>
</tr>
<tr>
<td>標準プラン</td>
<td>5000円</td>
<td>50立方メートルまで</td>
</tr>
<tr>
<td>プレミアムプラン</td>
<td>8000円</td>
<td>100立方メートルまで</td>
</tr>
</tbody>
</table>
</section>
<section id="calculator">
<h2>料金計算機</h2>
<label for="usage">使用量(立方メートル):</label>
<input type="number" id="usage" name="usage">
<button onclick="calculateRate()">計算する</button>
<p id="result"></p>
</section>
</main>
<footer>
<p>お問い合わせ: <a href="mailto:info@waterbureau.jp">info@waterbureau.jp</a></p>
<p>フォローしてください:
<a href="https://twitter.com/waterbureau">Twitter</a> |
<a href="https://facebook.com/waterbureau">Facebook</a>
</p>
</footer>
</body>
</html>
JavaScript (script.js)
// script.js
function calculateRate() {
const usage = document.getElementById('usage').value;
let rate;
let plan;
if (usage <= 20) {
rate = 3000;
plan = '基本プラン';
} else if (usage <= 50) {
rate = 5000;
plan = '標準プラン';
} else if (usage <= 100) {
rate = 8000;
plan = 'プレミアムプラン';
} else {
rate = 8000 + (usage - 100) * 100;
plan = 'プレミアムプラン (追加料金適用)';
}
const result = `使用量: ${usage}立方メートル\nプラン: ${plan}\n月額料金: ${rate}円`;
document.getElementById('result').innerText = result;
}
CSS (styles.css) 更新版
/* styles.css */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
box-sizing: border-box;
}
header {
background-color: #4CAF50;
color: white;
padding: 1em 0;
text-align: center;
}
nav ul {
list-style-type: none;
margin: 0;
padding: 0;
overflow: hidden;
background-color: #333;
}
nav ul li {
float: left;
}
nav ul li a {
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
nav ul li a:hover {
background-color: #111;
}
main {
padding: 1em;
}
section {
margin: 2em 0;
}
footer {
background-color: #333;
color: white;
text-align: center;
padding: 1em 0;
position: fixed;
bottom: 0;
width: 100%;
}
footer a {
color: #4CAF50;
text-decoration: none;
}
footer a:hover {
text-decoration: underline;
}
table {
width: 100%;
border-collapse: collapse;
margin: 1em 0;
}
table, th, td {
border: 1px solid #ddd;
}
th, td {
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
#calculator {
margin: 2em 0;
}
#calculator label {
margin-right: 1em;
}
#calculator input {
margin-right: 1em;
}
#result {
margin-top: 1em;
font-weight: bold;
}
これで、料金ページに使用量に基づいた料金計算機能が追加されました。ユーザーが使用量を入力し、「計算する」ボタンをクリックすると、対応する料金プランと月額料金が表示されます。
次のステップ
a. 使用量に基づいた料金計算機能にエラーハンドリングを追加します。
b. ホームページに最新のお知らせセクションを追加します。
次のステップとして、使用量に基づいた料金計算機能にエラーハンドリングを追加します。これにより、ユーザーが無効な入力をした場合に適切なエラーメッセージが表示されるようにします。
JavaScript (script.js) 更新版
// script.js
function calculateRate() {
const usageInput = document.getElementById('usage');
const usage = parseFloat(usageInput.value);
const resultElement = document.getElementById('result');
// 入力のバリデーション
if (isNaN(usage) || usage < 0) {
resultElement.innerText = '無効な入力です。正しい使用量を入力してください。';
return;
}
let rate;
let plan;
if (usage <= 20) {
rate = 3000;
plan = '基本プラン';
} else if (usage <= 50) {
rate = 5000;
plan = '標準プラン';
} else if (usage <= 100) {
rate = 8000;
plan = 'プレミアムプラン';
} else {
rate = 8000 + (usage - 100) * 100;
plan = 'プレミアムプラン (追加料金適用)';
}
const result = `使用量: ${usage}立方メートル\nプラン: ${plan}\n月額料金: ${rate}円`;
resultElement.innerText = result;
}
HTML (料金ページ) 更新版
HTML部分に変更はありませんが、最新のJavaScriptコードを反映するために、HTMLが適切にロードされていることを確認します。
HTML (料金ページ)
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>料金 - 水道局</title>
<link rel="stylesheet" href="styles.css">
<script src="script.js" defer></script>
</head>
<body>
<header>
<nav>
<ul>
<li><a href="index.html">ホーム</a></li>
<li><a href="index.html#services">サービス</a></li>
<li><a href="rates.html">料金</a></li>
<li><a href="index.html#contact">お問い合わせ</a></li>
</ul>
</nav>
<h1>料金</h1>
</header>
<main>
<section id="rates">
<h2>料金体系</h2>
<table>
<thead>
<tr>
<th>プラン</th>
<th>月額料金</th>
<th>詳細</th>
</tr>
</thead>
<tbody>
<tr>
<td>基本プラン</td>
<td>3000円</td>
<td>20立方メートルまで</td>
</tr>
<tr>
<td>標準プラン</td>
<td>5000円</td>
<td>50立方メートルまで</td>
</tr>
<tr>
<td>プレミアムプラン</td>
<td>8000円</td>
<td>100立方メートルまで</td>
</tr>
</tbody>
</table>
</section>
<section id="calculator">
<h2>料金計算機</h2>
<label for="usage">使用量(立方メートル):</label>
<input type="number" id="usage" name="usage">
<button onclick="calculateRate()">計算する</button>
<p id="result"></p>
</section>
</main>
<footer>
<p>お問い合わせ: <a href="mailto:info@waterbureau.jp">info@waterbureau.jp</a></p>
<p>フォローしてください:
<a href="https://twitter.com/waterbureau">Twitter</a> |
<a href="https://facebook.com/waterbureau">Facebook</a>
</p>
</footer>
</body>
</html>
これで、料金計算機能にエラーハンドリングが追加されました。無効な入力(例えば負の数や数値以外の入力)がされた場合に適切なエラーメッセージが表示されます。
次のステップ
a. ホームページに最新のお知らせセクションを追加します。
b. 料金ページに追加の計算機能(例えば、複数月の合計料金の計算)を追加します。
次のステップとして、料金ページに複数月の合計料金を計算する機能を追加します。ユーザーが使用量と月数を入力すると、合計料金が計算されて表示されるようにします。
HTML (料金ページ) 更新版
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>料金 - 水道局</title>
<link rel="stylesheet" href="styles.css">
<script src="script.js" defer></script>
</head>
<body>
<header>
<nav>
<ul>
<li><a href="index.html">ホーム</a></li>
<li><a href="index.html#services">サービス</a></li>
<li><a href="rates.html">料金</a></li>
<li><a href="index.html#contact">お問い合わせ</a></li>
</ul>
</nav>
<h1>料金</h1>
</header>
<main>
<section id="rates">
<h2>料金体系</h2>
<table>
<thead>
<tr>
<th>プラン</th>
<th>月額料金</th>
<th>詳細</th>
</tr>
</thead>
<tbody>
<tr>
<td>基本プラン</td>
<td>3000円</td>
<td>20立方メートルまで</td>
</tr>
<tr>
<td>標準プラン</td>
<td>5000円</td>
<td>50立方メートルまで</td>
</tr>
<tr>
<td>プレミアムプラン</td>
<td>8000円</td>
<td>100立方メートルまで</td>
</tr>
</tbody>
</table>
</section>
<section id="calculator">
<h2>料金計算機</h2>
<label for="usage">使用量(立方メートル):</label>
<input type="number" id="usage" name="usage">
<label for="months">月数:</label>
<input type="number" id="months" name="months">
<button onclick="calculateRate()">計算する</button>
<p id="result"></p>
</section>
</main>
<footer>
<p>お問い合わせ: <a href="mailto:info@waterbureau.jp">info@waterbureau.jp</a></p>
<p>フォローしてください:
<a href="https://twitter.com/waterbureau">Twitter</a> |
<a href="https://facebook.com/waterbureau">Facebook</a>
</p>
</footer>
</body>
</html>
JavaScript (script.js) 更新版
// script.js
function calculateRate() {
const usageInput = document.getElementById('usage');
const monthsInput = document.getElementById('months');
const usage = parseFloat(usageInput.value);
const months = parseInt(monthsInput.value);
const resultElement = document.getElementById('result');
// 入力のバリデーション
if (isNaN(usage) || usage < 0 || isNaN(months) || months <= 0) {
resultElement.innerText = '無効な入力です。正しい使用量と月数を入力してください。';
return;
}
let monthlyRate;
let plan;
if (usage <= 20) {
monthlyRate = 3000;
plan = '基本プラン';
} else if (usage <= 50) {
monthlyRate = 5000;
plan = '標準プラン';
} else if (usage <= 100) {
monthlyRate = 8000;
plan = 'プレミアムプラン';
} else {
monthlyRate = 8000 + (usage - 100) * 100;
plan = 'プレミアムプラン (追加料金適用)';
}
const totalRate = monthlyRate * months;
const result = `使用量: ${usage}立方メートル\nプラン: ${plan}\n月額料金: ${monthlyRate}円\n合計料金 (${months}ヶ月): ${totalRate}円`;
resultElement.innerText = result;
}
CSS (styles.css) 更新版
CSSには特に変更は必要ありませんが、念のために最新の状態を反映しておきます。
/* styles.css */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
box-sizing: border-box;
}
header {
background-color: #4CAF50;
color: white;
padding: 1em 0;
text-align: center;
}
nav ul {
list-style-type: none;
margin: 0;
padding: 0;
overflow: hidden;
background-color: #333;
}
nav ul li {
float: left;
}
nav ul li a {
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
nav ul li a:hover {
background-color: #111;
}
main {
padding: 1em;
}
section {
margin: 2em 0;
}
footer {
background-color: #333;
color: white;
text-align: center;
padding: 1em 0;
position: fixed;
bottom: 0;
width: 100%;
}
footer a {
color: #4CAF50;
text-decoration: none;
}
footer a:hover {
text-decoration: underline;
}
table {
width: 100%;
border-collapse: collapse;
margin: 1em 0;
}
table, th, td {
border: 1px solid #ddd;
}
th, td {
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
#calculator {
margin: 2em 0;
}
#calculator label {
margin-right: 1em;
}
#calculator input {
margin-right: 1em;
}
#result {
margin-top: 1em;
font-weight: bold;
}
これで、料金ページに使用量と月数に基づいて合計料金を計算する機能が追加されました。ユーザーが使用量と月数を入力し、「計算する」ボタンをクリックすると、対応する料金プラン、月額料金、および合計料金が表示されます。
次のステップ
a. ユーザーが計算結果をPDFとしてダウンロードできるようにします。
b. 入力フィールドにリアルタイムバリデーションを追加して、無効な入力を即座に通知します。
次のステップとして、ユーザーが計算結果をPDFとしてダウンロードできる機能を追加します。これを実現するために、HTMLをPDFに変換するためのライブラリを使用します。ここでは、人気のある `jsPDF` ライブラリを使用します。
HTML (料金ページ) 更新版
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>料金 - 水道局</title>
<link rel="stylesheet" href="styles.css">
<script src="https://cdnjs.cloudflare.com/ajax/libs/jspdf/2.4.0/jspdf.umd.min.js"></script>
<script src="script.js" defer></script>
</head>
<body>
<header>
<nav>
<ul>
<li><a href="index.html">ホーム</a></li>
<li><a href="index.html#services">サービス</a></li>
<li><a href="rates.html">料金</a></li>
<li><a href="index.html#contact">お問い合わせ</a></li>
</ul>
</nav>
<h1>料金</h1>
</header>
<main>
<section id="rates">
<h2>料金体系</h2>
<table>
<thead>
<tr>
<th>プラン</th>
<th>月額料金</th>
<th>詳細</th>
</tr>
</thead>
<tbody>
<tr>
<td>基本プラン</td>
<td>3000円</td>
<td>20立方メートルまで</td>
</tr>
<tr>
<td>標準プラン</td>
<td>5000円</td>
<td>50立方メートルまで</td>
</tr>
<tr>
<td>プレミアムプラン</td>
<td>8000円</td>
<td>100立方メートルまで</td>
</tr>
</tbody>
</table>
</section>
<section id="calculator">
<h2>料金計算機</h2>
<label for="usage">使用量(立方メートル):</label>
<input type="number" id="usage" name="usage">
<label for="months">月数:</label>
<input type="number" id="months" name="months">
<button onclick="calculateRate()">計算する</button>
<button onclick="downloadPDF()">PDFをダウンロード</button>
<p id="result"></p>
</section>
</main>
<footer>
<p>お問い合わせ: <a href="mailto:info@waterbureau.jp">info@waterbureau.jp</a></p>
<p>フォローしてください:
<a href="https://twitter.com/waterbureau">Twitter</a> |
<a href="https://facebook.com/waterbureau">Facebook</a>
</p>
</footer>
</body>
</html>
JavaScript (script.js) 更新版
// script.js
function calculateRate() {
const usageInput = document.getElementById('usage');
const monthsInput = document.getElementById('months');
const usage = parseFloat(usageInput.value);
const months = parseInt(monthsInput.value);
const resultElement = document.getElementById('result');
// 入力のバリデーション
if (isNaN(usage) || usage < 0 || isNaN(months) || months <= 0) {
resultElement.innerText = '無効な入力です。正しい使用量と月数を入力してください。';
return;
}
let monthlyRate;
let plan;
if (usage <= 20) {
monthlyRate = 3000;
plan = '基本プラン';
} else if (usage <= 50) {
monthlyRate = 5000;
plan = '標準プラン';
} else if (usage <= 100) {
monthlyRate = 8000;
plan = 'プレミアムプラン';
} else {
monthlyRate = 8000 + (usage - 100) * 100;
plan = 'プレミアムプラン (追加料金適用)';
}
const totalRate = monthlyRate * months;
const result = `使用量: ${usage}立方メートル\nプラン: ${plan}\n月額料金: ${monthlyRate}円\n合計料金 (${months}ヶ月): ${totalRate}円`;
resultElement.innerText = result;
}
function downloadPDF() {
const { jsPDF } = window.jspdf;
const doc = new jsPDF();
const usage = document.getElementById('usage').value;
const months = document.getElementById('months').value;
const resultText = document.getElementById('result').innerText;
if (!usage || !months || !resultText) {
alert('計算結果がありません。先に使用量と月数を入力し、計算してください。');
return;
}
doc.text(resultText, 10, 10);
doc.save('計算結果.pdf');
}
CSS (styles.css) 更新版
CSSに変更はありませんが、最新の状態を確認します。
/* styles.css */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
box-sizing: border-box;
}
header {
background-color: #4CAF50;
color: white;
padding: 1em 0;
text-align: center;
}
nav ul {
list-style-type: none;
margin: 0;
padding: 0;
overflow: hidden;
background-color: #333;
}
nav ul li {
float: left;
}
nav ul li a {
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
nav ul li a:hover {
background-color: #111;
}
main {
padding: 1em;
}
section {
margin: 2em 0;
}
footer {
background-color: #333;
color: white;
text-align: center;
padding: 1em 0;
position: fixed;
bottom: 0;
width: 100%;
}
footer a {
color: #4CAF50;
text-decoration: none;
}
footer a:hover {
text-decoration: underline;
}
table {
width: 100%;
border-collapse: collapse;
margin: 1em 0;
}
table, th, td {
border: 1px solid #ddd;
}
th, td {
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
#calculator {
margin: 2em 0;
}
#calculator label {
margin-right: 1em;
}
#calculator input {
margin-right: 1em;
}
#result {
margin-top: 1em;
font-weight: bold;
}
これで、料金ページに使用量と月数に基づいて合計料金を計算し、その結果をPDFとしてダウンロードできる機能が追加されました。
次のステップ
a. 使用量と月数の入力フィールドにリアルタイムバリデーションを追加して、無効な入力を即座に通知します。
b. ホームページに最新のお知らせセクションを追加します。
とまあ、こんな感じです。コードのみ書いたんで完成品のページはありませんが、非エンジニアの方でも上記のコードをVisual Studio Codeで読み込んでもらえればGoogle Chromeブラウザで表示確認ができるんで、興味がある人はやってみてください。
ではまた何か作ったら記事に書いてみようと思うので、お楽しみに!
この記事が気に入ったらサポートをしてみませんか?