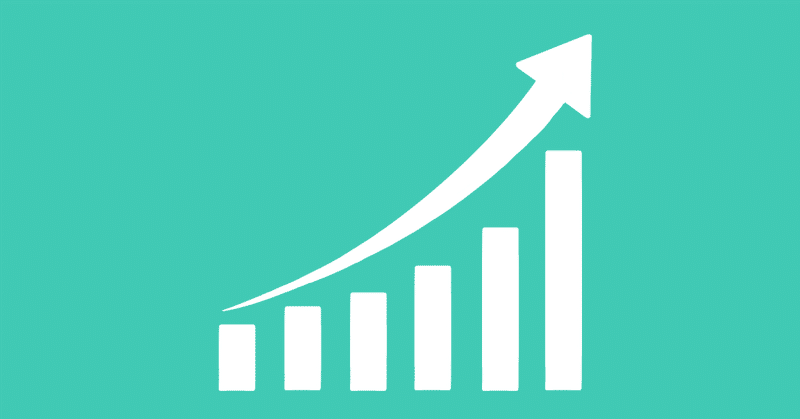
Photo by
tokyojack
ウォレットの損益を計算するコード
注意事項
ChatGptに作ってもらいました
ethチェーンのみ
利益・損失の計算
送金トランザクションの場合(ウォレットからの送金):その時点での価格と送金量を掛け合わせて損失として計上します。
受信トランザクションの場合(ウォレットへの受信):その時点での価格と受信量を掛け合わせて利益として計上します。
"YOUR_WALLET_ADDRESS_HERE"を取得したいウォレットアドレスに置き換えてください。
"YOUR_API_KEY"をEtherscanから取得したAPIキーに置き換えてください。
import requests
import pandas as pd
from datetime import datetime
def get_transactions(wallet_address):
url = f"https://api.etherscan.io/api?module=account&action=txlist&address={wallet_address}&startblock=0&endblock=99999999&sort=asc&apikey=YOUR_API_KEY"
response = requests.get(url)
transactions = response.json().get('result', [])
return transactions
def get_price_at_time(timestamp):
date = timestamp.strftime("%d-%m-%Y")
url = f"https://api.coingecko.com/api/v3/coins/ethereum/history?date={date}"
response = requests.get(url)
price = response.json().get('market_data', {}).get('current_price', {}).get('usd', 0)
return price
def calculate_total_profit_loss(wallet_address):
transactions = get_transactions(wallet_address)
total_profit_loss = 0
for transaction in transactions:
timestamp = datetime.utcfromtimestamp(int(transaction['timeStamp']))
price_at_time = get_price_at_time(timestamp)
value_in_eth = float(transaction['value']) / (10 ** 18) # Assuming 18 decimal places
profit_loss = price_at_time * value_in_eth
if transaction['from'] == wallet_address:
total_profit_loss -= profit_loss
else:
total_profit_loss += profit_loss
return total_profit_loss
def main():
wallet_address = "YOUR_WALLET_ADDRESS_HERE"
total_profit_loss = calculate_total_profit_loss(wallet_address)
if total_profit_loss > 0:
print(f"Total profit: ${total_profit_loss}")
else:
print(f"Total loss: ${total_profit_loss}")
if __name__ == "__main__":
main()
この記事が気に入ったらサポートをしてみませんか?