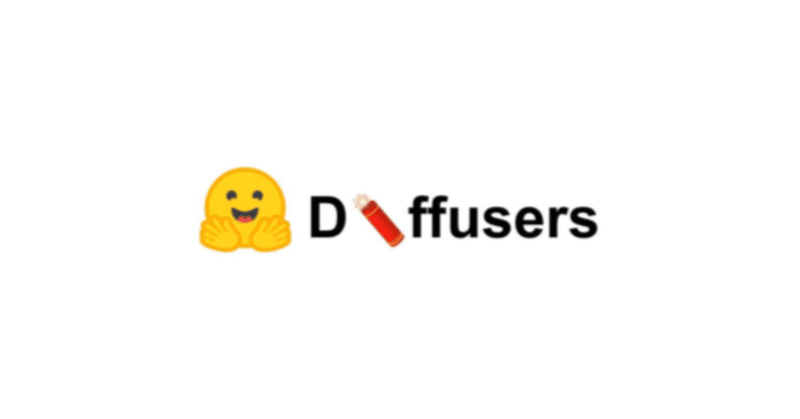
HuggingFace Diffusers v0.12.0の新機能
「Diffusers v0.12.0」の新機能についてまとめました。
前回
1. Diffusers v0.12.0 のリリースノート
情報元となる「Diffusers 0.12.0」のリリースノートは、以下で参照できます。
2. Instruct-Pix2Pix
「Instruct-Pix2Pix」は、人間の指示で画像編集するためにファインチューニングされた「Stable Diffusion」モデルです。 入力画像と、モデルに何をすべきかを与えられると、モデルがそれに従って画像編集します。
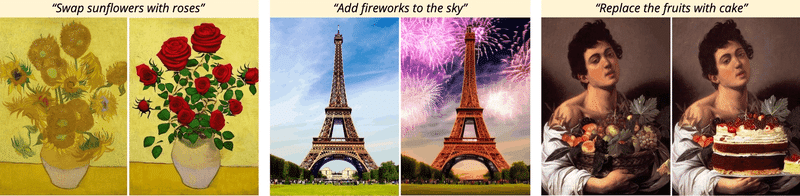
詳しくは、論文「InstructPix2Pix: Learning to Follow Image Editing Instructions」を参照。
!pip install diffusers transformers safetensors accelerate
import PIL
import requests
import torch
from diffusers import StableDiffusionInstructPix2PixPipeline
model_id = "timbrooks/instruct-pix2pix"
pipe = StableDiffusionInstructPix2PixPipeline.from_pretrained(model_id, torch_dtype=torch.float16).to("cuda")
url = "https://huggingface.co/datasets/diffusers/diffusers-images-docs/resolve/main/mountain.png"
def download_image(url):
image = PIL.Image.open(requests.get(url, stream=True).raw)
image = PIL.ImageOps.exif_transpose(image)
image = image.convert("RGB")
return image
image = download_image(url)
prompt = "make the mountains snowy"
edit = pipe(prompt, image=image, num_inference_steps=20, image_guidance_scale=1.5, guidance_scale=7).images[0]
images[0].save("snowy_mountains.png")
3. DiT
「DiTs」(Diffusion Transformers) は、一般的に使用される U-Net バックボーンを潜在パッチで動作するTransformerに置き換える、クラス条件付き潜在拡散モデルです。事前学習済みのモデルは ImageNet-1K データセットで学習されており、256x256 または 512x512 ピクセルのクラス条件付き画像を生成できます。
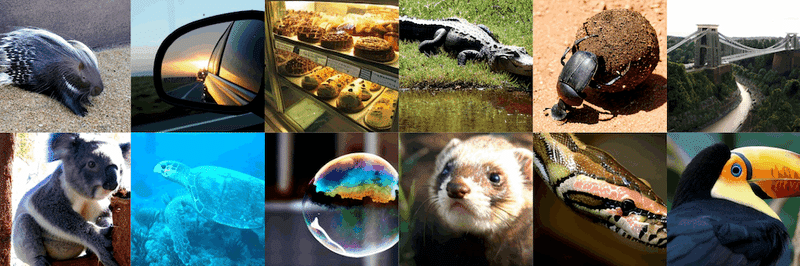
詳しくは、「Scalable Diffusion Models with Transformers」を参照。
import torch
from diffusers import DiTPipeline
model_id = "facebook/DiT-XL-2-256"
pipe = DiTPipeline.from_pretrained(model_id, torch_dtype=torch.float16).to("cuda")
# pick words that exist in ImageNet
words = ["white shark", "umbrella"]
class_ids = pipe.get_label_ids(words)
output = pipe(class_labels=class_ids)
image = output.images[0] # label 'white shark'
4. LoRA
「LoRA」は、大規模モデルに対してパラメータ効率の高いファインチューニングを実行するための手法です。「LoRA」は、いわゆる「更新行列」(update matrices) を事前学習済みモデルの特定のブロックに追加することで機能します。ファインチューニング中、これらの「更新行列」のみが更新され、事前学習済みのモデル パラメータは固定されたままになります。 これにより、ファインチューニング中の移植性が向上するだけでなく、メモリ効率が向上します。
「LoRA」は論文「LoRA: Low-Rank Adaptation of Large Language Models」で提案されました。元の論文では、GPT-3 のような大規模言語モデルのファインチューニングを調査しました。cloneofsimoは、人気のある lora GitHub リポジトリで「Stable Diffusion」のLoRA学習を最初に試しました。
from diffusers import StableDiffusionPipeline
import torch
model_path = "sayakpaul/sd-model-finetuned-lora-t4"
pipe = StableDiffusionPipeline.from_pretrained("CompVis/stable-diffusion-v1-4", torch_dtype=torch.float16)
pipe.unet.load_attn_procs(model_path)
pipe.to("cuda")
prompt = "A pokemon with blue eyes."
image = pipe(prompt, num_inference_steps=30, guidance_scale=7.5).images[0]
image.save("pokemon.png")

詳しくは、以下を参照。
5. Customizable Cross Attention
「Customizable Cross Attention」は、UNetの奥深くにあるクロスアテンションレイヤーをカスタマイズします。これは、Prompt-to-Promptなどの他のクリエイティブなアプローチに役立ち、xFormersなどのオプティマイザの適用が容易になります。
6. Flax => PyTorch
Flaxモデルの重みを PyTorch 用に変換する方法が作成されました。 これは、Google TPU を使用して超高速でモデルを学習またはファインチューニングし、重みをPyTorchに変換して誰もが使用できるようにすることを意味します。
7. Flax Img2Img
Image-to-ImageパイプラインがFlaxに移植されました。TPU v2-8 (Colab の無料利用枠で利用可能) を使用すると、数秒で一度に8つの画像を生成できます。
8. DEIS Scheduler
「DEIS」 (Diffusion Exponential Integrator Sampler) は、より少ないステップで高品質のサンプルを生成できる新しい高速マルチ ステップスケジューラです。
from diffusers import StableDiffusionPipeline, DEISMultistepScheduler
import torch
pipe = StableDiffusionPipeline.from_pretrained("runwayml/stable-diffusion-v1-5", torch_dtype=torch.float16)
pipe.scheduler = DEISMultistepScheduler.from_config(pipe.scheduler.config)
pipe = pipe.to("cuda")
prompt = "a photo of an astronaut riding a horse on mars"
generator = torch.Generator(device="cuda").manual_seed(0)
image = pipe(prompt, generator=generator, num_inference_steps=25).images[0
詳しくは、論文「Fast Sampling of Diffusion Models with Exponential Integrator」を参照。
9. Reproducibility
パイプラインがGPU上にある場合でも、CPU ジェネレータをすべてのパイプラインに渡すことができるようになりました。 これにより、
GPU ハードウェア全体での再現性が大幅に向上します。
import torch
from diffusers import DDIMPipeline
import numpy as np
model_id = "google/ddpm-cifar10-32"
# load model and scheduler
ddim = DDIMPipeline.from_pretrained(model_id)
ddim.to("cuda")
# create a generator for reproducibility
generator = torch.manual_seed(0)
# run pipeline for just two steps and return numpy tensor
image = ddim(num_inference_steps=2, output_type="np", generator=generator).images
print(np.abs(image).sum())
詳しくは、以下を参照。
10. ガイド
次回
この記事が気に入ったらサポートをしてみませんか?