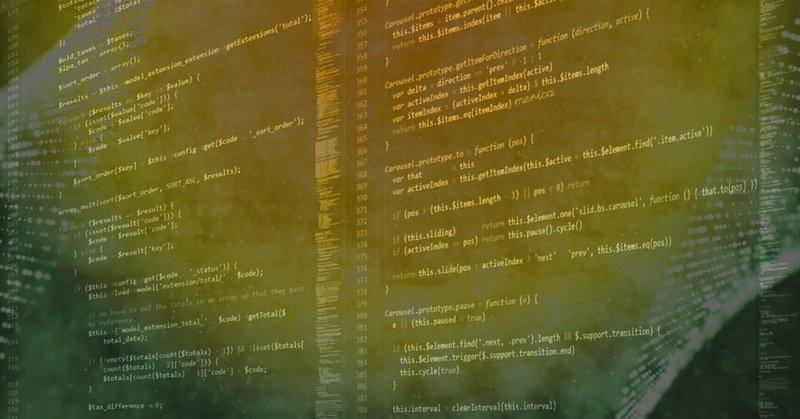
【Flutter】プロジェクトを新規作成したときにあるコメントアウトの和訳
新規作成時にデフォルトでコメントアウトされている文章は、Flutterについての簡単な紹介文です。
プログラミングスクールを運営している中で、生徒さんが意外と読まずに飛ばしているようなので、和訳して解説していきます。
以下がプロジェクト作成時のコードです。
コメントアウトの部分に番号を振っているので、その番号で参照してください。
デフォルトの『main.dart』コード
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
//(1) This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
//(2) This is the theme of your application.
//
// Try running your application with "flutter run". You'll see the
// application has a blue toolbar. Then, without quitting the app, try
// changing the primarySwatch below to Colors.green and then invoke
// "hot reload" (press "r" in the console where you ran "flutter run",
// or simply save your changes to "hot reload" in a Flutter IDE).
// Notice that the counter didn't reset back to zero; the application
// is not restarted.
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
//(3) This widget is the home page of your application. It is stateful, meaning
// that it has a State object (defined below) that contains fields that affect
// how it looks.
// This class is the configuration for the state. It holds the values (in this
// case the title) provided by the parent (in this case the App widget) and
// used by the build method of the State. Fields in a Widget subclass are
// always marked "final".
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
//(4) This call to setState tells the Flutter framework that something has
// changed in this State, which causes it to rerun the build method below
// so that the display can reflect the updated values. If we changed
// _counter without calling setState(), then the build method would not be
// called again, and so nothing would appear to happen.
_counter++;
});
}
@override
Widget build(BuildContext context) {
//(5) This method is rerun every time setState is called, for instance as done
// by the _incrementCounter method above.
//
// The Flutter framework has been optimized to make rerunning build methods
// fast, so that you can just rebuild anything that needs updating rather
// than having to individually change instances of widgets.
return Scaffold(
appBar: AppBar(
//(6) Here we take the value from the MyHomePage object that was created by
// the App.build method, and use it to set our appbar title.
title: Text(widget.title),
),
body: Center(
//(7) Center is a layout widget. It takes a single child and positions it
// in the middle of the parent.
child: Column(
//(8) Column is also a layout widget. It takes a list of children and
// arranges them vertically. By default, it sizes itself to fit its
// children horizontally, and tries to be as tall as its parent.
//
// Invoke "debug painting" (press "p" in the console, choose the
// "Toggle Debug Paint" action from the Flutter Inspector in Android
// Studio, or the "Toggle Debug Paint" command in Visual Studio Code)
// to see the wireframe for each widget.
//
// Column has various properties to control how it sizes itself and
// how it positions its children. Here we use mainAxisAlignment to
// center the children vertically; the main axis here is the vertical
// axis because Columns are vertical (the cross axis would be
// horizontal).
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
), //(9) This trailing comma makes auto-formatting nicer for build methods.
);
}
}
和訳
(1) This widget is the root of your application.
[和訳]
このウィジェットは、アプリケーションのルートです。
(2) This is the theme of your application.
Try running your application with "flutter run". You'll see the
application has a blue toolbar. Then, without quitting the app, try
changing the primarySwatch below to Colors.green and then invoke
"hot reload" (press "r" in the console where you ran "flutter run",
or simply save your changes to "hot reload" in a Flutter IDE).
Notice that the counter didn't reset back to zero; the application
is not restarted.
[和訳]
これがアプリケーションのテーマです。
「flutter run」でアプリケーションを実行してみてください。
アプリケーションには青いツールバーがあります。 次に、アプリを終了せずに、primarySwatchをColors.greenに変更してから、
「ホットリロード」を呼び出します。(「flutter run」を実行したコンソールで「r」を押すか、変更をIDE内で「ホットリロード」して保存するだけです)
カウンターがゼロにリセットされなかったことに注意してください。つまりアプリケーションは再起動されていません。
(3) This widget is the home page of your application. It is stateful, meaning
that it has a State object (defined below) that contains fields that affect
how it looks.
This class is the configuration for the state. It holds the values (in this
case the title) provided by the parent (in this case the App widget) and
used by the build method of the State. Fields in a Widget subclass are
always marked "final".
[和訳]
このウィジェットは、アプリケーションのホームページです。 これはステートフル、つまり見た目に影響を与えるフィールドを含む「状態オブジェクト(以下に定義)」であるという意味です。
このクラスは状態の構成です。 これは親(今回はAppウィジェット)に与えられた値(今回はtitle)と、状態のビルドメソッドで使用された値を保持しています。 ウィジェットサブクラスのフィールドは常に「final」と記述されます。
(4) This call to setState tells the Flutter framework that something has
changed in this State, which causes it to rerun the build method below
so that the display can reflect the updated values. If we changed
_counter without calling setState(), then the build method would not be
called again, and so nothing would appear to happen.
[和訳]
このsetStateの呼び出しは、Flutterフレームワークに状態に変化があったことを伝えることによって、以下のビルドメソッドが再実行され、画面に更新された値を反映できるようにします。 もしsetStateを呼び出さずに_counterを変更しても、ビルドメソッドは再度呼び出されずに何も起こりません。
(5) This method is rerun every time setState is called, for instance as done
by the _incrementCounter method above.
The Flutter framework has been optimized to make rerunning build methods
fast, so that you can just rebuild anything that needs updating rather
than having to individually change instances of widgets.
[和訳]
このメソッドは、上記の_incrementCounterメソッドのようにsetStateが呼び出されるたびに再実行されます。
Flutterフレームワークは、ビルドメソッドの再実行を高速化するように最適化されているため、ウィジェットのインスタンスを個別に変更する必要はなく、更新が必要なものをすべて再構築するできます。
(6) Here we take the value from the MyHomePage object that was created by
the App.build method, and use it to set our appbar title.
[和訳]
ここでは、App.buildメソッドによって作成されたMyHomePageオブジェクトから値を取得し、それを使用してAppBArのtitleを設定します。
(7) Center is a layout widget. It takes a single child and positions it
in the middle of the parent.
[和訳]
センターはレイアウトウィジェットです。 それはひとつのchildを取得し、それを親の真ん中に配置します。
(8) Column is also a layout widget. It takes a list of children and
arranges them vertically. By default, it sizes itself to fit its
children horizontally, and tries to be as tall as its parent.
Invoke "debug painting" (press "p" in the console, choose the
"Toggle Debug Paint" action from the Flutter Inspector in Android
Studio, or the "Toggle Debug Paint" command in Visual Studio Code)
to see the wireframe for each widget.
Column has various properties to control how it sizes itself and
how it positions its children. Here we use mainAxisAlignment to
center the children vertically; the main axis here is the vertical
axis because Columns are vertical (the cross axis would be
horizontal).
[和訳]
Columnはレイアウトウィジェットでもあります。 それはchildrenのリストを取り、それらを垂直方向に配置します。 デフォルトでは、childrenを水平方向に合わせてサイズを調整し、親と同じ高さにしようとします。
「デバッグペイント」を呼び出し(コンソールで「p」を押すか、AndroidStudioのFlutterInspectorから「ToggleDebugPaint」アクションを選択するか、Visual Studio Codeの「ToggleDebugPaint」コマンドを選択します)、各ウィジェットのワイヤーフレームを確認します 。
Columnには、それ自体のサイズとchildrenの配置方法を制御するためのさまざまなプロパティがあります。 ここでは、mainAxisAlignmentを使用して、子を垂直方向の中央に配置します。 Columnが垂直方向であるため、ここでの主軸は垂直軸です。(交差軸は水平方向になります)
(9) This trailing comma makes auto-formatting nicer for build methods.
[和訳]
この末尾のコンマによってビルドメソッドの自動フォーマットがより適切になります。
和訳版『main.dart』
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// このウィジェットは、アプリケーションのルートです。
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
// これがアプリケーションのテーマです。
//
// 「flutter run」でアプリケーションを実行してみてください。
// アプリケーションには青いツールバーがあります。 次に、アプリを終了せずに、primarySwatchをColors.greenに変更してから、
// 「ホットリロード」を呼び出します。(「flutter run」を実行したコンソールで「r」を押すか、変更をIDE内で「ホットリロード」して保存するだけです)
// カウンターがゼロにリセットされなかったことに注意してください。つまりアプリケーションは再起動されていません。
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
// このウィジェットは、アプリケーションのホームページです。
// これはステートフル、つまり見た目に影響を与えるフィールドを含む「状態オブジェクト(以下に定義)」であるという意味です。
// このクラスは状態の構成です。 これは親(今回はAppウィジェット)に与えられた値(今回はtitle)と、状態のビルドメソッドで使用された値を保持しています。
// ウィジェットサブクラスのフィールドは常に「final」と記述されます。
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
// このsetStateの呼び出しは、Flutterフレームワークに状態に変化があったことを伝えることによって、
// 以下のビルドメソッドが再実行され、画面に更新された値を反映できるようにします。
// もしsetStateを呼び出さずに_counterを変更しても、ビルドメソッドは再度呼び出されずに何も起こりません。
_counter++;
});
}
@override
Widget build(BuildContext context) {
// このメソッドは、上記の_incrementCounterメソッドのようにsetStateが呼び出されるたびに再実行されます。
// Flutterフレームワークは、ビルドメソッドの再実行を高速化するように最適化されているため、
// ウィジェットのインスタンスを個別に変更する必要はなく、更新が必要なものをすべて再構築するできます。
return Scaffold(
appBar: AppBar(
// ここでは、App.buildメソッドによって作成されたMyHomePageオブジェクトから値を取得し、
// それを使用してAppBArのtitleを設定します。
title: Text(widget.title),
),
body: Center(
// センターはレイアウトウィジェットです。 それはひとつのchildを取得し、それを親の真ん中に配置します。
child: Column(
// Columnはレイアウトウィジェットでもあります。 それはchildrenのリストを取り、それらを垂直方向に配置します。
// デフォルトでは、childrenを水平方向に合わせてサイズを調整し、親と同じ高さにしようとします。
// 「デバッグペイント」を呼び出し(コンソールで「p」を押すか、AndroidStudioのFlutterInspectorから「ToggleDebugPaint」アクションを選択するか、
// Visual Studio Codeの「ToggleDebugPaint」コマンドを選択します)、各ウィジェットのワイヤーフレームを確認します 。
// Columnには、それ自体のサイズとchildrenの配置方法を制御するためのさまざまなプロパティがあります。
// ここでは、mainAxisAlignmentを使用して、子を垂直方向の中央に配置します。 Columnが垂直方向であるため、ここでの主軸は垂直軸です。(交差軸は水平方向になります)
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
), // この末尾のコンマによってビルドメソッドの自動フォーマットがより適切になります。
);
}
}
この記事が気に入ったらサポートをしてみませんか?