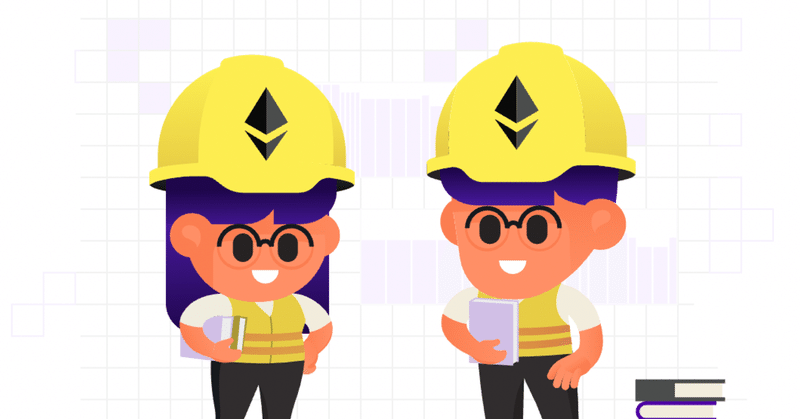
Hardhat入門④(コントラクトの作成) ~ブロックチェーンエンジニアになろう~
なんと、この第4章ではとうとうコントラクトを作ることになります。楽しみですね。
今回もHardhat公式のチュートリアルに沿って進んでいきますね。
コントラクトの作成
今回はトークンのコントラクトを作ります。
ERC20というのをご存知でしょうか?DAIやUSDCといった様々なトークンが存在します。
今回は直接のERC20ではありませんが、そのような仮想通貨のようなトークン用のコントラクトを作ってみましょう。
今回からsolidityという言語を扱うことになりますがその説明はざっくりにします。
こちらはHardhatの入門のためです。
機会があればsolidity入門についても書いていきたいと思います。
まずは「contracts」というフォルダに「Token.sol」というファイルを作ります。
「.sol」というのはsolidityのマーク(拡張子)です。
また、「contracts」フォルダがなければ作ってみてください。
まさにコントラクトを作りますので「contracts」フォルダに入れるのですね。
作りましたら、次のコードをコピペして「Token.sol」に入れてください。(チュートリアルにあるものそのままです。)
// Solidity files have to start with this pragma.
// It will be used by the Solidity compiler to validate its version.
pragma solidity ^0.7.0;
// This is the main building block for smart contracts.
contract Token {
// Some string type variables to identify the token.
// The `public` modifier makes a variable readable from outside the contract.
string public name = "My Hardhat Token";
string public symbol = "MHT";
// The fixed amount of tokens stored in an unsigned integer type variable.
uint256 public totalSupply = 1000000;
// An address type variable is used to store ethereum accounts.
address public owner;
// A mapping is a key/value map. Here we store each account balance.
mapping(address => uint256) balances;
/**
* Contract initialization.
*
* The `constructor` is executed only once when the contract is created.
*/
constructor() {
// The totalSupply is assigned to transaction sender, which is the account
// that is deploying the contract.
balances[msg.sender] = totalSupply;
owner = msg.sender;
}
/**
* A function to transfer tokens.
*
* The `external` modifier makes a function *only* callable from outside
* the contract.
*/
function transfer(address to, uint256 amount) external {
// Check if the transaction sender has enough tokens.
// If `require`'s first argument evaluates to `false` then the
// transaction will revert.
require(balances[msg.sender] >= amount, "Not enough tokens");
// Transfer the amount.
balances[msg.sender] -= amount;
balances[to] += amount;
}
/**
* Read only function to retrieve the token balance of a given account.
*
* The `view` modifier indicates that it doesn't modify the contract's
* state, which allows us to call it without executing a transaction.
*/
function balanceOf(address account) external view returns (uint256) {
return balances[account];
}
}
中身について、本当にざっくりと。
contract Token {
あーだこーだ
}
まずはこれが大きな括りです。
「Token」というコントラクトを作りますと宣言し、その中身が書いてあります。
constructor() {
あーだこーだ
}
上と名前がとても似ているのですが、これはコンストラクタです。
コントラクトをデプロイするときに、一番最初にやってねというのがこちらです。
function transfer(address to, uint256 amount) external {
あーだこーだ
}
function balanceOf(address account) external view returns (uint256) {
あーだこーだ
}
こちらがfunction(機能)です。
このコントラクトには
① transfer
②balanceOf
の2つが存在するようですね。
コンパイル
さて、次はコンパイルです。
デプロイとは違いますのでご注意ください。
ざっくり言いますと、私たちが作ったsolidityのファイル(.sol)を機械に読んでもらうために機械語に変換することです。
さっそくやってみましょう。
ターミナルに戻って、次を実行してください。
npx hardhat compile
やってみたらこんな風になりました。
黄色の状態で色々と警告を受けてはいますが、赤くなっておらず、一番下に
Compilation finished successfully
と出ていますのでひとまず成功です。
これでとうとうコントラクトを作成し、コンパイルすることができるようになりましたね。
続きは次回です。
サポートをしていただけたらすごく嬉しいです😄 いただけたサポートを励みに、これからもコツコツ頑張っていきます😊