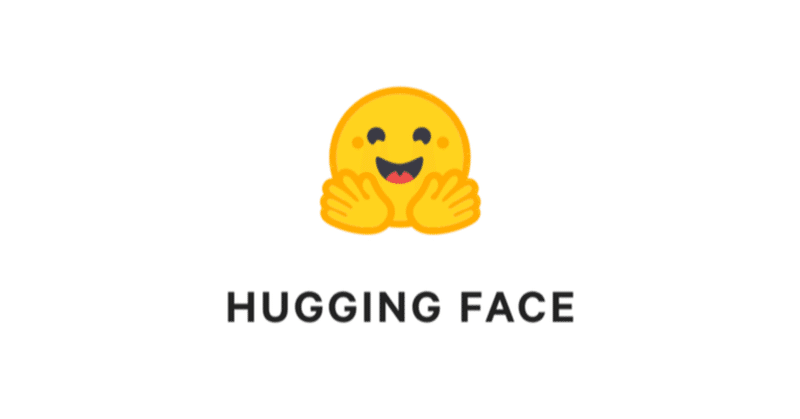
Huggingface Transformers 入門 (23) - 英語の言語モデルの学習
「Huggingface Transformers」による英語の言語モデルの学習手順をまとめました。
・Huggingface Transformers 4.4.2
・Huggingface Datasets 1.2.1
前回
1. 英語のマスク言語モデルの学習
「WikiText」を使って英語のマスク言語モデル(MLM: Masked Language Model)を学習します。
(1) データの永続化
# データの永続化
from google.colab import drive
drive.mount('/content/drive')
!mkdir -p '/content/drive/My Drive/work/'
%cd '/content/drive/My Drive/work/'
(2) ソースからの「Huggingface Transformers」のインストール
# ソースからのHuggingface Transformersのインストール
!git clone https://github.com/huggingface/transformers -b v4.4.2
!pip install -e transformers
(3) 「Huggingface Datasets」のインストール
# Huggingface Datasetsのインストール
!pip install datasets==1.2.1
(4) メニュー「ランタイム → ランタイムを再起動」で「Google Colab」を再起動し、作業フォルダに戻る
# メニュー「ランタイム → ランタイムを再起動」で「Google Colab」を再起動
# 作業フォルダに戻る
%cd '/content/drive/My Drive/work/'
(5) 事前学習の実行
%%time
# 事前学習の実行
!python ./transformers/examples/language-modeling/run_mlm.py \
--model_name_or_path=roberta-base \
--dataset_name=wikitext \
--dataset_config_name=wikitext-2-raw-v1 \
--do_train \
--do_eval \
--output_dir=output/
***** eval metrics *****
epoch = 3.0
eval_loss = 1.2824
eval_mem_cpu_alloc_delta = 0MB
eval_mem_cpu_peaked_delta = 0MB
eval_mem_gpu_alloc_delta = 0MB
eval_mem_gpu_peaked_delta = 1584MB
eval_runtime = 6.8891
eval_samples = 496
eval_samples_per_second = 71.998
perplexity = 3.6052
CPU times: user 7.37 s, sys: 873 ms, total: 8.24 s
Wall time: 14min 41s
2. 英語のマスク言語モデルの推論
import torch
from transformers import AutoTokenizer, AutoModelForMaskedLM
# トークナイザーとモデルの準備
tokenizer = AutoTokenizer.from_pretrained('roberta-base')
model = AutoModelForMaskedLM.from_pretrained('output/')
# テキスト
text = f'I am a {tokenizer.mask_token}. there is no name yet.'
# テキストをテンソルに変換
input_ids = tokenizer.encode(text, return_tensors='pt')
masked_index = torch.where(input_ids == tokenizer.mask_token_id)[1].tolist()[0]
# 推論
result = model(input_ids)
pred_ids = result[0][:, masked_index].topk(5).indices.tolist()[0]
for pred_id in pred_ids:
output_ids = input_ids.tolist()[0]
output_ids[masked_index] = pred_id
print(tokenizer.decode(output_ids))
<s>I am a girl. there is no name yet.</s>
<s>I am a student. there is no name yet.</s>
<s>I am a woman. there is no name yet.</s>
<s>I am a female. there is no name yet.</s>
<s>I am a writer. there is no name yet.</s>
3. 英語の因果言語モデルの学習
「WikiText」を使って英語の因果言語モデル(CLM: Causal Language Model)を学習します。
(1) データの永続化
# データの永続化
from google.colab import drive
drive.mount('/content/drive')
!mkdir -p '/content/drive/My Drive/work/'
%cd '/content/drive/My Drive/work/'
(2) ソースからの「Huggingface Transformers」のインストール
# ソースからのHuggingface Transformersのインストール
!git clone https://github.com/huggingface/transformers -b v4.4.2
!pip install -e transformers
(3) 「Huggingface Datasets」のインストール
# Huggingface Datasetsのインストール
!pip install datasets==1.2.1
(4) メニュー「ランタイム → ランタイムを再起動」で「Google Colab」を再起動し、作業フォルダに戻る
# メニュー「ランタイム → ランタイムを再起動」で「Google Colab」を再起動
# 作業フォルダに戻る
%cd '/content/drive/My Drive/work/'
(5) 事前学習の実行
%%time
# 事前学習の実行
!python ./transformers/examples/language-modeling/run_clm.py \
--model_name_or_path=gpt2 \
--dataset_name=wikitext \
--dataset_config_name=wikitext-2-raw-v1 \
--do_train \
--do_eval \
--per_device_train_batch_size=2 \
--per_device_eval_batch_size=2 \
--output_dir=output/
***** eval metrics *****
epoch = 3.0
eval_loss = 3.041
eval_mem_cpu_alloc_delta = 0MB
eval_mem_cpu_peaked_delta = 0MB
eval_mem_gpu_alloc_delta = 0MB
eval_mem_gpu_peaked_delta = 1399MB
eval_runtime = 16.3944
eval_samples = 240
eval_samples_per_second = 14.639
perplexity = 20.9267
CPU times: user 17.1 s, sys: 3.11 s, total: 20.2 s
Wall time: 26min 41s
2. 英語の因果言語モデルの推論
import transformers
# トークナイザーとモデルの準備
tokenizer = transformers.AutoTokenizer.from_pretrained("gpt2")
model = transformers.AutoModelForCausalLM.from_pretrained("output/")
# テキスト
text = 'I am a cat.'
# 推論
input = tokenizer.encode(text, return_tensors='pt')
output = model.generate(input, do_sample=True, top_p=0.95, top_k=50, num_return_sequences=3)
print(tokenizer.batch_decode(output))
['I am a cat. I have a cat. I have a dog. I have a cat.',
'I am a cat. I have been looking for someone with the right temperament. " \n =',
'I am a cat. The name " Petey " is meant to imply that Petey is a']
この記事が気に入ったらサポートをしてみませんか?