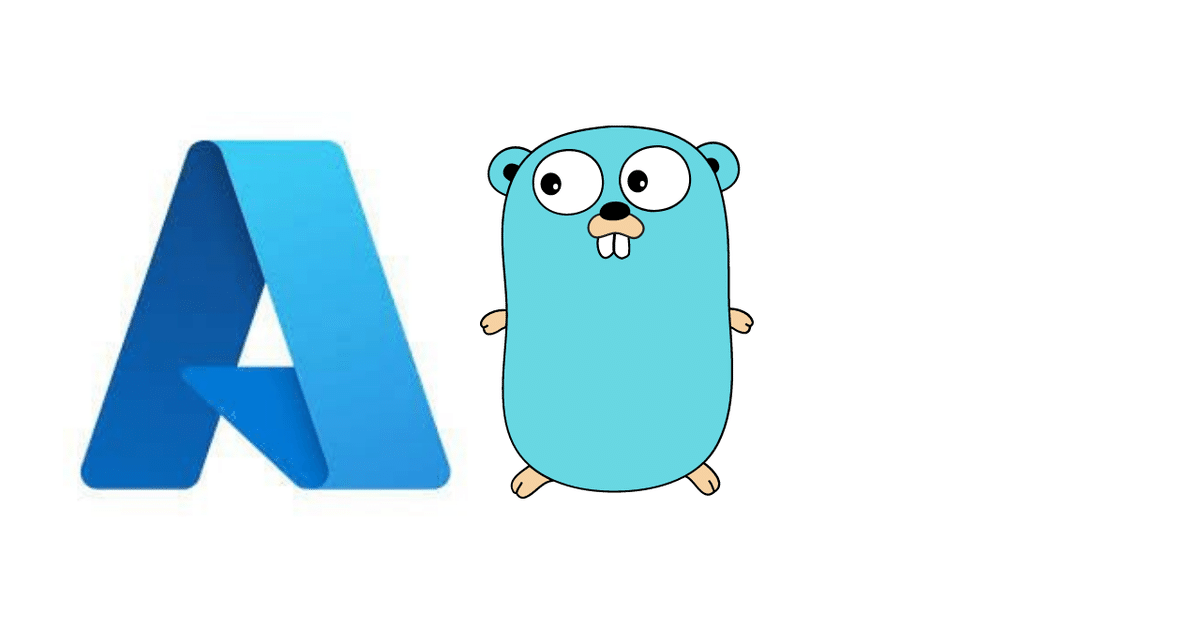
Azure App ServiceにAPIをデプロイする
Microsoft AzureのApp Service使って自作のAPIを公開しようと思います。
APIはDockerコンテナに格納されていて、そのDockerコンテナをPaaSのApp Serviceにデプロイします。
コードはこのような感じです。Go言語のGinというフレームワークを使い、APIは静的なJSONを返す簡単なものです。
package main
import "github.com/gin-gonic/gin"
type Customer struct {
Name string `json:"name"`
PhoneNo string `json:"phone_no"`
Email string `json:"email"`
}
func main() {
r := gin.Default()
r.GET("/customers", func(c *gin.Context){
var customers []Customer
customers = append(customers,
Customer{
Name: "John",
PhoneNo: "09011111111",
Email: "john@example.com",
},
Customer{
Name: "Beth",
PhoneNo: "09022222222",
Email: "beth@example.com",
},
)
c.JSON(200, gin.H{
"customers": customers,
})
})
r.Run()
}
こちらがDockerfileです。
軽量なAlpine Linuxを採用し、デプロイ時にコンパイルするように設定しておきます。
FROM golang:1.17.5-alpine3.15
RUN mkdir /app
WORKDIR /app
COPY . ./
RUN go mod download
RUN go build .
EXPOSE 8080
CMD [ "./gin-sample" ]
次にDockerコンテナを登録するためにAzureにコンテナーレジストリを作成します。
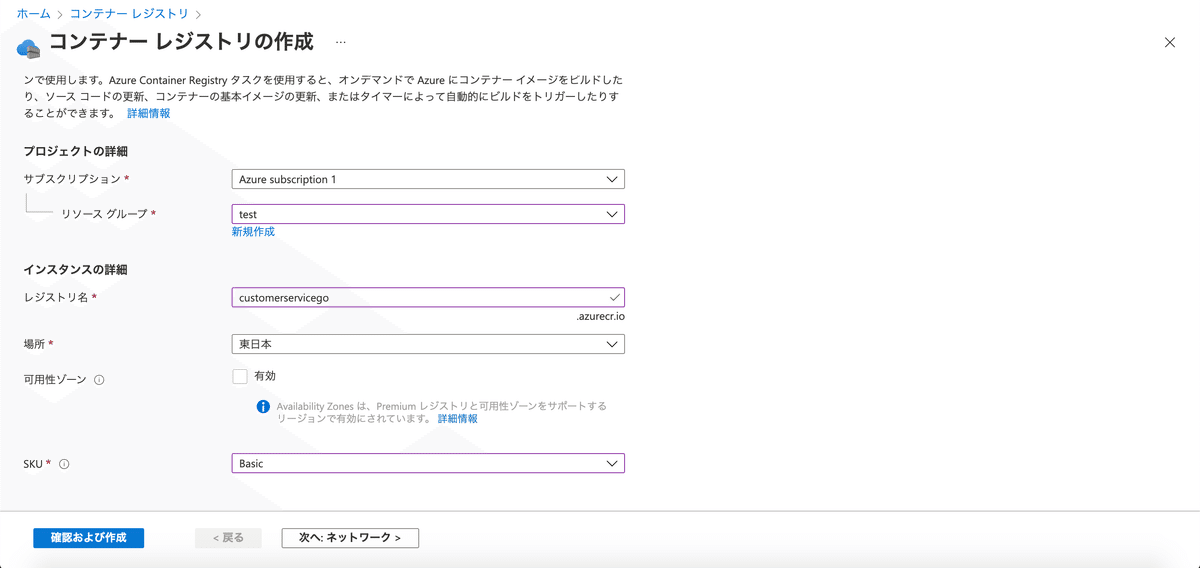
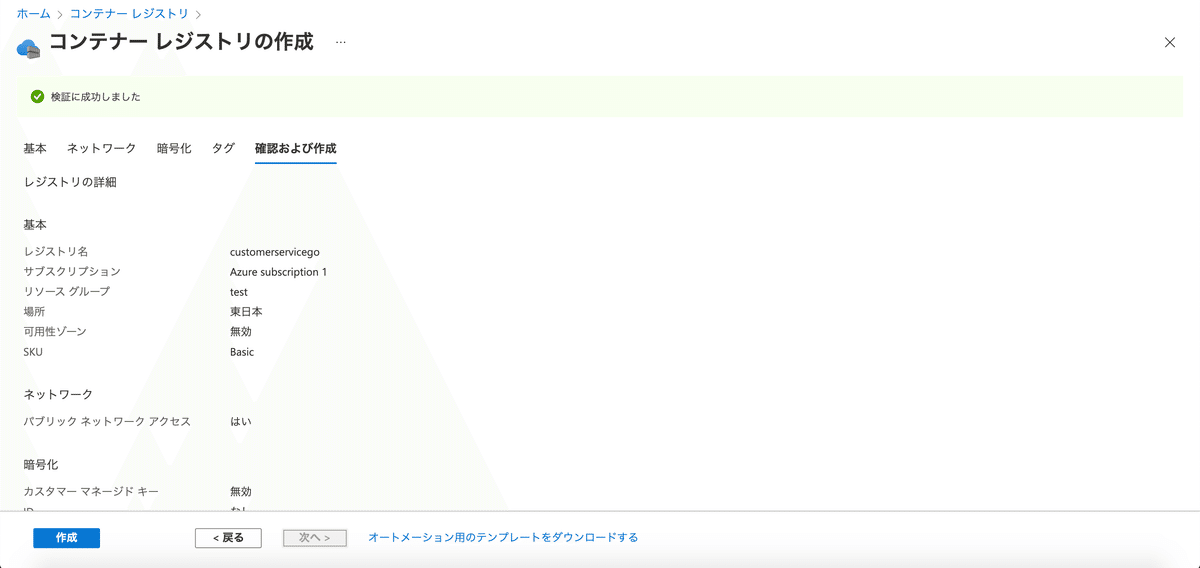
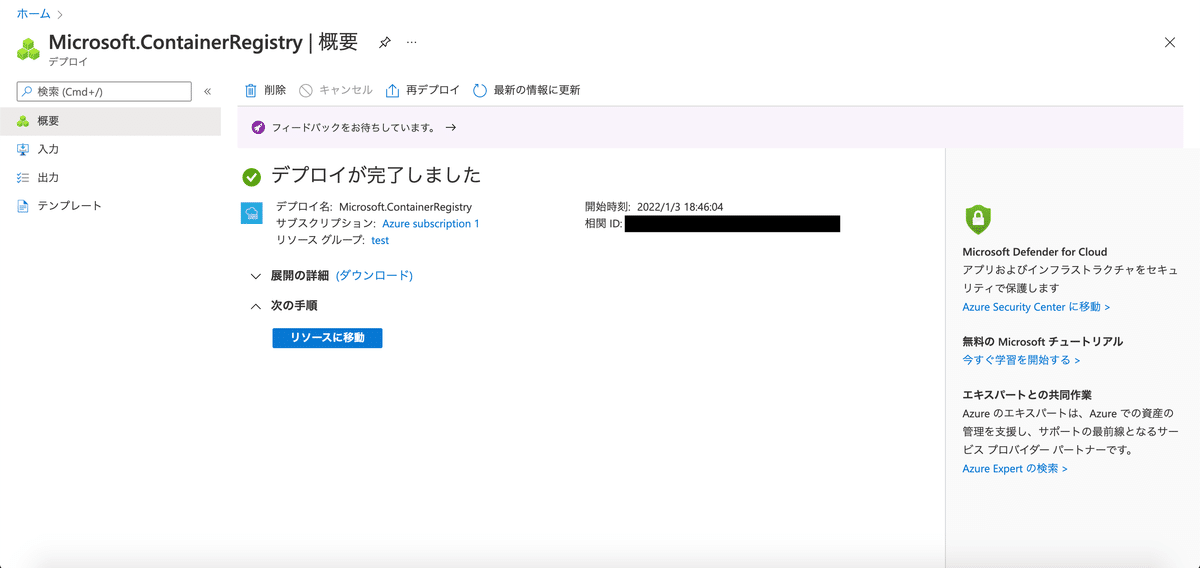
コンテナーレジストリにログインします。
$ az acr login --name customerservicego
Login Succeeded
Dockerイメージをビルドします。
$ docker build -t customerservicego.azurecr.io/customers:v1.0.0 .
[+] Building 17.0s (12/12) FINISHED
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 185B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/golang:1.17.5-alpine3.15 2.2s
=> [auth] library/golang:pull token for registry-1.docker.io 0.0s
=> CACHED [1/6] FROM docker.io/library/golang:1.17.5-alpine3.15@sha256:4918412049183afe42f1ecaf8f5c2a88917c2e 0.0s
=> [internal] load build context 0.1s
=> => transferring context: 3.67kB 0.0s
=> [2/6] RUN mkdir /app 0.4s
=> [3/6] WORKDIR /app 0.0s
=> [4/6] COPY . ./ 0.1s
=> [5/6] RUN go mod download 4.4s
=> [6/6] RUN go build . 8.1s
=> exporting to image 1.7s
=> => exporting layers 1.7s
=> => writing image sha256:2b50b322c2d82dd4e286269ab6d733af645dac861797fe739e8c1c4e9f083909 0.0s
=> => naming to customerservicego.azurecr.io/customers:v1.0.0 0.0s
Use 'docker scan' to run Snyk tests against images to find vulnerabilities and learn how to fix them
Dockerイメージをコンテナーレジストリにアップロードします。
$ docker push customerservicego.azurecr.io/customers:v1.0.0
The push refers to repository [customerservicego.azurecr.io/customers]
c0e0f58047a1: Pushed
c8aaa6bd771b: Pushed
0d59f3e6b54e: Pushed
5f70bf18a086: Pushed
74ed92ca381e: Pushed
97aa26b4fc43: Pushed
715cbda62b79: Pushed
b6f786c730a9: Pushed
63a6bdb95b08: Pushed
8d3ac3489996: Pushed
v1.0.0: digest: sha256:4b50a062a99d88626c447fbd98c1f6c4079c77450b6fa26217a8f513e1547384 size: 2413
アップロードができました。
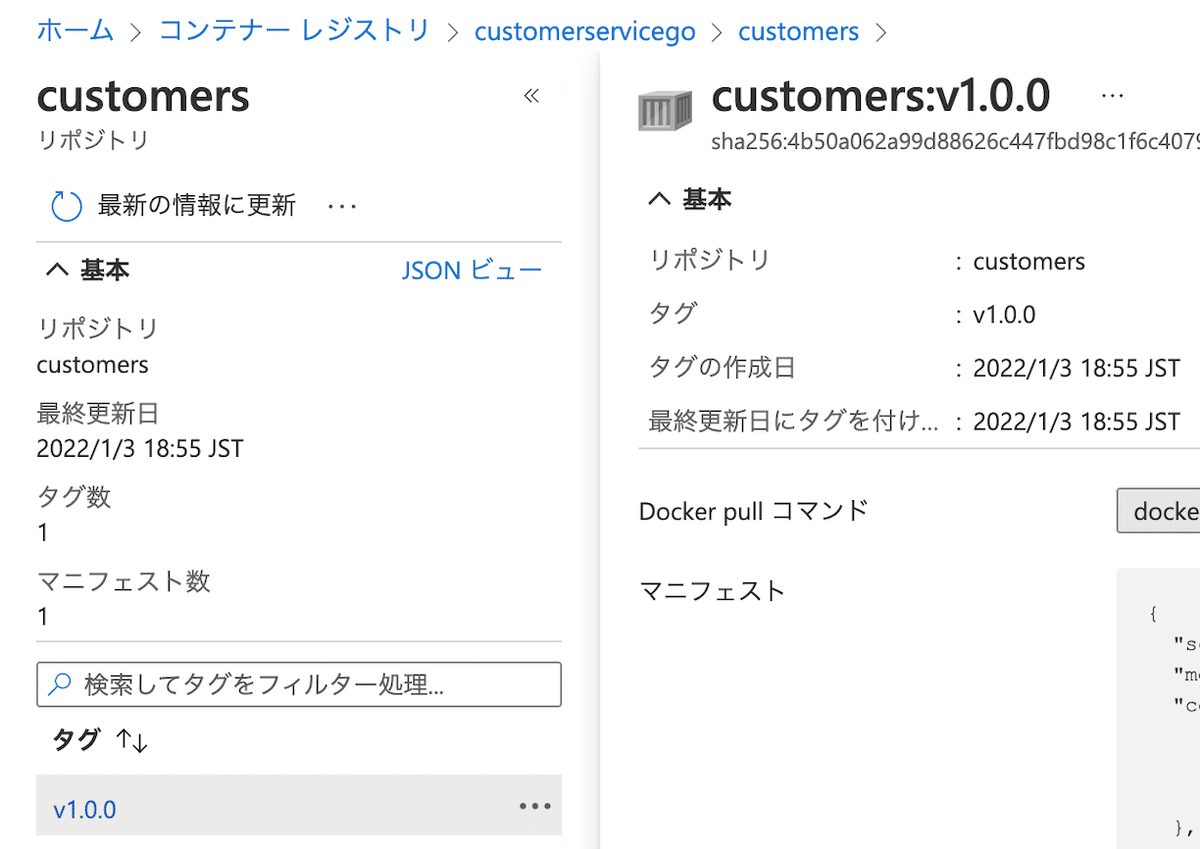
レジストリの管理者ユーザーを有効にします。
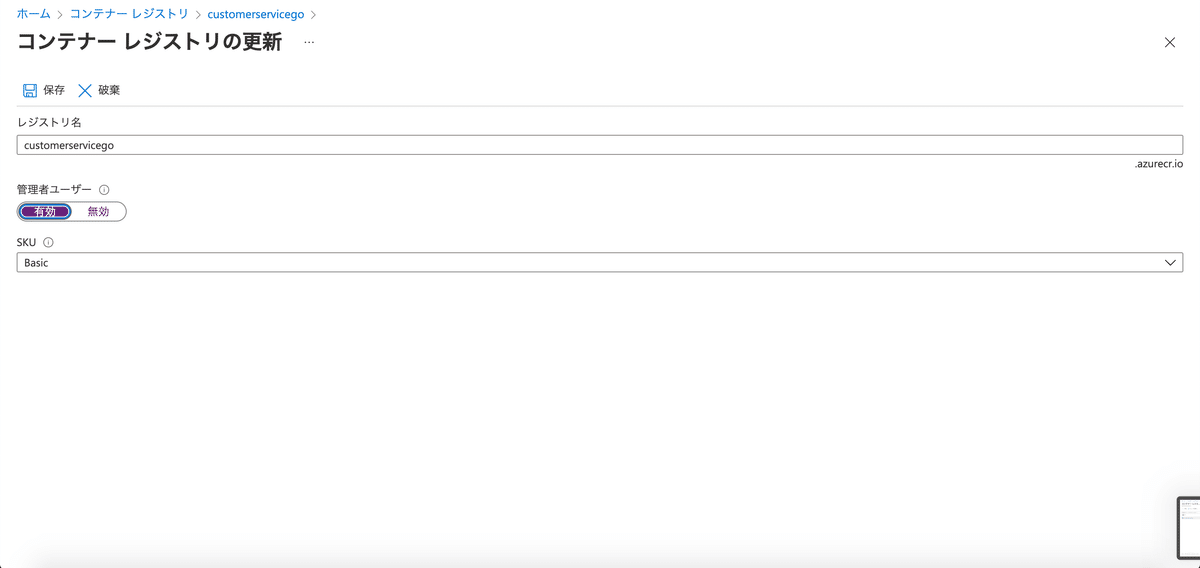
最後に登録したコンテナを使ってApp Serviceを作成し公開します。
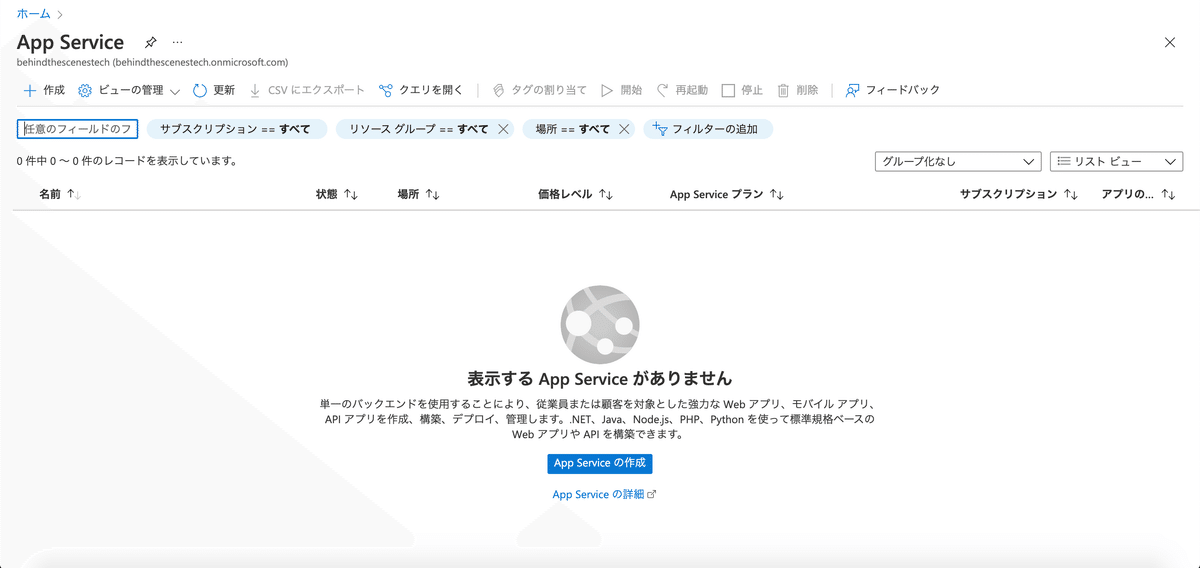
公開にDockerコンテナーを選択し、SKUは無料のF1を選択します。
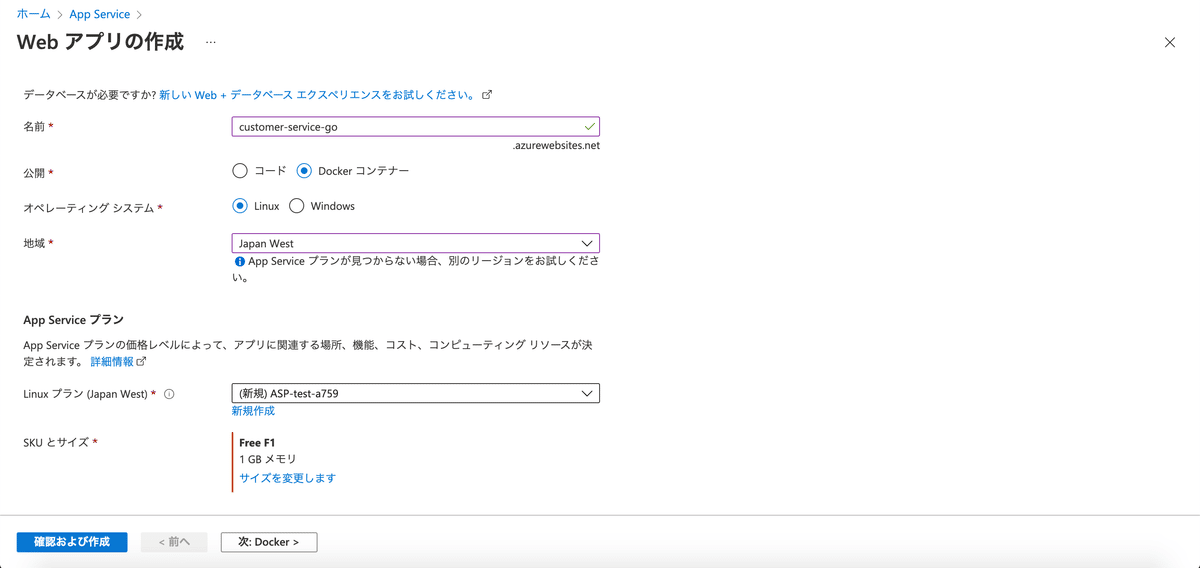
イメージソースにAzure Container Registryを選択し、先ほど登録したコンテナを指定します。
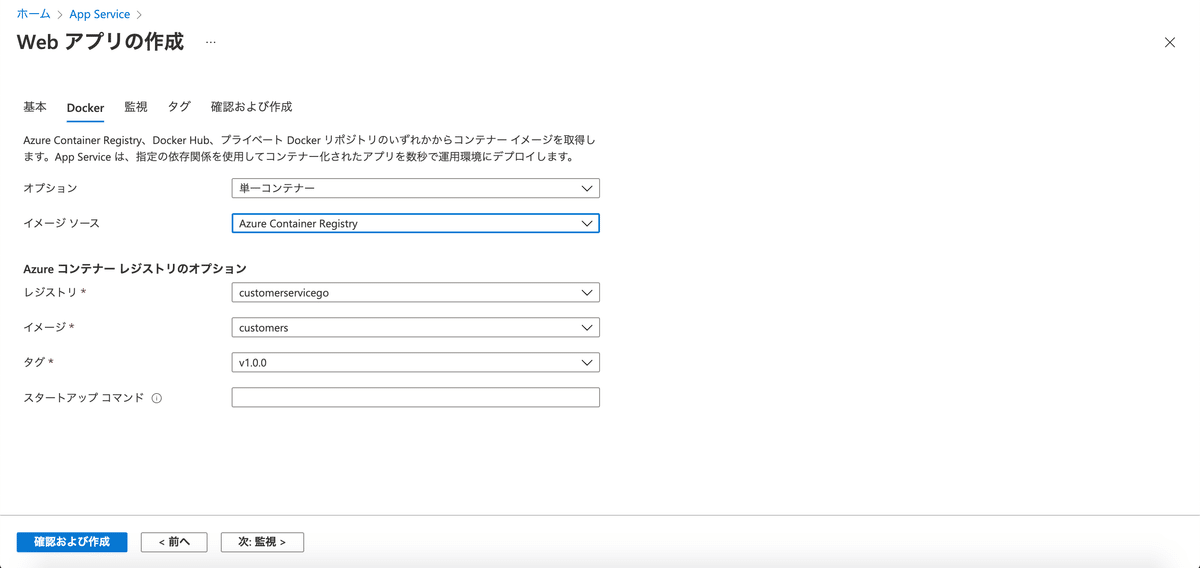
デプロイが完了しました。
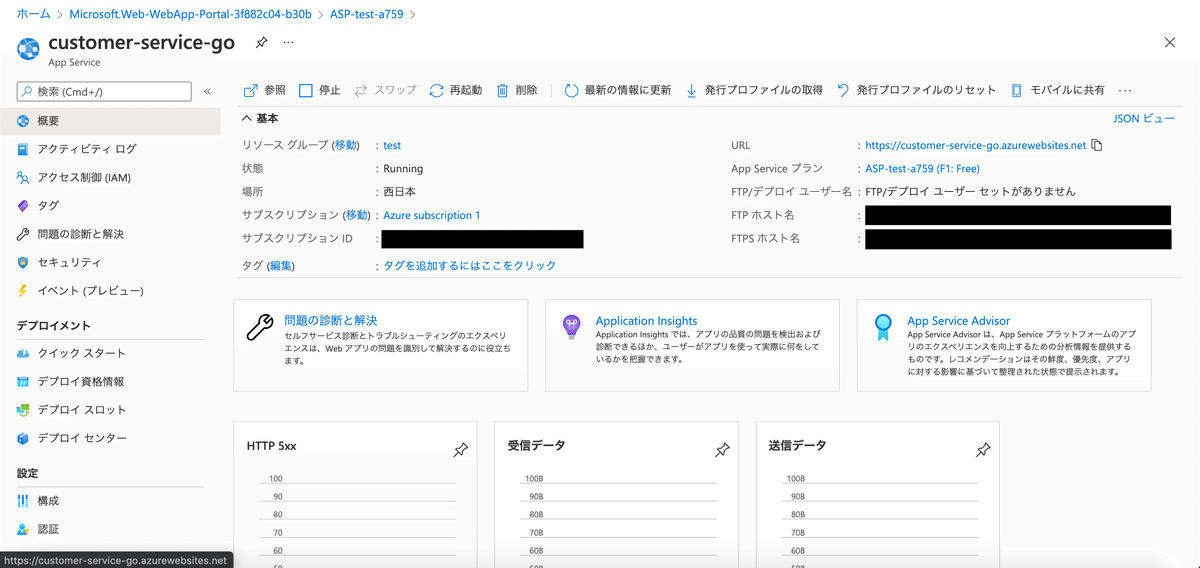
PostmanからもAPIが呼び出せています。
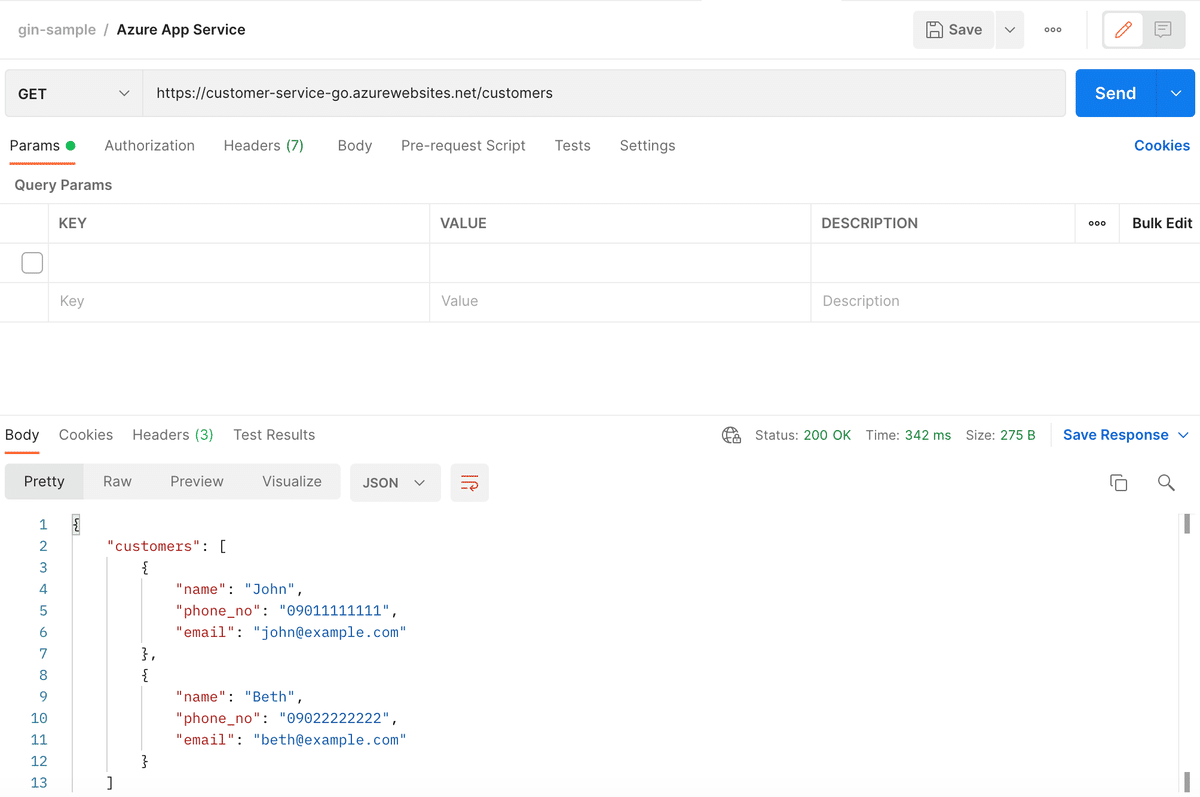
この記事が気に入ったらサポートをしてみませんか?