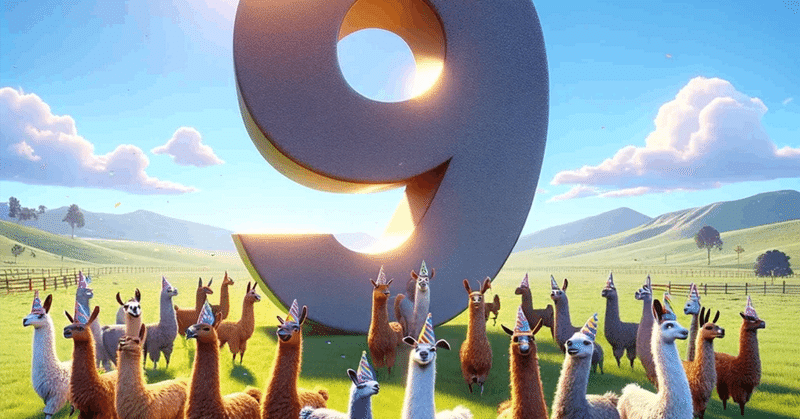
LlamaIndex v0.9 の概要
以下の記事が面白かったので、かるくまとめました。
1. LlamaIndex v0.9 の概要
「LlamaIndex v0.9」の新機能と変更点は、次のとおりです。
・IngestionPipline
・Transformation Caching
・ノード解析・テキスト分割・メタデータ抽出のインターフェース変更
・グローバルトークナイザー
・パッケージのスリム化 (LangChainとの分離)
・インポートパスの変更
・【Beta版】 マルチモーダルRAG
2. IngestionPipeline
「IngestionPipeline」は、純粋にデータを取り込むための新しい抽象化です。
データの取り込み時に適用される変換には、次のようなものがあります。
・Text Splitter
・Node Parser
・Metadata Extractor
・Embeddings Model
「IngestionPipeline」を使うことで、どのような変換を行うかを設定できます。
使用例は、次のとおりです。
from llama_index import Document
from llama_index.embeddings import OpenAIEmbedding
from llama_index.text_splitter import SentenceSplitter
from llama_index.extractors import TitleExtractor
from llama_index.ingestion import IngestionPipeline, IngestionCache
pipeline = IngestionPipeline(
transformations=[
SentenceSplitter(chunk_size=25, chunk_overlap=0),
TitleExtractor(),
OpenAIEmbedding(),
]
)
nodes = pipeline.run(documents=[Document.example()])
3. Transformation Caching
同じ「IngestionPipeline」を実行するたびに、パイプライン内の各変換について、入力ノード+変換のハッシュとその変換の出力をキャッシュします。
ローカルキャッシュの保存とロードの例は、次のとおりです。
from llama_index import Document
from llama_index.embeddings import OpenAIEmbedding
from llama_index.text_splitter import SentenceSplitter
from llama_index.extractors import TitleExtractor
from llama_index.ingestion import IngestionPipeline, IngestionCache
pipeline = IngestionPipeline(
transformations=[
SentenceSplitter(chunk_size=25, chunk_overlap=0),
TitleExtractor(),
OpenAIEmbedding(),
]
)
# パイプライン全体を一度だけ実行
nodes = pipeline.run(documents=[Document.example()])
nodes = pipeline.run(documents=[Document.example()])
# 保存とロード
pipeline.cache.persist("./test_cache.json")
new_cache = IngestionCache.from_persist_path("./test_cache.json")
new_pipeline = IngestionPipeline(
transformations=[
SentenceSplitter(chunk_size=25, chunk_overlap=0),
TitleExtractor(),
],
cache=new_cache,
)
# キャッシュにより即座に実行
nodes = pipeline.run(documents=[Document.example()])
次に、Redisをキャッシュとして使用し、Qdrantをベクトル ストアとして使用する別の例を示します。これを実行すると、ノードがベクトルストアに直接挿入され、Redisの各変換ステップがキャッシュされます。
import re
from llama_index import Document
from llama_index.embeddings import OpenAIEmbedding
from llama_index.text_splitter import SentenceSplitter
from llama_index.ingestion import IngestionPipeline
from llama_index.schema import TransformComponent
class TextCleaner(TransformComponent):
def __call__(self, nodes, **kwargs):
for node in nodes:
node.text = re.sub(r'[^0-9A-Za-z ]', "", node.text)
return nodes
pipeline = IngestionPipeline(
transformations=[
SentenceSplitter(chunk_size=25, chunk_overlap=0),
TextCleaner(),
OpenAIEmbedding(),
],
)
nodes = pipeline.run(documents=[Document.example()])
4. ノード解析・テキスト分割・メタデータ抽出のインターフェース変更
ノード解析・テキスト分割・メタデータ抽出のインターフェースをシンプルにフラット化させました。
・変更前
from llama_index.node_parser import SimpleNodeParser
from llama_index.node_parser.extractors import (
MetadataExtractor, TitleExtractor
)
from llama_index.text_splitter import SentenceSplitter
node_parser = SimpleNodeParser(
text_splitter=SentenceSplitter(chunk_size=512),
metadata_extractor=MetadataExtractor(
extractors=[TitleExtractor()]
),
)
nodes = node_parser.get_nodes_from_documents(documents)
・変更後
from llama_index.text_splitter import SentenceSplitter
from llama_index.extractors import TitleExtractor
node_parser = SentenceSplitter(chunk_size=512)
extractor = TitleExtractor()
# 変換を直接使用
nodes = node_parser(documents)
nodes = extractor(nodes)
5. グローバルトークナイザー
「LlamaIndex V0.9」では、グローバルトークナイザーの設定が可能になりました。「GPT-3.5」に一致する「CL100K」がデフォルトになります。
トークナイザーの設定例は、次のとおりです。
from llama_index import set_global_tokenizer
# tiktoken
import tiktoken
set_global_tokenizer(
tiktoken.encoding_for_model("gpt-3.5-turbo").encode
)
# huggingface
from transformers import AutoTokenizer
set_global_tokenizer(
AutoTokenizer.from_pretrained("HuggingFaceH4/zephyr-7b-beta").encode
)
6. パッケージのスリム化 (LangChainとの分離)
「LangChain」がオプションのパッケージになり、デフォルトではインストールされなくなりました。
「LangChain」を「llama-index」の一部としてインストールする例は、次のとおりです。
# langchainのインストール
pip install llama-index[langchain]
# ローカルモデルを実行するために必要なツールをインストール
pip install llama-index[local_models]
# postgresに必要なツールをインストール
pip install llama-index[postgres]
# 組み合わせ
pip isntall llama-index[local_models,postgres]
7. インポートパスの変更
インポートパスに、次の2つの変更を加えました。
(1) llama_index のインポートを高速化するために、ルートレベルからあまり使用されないインポートを削除
(2) 「user-facing」の概念をレベル1のモジュールでインポート可能にするための一貫したポリシーの採用
from llama_index.llms import OpenAI, ...
from llama_index.embeddings import OpenAIEmbedding, ...
from llama_index.prompts import PromptTemplate, ...
from llama_index.readers import SimpleDirectoryReader, ...
from llama_index.text_splitter import SentenceSplitter, ...
from llama_index.extractors import TitleExtractor, ...
from llama_index.vector_stores import SimpleVectorStore, ...
最もよく使用されるモジュールは依然としてルートレベルで公開されています。
from llama_index import SimpleDirectoryReader, VectorStoreIndex, ...
8. 【Beta版】 マルチモーダルRAG
「マルチモーダルRAG」のユースケースのサポートに役立つ多数の新モジュールの導入を開始しました。
・マルチモーダルLLM (GPT-4V / Llava / Fuyuなど)
・マルチモーダルEmbedding
・マルチモーダルRAG
この記事が気に入ったらサポートをしてみませんか?