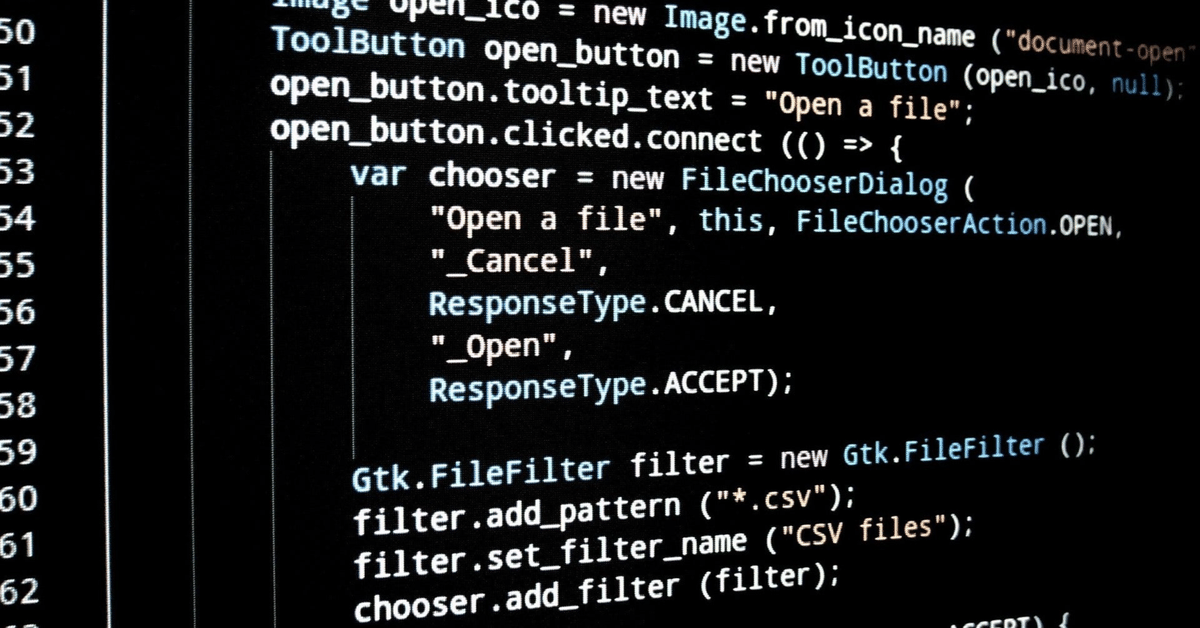
Photo by
ryonakano
アイズオンラインジャッジメント(AOJ)のプログラムコード置き場(C#) [ITP1_10]
こんにちは。シジミです。
この記事はAOJで回答したコードを随時置いていきます。
構成としまして、以下のように三弾構成にしています。
環境はvisual sutadio 2019、言語はC#です。
問題:ITP1_10_A
解説:2点間の距離は三平方の定理で求められます。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
namespace ITP_1_10A
{
class Program
{
static void Main(string[] args)
{
var N = Console.ReadLine().Trim().Split(' ').Select(s => double.Parse(s)).ToArray();
double distance = Math.Sqrt( Math.Pow(N[0] - N[2], 2)+Math.Pow(N[1] - N[3],2));
Console.WriteLine(distance);
return;
}
}
}
問題:ITP1_10_B
解説:残る一辺は余弦定理で導きます。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
namespace ITP_1_10B
{ class Program
{
static void Main(string[] args)
{
var N = Console.ReadLine().Trim().Split(' ').Select(s => double.Parse(s)).ToArray();
double sita = N[2] * Math.PI / 180;
double c = Math.Sqrt(Math.Pow(N[0], 2) + Math.Pow(N[1], 2) - 2 * N[0] * N[1]*Math.Cos(sita));
double h = N[1] * Math.Sin(sita);
Console.WriteLine("{0} {1} {2}",N[0]*h/2,N[0]+N[1]+c,h);
return;
}
}
}
問題:ITP1_10_C
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
namespace ITP_1_10C
{
class Program
{
static void Main(string[] args)
{
double a,n,s,m;
while (true)
{
a = n = s = m = 0;
var N = Console.ReadLine();
n = Int32.Parse(N);
if ( n == 0)
{
return;
}
var Array = Console.ReadLine().Trim().Split(' ').Select(x => double.Parse(x)).ToArray();
m = Array.Sum() / n;
foreach (var item in Array)
{
s += Math.Pow(item - m, 2);
}
a = Math.Sqrt(s / n);
Console.WriteLine(a);
}
return;
}
}
}
問題:ITP1_10_D
解説:注意点として、べき乗根はint型だと0として計算されるみたいです。double型にすると1/3など期待している根で計算してくれます。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
namespace ITP_1_10D
{
class Program
{
static void Main(string[] args)
{
int n = 0;
var N = Console.ReadLine();
n = Int32.Parse(N);
var X = Console.ReadLine().Trim().Split(' ').Select(s => double.Parse(s)).ToArray();
var Y = Console.ReadLine().Trim().Split(' ').Select(s => double.Parse(s)).ToArray();
double[] a = new double[n];
double d;
for (int i = 0; i < n; i++)
{
a[i] = Math.Abs(X[i] - Y[i]);
}
for (double i = 1; i < 5; i++)
{
d = 0;
if (i == 4)
{
Console.WriteLine(a.Max());
}
else
{
for (int j = 0; j < n; j++)
{
d += Math.Pow(a[j], i);
}
Console.WriteLine(Math.Pow(d, 1 / i));
}
}
return;
}
}
}
この記事が気に入ったらサポートをしてみませんか?