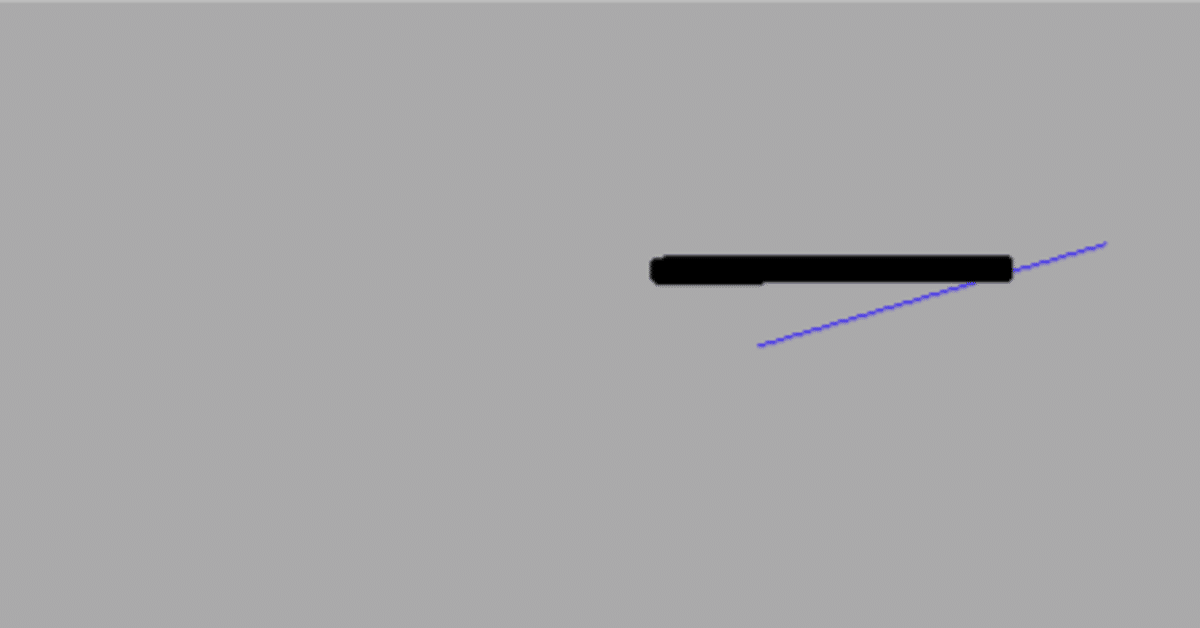
始点と終点を指定して円柱を線のように配置する関数
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Random Line</title>
<style>
body {
margin: 0;
padding: 0;
overflow: hidden;
}
</style>
</head>
<body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r132/three.min.js"></script>
<script>
function generatePoints() {
const startPoint = new THREE.Vector3(Math.random() * 50 - 25, Math.random() * 50 - 25, Math.random() * 50 - 25);
const endPoint = new THREE.Vector3(Math.random() * 50 - 25, Math.random() * 50 - 25, Math.random() * 50 - 25);
return {start: startPoint, end: endPoint};
}
function drawLine(start, end) {
const material = new THREE.LineBasicMaterial({ color: 0x0000ff });
const geometry = new THREE.BufferGeometry().setFromPoints([start, end]);
const line = new THREE.Line(geometry, material);
return line;
}
function extendCylinder(start, end) {
const radius = 1.0;
const height = start.distanceTo(end);
const position = start.clone().add(end).multiplyScalar(0.5);
const direction = end.clone().sub(start).normalize();
const geometry = new THREE.CylinderGeometry(radius, radius, height, 12);
const material = new THREE.MeshPhongMaterial({ color: 0x550000 });
const cylinder = new THREE.Mesh(geometry, material);
cylinder.position.set(position.x, position.y, position.z);
cylinder.quaternion.setFromUnitVectors(new THREE.Vector3(0, 1, 0), direction);
return cylinder;
}
function init() {
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(40, window.innerWidth / window.innerHeight, 0.1, 1000);
camera.position.y = 40;
camera.position.z = 120;
const renderer = new THREE.WebGLRenderer();
renderer.setClearColor(0xaaaaaa);
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
const points = generatePoints();
const line = drawLine(points.start, points.end);
const cylinder = extendCylinder(points.start, points.end);
scene.add(line);
scene.add(cylinder);
function animate() {
requestAnimationFrame(animate);
line.rotation.y += 0.002;
camera.lookAt(new THREE.Vector3(0, 0, 0));
renderer.render(scene, camera);
}
animate();
}
init();
</script>
</body>
</html>
この記事が気に入ったらサポートをしてみませんか?