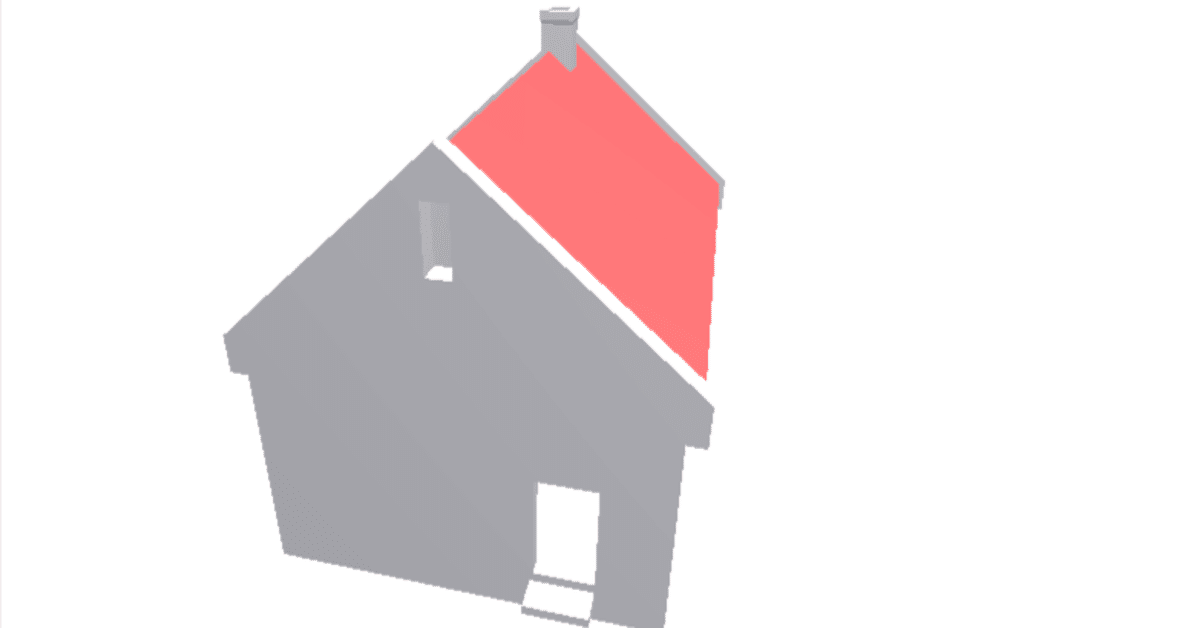
house.objを読み込んでオブジェクト毎にマテリアルを設定
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Load OBJ file</title>
<style>
body {
margin: 0;
overflow: hidden;
}
canvas {
display: block;
}
</style>
</head>
<body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r132/three.min.js"></script>
<script src="https://cdn.rawgit.com/mrdoob/three.js/r132/examples/js/loaders/OBJLoader.js"></script>
<script src="https://cdn.rawgit.com/mrdoob/three.js/r132/examples/js/controls/OrbitControls.js"></script>
<script>
let scene, camera, renderer, controls;
let obj;
init();
animate();
function init() {
// シーンを作成します
scene = new THREE.Scene();
// カメラを作成します
camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);
camera.position.y = 3;
camera.position.z = 4;
camera.lookAt(new THREE.Vector3(0, 1, 0));
// Add a point light to the scene
const sunLight = new THREE.PointLight(0xffffff, 1, 100, 1);
sunLight.position.set(10, 10, 10);
scene.add(sunLight);
// Add an ambient light to the scene
const ambientLight = new THREE.AmbientLight(0xeeeeff, 0.7);
scene.add(ambientLight);
// 平行光源を作成します
const directionalLight = new THREE.DirectionalLight(0xffffff, 1);
directionalLight.position.set(0, 3, 1);
scene.add(directionalLight);
// レンダラーを作成します
renderer = new THREE.WebGLRenderer();
renderer.setClearColor(0xffffff);
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// OrbitControlsを作成します
controls = new THREE.OrbitControls(camera, renderer.domElement);
// OBJファイルを読み込み、シーンに追加します
const house = createHouse(0, 0);
scene.add(house);
}
function createHouse(x, y) {
// OBJファイルを読み込み、シーンに追加します
const house = new THREE.Group();
const loader = new THREE.OBJLoader();
loader.load('house_002_3.obj', function(object) {
obj = object;
// マテリアルを作成します
const material1 = new THREE.MeshPhongMaterial({
color: new THREE.Color(0.5, 0.5, 0.5),
specular: new THREE.Color(0.1, 0.1, 0.1),
shininess: 0,
});
const material2 = new THREE.MeshPhongMaterial({
color: new THREE.Color(0.8, 0.2, 0.2),
specular: new THREE.Color(0.8, 0.1, 0.1),
shininess: 0,
});
const material3 = new THREE.MeshPhongMaterial({
color: new THREE.Color(0.8, 0.8, 0.8),
specular: new THREE.Color(0.5, 0.5, 0.5),
shininess: 0,
});
// オブジェクトの子要素にマテリアルを適用します
obj.traverse(function(child) {
if (child instanceof THREE.Mesh) {
// ここでオブジェクトの名前に基づいてマテリアルを割り当てます
if (child.name.includes('wall')) {
child.material = material1;
} else if (child.name.includes('roof')) {
child.material = material2;
} else if (child.name.includes('door')) {
child.material = material3;
}
}
});
house.add(obj);
house.position.set(x, 0, y);
});
return house;
}
function animate() {
requestAnimationFrame(animate);
// OrbitControlsを更新します
controls.update();
// オブジェクトを回転させます
if (obj) {
obj.rotation.y += 0.01;
}
camera.lookAt(new THREE.Vector3(0, 1, 0));
// レンダリングします
renderer.render(scene, camera);
}
</script>
</body>
</html>
この記事が気に入ったらサポートをしてみませんか?