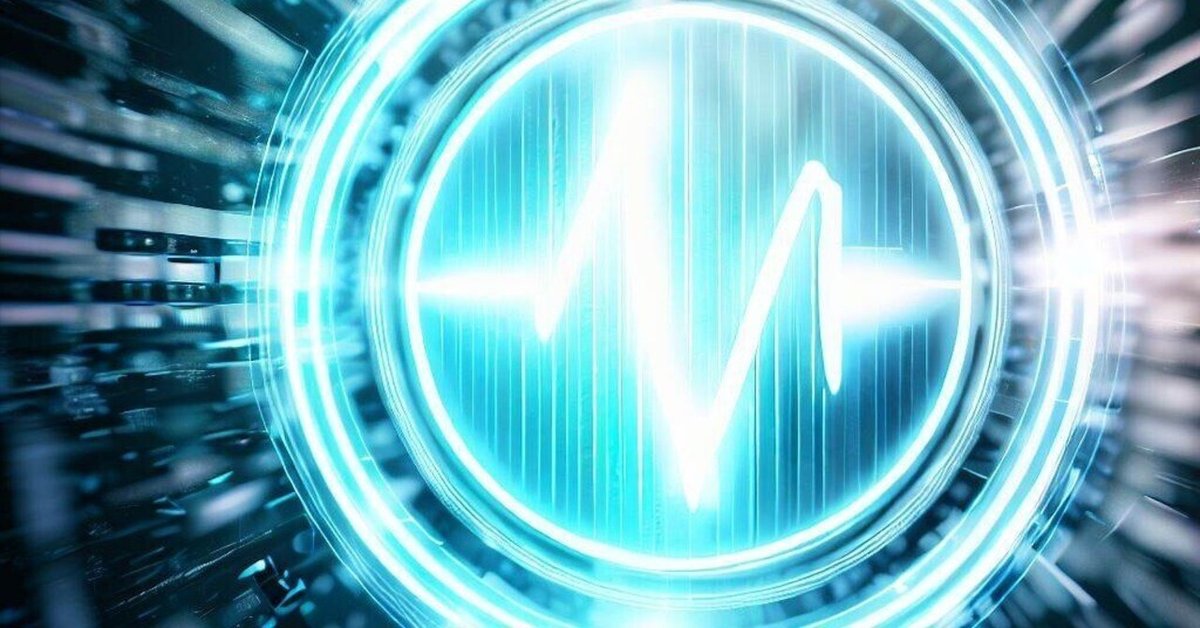
serp-ai/bark-with-voice-cloneで自分の音声を作成してみよう!
以前に、Barkという音声合成モデルを紹介しました。それから2,3日しか経過していませんが、7秒以上の音声から学習をして、音声生成してくれるモデルが登場しましたので紹介していきます。
今回は、下記を参考にしております。
私の理解が追い付かない部分もありますが、少し修正をしております。おそらくこのやり方で良いのだろうとは思っていますが、修正箇所が間違っていましたらすみません。
今回利用したコードは以下です。今回はGoogle Colabを想定しております。
!pip install git+https://github.com/suno-ai/bark.git
from bark.generation import load_codec_model, generate_text_semantic
from encodec.utils import convert_audio
import torchaudio
import torch
model = load_codec_model(use_gpu=True)
次に/contentにaudio.wavという自分の音声ファイルを配置します。
# Load and pre-process the audio waveform
audio_filepath = '/content/audio.wav' # the audio you want to clone (will get truncated so 5-10 seconds is probably fine, existing samples that I checked are around 7 seconds)
device = 'cuda' # or 'cpu'
wav, sr = torchaudio.load(audio_filepath)
wav = convert_audio(wav, sr, model.sample_rate, model.channels)
wav = wav.unsqueeze(0).to(device)
# Extract discrete codes from EnCodec
with torch.no_grad():
encoded_frames = model.encode(wav)
codes = torch.cat([encoded[0] for encoded in encoded_frames], dim=-1).squeeze() # [n_q, T]
text = "Transcription of the audio you are cloning"
# get seconds of audio
seconds = wav.shape[-1] / model.sample_rate
# generate semantic tokens
semantic_tokens = generate_text_semantic(text, max_gen_duration_s=seconds, top_k=50, top_p=.95, temp=0.7)
# move codes to cpu
codes = codes.cpu().numpy()
ここが少しトリッキーなのですが、Google Colab上で、/usr/local/lib/python3.9/dist-packages/bark/assets/promptsにあるja_speaker_0を削除します。
次に、voice_nameは、ja_speaker_0にします。output_pathも/usr/local/lib/python3.9/dist-packages/bark/assets/prompts/にします。そして、実行します。
学習する音声が制限されているようですので、既に学習済みの音声名ja_speker_0を利用するのがここでの目的です。
import numpy as np
voice_name = 'ja_speaker_0' # whatever you want the name of the voice to be
output_path = '/usr/local/lib/python3.9/dist-packages/bark/assets/prompts/' + voice_name + '.npz'
np.savez(output_path, fine_prompt=codes, coarse_prompt=codes[:2, :], semantic_prompt=semantic_tokens)
text_promptには、音声にしたいテキストを記載してください。そして、voice_nameにはja_spekaer_0にします。
from bark.api import generate_audio
from transformers import BertTokenizer
from bark.generation import SAMPLE_RATE, preload_models, codec_decode, generate_coarse, generate_fine, generate_text_semantic
# Enter your prompt and speaker here
text_prompt = "It is very cool. I am happy today. Because I want to talk to you!"
voice_name = "ja_speaker_0" # use your custom voice name here if you have one
# load the tokenizer
tokenizer = BertTokenizer.from_pretrained("bert-base-multilingual-cased")
# download and load all models
preload_models(
text_use_gpu=True,
text_use_small=False,
coarse_use_gpu=True,
coarse_use_small=False,
fine_use_gpu=True,
fine_use_small=False,
codec_use_gpu=True,
force_reload=False
)
# simple generation
audio_array = generate_audio(text_prompt, history_prompt="ja_speaker_0", text_temp=0.7, waveform_temp=0.7)
# generation with more control
x_semantic = generate_text_semantic(
text_prompt,
history_prompt=voice_name,
temp=0.7,
top_k=50,
top_p=0.95,
)
x_coarse_gen = generate_coarse(
x_semantic,
history_prompt=voice_name,
temp=0.7,
top_k=50,
top_p=0.95,
)
x_fine_gen = generate_fine(
x_coarse_gen,
history_prompt=voice_name,
temp=0.5,
)
audio_array = codec_decode(x_fine_gen)
from IPython.display import Audio
# play audio
Audio(audio_array, rate=SAMPLE_RATE)
と完了となります。
この記事が気に入ったらサポートをしてみませんか?