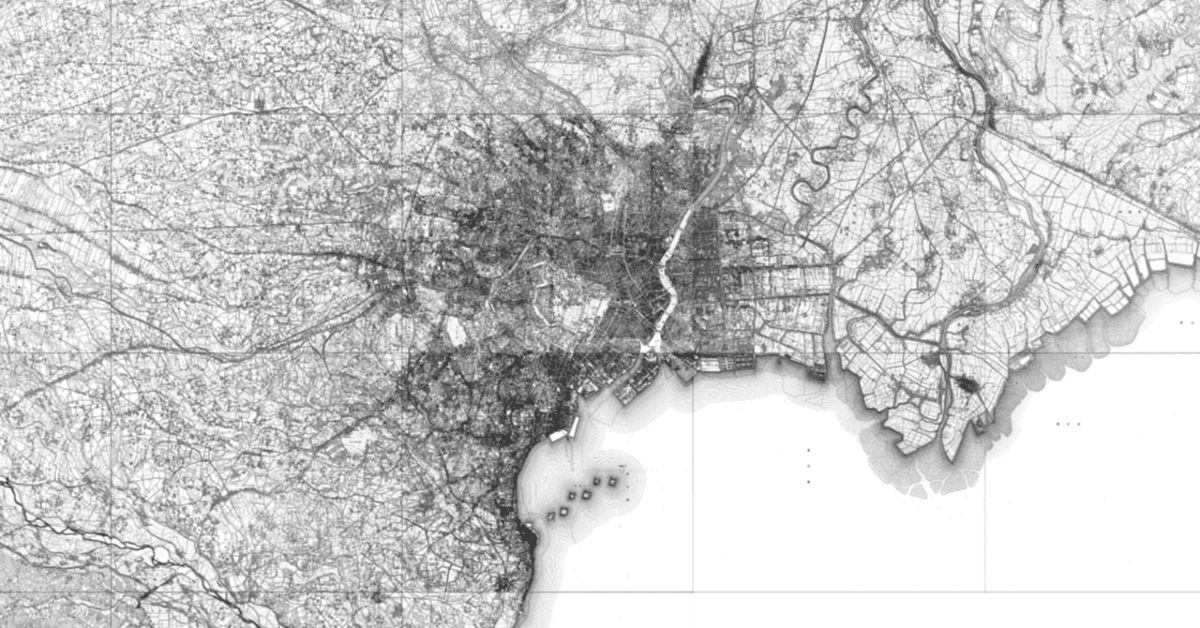
[GIS] #16 How can I use python to display GSI tiles and Konjyaku Map (Time series topographic map viewer of Japan) tile map?
This article shows how to load, display, and save Geospatial Information Authority of Japan (GSI )Tiles and Konjaku Map (Time series topographic map viewer of Japan) in python.
This is just a small tip for loading the tile maps in python, especially for the Konjaku Map. The same method can be used for any map provided in a tile map service, not limited to the Konjaku Map.
The key point is the methodology of the staticmap library.
Preparation: staticmap installation
The operation was verified by building a new environment on Windows 10 with Anaconda (Jupyter Notebook) as the IDE. The staticmap library was installed at the command prompt using the usual pip install method.
(GIS) C:\Users\Kunimichi> pip install staticmap
Pillow, the image library, and request, the WEB-API control library, are automatically installed.
Case Studies
Example 1: GSI Tile (Standard Map)
The standard GSI Tile map has the following description style.
https://cyberjapandata.gsi.go.jp/xyz/std/{z}/{x}/{y}.png
The GSI tiles are available in a variety of thematic maps, so you can change the settings to obtain output according to your preference.
Below is an example of standard map output by staticmap (Jupyter Notebook notation).
# Library Designation
from staticmap import StaticMap
# Tile Map (GSI Tile)
TILE_MAP = 'https://cyberjapandata.gsi.go.jp/xyz/std/{z}/{x}/{y}.png'
# Setting
Lon_Lat = [139.76730676352,35.680959106959] # Lon and Lat of Tokyo Station.
ZOOM = 12 # Zoom Level
WIDTH = 1200 # Width in pxcel
HEIGHT = 800 # Height in pixcel
# staticmap object
m = StaticMap(width = WIDTH, height = HEIGHT,
url_template = TILE_MAP
)
# rendering(visualization)
map = m.render(zoom = ZOOM, center= Lon_Lat)
map
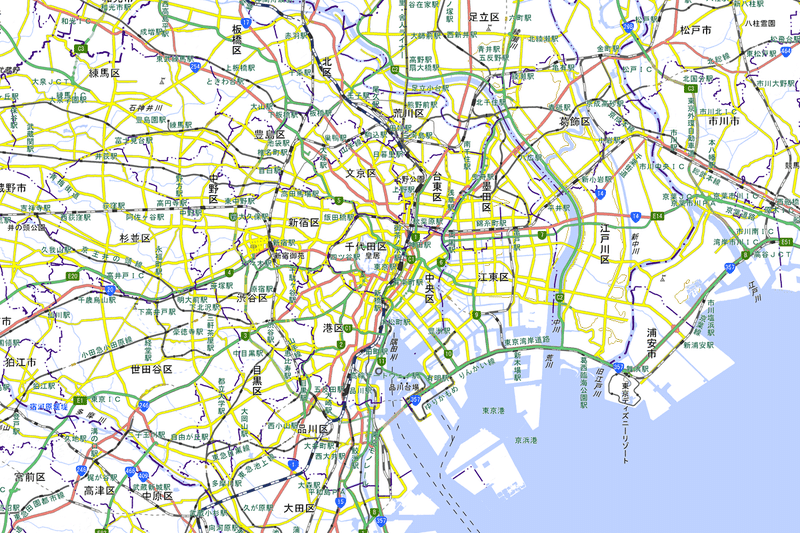
The map can be saved using Pillow's save method.
# png saving
map.save('gsi_standard.png')
Example 2: Konjaku Map (Time series topographic map viewer of Japan)
The tile map service of Konjaku Map has the following description style.
http://ktgis.net/kjmapw/kjtilemap/{dataset folder}/{term folder}/{z}/{x}/{y}.png
The origin point of the tile map is important to keep in mind. The origin is South West for Konjaku Map, which is different from the North West in the GSI tile. This causes an error in the staticmap default setting.
To avoid this error, reverse_y=True must be given as an argument in the StaticMap class options.
The following is an example of drawing the "1896-1909" metropolitan area on the Konjaku Map.
from staticmap import StaticMap
#Tile Map(Metropolitan Area in 1896-1909)
TILE_MAP = 'https://ktgis.net/kjmapw/kjtilemap/tokyo50/2man/{z}/{x}/{y}.png'
# Setting
Lon_Lat = [139.76730676352,35.680959106959] # Lon and Lat of Tokyo Station.
ZOOM = 12 # Zoom Level
WIDTH = 1200 # Width in pxcel
HEIGHT = 800 # Height in pixcel
# staticmap object
m = StaticMap(width = WIDTH, height = HEIGHT,
url_template = TILE_MAP,
reverse_y = True
)
# rendering(visualization)
map = m.render(zoom = ZOOM, center= Lon_Lat)
map
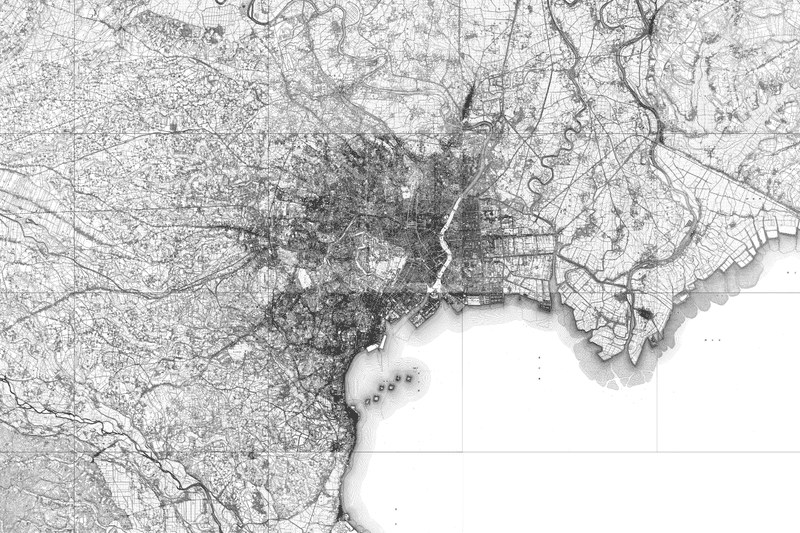
The map can also be saved using Pillow's save method.
# png saving
map.save('konjyaku_tokyo50_2man.png')
Syntax
Basic syntax of the StaticMap class.
https://github.com/komoot/staticmap/blob/master/staticmap/staticmap.py
m = StaticMap(width, height,
padding_x=0, padding_y=0,
url_template="http://a.tile.komoot.de/komoot-2/{z}/{x}/{y}.png",
tile_size=256,
tile_request_timeout=None,
headers=None,
reverse_y=False,
background_color="#fff",
delay_between_retries=0
)
Typical arguments of the StaticMap class are as follows
width: Horizontal size of the map (in pixels)
height: vertical size of the map (in pixels)
url_template (optional): URL address of the tile map server
reverse_y (optional): Tile origin point. Default is False (origin point: northwest). True if the origin point is "southwest".
render method
map = m.render(zoom=None, center=None)
The render method acts on a StaticMap instance. The arguments are as follows
zoom: Zoom level
center: The center point of the drawing is given as a [longitude, latitude] list.
Reference
[1] Static Map:
[2] GSI Tile (in Japanese)
[3] Konjyaku map: Tile map service (in Japanese)
この記事が気に入ったらサポートをしてみませんか?