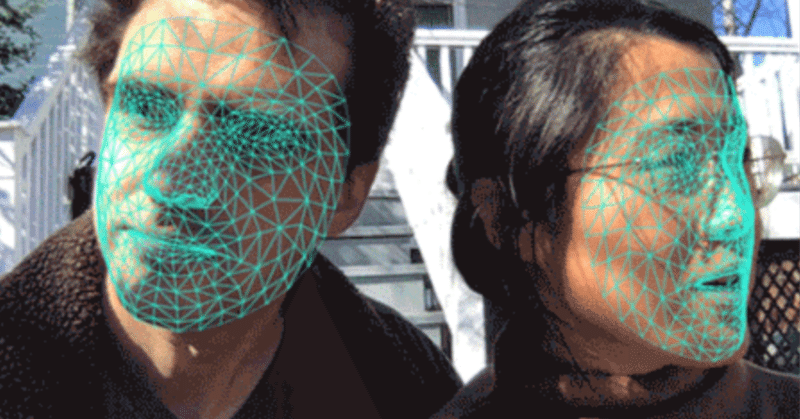
MediaPipe Facemesh
以下の記事を参考に書いてます。
1. はじめに
MediaPipe Facemesh は、顔の表面形状を486個のランドマークの3次元座標で予測する、軽量モデルです。モデルの特性については、モデルカードを参照してください。
このモデルは、携帯の 前面カメラ向け に設計されており、顔が画面の大部分を占有することが期待されます。そのため、遠くにある顔の特定は苦手です。Webカメラに映った顔のランドマークを検出するデモを参照してください。また、MediaPipe の一部としても利用できます。
2. インストール
◎ yarn
$ yarn add @tensorflow-models/facemesh
◎ npm
$ npm install @tensorflow-models/facemesh
このパッケージは、依存関係として @tensorflow/tfjs-core および @tensorflow/tfjs-converter が指定されています。
3. 使用方法
◎ npm
const facemesh = require('@tensorflow-models/facemesh');
◎ CDN
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs"></script>
<script src="https://cdn.jsdelivr.net/npm/@tensorflow-models/facemesh"></script>
◎ コード例
async function main() {
// facemeshモデルの読み込み
const model = await facemesh.load();
// ビデオストリーム (または画像、キャンバス、3Dテンソル)を渡し、検出結果を取得
const predictions = await model.estimateFaces(document.querySelector("video"));
if (predictions.length > 0) {
/*
predictionsは、検出された各顔を示すオブジェクトの配列 :
[
{
faceInViewConfidence: 1, // 顔が存在する確率
boundingBox: { // 顔を囲むバウンディングボックス
topLeft: [232.28, 145.26],
bottomRight: [449.75, 308.36],
},
mesh: [ // 顔のランドマークの3次元座標
[92.07, 119.49, -17.54],
[91.97, 102.52, -30.54],
...
],
scaledMesh: [ // 正規化された顔のランドマークの3次元座標
[322.32, 297.58, -17.54],
[322.18, 263.95, -30.54]
],
annotations: { // セマンティックグループ
silhouette: [
[326.19, 124.72, -3.82],
[351.06, 126.30, -3.00],
...
],
...
}
}
]
*/
for (let i = 0; i < predictions.length; i++) {
const keypoints = predictions[i].scaledMesh;
// 顔のキーポイントのログ出力
for (let i = 0; i < keypoints.length; i++) {
const [x, y, z] = keypoints[i];
console.log(`Keypoint ${i}: [${x}, ${y}, ${z}]`);
}
}
}
}
main();
4. facemesh.load() のパラメータ
・maxContinuousChecks : 何フレーム毎にBoundingBoxを検出するか (デフォルト:5)
・detectionConfidence : 予測破棄のしきい値 (デフォルト:0.9)
・maxFaces : 検出する顔の最大数 (デフォルト:10)
・iouThreshold : BoundingBoxの重なり具合のしきい値 (デフォルト:0.3)
・scoreThreshold : BoundingBoxのスコアに基づいた削除のしきい値 (デフォルト:0.75)
5. model.estimateFace() のパラメータ
・input : 分類する画像 (テンソル、DOM要素、ビデオ、キャンバス)
・returnTensors : 値ではなくテンソルを返すか (デフォルト:false)
・flipHorizontal : キーポイントを水平反転するか(デフォルト:false)
6. キーポイント
キーポイントは次のとおり。
これらのキーポイントのUV座標は、facemeshモデルの getUVCoords() を介して利用できます。これらは src/uv_coords.ts にもあります。
この記事が気に入ったらサポートをしてみませんか?