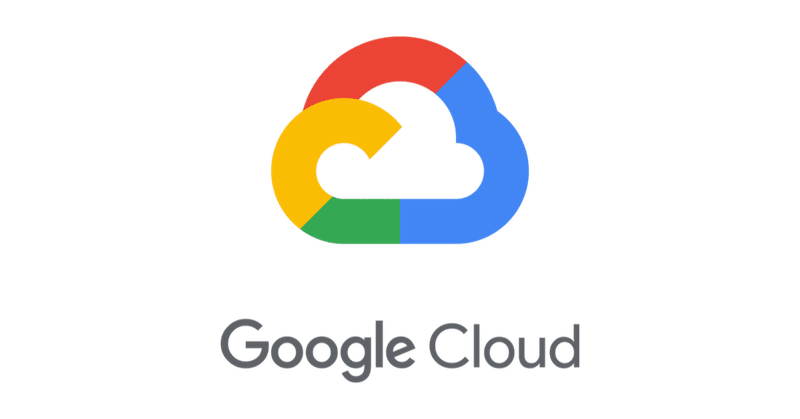
GCP 入門 (3) - Natural Language
「GCP」(Google Cloud Platform)の「Natural Language」で自然言語処理を行う方法をまとめました。
・macOS 10.15.7
・Python 3.6
前回
1. Natural Language
「Natural Language」は、自然言語テキストから分析情報を抽出するAPIです。
主なタスクは、次のとおりです。
・感情分析
自然言語テキストから全体的な意見、感想、態度の感情を読み取る。
・エンティティ分析
自然言語テキスト内のエンティティを識別し、ラベル付け(日付、人、連絡先情報、組織、場所、イベント、商品、メディアなど)を行う。
・エンティティ感情分析
自然言語テキスト内のエンティティに対する感情(ポジティブ、ネガティブ)を読み取る。
・構文解析
トークンと文の抽出、品詞の特定、各文の係り受け解析ツリーの作成。
・コンテンツの分類 【日本語未対応】
事前定義された700以上のカテゴリで自然言語テキストを分類。
料金は、次のとおりです。
2. プロジェクトの作成とAPIの有効化
(1) GCPの「Cloud Console」を開く。
(2) トップ画面上端の「プロジェクト名」から新規プロジェクトを作成。
(3) トップ画面上端の「検索ボックス」で「Natural Language」を検索し、「有効にする」ボタンを押す。
3. サービスアカウントキーの作成
(1) 「認証情報 → 認証情報を作成 → ウィザードで選択」を選択。
(2) 「1. 必要な認証情報の種類を調べる」で、以下を設定し、「必要な認証情報」ボタンを押す。
・使用するAPI : Cloud Natural Language API
・"App Engine または Compute Engine でこの API を使用する予定はありますか?" : いいえ
(3) 「2. 認証情報を取得する」で、以下を設定し、「次へ」ボタンを押す。
・サービスアカウント名 : test
・ロール : オーナー ※ どのリソースにアクセスできるかに影響
・キーのタイプ : JSON
成功すると、 「サービスアカウントキー」(JSONファイル)がダウンロードされます。
4. Pythonの開発環境の準備
(1) 「Python3.6」の仮想環境の作成。
(2) 「クライアントライブラリ」のインストール。
$ pip install --upgrade google-cloud-language
(3) サービスアカウントキーの登録。
$ export GOOGLE_APPLICATION_CREDENTIALS="[サービスアカウントキーのパス]"
5. 感情分析
「感情分析」を実行するコードは、次のとおりです。
from google.cloud import language_v1
# クライアントの生成
client = language_v1.LanguageServiceClient()
# ドキュメントの生成
text_content = "むかしむかし、あるところに、おじいさんとおばあさんが住んでいました。おじいさんは山へしばかりに、おばあさんは川へせんたくに行きました。おばあさんが川でせんたくをしていると、ドンブラコ、ドンブラコと、大きな桃が流れてきました。「おや、これは良いおみやげになるわ」おばあさんは大きな桃をひろいあげて、家に持ち帰りました。"
document = {
"content": text_content,
"type_": language_v1.Document.Type.PLAIN_TEXT,
"language": "ja"}
# 感情分析の実行
response = client.analyze_sentiment(request = {
'document': document,
'encoding_type': language_v1.EncodingType.UTF8})
# ドキュメント全体の感情分析結果
print(u"Document sentiment score: {}".format(response.document_sentiment.score))
print(u"Document sentiment magnitude: {}".format(response.document_sentiment.magnitude))
# 文ごとの感情分析結果
for sentence in response.sentences:
print(u"Sentence text: {}".format(sentence.text.content))
print(u"Sentence sentiment score: {}".format(sentence.sentiment.score))
print(u"Sentence sentiment magnitude: {}".format(sentence.sentiment.magnitude))
Document sentiment score: 0.10000000149011612
Document sentiment magnitude: 0.6000000238418579
Sentence text: むかしむかし、あるところに、おじいさんとおばあさんが住んでいました。
Sentence sentiment score: 0.0
Sentence sentiment magnitude: 0.0
Sentence text: おじいさんは山へしばかりに、おばあさんは川へせんたくに行きました。
Sentence sentiment score: 0.0
Sentence sentiment magnitude: 0.0
Sentence text: おばあさんが川でせんたくをしていると、ドンブラコ、ドンブラコと、大きな桃が流れてきました。「
Sentence sentiment score: 0.10000000149011612
Sentence sentiment magnitude: 0.10000000149011612
Sentence text: おや、これは良いおみやげになるわ」おばあさんは大きな桃をひろいあげて、家に持ち帰りました。
Sentence sentiment score: 0.5
Sentence sentiment magnitude: 0.5
感情分析結果は、score と magnitude で表現します。
・score : -1.0(ネガティブ)~1.0(ポジティブ)のスコアで感情を表す。「怒り」「悲しみ」もネガティブとみなされる。
・magnitude : 0.0~+inf の値で感情の強度(ポジティブとネガティブの両方)を表す。テキスト内で感情が表現されるたびに増減するため、長いテキストほど値が高くなる傾向がある。
6. エンティティ分析
「エンティティ分析」を実行するコードは、次のとおりです。
from google.cloud import language_v1
# クライアントの生成
client = language_v1.LanguageServiceClient()
# ドキュメントの生成
text_content = "むかしむかし、あるところに、おじいさんとおばあさんが住んでいました。おじいさんは山へしばかりに、おばあさんは川へせんたくに行きました。おばあさんが川でせんたくをしていると、ドンブラコ、ドンブラコと、大きな桃が流れてきました。「おや、これは良いおみやげになるわ」おばあさんは大きな桃をひろいあげて、家に持ち帰りました。"
document = {
"content": text_content,
"type_": language_v1.Document.Type.PLAIN_TEXT,
"language": "ja"}
# エンティティ分析の実行
response = client.analyze_entities(request = {
'document': document,
'encoding_type': language_v1.EncodingType.UTF8})
# エンティティループ
for entity in response.entities:
# エンティティ名
print(u"Representative name for the entity: {}".format(entity.name))
# エンティティ種別 (例:PERSON,LOCATION,ADDRESS,NUMBER)
print(u"Entity type: {}".format(language_v1.Entity.Type(entity.type_).name))
# テキスト全体の関連性スコア (0.0〜1.0)
print(u"Salience score: {}".format(entity.salience))
# エンティティのメタデータ
for metadata_name, metadata_value in entity.metadata.items():
print(u"{}: {}".format(metadata_name, metadata_value))
# メンション
for mention in entity.mentions:
print(u"Mention text: {}".format(mention.text.content))
# メンション種別(例:PROPER)
print(
u"Mention type: {}".format(language_v1.EntityMention.Type(mention.type_).name)
)
Representative name for the entity: おばあさん
Entity type: PERSON
Salience score: 0.34470394253730774
Mention text: おばあさん
Mention type: COMMON
Mention text: おばあさん
Mention type: COMMON
Mention text: おばあさん
Mention type: COMMON
Mention text: おばあさん
Mention type: COMMON
Representative name for the entity: むかしむかし
Entity type: CONSUMER_GOOD
Salience score: 0.13178835809230804
Mention text: むかしむかし
Mention type: COMMON
Representative name for the entity: じいさん
Entity type: PERSON
Salience score: 0.13178835809230804
Mention text: じいさん
Mention type: COMMON
Representative name for the entity: ところ
Entity type: OTHER
Salience score: 0.103628009557724
Mention text: ところ
Mention type: COMMON
Representative name for the entity: 川
Entity type: LOCATION
Salience score: 0.05932701751589775
Mention text: 川
Mention type: COMMON
Mention text: 川
Mention type: COMMON
Representative name for the entity: ドンブラコ
Entity type: OTHER
Salience score: 0.05709021911025047
Mention text: ドンブラコ
Mention type: PROPER
Mention text: ドンブラコ
Mention type: PROPER
Representative name for the entity: 桃
Entity type: OTHER
Salience score: 0.05427694320678711
Mention text: 桃
Mention type: COMMON
Mention text: 桃
Mention type: COMMON
Representative name for the entity: おみやげ
Entity type: OTHER
Salience score: 0.05043629929423332
Mention text: おみやげ
Mention type: COMMON
Representative name for the entity: 山
Entity type: LOCATION
Salience score: 0.04893800616264343
Mention text: 山
Mention type: COMMON
Representative name for the entity: 家
Entity type: LOCATION
Salience score: 0.01802285760641098
Mention text: 家
Mention type: COMMON
エンティティのパラメータは、次のとおりです。
・type : エンティティ種別。
・metadata : エンティティのナレッジ リポジトリの参照元情報。
・wikipedia_url : WikipediaのURL。
・mid : Google Knowledge Graphエントリに対応する MID(Machine-generated Identifier)。midは異なる言語間でも一意。
・salience : テキスト全体に対するこのエンティティの関連度(0.0〜1.0)。
・mentions : エンティティが記述されているテキスト内のオフセット位置。
エンティティ種別とメタデータは、次のとおりです。
・UNKNOWN : 不明
・PERSON : 人物
・LOCATION :場所
・ORGANIZATION : 組織
・EVENT : イベント
・WORK_OF_ART : アート
・CONSUMER_GOOD : 消費者製品
・OTHER : その他
・PHONE_NUMBER : 電話番号
・number : 番号
・national_prefix : 国コード
・area_code : リージョンまたはエリアのコード
・extension : 接続後にダイヤルされる内線番号
・ADDRESS : 住所
・street_number : 番地
・locality : 市区町村
・street_name : 通り名または路線名
・postal_code : 郵便番号
・country : 国
・broad_region : 都道府県などの行政区域
・narrow_region : 郡などの小規模な行政区域
・sublocality : アジア地域の住所で、市区町村内の地区
・DATE : 日付
・year : 4 桁の年(検出された場合)
・month : 月を表す 2 桁の数字(検出された場合)
・day : 日を表す 2 桁の数字(検出された場合)
・NUMBER : 番号
・メタデータ : 番号
・PRICE : 料金
・メタデータ : 料金
「Google Knowledge Graph」については、以下を参照してください。
7. エンティティ感情分析
「エンティティ感情分析」を実行するコードは、次のとおりです。
from google.cloud import language_v1
# クライアントの生成
client = language_v1.LanguageServiceClient()
# ドキュメントの生成
text_content = "むかしむかし、あるところに、おじいさんとおばあさんが住んでいました。おじいさんは山へしばかりに、おばあさんは川へせんたくに行きました。おばあさんが川でせんたくをしていると、ドンブラコ、ドンブラコと、大きな桃が流れてきました。「おや、これは良いおみやげになるわ」おばあさんは大きな桃をひろいあげて、家に持ち帰りました。"
document = {
"content": text_content,
"type_": language_v1.Document.Type.PLAIN_TEXT,
"language": "ja"}
# エンティティ感情分析
response = client.analyze_entity_sentiment(request = {
'document': document,
'encoding_type': language_v1.EncodingType.UTF8})
# エンティティループ
for entity in response.entities:
# エンティティ名
print(u"Representative name for the entity: {}".format(entity.name))
# エンティティ種別 (例: PERSON, LOCATION, ADDRESS, NUMBER)
print(u"Entity type: {}".format(language_v1.Entity.Type(entity.type_).name))
# テキスト全体の関連性スコア (0.0〜1.0)
print(u"Salience score: {}".format(entity.salience))
# エンティティ感情分析
sentiment = entity.sentiment
print(u"Entity sentiment score: {}".format(sentiment.score))
print(u"Entity sentiment magnitude: {}".format(sentiment.magnitude))
# エンティティのメタデータ
for metadata_name, metadata_value in entity.metadata.items():
print(u"{} = {}".format(metadata_name, metadata_value))
# メンション(テキスト内のこのエンティティへの言及)
for mention in entity.mentions:
print(u"Mention text: {}".format(mention.text.content))
# メンション種別(例:PROPER)
print(
u"Mention type: {}".format(language_v1.EntityMention.Type(mention.type_).name)
)
Representative name for the entity: おばあさん
Entity type: PERSON
Salience score: 0.34470394253730774
Entity sentiment score: 0.0
Entity sentiment magnitude: 0.10000000149011612
Mention text: おばあさん
Mention type: COMMON
Mention text: おばあさん
Mention type: COMMON
Mention text: おばあさん
Mention type: COMMON
Mention text: おばあさん
Mention type: COMMON
Representative name for the entity: むかしむかし
Entity type: CONSUMER_GOOD
Salience score: 0.13178835809230804
Entity sentiment score: 0.0
Entity sentiment magnitude: 0.0
Mention text: むかしむかし
Mention type: COMMON
Representative name for the entity: じいさん
Entity type: PERSON
Salience score: 0.13178835809230804
Entity sentiment score: 0.0
Entity sentiment magnitude: 0.0
Mention text: じいさん
Mention type: COMMON
Representative name for the entity: ところ
Entity type: OTHER
Salience score: 0.103628009557724
Entity sentiment score: 0.0
Entity sentiment magnitude: 0.0
Mention text: ところ
Mention type: COMMON
Representative name for the entity: 川
Entity type: LOCATION
Salience score: 0.05932701751589775
Entity sentiment score: 0.0
Entity sentiment magnitude: 0.0
Mention text: 川
Mention type: COMMON
Mention text: 川
Mention type: COMMON
Representative name for the entity: ドンブラコ
Entity type: OTHER
Salience score: 0.05709021911025047
Entity sentiment score: 0.0
Entity sentiment magnitude: 0.0
Mention text: ドンブラコ
Mention type: PROPER
Mention text: ドンブラコ
Mention type: PROPER
Representative name for the entity: 桃
Entity type: OTHER
Salience score: 0.05427694320678711
Entity sentiment score: 0.0
Entity sentiment magnitude: 0.10000000149011612
Mention text: 桃
Mention type: COMMON
Mention text: 桃
Mention type: COMMON
Representative name for the entity: おみやげ
Entity type: OTHER
Salience score: 0.05043629929423332
Entity sentiment score: 0.6000000238418579
Entity sentiment magnitude: 0.6000000238418579
Mention text: おみやげ
Mention type: COMMON
Representative name for the entity: 山
Entity type: LOCATION
Salience score: 0.04893800616264343
Entity sentiment score: 0.0
Entity sentiment magnitude: 0.0
Mention text: 山
Mention type: COMMON
Representative name for the entity: 家
Entity type: LOCATION
Salience score: 0.01802285760641098
Entity sentiment score: 0.0
Entity sentiment magnitude: 0.0
Mention text: 家
Mention type: COMMON
8. 構文分析
「構文分析」を実行するコードは、次のとおりです。
from google.cloud import language_v1
# クライアントの生成
client = language_v1.LanguageServiceClient()
# ドキュメントの生成
text_content = "むかしむかし、あるところに、おじいさんとおばあさんが住んでいました。"
document = {
"content": text_content,
"type_": language_v1.Document.Type.PLAIN_TEXT,
"language": "ja"}
# 構文解析の実行
response = client.analyze_syntax(request = {
'document': document,
'encoding_type': language_v1.EncodingType.UTF8})
# トークンループ
for token in response.tokens:
# トークンのコンテンツ(単語,句読点)
text = token.text
print(u"Token text: {}".format(text.content))
print(
u"Location of this token in overall document: {}".format(text.begin_offset)
)
# 品詞 (例:NOUN,ADJ)
part_of_speech = token.part_of_speech
print(
u"Part of Speech tag: {}".format(
language_v1.PartOfSpeech.Tag(part_of_speech.tag).name
)
)
# 声 (ACTIVE or PASSIVE)
print(u"Voice: {}".format(language_v1.PartOfSpeech.Voice(part_of_speech.voice).name))
# 時制 (例:PAST,FUTURE,PRESENT)
print(u"Tense: {}".format(language_v1.PartOfSpeech.Tense(part_of_speech.tense).name))
# レンマの
print(u"Lemma: {}".format(token.lemma))
# 依存関係ツリー
dependency_edge = token.dependency_edge
print(u"Head token index: {}".format(dependency_edge.head_token_index))
print(
u"Label: {}".format(language_v1.DependencyEdge.Label(dependency_edge.label).name)
)
Token text: むかし
Location of this token in overall document: 0
Part of Speech tag: NOUN
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: むかし
Head token index: 1
Label: NN
Token text: むかし
Location of this token in overall document: 9
Part of Speech tag: NOUN
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: むかし
Head token index: 3
Label: TMOD
Token text: 、
Location of this token in overall document: 18
Part of Speech tag: PUNCT
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: 、
Head token index: 3
Label: P
Token text: ある
Location of this token in overall document: 21
Part of Speech tag: VERB
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: ある
Head token index: 4
Label: RCMOD
Token text: ところ
Location of this token in overall document: 27
Part of Speech tag: NOUN
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: ところ
Head token index: 12
Label: ADVPHMOD
Token text: に
Location of this token in overall document: 36
Part of Speech tag: PRT
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: に
Head token index: 4
Label: PRT
Token text: 、
Location of this token in overall document: 39
Part of Speech tag: PUNCT
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: 、
Head token index: 12
Label: P
Token text: お
Location of this token in overall document: 42
Part of Speech tag: AFFIX
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: お
Head token index: 8
Label: PREF
Token text: じいさん
Location of this token in overall document: 45
Part of Speech tag: NOUN
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: じいさん
Head token index: 12
Label: NSUBJ
Token text: と
Location of this token in overall document: 57
Part of Speech tag: PRT
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: と
Head token index: 8
Label: CC
Token text: おばあさん
Location of this token in overall document: 60
Part of Speech tag: NOUN
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: おばあさん
Head token index: 8
Label: CONJ
Token text: が
Location of this token in overall document: 75
Part of Speech tag: PRT
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: が
Head token index: 8
Label: PRT
Token text: 住ん
Location of this token in overall document: 78
Part of Speech tag: VERB
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: 住ん
Head token index: 12
Label: ROOT
Token text: で
Location of this token in overall document: 84
Part of Speech tag: PRT
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: で
Head token index: 12
Label: PRT
Token text: い
Location of this token in overall document: 87
Part of Speech tag: VERB
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: い
Head token index: 12
Label: AUXVV
Token text: まし
Location of this token in overall document: 90
Part of Speech tag: VERB
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: まし
Head token index: 12
Label: AUX
Token text: た
Location of this token in overall document: 96
Part of Speech tag: VERB
Voice: VOICE_UNKNOWN
Tense: PAST
Lemma: た
Head token index: 12
Label: AUX
Token text: 。
Location of this token in overall document: 99
Part of Speech tag: PUNCT
Voice: VOICE_UNKNOWN
Tense: TENSE_UNKNOWN
Lemma: 。
Head token index: 12
Label: P
・text :TextSpan
テキスト。
・partOfSpeech : PartOfSpeech
品詞タグ。
・dependencyEdge : DependencyEdge
依存関係ツリー。
・lemma : string
レンマ。
品詞タグは、次のとおりです。
・UNKNOWN : 不明。
・ADJ : 形容詞。
・ADP : 後置詞(前置詞と後置詞)。
・ADV : 副詞。
・CONJ : 接続詞。
・DET : 限定詞。
・NOUN : 名詞(固有名詞)。
・NUM : 基数。
・PRON : 代名詞。
・PRT : 粒子または他の機能語。
・PUNCT : 句読点。
・VERB : 動詞(全ての時制とモード)。
・X : その他 (外国語、タイプミス、略語)。
・AFFIX : 接辞。
依存関係ツリーのラベルは、次のとおりです。
・UNKNOWN : わからない。
・ABBREV : 略語修飾子。
・ACOMP : 形容詞の補語。
・ADVCL : 副詞句修飾子。
・ADVMOD : 副詞修飾語。
・AMOD : NPの形容詞修飾子。
・APPOS : NPの同格修飾子。
・ATTR : コピュラ動詞に依存する属性。
・AUX : 助動詞(非主動詞)。
・AUXPASS : パッシブ補助。
・CC : 接続詞の調整。
・CCOMP : 動詞または形容詞の節の補語。
・CONJ : 結合。
・CSUBJ : 条項の主題。
・CSUBJPASS : 節の受動態。
・DEP : 依存関係(決定できない)。
・DET : 限定詞。
・DISCOURSE : 談話。
・DOBJ : 直接目的語。
・EXPL : 罵倒。
・GOESWITH : 一緒に行く(よく編集されていないテキスト内の単語の一部)。
・IOBJ : 間接目的語。
・MARK : マーカー(従属節を紹介する単語)。
・MWE : マルチワード表現。
・MWV : 複数単語の言語表現。
・NEG : 否定修飾子。
・NN : 名詞複合修飾子。
・NPADVMOD : 副詞修飾語として使用される名詞句。
・NSUBJ : 名目上の主題。
・NSUBJPASS : 受動的な名目上の主題。
・NUM : 名詞の数値修飾子。
・NUMBER : 化合物番号の要素。
・P : 句読点。
・PARATAXIS : パラタキシス関係。
・PARTMOD : 分詞修飾子。
・PCOMP : 前置詞の補集合は節。
・POBJ : 前置詞の目的語。
・POSS : 所持修飾子。
・POSTNEG : ポストバーバルネガティブパーティクル。
・PRECOMP : 述語補語。
・PRECONJ : プレコンジャント。
・PREDET : 事前決定者。
・PREF : プレフィックス。
・PREP : 前置詞修飾子。
・PRONL : 動詞と形態素の関係。
・PRT : 粒子。
・PS : 連想または所有格マーカー。
・QUANTMOD : 数量詞句修飾子。
・RCMOD : 関係節修飾子。
・RCMODREL : 関係節の補文標識。
・RDROP : 先行する述語のない省略記号。
・REF : 指示対象。
・REMNANT : レムナント。
・REPARANDUM : 報酬。
・ROOT : ルート。
・SNUM : 数値の単位を指定するサフィックス。
・SUFF : サフィックス。
・TMOD : 時間修飾子。
・TOPIC : トピックマーカー。
・VMOD : 名詞を修飾する動詞の無限形が先頭にある節。
・VOCATIVE : 呼応。
・XCOMP : オープン節の補語。
・SUFFIX : 名前の接尾辞。
・TITLE : 名前のタイトル。
・ADVPHMOD : 副詞句修飾子。
・AUXCAUS : 使役助動詞。
・AUXVV : ヘルパー補助。
・DTMOD : 蓮太石(プレノミナル修飾子)。
・FOREIGN : 外国語。
・KW : キーワード。
・LIST : 同等のアイテムのチェーンのリスト。
・NOMC : 名詞化された句。
・NOMCSUBJ : 名詞化された節の主語。
・NOMCSUBJPASS : 名詞化された節の受動態。
・NUMC : 数値修飾子の複合。
・COP : コピュラ。
・DISLOCATED : ずれた関係(前面/話題化された要素の場合)。
・ASP : アスペクトマーカー。
・GMOD : 属格修飾子。
・GOBJ : 属格。
・INFMOD : 不定詞修飾子。
・MES : 測定。
・NCOMP : 名詞の名目上の補数。
9. コンテンツの分類 【日本語未対応】
「コンテンツの分類」を実行するコードは、次のとおりです。
from google.cloud import language_v1
# クライアントの生成
client = language_v1.LanguageServiceClient()
# ドキュメントの生成
text_content = "That actor on TV makes movies in Hollywood and also stars in a variety of popular new TV shows."
document = {
"content": text_content,
"type_": language_v1.Document.Type.PLAIN_TEXT,
"language": "en"}
# コンテンツの分類の実行
response = client.classify_text(request = {'document': document})
# カテゴリループ
for category in response.categories:
# カテゴリ名
print(u"Category name: {}".format(category.name))
# 信頼度
print(u"Confidence: {}".format(category.confidence))
Category name: /Arts & Entertainment/TV & Video/TV Shows & Programs
Confidence: 0.5199999809265137
カテゴリ名は、以下で確認できます。
次回
この記事が気に入ったらサポートをしてみませんか?