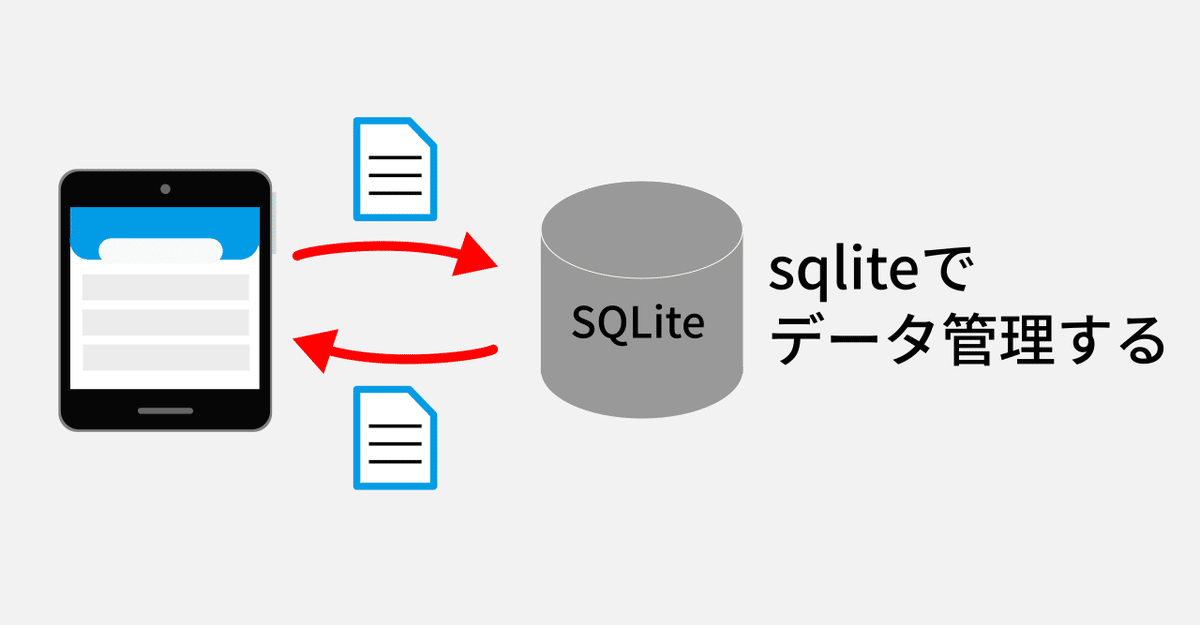
【Flutter】シンプルメモ帳作ろう4~sqliteでデータ管理する
Flutterでアプリケーションの中で取り扱うデータの管理にはsqliteを使用します。
初期設定(テーブル作成)からデータ追加、更新、削除のメソッドを定義し、
アプリの操作に応じて呼び出すようにします。
メモ帳アプリでは作ったメモの保存や削除に使用します。
■初期設定(テーブル作成)~ソースコード(抜粋)
void createAritcle() async {
debugPrint("createAritcle start");
final database = openDatabase(
join(await getDatabasesPath(), 'article_database.db'),
onCreate: (db, version) {
return db.execute(
"CREATE TABLE articles(id INTEGER PRIMARY KEY, title TEXT, body TEXT, updateDate TEXT)",
);
},
version: 1,
);
debugPrint("createAritcle end");
}
■データの追加・更新~ソースコード(抜粋)
Future<void> insertArticle(Article article) async {
debugPrint("insertArticle start");
final database = openDatabase(
join(await getDatabasesPath(), 'article_database.db'),
version: 1,
);
// Get a reference to the database.
final Database db = await database;
await db.insert(
'articles',
article.toMap(),
conflictAlgorithm: ConflictAlgorithm.replace,
);
debugPrint("insertArticle end");
}
■データの削除~ソースコード(抜粋)
Future<void> deleteArticle(int id) async {
final database = openDatabase(
join(await getDatabasesPath(), 'article_database.db'),
version: 1,
);
// Get a reference to the database.
final db = await database;
// Remove the Dog from the database.
await db.delete(
'articles',
// Use a `where` clause to delete a specific dog.
where: "id = ?",
// Pass the Dog's id as a whereArg to prevent SQL injection.
whereArgs: [id],
);
}
■データの参照~ソースコード(抜粋)
Future<List<Article>> getArticles(String searchStr) async {
final database = openDatabase(
join(await getDatabasesPath(), 'article_database.db'),
version: 1,
);
// Get a reference to the database.
final Database db = await database;
List<Map<String, dynamic>> maps;
// Query the table for all The Dogs.
if(searchStr != null && searchStr.length > 0){
maps = await db.query('articles',where: 'title like ? or body like ?',whereArgs: ["%$searchStr%","%$searchStr%"],orderBy: "updateDate DESC");
}else{
maps = await db.query('articles',orderBy: "updateDate DESC");
}
// Convert the List<Map<String, dynamic> into a List<Dog>.
return List.generate(maps.length, (i) {
return Article(
id: maps[i]['id'],
title: maps[i]['title'],
body: maps[i]['body'],
updateDate: maps[i]['updateDate'],
);
});
}
■ソースコード(全体)
↓にシンプルメモ帳アプリの全ソースコードをアップしています。
https://github.com/YasuoFromDeadScream/flutter_simple_memo
この記事が気に入ったらサポートをしてみませんか?