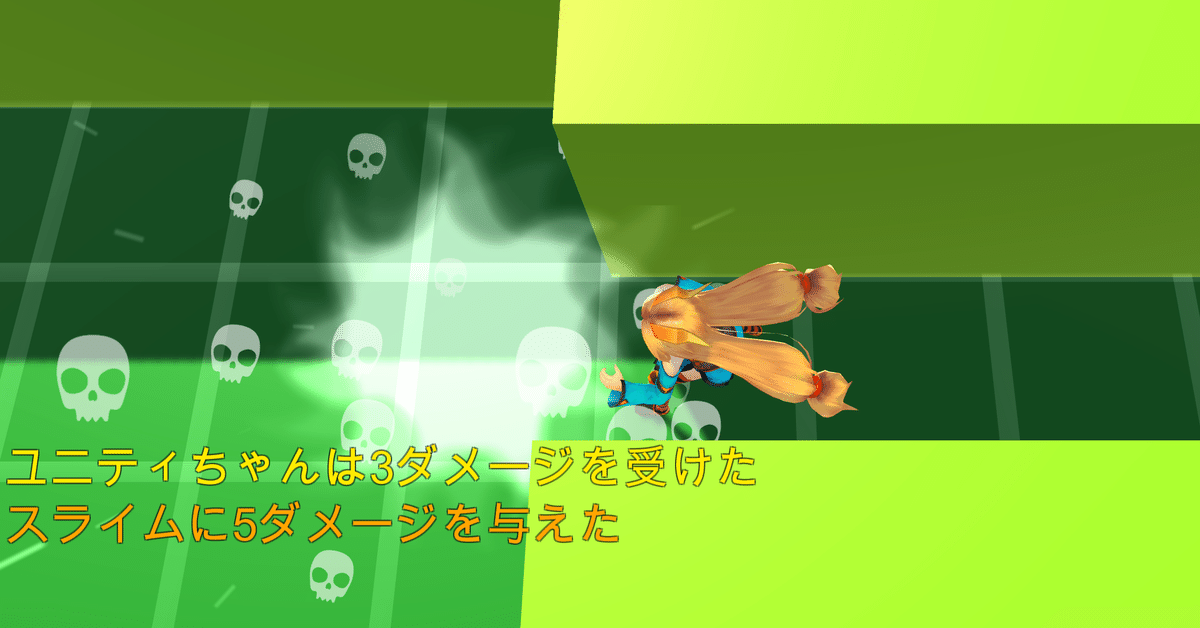
【Unity】3Dローグライクゲームの作り方〜Step13-1〜
前回の記事はこちら。
前回は敵をランダム配置しました。
キャラクターを倒せるようにする
今回からレベルアップのコードを書いていきたいと思います。
まずはキャラクターを倒せるようにするところから始めます。
ActorParamsControllerクラスに新しいメソッドを追加し、体力を減少するメソッドを変更します。
// メソッドを追加
/**
* 死亡判定
*/
private bool DeathJudgment()
{
if (parameter.hp <= 0)
{
if (parameter.id > EActor.PLAYER) Message.Add(28, actorName);
Destroy(gameObject);
return true;
}
return false;
}
// メソッドを変更
public void Damaged(int str)
{
int d = CalcDamage(str, parameter.def + equipment.GetAllDef());
parameter.hp -= d;
if (parameter.id == EActor.PLAYER) Message.Add(1, actorName, d.ToString());
else Message.Add(2, actorName, d.ToString());
if (!DeathJudgment()) ClearCondition(ECondition.Sleep); // ここを変更
}
public void DecreaseFood()
{
if (parameter.food > 0)
{
/* 省略 */
}
else
{
parameter.hp -= parameter.hpmax * decHungerHpPer;
DeathJudgment();
}
}
public void DamagedPoison()
{
if (conditions.Contains(ECondition.Poison))
{
effect.Play(EffectManager.EType.Poison, gameObject);
parameter.hp -= decPoisonPt;
DeathJudgment(); // これを追加
}
}
テストして、ダメージを与え続けると敵が倒れることを確認します。
また、毒でも倒れることを確認して下さい。
倒れたらエフェクトを表示する
折角なので、倒れたらエフェクトを再生するようにしてみましょう。なお、今回はEffectManagerスクリプトを使わず実装したいと思います。
まず、倒れた時のエフェクトを「DeathEffect」という名前で作成します。この際、 「アクションを停止」という項目があると思いますが、「破棄」を指定して下さい。これをプレハブ化します。
先ほど作成したDeathJudgmentメソッドに追記しましょう。
private bool DeathJudgment()
{
if (parameter.hp <= 0)
{
if (parameter.id > EActor.PLAYER) Message.Add(28, actorName);
// 以下3行を追記
GameObject effectObj = (GameObject)Resources.Load("Prefabs/DeathEffect");
GameObject effect = Instantiate(effectObj, GetComponentInParent<Field>().transform);
effect.transform.position += transform.position;
Destroy(gameObject);
return true;
}
return false;
}
テストしてみます。敵を倒した時やプレイヤーが倒れた時にエフェクトが再生されればOKです。
キャラクターを倒したら経験値を得られるようにする
それでは次に、キャラクターを倒したら経験値を得られるようにします。
なお、今回は直接キャラクターを倒した時のみ経験値が入ることにします。
ActorParamsControllerクラスに新しいメソッドを加え、Damagedメソッドを変更します。
// メソッドを追加
/*
* 経験値を得る
*/
public void GetXp(int xp)
{
parameter.exp += xp;
}
// メソッドを変更
public void Damaged(int str, out int xp)
{
int d = CalcDamage(str, parameter.def + equipment.GetAllDef());
parameter.hp -= d;
if (parameter.id == EActor.PLAYER) Message.Add(1, actorName, d.ToString());
else Message.Add(2, actorName, d.ToString());
xp = 0;
if (DeathJudgment()) xp = parameter.xp;
else ClearCondition(ECondition.Sleep);
}
これに伴い、ActorAttackとItemMovementクラスのメソッドを変更します。
// ActorAttackクラスのメソッド
public void DamageOpponent(GameObject actor)
{
if (actor == null) return;
ActorParamsController param = GetComponent<ActorParamsController>();
int str = param.parameter.str + param.equipment.GetAllAtk();
int xp = 0;
actor.GetComponent<ActorParamsController>().Damaged(str, out xp);
GetComponent<ActorParamsController>().GetXp(xp);
actor.GetComponent<ActorMovement>().TurnAround(GetComponent<ActorMovement>().direction);
}
// ItemMovementクラスのメソッド
private void HitActor(ActorParamsController tParam, GameObject actor)
{
/* 省略 */
else
{
ActorParamsController actorParam = actor.GetComponent<ActorParamsController>();
if (param.dmg > 0 || param.condition != ECondition.Normal)
{
actor.GetComponent<ActorMovement>().TurnAround(tParam.GetComponent<ActorMovement>().direction);
int str = param.dmg;
if (param.type != EItemType.Magic) str += tParam.parameter.str;
if (str > 0)
{
int xp = 0;
actorParam.Damaged(str, out xp);
tParam.GetXp(xp);
}
/* 省略 */
}
else if (param.hp > 0)
{
/* 省略 */
}
}
}
テストしてみます。敵を倒した時にexpが増えていればOKです。
という訳で、今回はここまでに致します。
次回はレベルアップの処理を実際に書いていきたいと思います。
この記事が気に入ったらサポートをしてみませんか?