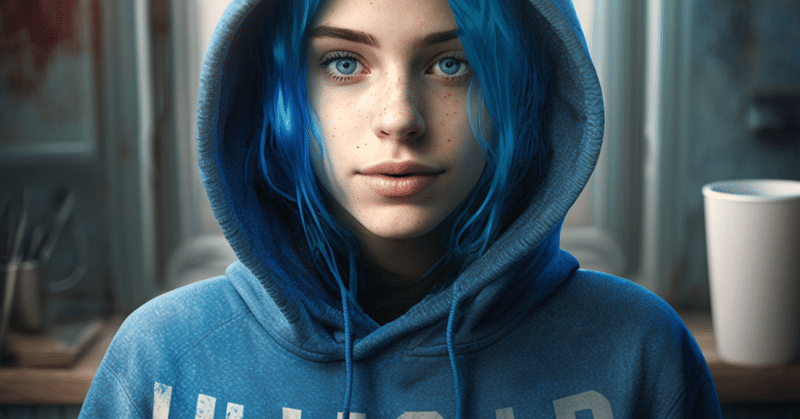
【コピペで使える】GPT4と協力して1時間でウェブスクレイピング作成したまとめ
流れと結論だけ
最新AIニュースを収集するプログラム
— Kaito │ AI活用のプロ (@ishizuka_kaito) April 25, 2023
GPT-4と協力したら1時間足らずで作成できた
コード書くのが好きじゃない私でもこういうものをサクッと作れる時代に感謝です。。。#GPT4 pic.twitter.com/Y09Q8dvMda
流れ
①世界中のAI toolの情報を集めたい。アイデアをください。と GPTに投げる
②いくつかアイデアが貰えたが、無料でできるものをピックアップしてもらう
③再度貰ったアイデアの中からWebスクレイピングを選択。ステップバイステップで進めていくことを伝える
④指示に従う。わからない部分はわからないと言う。
⑤作成されたコードを実行する。エラーが起きたらエラー文を丸投げし、修正されたものを再度実行する。この繰り返し。
収集結果
E列では、ついでにDeepLのAPIを使って、D列の本文冒頭の文章を翻訳しております
最終的なpythonコード
import requests
import os
import google.auth
from google.oauth2 import service_account
from google_auth_oauthlib.flow import InstalledAppFlow
from google.auth.transport.requests import Request
from googleapiclient.discovery import build
from googleapiclient.errors import HttpError
api_key = "your_api_key_here" # NewsAPIのAPIキー
url = "https://newsapi.org/v2/everything" # NewsAPIのエンドポイント
# 検索クエリとパラメータを設定
query = "検索したいテーマ"
params = {
"q": query,
"apiKey": api_key,
"language": "en", # 言語を日本語に設定
"sortBy": "publishedAt", # 公開日時でソート
"pageSize": 100, # 取得する記事数
}
# APIリクエストを送信し、レスポンスを取得
response = requests.get(url, params=params)
# レスポンスから記事データを抽出
articles = response.json()["articles"]
# 記事データを表示
for article in articles:
print(f"Title: {article['title']}")
print(f"URL: {article['url']}")
print(f"Published at: {article['publishedAt']}")
print("\n")
#動作確認
response = requests.get(url, params=params)
print(f"HTTP Status Code: {response.status_code}")
data = response.json()
print(data)
#データの整形
import json
# ステップ1: 記事データの整形
formatted_articles = [
{
"title": article["title"],
"url": article["url"],
"published_at": article["publishedAt"],
"content": article["content"],
}
for article in data["articles"]
]
# ステップ2: データをJSON形式でファイルに保存
with open("ai_tool_news.json", "w", encoding="utf-8") as f:
json.dump(formatted_articles, f, ensure_ascii=False, indent=2)
# スプレッドシート ID とシート名を設定(あなたのスプレッドシートに応じて変更してください)
SPREADSHEET_ID = 'your_spreadsheet_id'
SHEET_NAME = 'your_sheet_name'
# API クレデンシャルを設定
creds = None
if os.path.exists('credentials.json'):
creds = service_account.Credentials.from_service_account_file('credentials.json', scopes=['https://www.googleapis.com/auth/spreadsheets'])
service = build('sheets', 'v4', credentials=creds)
def add_data_to_spreadsheet(data):
try:
values = [list(article.values()) for article in data]
body = {"values": values}
range_name = f'{SHEET_NAME}!A2:D'
result = (
service.spreadsheets()
.values()
.append(
spreadsheetId=SPREADSHEET_ID,
range=range_name,
valueInputOption="RAW",
insertDataOption="INSERT_ROWS",
body=body,
)
.execute()
)
print(f"{result.get('updates').get('updatedCells')} cells updated.")
except HttpError as error:
print(f"An error occurred: {error}")
result = None
return result
# スプレッドシートにデータを追加
add_data_to_spreadsheet(formatted_articles)
簡単にまとめ
上記のコードをコピペして、下記を自分用にカスタマイズ
※注意点として、検索ワードの設定次第で取得するニュースが無い場合がある。英語で、連語の場合は半角スペースで区切って指定した方が良い。
api_key = "your_api_key_here" # NewsAPIのAPIキー url =
# 検索クエリとパラメータを設定
query = "検索したいテーマ"
# スプレッドシート ID とシート名を設定(あなたのスプレッドシートに応じて変更してください)
SPREADSHEET_ID = 'your_spreadsheet_id'
SHEET_NAME = 'your_sheet_name'
用意するもの
実行環境:VS codeで編集し、ターミナルにて実行
A:News APIに登録(無料)し、APIキーを取得する
※他人に知られないように注意
B:python のインストール(https://www.python.org/downloads/)
この記事が気に入ったらサポートをしてみませんか?