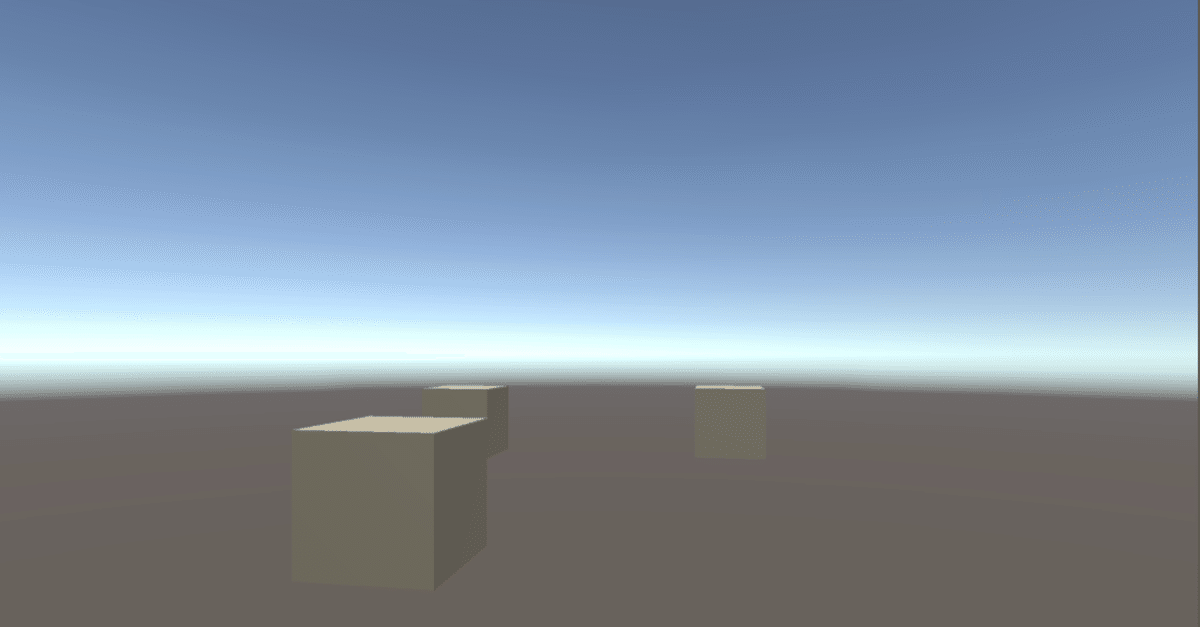
Unity DOTS 入門 (9) - キー操作とタグ
Unity DOTS で「キー操作」を行う方法と、エンティティに「タグ」を使って任意のエンティティのみ操作する方法を説明します。
・Unity 2019.3.14.f1
・Entities 0.11.1
1. キー操作
「Unity DOTS 入門 (1)」のサンプルに「キー操作」で立方体を移動させる機能を追加します。
(1) Projectウィンドウにスクリプト「PlayerInputSystem」を追加。
using UnityEngine;
using Unity.Entities;
using Unity.Jobs;
using Unity.Mathematics;
using Unity.Transforms;
using System;
public class PlayerInputSystem : SystemBase
{
protected override void OnUpdate()
{
float deltaTime = Time.DeltaTime;
float dy = Input.GetAxis("Vertical");
float dx = Input.GetAxis("Horizontal");
float speed = 10f;
Entities.ForEach((ref Translation translation) =>
{
translation.Value.x += dx * deltaTime * speed;
translation.Value.z += dy * deltaTime * speed;
}).Run();
}
}
◎ Entities.Run()
Entities.Schedule()でなく、Entities.Run() を使うことで、ジョブの処理をメインスレッドで実行できます。キー操作はメインスレッドで実行する必要があります。
(2) 実行。
方向キーで立方体を操作できます。
2. タグの利用
Entities.ForEach((ref Translation translation) では、Translationコンポーネントを持つ全てのエンティティを動かしてしまいます。
「Cube」を複数コピーして、位置を若干ずらして実行すると、複数の「Cube」が同時に動きます。
そこで、「タグ」を利用して、任意のエンティティのみ操作対象とします。エンティティの「タグ」は、データを持たないコンポーネントで表現します。
(1) 「Cube」の1つにスクリプト「PlayerTag」を追加。
using Unity.Entities;
[GenerateAuthoringComponent]
public struct PlayerTag : IComponentData
{
}
(2) スクリプト「PlayerInputSystem」の Entities.ForEach() を以下のように変更。
Entities.
WithAll<PlayerTag>().
ForEach((ref Translation translation) =>
{
translation.Value.x += dx * deltaTime * speed;
translation.Value.z += dy * deltaTime * speed;
}).Run();
Entities.ForEach() には以下でフィルタを指定できます。
・WithAll<コンポーネント名>() : 指定コンポーネントの全てを含む
・WithAny<コンポーネント名>() : 指定コンポーネントのいずれかを含む
・WithNone<コンポーネント名>() : 指定コンポーネントを含まない
これで、先程追加した「タグ」を指定することで、任意のエンティティのみを操作できます。
(3) 実行。
「タグ」を指定したCubeのみ操作できることを確認します。
この記事が気に入ったらサポートをしてみませんか?