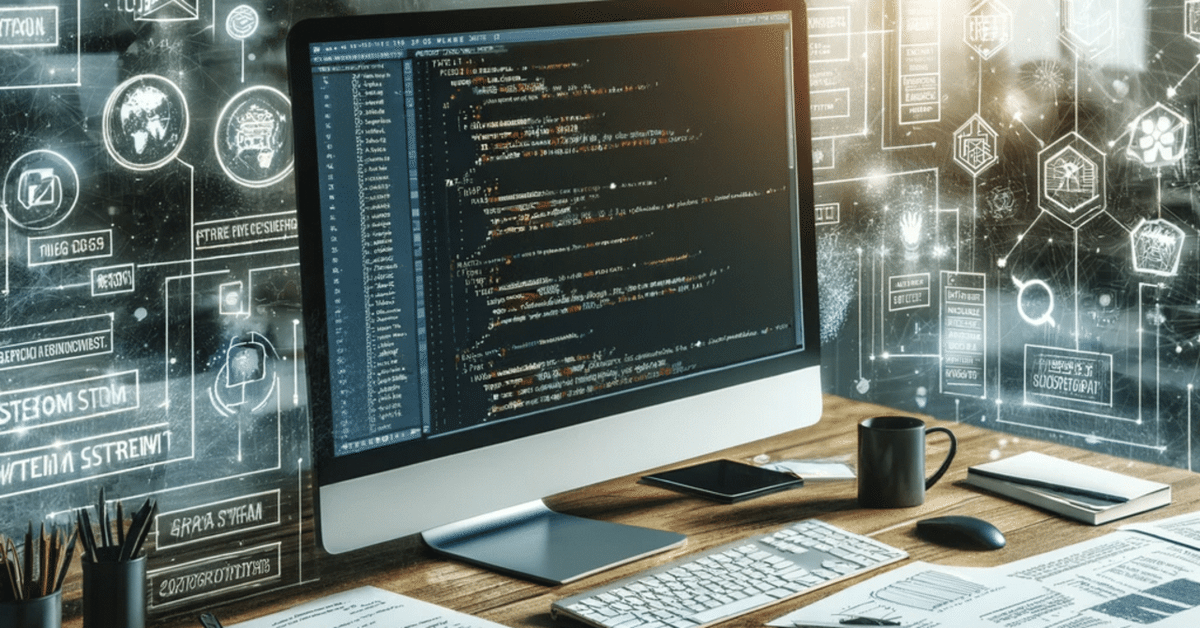
Leetcode を英語で説明する②(Java)
お題
https://leetcode.com/problems/palindrome-number/
説明順序
define the problem
identify parameters and return type
identify unique attributes
explain constraints
explain algorithms
explain the code you wrote
answer time complexity
1. define the problem ( name of problem)
The given problem is to find out if the number is a Palindrome or not
2. identify parameters and return type
There are one given parameter, x which is integer.
Return type is boolean that state whether the number is palindrome or not. If the number is Palindrome then return true, otherwise return false.
3. identify unique attributes
None
4. explain constraints
x can minimum -2 to the power of 31 and max 2 to the power of 31 - 1.
5. explain algorithms (how to solve the solution)
6. explain the code you wrote
class Solution {
public boolean isPalindrome(int x) {
String temp = Integer.toString(x);
int k = temp.length() - 1;
for (int i = 0; i < temp.length()/2; i++){
if(temp.charAt(k-i) - '0' != temp.charAt(i) - '0')
return false;
}
return true;
}
}
We convert large integer into an array so we can grab specific values at specific indexes. Once we have the new array, we can iterate through the array only first half of the values of the array.
For each iteration, we can check i, which is an index in the first half and compare it to the value at index k which starts at the end of the array and decrement.
If at any point, the value at k and the value at i are not the same we can conclude the integer is not palindrome, otherwise integer is palindrome.
7. answer time complexity
The time complexity of this algorithm is big O of n + n/2.
If considering infinity, it is big O of n.
この記事が気に入ったらサポートをしてみませんか?