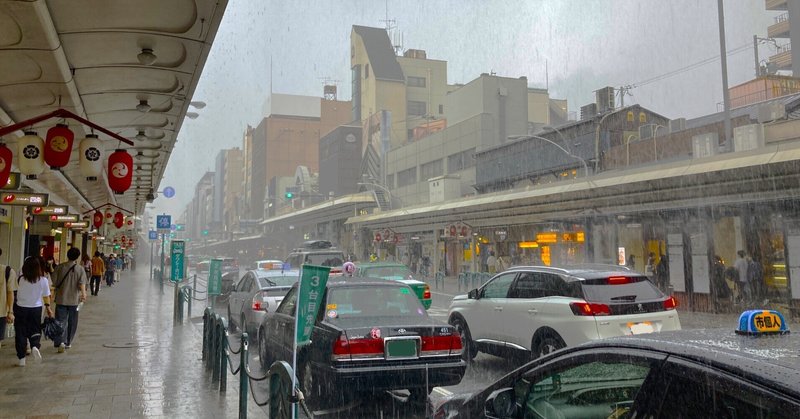
ChatGPTを用いたプログラミング:C言語での数値計算
OpenAI が開発した対話に特化した人工知能チャットボットのプロトタイプであるChatGPTを用いて、C言語のプログラムコードを書かせてみた。うまく使えば、学習に行き詰ったときに便利なツールとなるかもしれない。ChatGPTは、日本語でも使用可能だが、英語で使用した方がおもしろいと思う。
以前、仮想的な議論を行わせてみたときの記録のリンクを載せておく。
円の面積
---Write a code of C language that calculate the area of circle.
Here is a C program that calculates the area of a circle:
Copy code
#include <stdio.h>
#define PI 3.14159
int main(void) {
float radius, area;
printf("Enter the radius of the circle: ");
scanf("%f", &radius);
area = PI * radius * radius;
printf("The area of the circle is: %.2f\n", area);
return 0;
}
This program takes user input for the radius of the circle, calculates the area using the formula area = PI * radius * radius, and then prints the result to the console. The value of PI is defined as a constant at the top of the program using the #define directive. This allows us to use the symbol PI throughout the program to represent the value of PI.
I hope this helps! Let me know if you have any questions.
3整数の最大公約数
---Write a code of C language that calculate the GCD of 3 numbers.
Here is a C program that calculates the greatest common divisor (GCD) of three numbers:
#include <stdio.h>
int main(void) {
int a, b, c, gcd;
printf("Enter the first number: ");
scanf("%d", &a);
printf("Enter the second number: ");
scanf("%d", &b);
printf("Enter the third number: ");
scanf("%d", &c);
// Calculate the GCD of the three numbers
int i;
for (i = 1; i <= a && i <= b && i <= c; i++) {
if (a % i == 0 && b % i == 0 && c % i == 0) {
gcd = i;
}
}
printf("The GCD of %d, %d, and %d is: %d\n", a, b, c, gcd);
return 0;
}
This program takes user input for the three numbers, and then calculates the GCD using a loop. The loop starts at 1 and goes up to the minimum of the three numbers. For each iteration of the loop, it checks if the current number (i) is a divisor of all three of the numbers. If it is, then it sets gcd to the current number. After the loop has completed, the program prints the result to the console.
I hope this helps! Let me know if you have any questions.
三体問題
---Write a code of C language that calculate three body problem.
The three-body problem is a mathematical problem that involves three celestial bodies, such as planets or asteroids, that are interacting with each other due to the gravitational forces between them. It is a difficult problem to solve because it involves finding the motion of the three bodies over time, which can be very complex due to the interactions between them.
There are various ways to approach the three-body problem in C, and the specific approach will depend on the specific requirements of the problem. Here is one possible way to solve the three-body problem using C:
Define the initial positions and velocities of the three bodies.
Define the masses of the three bodies.
Define the gravitational constant (G).
Define the time step (dt) for the simulation.
Define the total time (t) for the simulation.
Set up a loop that will iterate for each time step.
Inside the loop, use the equations of motion for the three-body problem to calculate the new positions and velocities of the three bodies.
Update the positions and velocities of the three bodies.
Repeat the loop until the total time has been reached.
Here is an example of C code that implements this approach:
#include <stdio.h>
#include <math.h>
#define G 6.67408e-11 // gravitational constant
#define dt 0.01 // time step
#define t 10 // total time
// Structure to represent a celestial body
typedef struct {
double x; // x-coordinate
double y; // y-coordinate
double z; // z-coordinate
double vx; // x-velocity
double vy; // y-velocity
double vz; // z-velocity
double mass; // mass
} Body;
int main(void) {
// Initialize the three bodies
Body body1 = {1, 0, 0, 0, 0.1, 0, 1};
Body body2 = {0, 1, 0, -0.1, 0, 0, 1};
Body body3 = {0, 0, 1, 0, 0, -0.1, 1};
// Loop through the simulation
int i;
for (i = 0; i < t / dt; i++) {
// Calculate the acceleration of each body
double ax1 = (G * body2.mass * (body2.x - body1.x) / pow(pow(body2.x - body1.x, 2) + pow(body2.y - body1.y, 2) + pow(body2.z - body1.z, 2), 1.5)) + (G * body3.mass * (body3.x - body1.x) / pow(pow(body3.x - body1.x, 2) + pow(body3.y - body1.y, 2) + pow(body3.z - body1.z, 2), 1.5));
double ay1 = (G * body2.mass * (body2.y - body1.y) / pow(pow(body2.x - body1.x, 2) + pow(body2.y - body1.y, 2) + pow(body2.z - body1.z, 2), 1.5)) + (G * body3.mass * (body3.y - body1.y) / pow(pow(body3.x - body1.x, 2) + pow(body3.y - body1.y, 2) + pow(body3.z - body1.z, 2), 1.5));
double az1 = (G * body2.mass * (body2.z - body1.z) / pow(pow(body2.x - body1.x, 2) + pow(body2.y - body1.y, 2) + pow(body2.z - body1.z, 2), 1.5)) + (G * body3.mass * (body3.z - body1.z) / pow(pow(body3.x - body1.x, 2) + pow(body3.y - body1.y, 2) + pow(body3.z - body1.z, 2), 1.5));
double ax2 = (G * body1.mass * (body1.x - body2.x) / pow(pow(body1.x - body2.x, 2) + pow(body1.y - body2.y, 2) + pow(body1.z - body2.z, 2), 1.5)) + (G * body3.mass * (body3.x - body2.x) / pow(pow(body3.x - body2.x, 2) + pow(body3.y - body2.y, 2) + pow(body3.z - body2.z, 2), 1.5));
double ay2 = (G * body1.mass * (body1.y - body2.y) / pow(pow(body1.x - body2.x, 2) + pow(body1.y - body2.y, 2) + pow(body1.z - body2.z, 2), 1.5)) + (G * body3.mass * (body3.y - body2.y) / pow(pow(body3.x - body2.x, 2) + pow(body3.y - body2.y, 2) + pow(body3.z - body2.z, 2), 1.5));
double az2 = (G * body1.mass * (body1.z - body2.z) / pow(pow(body1.x - body2.x, 2) + pow(body1.y - body2.y, 2) + pow(body1.z - body2.z, 2), 1.5)) + (G * body3.mass * (body3.z - body2.z) / pow(pow(body3.x - body2.x, 2) + pow(body3.y - body2.y, 2) + pow(body3.z - body2.z, 2), 1.5));
double ax3 = (G * body1.mass * (body1.x - body3.x) / pow(pow(body1.x - body3.x, 2) + pow(body1.y - body3.y, 2) + pow(body1.z - body3.z, 2), 1.5)) + (G * body2.mass * (body2.x - body3.x) / pow(pow(body2.x - body3.x, 2) + pow(body2.y - body3.y, 2) + pow(body2.z - body3.z, 2), 1.5));
double ay3 = (G * body1.mass * (body1.y - body3.y) / pow(pow(body1.x - body3.x, 2) + pow(body1.y - body3.y, 2) + pow(body1.z - body3.z, 2), 1.5)) + (G * body2.mass * (body2.y - body3.y) / pow(pow(body2.x - body3.x, 2) + pow(body2.y - body3.y, 2) + pow(body2.z - body3.z, 2), 1.5));
double az3 = (G * body1.mass * (body1.z - body3.z) / pow(pow(body1.x - body3.x, 2) + pow(body1.y - body3.y, 2) + pow(body1.z - body3.z, 2), 1.5)) + (G * body2.mass * (body2.z - body3.z) / pow(pow(body2.x - body3.x, 2) + pow(body2.y - body3.y, 2) + pow(body2.z - body3.z, 2), 1.5));
// Update the velocities of each body
body1.vx += ax1 * dt;
body1.vy += ay1 * dt;
body1.vz += az1 * dt;
body2.vx += ax2 * dt;
body2.vy += ay2 * dt;
body2.vz += az2 * dt;
body3.vx += ax3 * dt;
body3.vy += ay3 * dt;
body3.vz += az3 * dt;
// Update the positions of each body
body1.x += body1.vx * dt;
body1.y += body1.vy * dt;
body1.z += body1.vz * dt;
body2.x += body2.vx * dt;
body2.y += body2.vy * dt;
body2.z += body2.vz * dt;
body3.x += body3.vx * dt;
body3.y += body3.vy * dt;
body3.z += body3.vz * dt;
}
// Print the final positions of the bodies
printf("Body 1: (%.2f, %.2f, %.2f)\n", body1.x, body1.y, body1.z);
printf("Body 2: (%.2f, %.2f, %.2f)\n", body2.x, body2.y, body2.z);
printf("Body 3: (%.2f, %.2f, %.2f)\n", body3.x, body3.y, body3.z);
return 0;
}
This code defines a `Body` structure to represent a celestial body, and then creates three `Body` objects to represent the three celestial bodies in the simulation. It then sets up a loop to iterate through the simulation, using the equations of motion for the three-body problem to calculate the new positions and velocities of the bodies at each time step. Finally, it prints the final positions of the bodies to the console.
I hope this helps! Let me know if you have any questions.
-----
動け!タイムライン
動物園か水族館にいきたいですね。