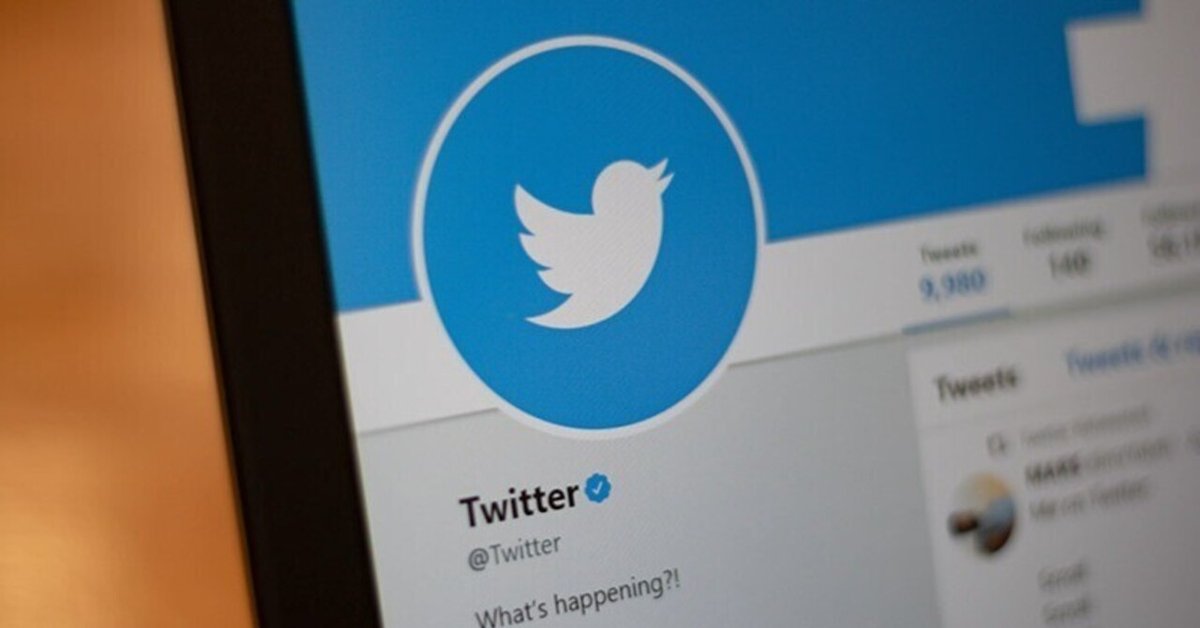
Pythonを使いTwitterの動画を保存する方法
まず最初に、所有者の許可なしに Twitter から動画をダウンロードすることは Twitter の利用規約に反するため、このコードは自己責任で使用してください。
以下は、Twitter の動画を保存できるプログラムの Python コードです。
import requests
import re
from bs4 import BeautifulSoup
# Define the Twitter video URL
video_url = "https://twitter.com/TwitterVideo/status/1234567890123456789"
# Send a GET request to the Twitter video URL
response = requests.get(video_url)
# Parse the HTML content using BeautifulSoup
soup = BeautifulSoup(response.content, 'html.parser')
# Find the video URL in the HTML content
video_element = soup.find('meta', {'property': 'og:video'})
video_url = video_element['content']
# Extract the video ID from the video URL
video_id = re.search(r'/status/(\d+)', video_url).group(1)
# Define the download URL for the video
download_url = f"https://twitter.com/i/videos/tweet/{video_id}?src=5"
# Send a GET request to the download URL
response = requests.get(download_url)
# Extract the video URL from the response JSON data
data = response.json()
video_url = data['track']['playbackUrl']
# Send a GET request to the video URL and save the video
response = requests.get(video_url)
with open(f"{video_id}.mp4", "wb") as f:
f.write(response.content)
このプログラムでは、まず Twitter の動画 URL に GET リクエストを送信し、BeautifulSoup を使用して動画の URL を抽出します。次に、動画 URL から動画 ID を抽出し、それを使用してダウンロード URL を作成します。ダウンロード URL に GET リクエストを送信し、レスポンスの JSON データから動画 URL を抽出します。最後に、動画の URL に GET リクエストを送信し、動画を MP4 ファイルとして保存します。
このプログラムを使用するには、'video_url'変数を保存したい Twitter ビデオの URL に置き換えて、プログラムを実行します。プログラムは、ビデオをプログラム ファイルと同じディレクトリに保存します。
この記事が気に入ったらサポートをしてみませんか?