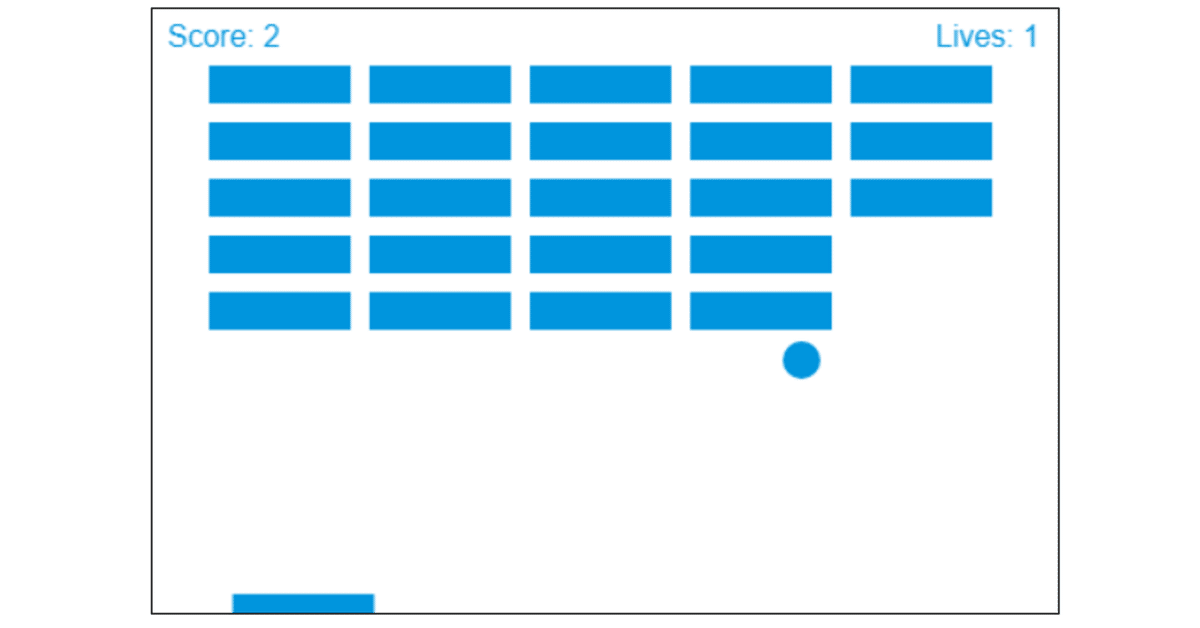
インベーダーゲーム
chatGPT3.5
「インベーダーゲームのようなゲームを web 上で遊びたいのでソースコードください」と chatGPT 3.5 にお願いしたところ、上の画像のようなゲームを頂けました笑
あまりに古くて学習されてないのかもですね・・・記念に頂いたコードをおいておきます。
<!DOCTYPE html>
<html>
<head>
<style>
canvas {
border: 1px solid #000;
display: block;
margin: 0 auto;
}
</style>
</head>
<body>
<canvas id="myCanvas" width="480" height="320"></canvas>
<script>
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
// パドルの初期設定
var paddleHeight = 10;
var paddleWidth = 75;
var paddleX = (canvas.width - paddleWidth) / 2;
// ボールの初期設定
var ballRadius = 10;
var x = canvas.width / 2;
var y = canvas.height - 30;
var dx = 2;
var dy = -2;
// パドルの操作
var rightPressed = false;
var leftPressed = false;
// インベーダーの初期設定
var invaderRowCount = 5;
var invaderColumnCount = 5;
var invaderWidth = 75;
var invaderHeight = 20;
var invaderPadding = 10;
var invaderOffsetTop = 30;
var invaderOffsetLeft = 30;
var invaders = [];
for (var c = 0; c < invaderColumnCount; c++) {
invaders[c] = [];
for (var r = 0; r < invaderRowCount; r++) {
invaders[c][r] = { x: 0, y: 0, status: 1 };
}
}
// スコアとライフ
var score = 0;
var lives = 3;
// キーボードイベント
document.addEventListener("keydown", keyDownHandler, false);
document.addEventListener("keyup", keyUpHandler, false);
function keyDownHandler(e) {
if (e.key == "Right" || e.key == "ArrowRight") {
rightPressed = true;
} else if (e.key == "Left" || e.key == "ArrowLeft") {
leftPressed = true;
}
}
function keyUpHandler(e) {
if (e.key == "Right" || e.key == "ArrowRight") {
rightPressed = false;
} else if (e.key == "Left" || e.key == "ArrowLeft") {
leftPressed = false;
}
}
// 衝突判定
function collisionDetection() {
for (var c = 0; c < invaderColumnCount; c++) {
for (var r = 0; r < invaderRowCount; r++) {
var b = invaders[c][r];
if (b.status == 1) {
if (x > b.x && x < b.x + invaderWidth && y > b.y && y < b.y + invaderHeight) {
dy = -dy;
b.status = 0;
score++;
if (score == invaderRowCount * invaderColumnCount) {
alert("YOU WIN, CONGRATS!");
document.location.reload();
}
}
}
}
}
}
// 描画
function drawBall() {
ctx.beginPath();
ctx.arc(x, y, ballRadius, 0, Math.PI * 2);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
function drawPaddle() {
ctx.beginPath();
ctx.rect(paddleX, canvas.height - paddleHeight, paddleWidth, paddleHeight);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
function drawInvaders() {
for (var c = 0; c < invaderColumnCount; c++) {
for (var r = 0; r < invaderRowCount; r++) {
if (invaders[c][r].status == 1) {
var invaderX = c * (invaderWidth + invaderPadding) + invaderOffsetLeft;
var invaderY = r * (invaderHeight + invaderPadding) + invaderOffsetTop;
invaders[c][r].x = invaderX;
invaders[c][r].y = invaderY;
ctx.beginPath();
ctx.rect(invaderX, invaderY, invaderWidth, invaderHeight);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
}
}
}
function drawScore() {
ctx.font = "16px Arial";
ctx.fillStyle = "#0095DD";
ctx.fillText("Score: " + score, 8, 20);
}
function drawLives() {
ctx.font = "16px Arial";
ctx.fillStyle = "#0095DD";
ctx.fillText("Lives: " + lives, canvas.width - 65, 20);
}
// ゲームループ
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawInvaders();
drawBall();
drawPaddle();
drawScore();
drawLives();
collisionDetection();
if (x + dx > canvas.width - ballRadius || x + dx < ballRadius) {
dx = -dx;
}
if (y + dy < ballRadius) {
dy = -dy;
} else if (y + dy > canvas.height - ballRadius) {
if (x > paddleX && x < paddleX + paddleWidth) {
dy = -dy;
} else {
lives--;
if (!lives) {
alert("GAME OVER");
document.location.reload();
} else {
x = canvas.width / 2;
y = canvas.height - 30;
dx = 2;
dy = -2;
paddleX = (canvas.width - paddleWidth) / 2;
}
}
}
if (rightPressed && paddleX < canvas.width - paddleWidth) {
paddleX += 7;
} else if (leftPressed && paddleX > 0) {
paddleX -= 7;
}
x += dx;
y += dy;
requestAnimationFrame(draw);
}
document.addEventListener("mousemove", mouseMoveHandler, false);
function mouseMoveHandler(e) {
var relativeX = e.clientX - canvas.offsetLeft;
if (relativeX > 0 && relativeX < canvas.width) {
paddleX = relativeX - paddleWidth / 2;
}
}
draw();
</script>
</body>
</html>
google で検索した場合
ちなみに google にて「invador game」 で検索した場合の画像としては以下のとおり。こういうのが動くことを期待してたんよね笑
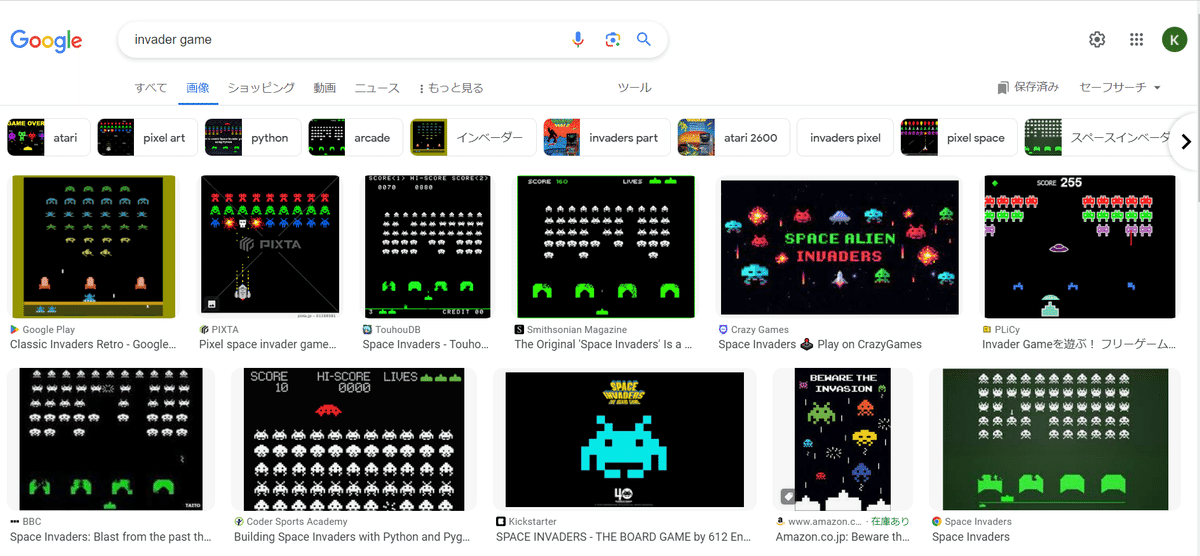
以上です。