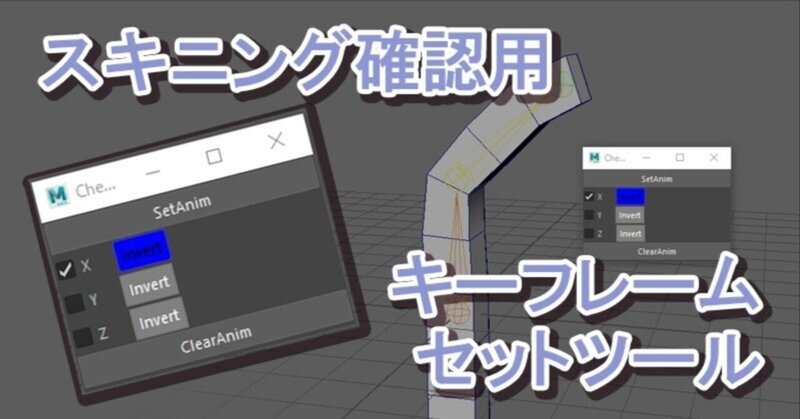
【MAYA】ワンクリックで360度回転するキーをセットする”CheckJointRotate”
先日のジョイント回転ツールがやっぱり良くなかったので、ベットでスヤァってしてるときに思いついたので作ってみました。
タイムスライダに選択ジョイントのRotateの値をキーに設定するツールです。
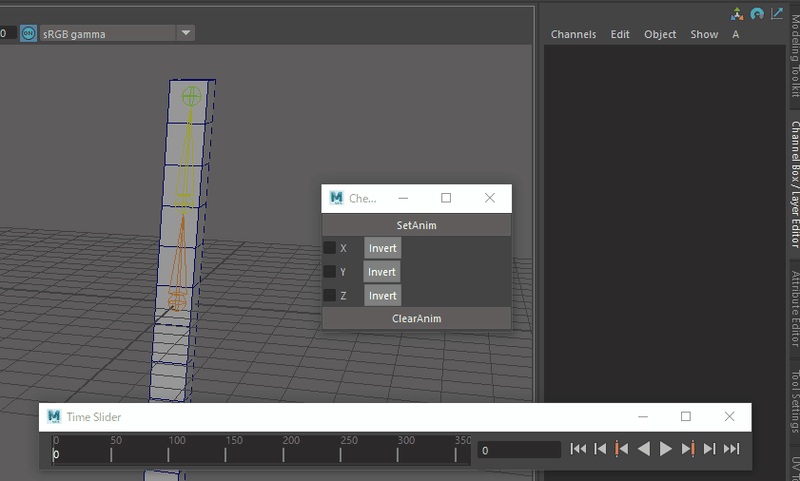
任意のジョイントを選択し、どの軸のキーをセットするかチェックボックスを入れてSetAnimを押すと
・0フレーム目に180度
・180フレーム目に0度
・360フレーム目に-180度
を入れてくれます。
Invertボタンを有効にすると360フレーム目が180度になり回転方向が逆になります。
フレームを削除するときはジョイント選択をしてClearAnimボタンを押すとキーを削除してくれます。
MAYA2024で動作確認しています。
MAYA2019などPython2の場合は使えません。GPTに書き換えるようお願いすると良いです。
import pymel.core as pm
class SetAnimUI(object):
def __init__(self):
# ウィンドウとレイアウトの設定
self.window = pm.window(title="SetAnimUI", widthHeight=(300, 200))
self.mainLayout = pm.columnLayout(parent=self.window, adjustableColumn=True)
# invertステートの初期化
self.invert = {"X": False, "Y": False, "Z": False}
self.create_ui()
def create_ui(self):
# Applyボタンの生成
self.apply_button = pm.button(label="SetAnim", parent=self.mainLayout, command=self.apply_anim)
# 各軸についてのチェックボックスとinvertボタンの生成
self.checkboxes = {}
self.invert_buttons = {}
for attr in ["X", "Y", "Z"]:
rowLayout = pm.rowLayout(parent=self.mainLayout, numberOfColumns=3)
self.checkboxes[attr] = pm.checkBox(label=attr, parent=rowLayout)
pm.separator(style='none', width=10, parent=rowLayout)
self.invert_buttons[attr] = pm.button(label="Invert", parent=rowLayout, c=pm.Callback(self.invert_toggle, attr), bgc=(0.5,0.5,0.5))
# ZeroDegreeボタンの生成
self.zeroDegree_button = pm.button(label="0°", parent=self.mainLayout, command=self.set_zero_degree)
# ClearAnimボタンの生成
self.clearAnim_button = pm.button(label="ClearAnim", parent=self.mainLayout, command=self.clear_anim)
def invert_toggle(self, attr):
# invertステートの切り替えとボタンの色の変更
self.invert[attr] = not self.invert[attr]
color = (0, 0, 1) if self.invert[attr] else (0.5, 0.5, 0.5)
self.invert_buttons[attr].setBackgroundColor(color)
def apply_anim(self, *args):
# 選択したジョイントの取得
selected = pm.ls(selection=True)
if not selected:
pm.warning("ジョイントが選択されていません。")
return
for joint in selected:
# チェックボックスに基づいてキーフレームを設定
for axis, checkbox in self.checkboxes.items():
endValue = -180 if self.invert[axis] else 180
if checkbox.getValue():
pm.setKeyframe(joint, attribute='rotate'+axis, time=0, value=180)
pm.setKeyframe(joint, attribute='rotate'+axis, time=180, value=0)
pm.setKeyframe(joint, attribute='rotate'+axis, time=360, value=endValue)
else:
# キーフレームを削除
pm.cutKey(joint, time=(0, 360), attribute='rotate'+axis, option="keys")
def clear_anim(self, *args):
# 選択したジョイントの取得
selected = pm.ls(selection=True)
if not selected:
pm.warning("ジョイントが選択されていません。")
return
for joint in selected:
# すべてのキーフレームを削除
pm.cutKey(joint, time=(0, 360), attribute='rotateX', option="keys")
pm.cutKey(joint, time=(0, 360), attribute='rotateY', option="keys")
pm.cutKey(joint, time=(0, 360), attribute='rotateZ', option="keys")
def set_zero_degree(self, *args):
# タイムラインのカレントフレームを180に設定
pm.currentTime(180)
SetAnimUI().window.show()
本ツールはChatGPTを使って作成しています。
AI生成のリスクを理解した上でご利用ください。
更新:
2024/01/01
MAYA2024用に書き換え、フレームに設定する回転値を変更
#MAYA2019 Python2版
import pymel.core as pm
class SetAnimUI(object):
def __init__(self):
# ウィンドウとレイアウトの設定
self.window = pm.window(title="SetAnimUI", widthHeight=(300, 200))
self.mainLayout = pm.columnLayout(parent=self.window, adjustableColumn=True)
# invertステートの初期化
self.invert = {"X": False, "Y": False, "Z": False}
self.create_ui()
def create_ui(self):
# Applyボタンの生成
self.apply_button = pm.button(label="SetAnim", parent=self.mainLayout, command=self.apply_anim)
# 各軸についてのチェックボックスとinvertボタンの生成
self.checkboxes = {}
self.invert_buttons = {}
for attr in ["X", "Y", "Z"]:
rowLayout = pm.rowLayout(parent=self.mainLayout, numberOfColumns=3)
self.checkboxes[attr] = pm.checkBox(label=attr, parent=rowLayout)
pm.separator(style='none', width=10, parent=rowLayout) # Add some space
self.invert_buttons[attr] = pm.button(label="Invert", parent=rowLayout, c=pm.Callback(self.invert_toggle, attr), bgc=(0.5,0.5,0.5))
# ClearAnimボタンの生成
self.clearAnim_button = pm.button(label="ClearAnim", parent=self.mainLayout, command=self.clear_anim)
def invert_toggle(self, attr):
# invertステートの切り替えとボタンの色の変更
self.invert[attr] = not self.invert[attr]
color = (0, 0, 1) if self.invert[attr] else (0.5, 0.5, 0.5)
self.invert_buttons[attr].setBackgroundColor(color)
def apply_anim(self, *args):
# 選択したジョイントの取得
selected = pm.ls(selection=True)
if not selected:
pm.warning("ジョイントが選択されていません。")
return
for joint in selected:
# チェックボックスに基づいてキーフレームを設定
for axis, checkbox in self.checkboxes.items():
endValue = -360 if self.invert[axis] else 360
if checkbox.getValue():
pm.setKeyframe(joint, attribute='rotate'+axis, time=0, value=0)
pm.setKeyframe(joint, attribute='rotate'+axis, time=360, value=endValue)
else:
# キーフレームを削除
pm.cutKey(joint, time=(0, 360), attribute='rotate'+axis, option="keys")
def clear_anim(self, *args):
# 選択したジョイントの取得
selected = pm.ls(selection=True)
if not selected:
pm.warning("ジョイントが選択されていません。")
return
for joint in selected:
# すべてのキーフレームを削除
pm.cutKey(joint, time=(0, 360), attribute='rotateX', option="keys")
pm.cutKey(joint, time=(0, 360), attribute='rotateY', option="keys")
pm.cutKey(joint, time=(0, 360), attribute='rotateZ', option="keys")
SetAnimUI().window.show()
この記事が気に入ったらサポートをしてみませんか?