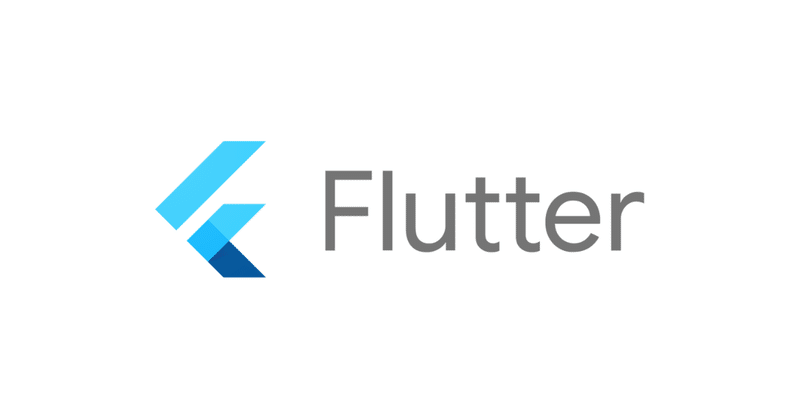
Photo by
blue_69
Flutterでチャット画面(見た目だけ)を作ってみた
初めましてblueです。
現在、ITベンチャー企業でWEBキャンペーンシステムの開発並びに要件定義を行なっています。
今回はFlutterでチャット画面(見た目だけ)を作ってみました!
※コードのみになります。
全体コード
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const ChatDetailPage(),
);
}
}
class ChatDetailPage extends StatefulWidget{
const ChatDetailPage({Key? key}) : super(key: key);
@override
_ChatDetailPageState createState() => _ChatDetailPageState();
}
class _ChatDetailPageState extends State<ChatDetailPage> {
List<ChatMessage> messages = [
ChatMessage(messageContent: "こんにちは", messageType: "receiver"),
ChatMessage(messageContent: "元気ですか?", messageType: "receiver"),
ChatMessage(messageContent: "元気です!", messageType: "sender"),
ChatMessage(messageContent: "良かったです。", messageType: "receiver"),
ChatMessage(messageContent: "ありがとうございます。最近どうですか?", messageType: "sender"),
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("チャット画面"),
),
body: Stack(
children: <Widget>[
ListView.builder(
itemCount: messages.length,
shrinkWrap: true,
padding: const EdgeInsets.only(top: 10,bottom: 10),
physics: const NeverScrollableScrollPhysics(),
itemBuilder: (context, index){
return Container(
padding: const EdgeInsets.only(left: 14,right: 14,top: 10,bottom: 10),
child: Align(
alignment: (messages[index].messageType == "receiver"?Alignment.topLeft:Alignment.topRight),
child: Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(20),
color: (messages[index].messageType == "receiver"?Colors.grey.shade200:Colors.blue[200]),
),
padding: const EdgeInsets.all(16),
child: Text(messages[index].messageContent, style: const TextStyle(fontSize: 15),),
),
),
);
},
),
Align(
alignment: Alignment.bottomLeft,
child: Container(
padding: const EdgeInsets.only(left: 10,bottom: 10,top: 10),
height: 60,
width: double.infinity,
color: Colors.white,
child: Row(
children: <Widget>[
GestureDetector(
onTap: (){
},
child: Container(
height: 30,
width: 30,
decoration: BoxDecoration(
color: Colors.lightBlue,
borderRadius: BorderRadius.circular(30),
),
child: const Icon(Icons.add, color: Colors.white, size: 20, ),
),
),
const SizedBox(width: 15,),
const Expanded(
child: TextField(
decoration: InputDecoration(
hintStyle: TextStyle(color: Colors.black54),
border: InputBorder.none
),
),
),
const SizedBox(width: 15,),
FloatingActionButton(
onPressed: (){},
child: const Icon(Icons.send,color: Colors.white,size: 18,),
backgroundColor: Colors.blue,
elevation: 0,
),
],
),
),
),
],
),
);
}
}
class ChatMessage{
String messageContent;
String messageType;
ChatMessage({required this.messageContent, required this.messageType});
}
実装画面
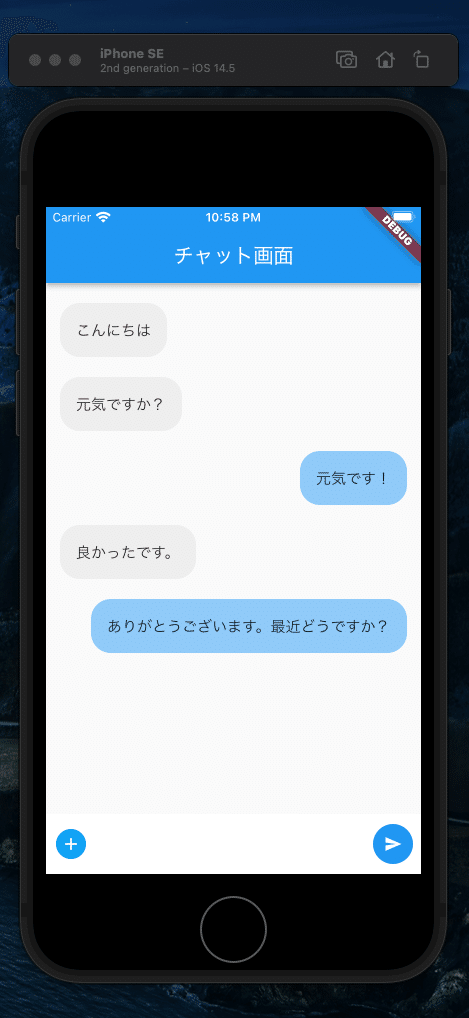
良かったらサポートしていただけると嬉しいです!