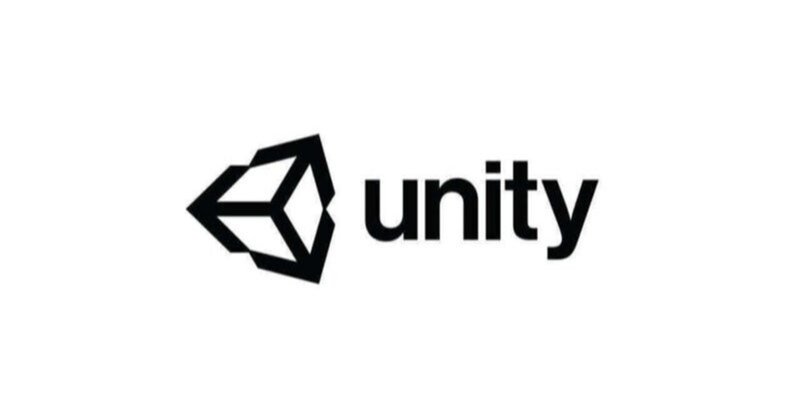
Unity InputSystem 入門 (1) - 事始め
Unityの「InputSystem」での「キーボード」と「マウス」の使い方をまとめました。
・Unity 2020.3.1f1
・InputSystem 1.0.3
1. InputSystem
「InputSystem」は、「InputManager」に変わるUnityの新しい入力システムです。
2. InputSystemのインストール
(1) 「Package Manager」で「InputSystem」をインストール。
(2) Unityメニュー「Edit → Project Settings」の「Player → Other Settings → Configuration → Active Input Handling」で「Input System Package」を選択。
選択項目は以下の3つです。
・Input Manager (Old)
・Input System Package (New)
・Both
(3) Unityメニュー「Edit → Project Settings」の「Input System Package」で「Create settings asset」をクリック。
「Assets」に「InputSystem.inputsettings.asset」が生成されます。
3. InputSystemの設定
Unityメニュー「Edit → Project Settings」の「Input System Package」でInputSystemの設定ができます。
◎ Update Mode
入力情報の更新タイミングを指定します。
・Process Events In Dynamic Update : Update()毎に入力情報を更新。
・Process Events In Fixed Update : FixedUpdate()毎に入力情報を更新。
・Process Events In Manually : スクリプトからInputSystem.Update()を呼んだ時に入力情報を更新。
◎ Filter Noise on current
入力ノイズをカットします。(PS4コントローラのジャイロなど)
◎ Compensate Orientation
センサーによって得られる回転値をZ軸を中心に回転させます。
・ScreenOrientation.Portrait : 0度
・ScreenOrientation.PortraitUpsideDown : 180度
・ScreenOrientation.LandscapeLeft : 90度
・ScreenOrientation.LandscapeRight : 270度
◎ デフォルト値
各プロパティのデフォルト値を指定します。
・Default Deadzone Min : Stick DeadzoneまたはAxis Deadzone processorsで最小値が設定されていない場合のデフォルト値。
・Default Deadzone Max : Stick DeadzoneまたはAxis Deadzone processorsで最大値が設定されていない場合のデフォルト値。
・Default Button Press Point : デフォルトのボタン押下値。アナログ物理入力ボタン(ゲームパッドのトリガーなど)では、押されたと判断するために、どの程度押し続ける必要があるかを設定。
・Default Tap Time : タップおよびマルチタップのデフォルト時間。タッチスクリーンで、新しいタップを区別するために使用。
・Default Slow Tap Time : スロータップのデフォルト時間。
・Default Hold Time : ホールドタップのデフォルト時間。
・Tap Radius : 2つのタップの最大距離。タッチスクリーンで、新しいタップを区別するために使用。
・Multi Tap Delay Time : マルチタップのタップ間のデフォルトの遅延時間。また、タッチスクリーンがマルチタップをカウントする際にも使用。
◎ Supported Devices
認識出来るデバイスを限定したい時に指定します。
4. キーボード入力
現在の「キーボード」の状態は、「Keyboard.current」で取得します。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.InputSystem;
using UnityEngine.InputSystem.Controls;
public class Main : MonoBehaviour
{
void Update()
{
// キーボードの状態の取得
var keyboard = Keyboard.current;
if (keyboard != null) {
// スペースキーの状態の取得
if (keyboard.spaceKey.wasPressedThisFrame) Debug.Log("SpaceKey:Down");
if (keyboard.spaceKey.wasReleasedThisFrame) Debug.Log("SpaceKey:Up");
}
}
}
◎ キーのプロパティ
Keyboardオブジェクトが持つ「キー」のプロパティは、次のとおりです。
・KeyControl aKey
・KeyControl allKeys
・KeyControl altKey
・KeyControl anyKey
・KeyControl backquoteKey
・KeyControl backslashKey
・KeyControl backspaceKey
・KeyControl bKey
・KeyControl cKey
・KeyControl commaKey
・KeyControl contextMenuKey
・KeyControl ctrlKey
・KeyControl digit0Key
・KeyControl digit1Key
・KeyControl digit2Key
・KeyControl digit3Key
・KeyControl digit4Key
・KeyControl digit5Key
・KeyControl digit6Key
・KeyControl digit7Key
・KeyControl digit8Key
・KeyControl digit9Key
・KeyControl dKey
・KeyControl downArrowKey
・KeyControl eKey
・KeyControl endKey
・KeyControl enterKey
・KeyControl equalsKey
・KeyControl escapeKey
・KeyControl f10Key
・KeyControl f11Key
・KeyControl f12Key
・KeyControl f1Key
・KeyControl f2Key
・KeyControl f3Key
・KeyControl f4Key
・KeyControl f5Key
・KeyControl f6Key
・KeyControl f7Key
・KeyControl f8Key
・KeyControl f9Key
・KeyControl fKey
・KeyControl gKey
・KeyControl hKey
・KeyControl homeKey
・KeyControl iKey
・KeyControl imeSelected
・KeyControl insertKey
・KeyControl jKey
・KeyControl keyboardLayout
・KeyControl kKey
・KeyControl leftAltKey
・KeyControl leftAppleKey
・KeyControl leftArrowKey
・KeyControl leftBracketKey
・KeyControl leftCommandKey
・KeyControl leftCtrlKey
・KeyControl leftMetaKey
・KeyControl leftShiftKey
・KeyControl leftWindowsKey
・KeyControl lKey
・KeyControl minusKey
・KeyControl mKey
・KeyControl nKey
・KeyControl numLockKey
・KeyControl numpad0Key
・KeyControl numpad1Key
・KeyControl numpad2Key
・KeyControl numpad3Key
・KeyControl numpad4Key
・KeyControl numpad5Key
・KeyControl numpad6Key
・KeyControl numpad7Key
・KeyControl numpad8Key
・KeyControl numpad9Key
・KeyControl numpadDivideKey
・KeyControl numpadEnterKey
・KeyControl numpadEqualsKey
・KeyControl numpadMinusKey
・KeyControl numpadMultiplyKey
・KeyControl numpadPeriodKey
・KeyControl numpadPlusKey
・KeyControl oem1Key
・KeyControl oem2Key
・KeyControl oem3Key
・KeyControl oem4Key
・KeyControl oem5Key
・KeyControl oKey
・KeyControl pageUpKey
・KeyControl pauseKey
・KeyControl periodKey
・KeyControl pKey
・KeyControl printScreenKey
・KeyControl qKey
・KeyControl quoteKey
・KeyControl rightAltKey
・KeyControl rightAppleKey
・KeyControl rightArrowKey
・KeyControl rightBracketKey
・KeyControl rightCommandKey
・KeyControl rightCtrlKey
・KeyControl rightMetaKey
・KeyControl rightShiftKey
・KeyControl rightWindowsKey
・KeyControl rKey
・KeyControl scrollLockKey
・KeyControl semicolonKey
・KeyControl shiftKey
・KeyControl sKey
・KeyControl slashKey
・KeyControl spaceKey
・KeyControl tabKey
・KeyControl tKey
・KeyControl uKey
・KeyControl upArrowKey
・KeyControl vKey
・KeyControl wKey
・KeyControl xKey
・KeyControl yKey
・KeyControl zKey
◎ キー状態のプロパティ
キーが持つ「キー状態」のプロパティは、次のとおりです。
・bool isPressed : 押されているかどうか。
・bool wasPressedThisFrame : 押された瞬間かどうか。
・bool wasReleasedThisFrame : 離された瞬間かどうか。
5. マウス入力
現在の「マウス」の状態は、「Mouse.current」で取得します。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.InputSystem;
using UnityEngine.InputSystem.Controls;
public class Main : MonoBehaviour
{
void Update()
{
// マウスの状態の取得
var mouse = Mouse.current;
if (mouse != null) {
// マウスポインタの状態の取得
Debug.Log("MousePosition:"+mouse.position.ReadValue());
// マウス左ボタンの状態の取得
if (mouse.leftButton.wasPressedThisFrame) Debug.Log("MouseLeftButton:Down");
if (mouse.leftButton.wasReleasedThisFrame) Debug.Log("MouseLeftButton:Up");
}
}
}
◎ ポインタのプロパティ
Mouseオブジェクトが持つ「ポインタ」のプロパティは、次のとおりです。
・Vector2Control position : ウィンドウ空間での現在のポインタ座標。
・Vector2Control delta : 前フレームからのポインタ座標の差。
「Vector2Control」は、ReadValue()でVector2の値を取得できます。
◎ ボタンのプロパティ
Mouseオブジェクトが持つ「ボタン」のプロパティは、次のとおりです。
・ButtonControl leftButton : マウスの左ボタン。
・ButtonControl rightButton : マウスの右ボタン。
・ButtonControl middleButton : マウスの中央ボタン。
・ButtonControl forwardButton : マウスのフォワードボタン。
・ButtonControl backButton : マウスのバックボタン。
・IntegerControl clickCount : 連続クリック数(ダブルクリックの判別に利用)。
・Vector2Control scroll : スクロール。
◎ ボタン状態のプロパティ
ボタンが持つ「ボタン状態」のプロパティは、次のとおりです。
・bool isPressed : 押されているかどうか。
・bool wasPressedThisFrame : 押された瞬間かどうか。
・bool wasReleasedThisFrame : 離された瞬間かどうか。
6. EventSystemでのInputSystemの利用
UI操作のため「EventSystem」を使う時は、「Event System → Standalone Input Module → Replace with InputSystemUIInputModule」を押して、InputSystemに置き換える必要があります。
【おまけ】 カメラコントローラ
「InputSystem」でカメラを移動させるコードを作成します。
・WSADキー : カメラの前後左右移動。
・QEキー : カメラの上下移動。
・マウス左ボタンのドラッグ : カメラ向きの変更。
以下のコードを「カメラ」に追加することで、カメラの位置と角度を操作できます。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.InputSystem;
using UnityEngine.InputSystem.Controls;
// カメラコントローラ
public class CameraController : MonoBehaviour
{
// マウスの開始位置とカメラの開始角度
private Vector2 mouseStartPos;
private Vector3 camStartRot;
// フレーム毎に呼ばれる
public void Update()
{
Transform camTrans = this.gameObject.transform;
// カメラの位置の操作 (WSADQE)
var keyboard = Keyboard.current;
if (keyboard != null) {
// カメラ位置の取得
Vector3 camPos = transform.position;
// キー押下中の処理
if (keyboard.wKey.isPressed) {camPos += camTrans.forward * Time.deltaTime * 5.0f;}
if (keyboard.sKey.isPressed) {camPos -= camTrans.forward * Time.deltaTime * 5.0f;}
if (keyboard.aKey.isPressed) {camPos -= camTrans.right * Time.deltaTime * 5.0f;}
if (keyboard.dKey.isPressed) {camPos += camTrans.right * Time.deltaTime * 5.0f;}
if (keyboard.qKey.isPressed) {camPos -= camTrans.up * Time.deltaTime * 5.0f;}
if (keyboard.eKey.isPressed) {camPos += camTrans.up * Time.deltaTime * 5.0f;}
// カメラ位置の更新
this.gameObject.transform.position = camPos;
}
// カメラの角度の操作 (マウス)
var mouse = Mouse.current;
if (mouse != null) {
// マウス位置の取得
Vector2 mousePos = mouse.position.ReadValue();
// マウス左ボタンの押下時の処理
if (mouse.leftButton.wasPressedThisFrame) {
// マウスの開始位置とカメラの開始角度の取得
this.mouseStartPos = mousePos;
this.camStartRot.x = camTrans.transform.eulerAngles.x;
this.camStartRot.y = camTrans.transform.eulerAngles.y;
}
// マウス左ボタンの押下中の処理
if (mouse.leftButton.isPressed) {
// カメラの角度の更新
float x = (this.mouseStartPos.x - mousePos.x) / Screen.width;
float y = (this.mouseStartPos.y - mousePos.y) / Screen.height;
camTrans.rotation = Quaternion.Euler(
this.camStartRot.x + y * 90f, this.camStartRot.y + x * 90f, 0);
}
}
}
}
この記事が気に入ったらサポートをしてみませんか?