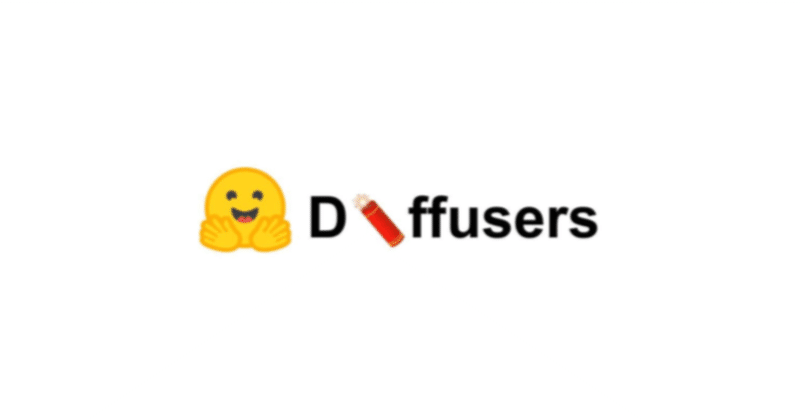
HuggingFace Diffusers v0.13.0の新機能
「Diffusers v0.13.0」の新機能についてまとめました。
前回
1. Diffusers v0.13.0 のリリースノート
情報元となる「Diffusers 0.13.0」のリリースノートは、以下で参照できます。
2. 拡散モデルの画像生成の制御
現在、拡散モデルの画像生成の制御に関する研究が数多く行われています。
・Instruct Pix2Pix
・Pix2Pix 0 (docs)
・Attend and excite (docs)
・Semantic guidance (docs)
・Self-attention guidance (docs)
・Depth2image
・MultiDiffusion panorama (docs)
詳しくは「拡散モデルの画像生成の制御」を参照してください。
3. Latent Upscaler
「Latent Upscaler」は、「Stable Diffusion」用に設計された拡散モデルです。「Stable Diffusion」で生成されたLatentをアップスケーラに渡し、標準的なVAEでデコードすることができます。
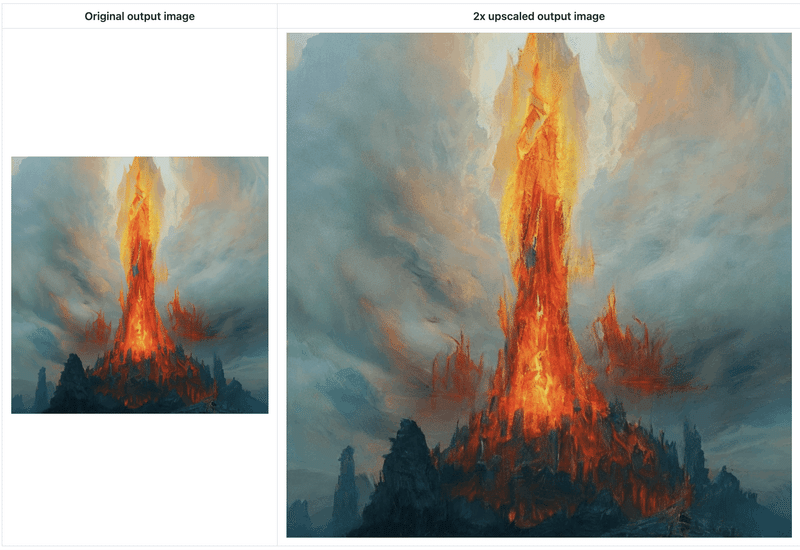
from diffusers import StableDiffusionLatentUpscalePipeline, StableDiffusionPipeline
import torch
# パイプラインの準備
pipeline = StableDiffusionPipeline.from_pretrained(
"CompVis/stable-diffusion-v1-4",
torch_dtype=torch.float16
)
pipeline.to("cuda")
# アップスケーラーの準備
upscaler = StableDiffusionLatentUpscalePipeline.from_pretrained(
"stabilityai/sd-x2-latent-upscaler",
torch_dtype=torch.float16
)
upscaler.to("cuda")
# プロンプトとジェネレータの準備
prompt = "a photo of an astronaut high resolution, unreal engine, ultra realistic"
generator = torch.manual_seed(33)
# パイプラインの実行
low_res_latents = pipeline(
prompt,
generator=generator,
output_type="latent"
).images
# アップスケーラーの実行
upscaled_image = upscaler(
prompt=prompt,
image=low_res_latents,
num_inference_steps=20,
guidance_scale=0,
generator=generator,
).images[0]
# 画像の保存
upscaled_image.save("astronaut_1024.png")
# 比較用に低解像度の画像の保存
with torch.no_grad():
image = pipeline.decode_latents(low_res_latents)
image = pipeline.numpy_to_pil(image)[0]
image.save("astronaut_512.png")
4. 最適化
4-1. xFormers
メモリが大幅に節約され、推論も高速化される「xFormers」のインストールが簡単になりました。
(1) xformersパッケージのインストール。
!pip install xformers
(2) パイプラインでのxformersの有効化。
pipe.enable_xformers_memory_efficient_attention()
詳しくは、ドキュメントを参照してください。
4-2. Torch 2.0
「Accelerated PyTorch 2.0 Transformers」がビルトインのネイティブサポートしました。PyTorch 2.0リリース後は、「xFormers」やサードパーティパッケージをインストールする必要がなくなります。テストでは、PyTorch 2.0の実装は「xFormers」と同じくらい高速で、時にはそれ以上になることもあります。詳しくはドキュメントを参照してください。
4-3. Coarse-grained CPU offload
enable_sequential_cpu_offload() で「sequential cpu offloading」を有効にすると、多くのメモリを節約しますが、推論がかなり遅くなります。
今回の提供された enable_model_cpu_offload() を利用すると、enable_sequential_cpu_offload() よりメモリ節約量は少ないですが、
オフロードなしの場合とほぼ同じ速さで推論できます。
5. Pix2Pix Zero
「Pix2Pix Zero」を使用すると、ユーザーは特定の画像 (実物または生成物) を編集し、ソースコンセプト (馬など) をターゲットコンセプト (シマウマなど) に置き換えることができます。
(1) 入力プロンプトから生成された画像を編集
(2) 入力画像を提供して編集
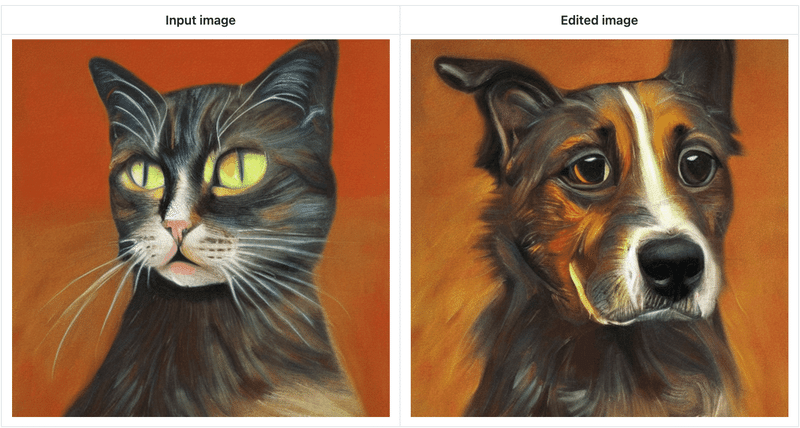
詳しくは、ドキュメントを参照してください。
6. Attend and excite
「Attend-and-Excite」は、生成モデルをガイドして、画像合成プロセス中に相互注意値を変更し、入力テキストプロンプトをより忠実に描写する画像を生成します。入力テキストプロンプトに関して、より意味的に忠実な画像を作成できます。
7. Semantic guidance
「SEGA」は、画像生成に対する強力なセマンティック制御を提供します。通常、テキストプロンプトを少し変更すると、まったく異なりる出力画像がになります。しかし、「SEGA」では、オリジナルのイメージ構成に忠実でありながら、簡単かつ直感的に制御できるさまざまなイメージの変更が可能です。
import torch
from diffusers import SemanticStableDiffusionPipeline
pipe = SemanticStableDiffusionPipeline.from_pretrained(
"runwayml/stable-diffusion-v1-5",
torch_dtype=torch.float16
)
pipe = pipe.to("cuda")
out = pipe(
prompt="a photo of the face of a woman",
num_images_per_prompt=1,
guidance_scale=7,
editing_prompt=[
"smiling, smile", # 適用する概念
"glasses, wearing glasses",
"curls, wavy hair, curly hair",
"beard, full beard, mustache",
],
reverse_editing_direction=[False, False, False, False], # ガイダンスの方向性
edit_warmup_steps=[10, 10, 10, 10], # 各コンセプトの準備期間
edit_guidance_scale=[4, 5, 5, 5.4], # 各コンセプトのガイダンススケール
edit_threshold=[
0.99,
0.975,
0.925,
0.96,
], # 各概念のしきい値
edit_momentum_scale=0.3, # 潜在ガイダンスに追加されるモメンタムスケール
edit_mom_beta=0.6, # モメンタムベータ
edit_weights=[1, 1, 1, 1, 1], # 個々の概念の相互に対する重み
8. Self-attention guidance
「SAG」は、反復ごとに拡散モデルから中間アテンションマップを抽出することで機能し、特定のアテンションスコアを超えるトークンを選択してマスキングとぼかしを行い、部分的にぼかした入力を取得します。 次に、ぼやけた入力と元の入力を拡散モデルに供給することで得られた予測ノイズ出力間の差異が測定され、これがガイダンスとしてさらに活用されます。このガイダンスにより、著者は幅広い拡散モデルで明らかな改善を観察しています。
import torch
from diffusers import StableDiffusionSAGPipeline
from accelerate.utils import set_seed
pipe = StableDiffusionSAGPipeline.from_pretrained(
"CompVis/stable-diffusion-v1-4",
torch_dtype=torch.float16
)
pipe = pipe.to("cuda")
seed = 8978
prompt = "."
guidance_scale = 7.5
num_images_per_prompt = 1
sag_scale = 1.0
set_seed(seed)
images = pipe(
prompt,
num_images_per_prompt=num_images_per_prompt,
guidance_scale=guidance_scale,
sag_scale=sag_scale
).images
images[0].save("example.png")
9. MultiDiffusion panorama
「MultiDiffusion」は新しい生成プロセスです。複数の拡散生成プロセスを共有のパラメーターまたは制約のセットと結び付ける最適化タスクに基づいています。
import torch
from diffusers import StableDiffusionPanoramaPipeline, DDIMScheduler
model_ckpt = "stabilityai/stable-diffusion-2-base"
scheduler = DDIMScheduler.from_pretrained(model_ckpt, subfolder="scheduler")
pipe = StableDiffusionPanoramaPipeline.from_pretrained(
model_ckpt,
scheduler=scheduler,
torch_dtype=torch.float16
)
pipe = pipe.to("cuda")
prompt = "a photo of the dolomites"
image = pipe(prompt).images[0]
image.save("dolomites.png")
10. 倫理指針
diffusersはジェネレーティブテクノロジーがもたらす課題についての様々な意見や見解を知らないわけではありません。diffusersの「倫理指針」を作成し、コミュニティとの実りある会話を始めたいと考えています。
11. UniPCスケジューラ
「UniPC」は新しい高速スケジューラです。拡散モデルの高速サンプリング用に設計された学習不要のフレームワークになります。
12. 学習のEMAサポート
学習のEMAサポートを改善しました。すべてのスクリプトで使用できる、diffusers.training_utilsに共通のEMAModelを追加しました。EMAModel は、分散学習をサポートするように改善されました。
学習中に EMAモデルを簡単に評価する新しい方法と、diffusersの他のモデルと同様にEMAモデルを保存およびロードする一貫した方法を提供します。
13. ruff & black
「flake8」を「ruff」に置き換え (高速)、「black」のバージョンを更新しました。
次回
この記事が気に入ったらサポートをしてみませんか?