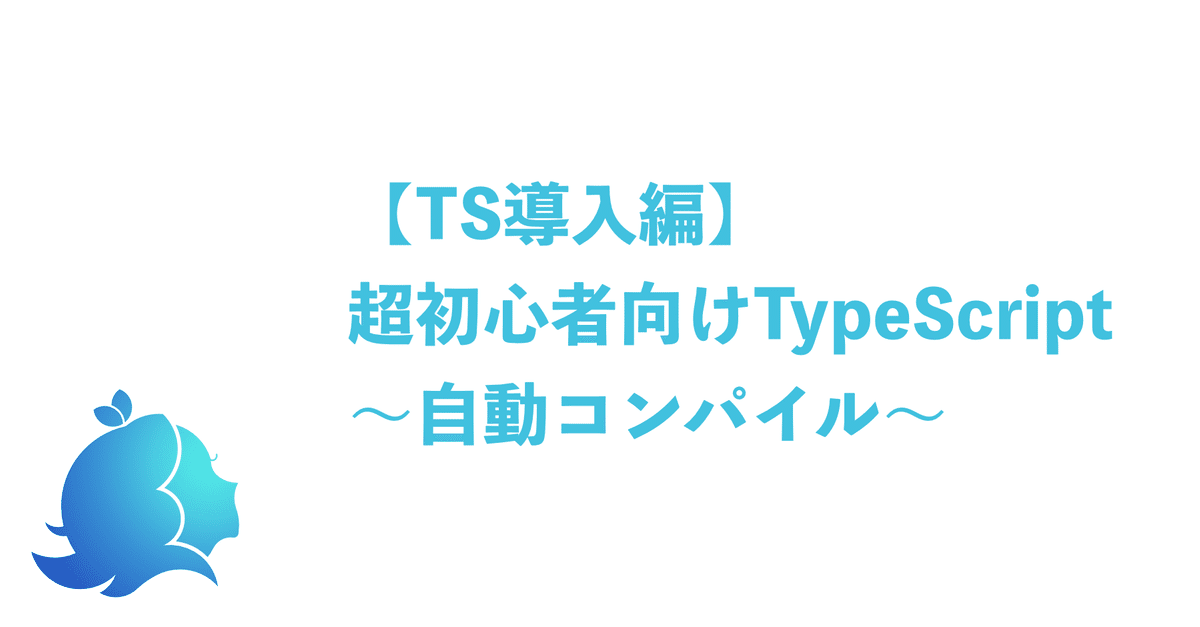
【TS導入編】超初心者向けTypeScript〜自動コンパイル〜
🎈 WPでも公開中です
https://wp.me/pc9NHC-1qd
前置き
前回の続きです❣️
型指定, 型宣言をすることで
実行前のコンパイル時に
エラーを発見できるTS💕
こんな感じで教えてくれます。
数字で定義したのに
文字列が入っているよ❗️
という内容ですね。
// terminal
app.ts:8:21 - error TS2322: Type 'number' is not assignable to type 'string'.
今回は自動コンパイルの設定です✨
それから前回できていない、
複数のtsファイルの使用について。
そして最後に少し書き方についても。
前回コンパイラと書いていましたが、
コンパイルが正しい所があります🙇♀️💦
申し訳ございません。
基本的に上書き的な作業…
動詞はコンパイルですね。
コンパイラは名詞。
コンパイルとは、プログラミング言語で書かれた文字列(ソースコード)を、コンピュータ上で実行可能な形式(オブジェクトコード)に変換することです。
コンパイルを行うソフトウェアをコンパイラといい、変換されるプログラミング言語をコンパイラ型言語と呼びます。
コンパイルって何?
自動コンパイル
tsファイルを書き換えたら
いちいちtscコマンドで
jsファイルを上書きし、
npm startするのか…❓🙄
結構面倒ですよね😂笑
ということで、
自動の設定をしましょう✨
前提として、
フレームワークなしの
HTML, JSファイルを想定しています。
Nuxt TypeScriptも今後やります。
こちらの記事の通りにやればOK⭕️
TypeScriptを簡単に動かす環境構築
⬇️TS使用可能にするコマンド
// terminal
$ npm install // note_modulesの作成
$ sudo npm install -g typescript // TSのインストール
$ tsc -v // tscコマンドが使えるか、バージョン確認
これでTSが使用可能に。
あとは参考記事の通り、
自動コンパイルに
最低限必要なものを
書き足したり
コメントアウトを外したり…
(★が該当箇所です)
// package.json
{
"name": "understanding-typescript",
"version": "1.0.0",
"private": true, ★
"description": "Understanding TypeScript Course Setup",
"main": "app.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "lite-server",
"build": "tsc", ★
"watch": "tsc -w", ★
},
"keywords": [
"typescript",
"course"
],
"author": "Maximilian Schwarzmüller",
"license": "ISC",
"devDependencies": {
"lite-server": "^2.5.4",
"typescript": "^4.3.4"
}
}
そしてtscコマンドで
tsconfig.jsonを作成します。
$ tsc --init
作成時には大体が
コメントアウトになっています。
ourDirはデフォルトの"./"だと
エラーが出て"** /*"の指示が。
人によってファイルパスが変わるので、
その辺りはエラー文を見ながら
書き換えましょう✍️
rootDirは必須ですが、
rootDirsは違うのでお間違えなく。
// tsconfig.json
{
"compilerOptions": {
/* Visit https://aka.ms/tsconfig.json to read more about this file */
/* Basic Options */
// "incremental": true, /* Enable incremental compilation */
"target": "es5", ★ /* Specify ECMAScript target version: 'ES3' (default), 'ES5', 'ES2015', 'ES2016', 'ES2017', 'ES2018', 'ES2019', 'ES2020', 'ES2021', or 'ESNEXT'. */
"module": "commonjs", ★ /* Specify module code generation: 'none', 'commonjs', 'amd', 'system', 'umd', 'es2015', 'es2020', or 'ESNext'. */
// "lib": [], /* Specify library files to be included in the compilation. */
// "allowJs": true, /* Allow javascript files to be compiled. */
// "checkJs": true, /* Report errors in .js files. */
// "jsx": "preserve", /* Specify JSX code generation: 'preserve', 'react-native', 'react', 'react-jsx' or 'react-jsxdev'. */
// "declaration": true, /* Generates corresponding '.d.ts' file. */
// "declarationMap": true, /* Generates a sourcemap for each corresponding '.d.ts' file. */
// "sourceMap": true, /* Generates corresponding '.map' file. */
// "outFile": "./", /* Concatenate and emit output to single file. */
"outDir": "** / *", ★ /* Redirect output structure to the directory. */
"rootDir": "./", ★ /* Specify the root directory of input files. Use to control the output directory structure with --outDir. */
// "composite": true, /* Enable project compilation */
// "tsBuildInfoFile": "./", /* Specify file to store incremental compilation information */
// "removeComments": true, /* Do not emit comments to output. */
// "noEmit": true, /* Do not emit outputs. */
// "importHelpers": true, /* Import emit helpers from 'tslib'. */
// "downlevelIteration": true, /* Provide full support for iterables in 'for-of', spread, and destructuring when targeting 'ES5' or 'ES3'. */
// "isolatedModules": true, /* Transpile each file as a separate module (similar to 'ts.transpileModule'). */
/* Strict Type-Checking Options */
"strict": true, ★ /* Enable all strict type-checking options. */
// "noImplicitAny": true, /* Raise error on expressions and declarations with an implied 'any' type. */
// "strictNullChecks": true, /* Enable strict null checks. */
// "strictFunctionTypes": true, /* Enable strict checking of function types. */
// "strictBindCallApply": true, /* Enable strict 'bind', 'call', and 'apply' methods on functions. */
// "strictPropertyInitialization": true, /* Enable strict checking of property initialization in classes. */
// "noImplicitThis": true, /* Raise error on 'this' expressions with an implied 'any' type. */
// "alwaysStrict": true, /* Parse in strict mode and emit "use strict" for each source file. */
/* Additional Checks */
// "noUnusedLocals": true, /* Report errors on unused locals. */
// "noUnusedParameters": true, /* Report errors on unused parameters. */
// "noImplicitReturns": true, /* Report error when not all code paths in function return a value. */
// "noFallthroughCasesInSwitch": true, /* Report errors for fallthrough cases in switch statement. */
// "noUncheckedIndexedAccess": true, /* Include 'undefined' in index signature results */
// "noImplicitOverride": true, /* Ensure overriding members in derived classes are marked with an 'override' modifier. */
// "noPropertyAccessFromIndexSignature": true, /* Require undeclared properties from index signatures to use element accesses. */
/* Module Resolution Options */
// "moduleResolution": "node", /* Specify module resolution strategy: 'node' (Node.js) or 'classic' (TypeScript pre-1.6). */
// "baseUrl": "./", /* Base directory to resolve non-absolute module names. */
// "paths": {}, /* A series of entries which re-map imports to lookup locations relative to the 'baseUrl'. */
// "rootDirs": [], /* List of root folders whose combined content represents the structure of the project at runtime. */
// "typeRoots": [], /* List of folders to include type definitions from. */
// "types": [], /* Type declaration files to be included in compilation. */
// "allowSyntheticDefaultImports": true, /* Allow default imports from modules with no default export. This does not affect code emit, just typechecking. */
"esModuleInterop": true, ★ /* Enables emit interoperability between CommonJS and ES Modules via creation of namespace objects for all imports. Implies 'allowSyntheticDefaultImports'. */
// "preserveSymlinks": true, /* Do not resolve the real path of symlinks. */
// "allowUmdGlobalAccess": true, /* Allow accessing UMD globals from modules. */
/* Source Map Options */
// "sourceRoot": "", /* Specify the location where debugger should locate TypeScript files instead of source locations. */
// "mapRoot": "", /* Specify the location where debugger should locate map files instead of generated locations. */
// "inlineSourceMap": true, /* Emit a single file with source maps instead of having a separate file. */
// "inlineSources": true, /* Emit the source alongside the sourcemaps within a single file; requires '--inlineSourceMap' or '--sourceMap' to be set. */
/* Experimental Options */
// "experimentalDecorators": true, /* Enables experimental support for ES7 decorators. */
// "emitDecoratorMetadata": true, /* Enables experimental support for emitting type metadata for decorators. */
/* Advanced Options */
"skipLibCheck": true, /* Skip type checking of declaration files. */
"forceConsistentCasingInFileNames": true /* Disallow inconsistently-cased references to the same file. */
}
}
これで準備完了。
あとはこちらを実行している間は
自動でコンパイルされます🙌✨
停止はcontrol + Cです。
// terminal
$ npm run watch
これをやった後に知ったのですが、
上記の手順を踏まずとも
このコマンドで
watchモードにできるかも。
まだ検証できていないので、
一応最後に書いておきました。
// terminal
$ tsc app.ts -w
全てのtsファイルで
やりたい場合はファイル指定なし
// terminal
$ tsc -w
複数のtsファイルを使用する場合
tscコマンドで
tsconfig.jsonを作成、
自動コンパイルの場合は
🔼を参考にしてください🍀
// terminal
$ tsc --init
あとは単一の時と同じで、
HTMLファイルにjsを読み込んで
tsファイルをコンパイラすればOK⭕️
この場合だと
app.js/app.ts
basic.js/basic.ts
これらが存在しています。
// index.vue
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Understanding TypeScript</title>
<script src="app.js" defer></script>
<script src="basic.js" defer></script>
</head>
<body>
</body>
</html>
書き方
変数: 型
定番の型はこちら。
string, number, boolean, object, array
これ以外にもtupleなどがあります。
enumは確信が持てない限り使用しない方が良い、
と公式でも名言されているので、
union型を使用する方が良さそうです。
TypeScript のオブジェクト型リテラルいろいろ
TypeScriptの型について
Everyday Types
さようなら、TypeScript enum
##object
このオブジェクトに
person.nameで通常は
アクセスできます。
object
このオブジェクトに
person.nameで通常は
アクセスできます。
しかし型定義をするとエラーになります。
これはそういう仕様です。
const person: object = {
name: 'Yossy',
age: 30,
}
console.log(person.name)
person.nameを出力するには
こんな感じで書きます🌱
const person: {
name: string
age: number
} = {
name: 'Yossy',
age: 30,
}
console.log(person.name)
##array
string[]で
文字列の配列に。
number[]で
数値の配列に。
const person: {
name: string
age: number
hobbies: string[]
} = {
name: 'Yossy',
age: 30,
hobbies: ['game', 'art'],
}
console.log(person.hobbies[0])
['art', 0, true]
型をmixさせたい時は
このように指定します。
(string | number | boolean)[]
要素の順番が明確なら
tupleを使用します。
[]を使うので配列っぽいですが、
tupleです。
順番通りに型を指定します。
TypeScriptの型: タプルを定義する (Tuple types)
##void
書き方はアロー関数に似ています。
関数の戻り値がない場合に使用。
この場合はprintResult内で
戻り値が必要ありません。
function add(n1: number, n2: number) {
return n1 + n2
}
function printResult(num: number): void {
console.log('Result' + num)
}
printResult(add(5, 12))
まとめ
自動コンパイルで
効率よくチェックできて良いです🙌✨
本当はがっつり実践的なことを
やりたかったのですが、
最初にできるだけ
ストレスを軽減するのは良いことです🍀
🎈 WPでも公開中です
https://wp.me/pc9NHC-1qd
この記事が気に入ったらサポートをしてみませんか?