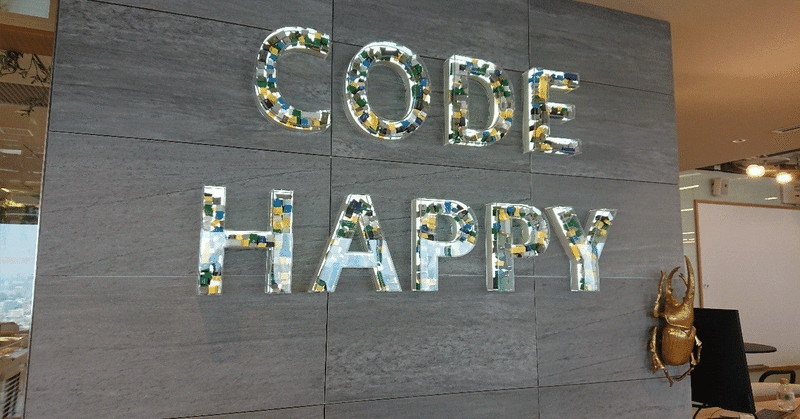
Photo by
m_miyamoto_529
CDK for Terraformでmoduleを利用する
事前準備
CDKTF のインストール(Mac)
terraformが別途インストールしてあるとエラーになるのでbrewで実施する場合はterraformはアンインストール推奨
brewでインストールするとterraformも合わせてインストールされる。
brew install cdktf
プロジェクト初期化
cdktf init --template="typescript" --providers="aws@~>4.0"
実行すると対話モードが開始されるのでそれぞれ入力
Newer version of Terraform CDK is available [0.19.0] - Upgrade recommended
Welcome to CDK for Terraform!
By default, cdktf allows you to manage the state of your stacks using Terraform Cloud for free.
cdktf will request an API token for app.terraform.io using your browser.
If login is successful, cdktf will store the token in plain text in
the following file for use by subsequent Terraform commands:
/Users/goalsymabashi/.terraform.d/credentials.tfrc.json
Note: The local storage mode isn't recommended for storing the state of your stacks.
? Do you want to continue with Terraform Cloud remote state management? no
? Project Name samplemodule
? Project Description samplemodule
? Do you want to start from an existing Terraform project? no
? Do you want to send crash reports to the CDKTF team? Refer to
https://developer.hashicorp.com/terraform/cdktf/create-and-deploy/configuration-file#enable-crash-reporting-for-the-cli for more information no
added 2 packages, and audited 57 packages in 1s
7 packages are looking for funding
run `npm fund` for details
found 0 vulnerabilities
added 314 packages, and audited 371 packages in 8s
38 packages are looking for funding
run `npm fund` for details
found 0 vulnerabilities
========================================================================================================
Your cdktf typescript project is ready!
cat help Print this message
Compile:
npm run get Import/update Terraform providers and modules (you should check-in this directory)
npm run compile Compile typescript code to javascript (or "npm run watch")
npm run watch Watch for changes and compile typescript in the background
npm run build Compile typescript
Synthesize:
cdktf synth [stack] Synthesize Terraform resources from stacks to cdktf.out/ (ready for 'terraform apply')
Diff:
cdktf diff [stack] Perform a diff (terraform plan) for the given stack
Deploy:
cdktf deploy [stack] Deploy the given stack
Destroy:
cdktf destroy [stack] Destroy the stack
Test:
npm run test Runs unit tests (edit __tests__/main-test.ts to add your own tests)
npm run test:watch Watches the tests and reruns them on change
Upgrades:
npm run upgrade Upgrade cdktf modules to latest version
npm run upgrade:next Upgrade cdktf modules to latest "@next" version (last commit)
Use Providers:
You can add prebuilt providers (if available) or locally generated ones using the add command:
cdktf provider add "aws@~>3.0" null kreuzwerker/docker
You can find all prebuilt providers on npm: https://www.npmjs.com/search?q=keywords:cdktf
You can also install these providers directly through npm:
npm install @cdktf/provider-aws
npm install @cdktf/provider-google
npm install @cdktf/provider-azurerm
npm install @cdktf/provider-docker
npm install @cdktf/provider-github
npm install @cdktf/provider-null
You can also build any module or provider locally. Learn more https://cdk.tf/modules-and-providers
========================================================================================================
[2023-10-30T14:40:01.148] [INFO] default - Checking whether pre-built provider exists for the following constraints:
provider: aws
version : ~>4.0
language: typescript
cdktf : 0.18.0
[2023-10-30T14:40:03.024] [INFO] default - Pre-built provider does not exist for the given constraints.
[2023-10-30T14:40:03.024] [INFO] default - Adding local provider registry.terraform.io/hashicorp/aws with version constraint ~>4.0 to cdktf.json
Local providers have been updated. Running cdktf get to update...
Generated typescript constructs in the output directory: .gen
以下のような感じで構成が作成される。
.
├── __tests__
├── cdktf.json
├── help
├── jest.config.js
├── main.ts
├── node_modules
├── package-lock.json
├── package.json
├── setup.js
└── tsconfig.json
providerインストール
npm install @cdktf/provider-aws
エラーになる場合
npm ERR! code ERESOLVE
npm ERR! ERESOLVE unable to resolve dependency tree
npm ERR!
npm ERR! While resolving: ModuleSample@1.0.0
npm ERR! Found: cdktf@0.18.2
npm ERR! node_modules/cdktf
npm ERR! cdktf@"^0.18.2" from the root project
npm ERR!
npm ERR! Could not resolve dependency:
npm ERR! peer cdktf@"^0.19.0" from @cdktf/provider-aws@18.0.3
npm ERR! node_modules/@cdktf/provider-aws
npm ERR! @cdktf/provider-aws@"*" from the root project
npm ERR!
npm ERR! Fix the upstream dependency conflict, or retry
npm ERR! this command with --force or --legacy-peer-deps
npm ERR! to accept an incorrect (and potentially broken) dependency resolution.
npm ERR!
npm ERR!
npm ERR! For a full report see:
Version指定でインストールする
npm install @cdktf/provider-aws@17.0.11
added 1 package, and audited 372 packages in 4s
38 packages are looking for funding
run `npm fund` for details
found 0 vulnerabilities
cdktf.jsonの変更
利用するモジュールを定義する。
"terraformModules": [
{
"name": "sqs",
"source": "terraform-aws-modules/sqs/aws",
"version": "4.1.0"
}
main.ts修正
import { Construct } from "constructs";
import { App, TerraformStack } from "cdktf";
import { AwsProvider } from "@cdktf/provider-aws/lib/provider";
import { sqsQueue } from '@cdktf/provider-aws';
class MyStack extends TerraformStack {
constructor(scope: Construct, id: string) {
super(scope, id);
new AwsProvider(this, 'aws', {
region: 'ap-northeast-1', // Example: 'us-west-2'
defaultTags: [{
tags: {
environment: process.env.ENV_ID || `${id}`,
}
}]
});
// define resources here
new sqsQueue.SqsQueue(this, 'queue', {
name: `${id}-queue`,
});
}
}
const app = new App();
new MyStack(app, "samplemodule");
app.synth();
Getする
moduleがダウンロードされる。
cdktf get
CDKTFでのプロビジョニング
DryRun
cdktf diff
Apply
cdktf deploy
確認
cdktf list
Stack name Path
samplemodule cdktf.out/stacks/samplemodule
Terraformでの実行
chdir指定でterraformのコマンドも実行できる。
作成したリソースの詳細を確認するためのshowはCDKTFでは未実装なので、確認したい場合はterraformで実行することもできる。
CDKTFではterraformで実装されているtargetオプションも存在しないため、特定のリソースのみに更新かけたい場合はterraformで実行する必要がある。※2023/10/30時点
terraform -chdir="cdktf.out/stacks/samplemodule" state list
aws_sqs_queue.queue
terraform -chdir="cdktf.out/stacks/samplemodule" show
# aws_sqs_queue.queue:
resource "aws_sqs_queue" "queue" {
arn = "arn:aws:sqs:ap-northeast-1:0123456789:samplemodule-queue"
content_based_deduplication = false
delay_seconds = 0
fifo_queue = false
id = "https://sqs.ap-northeast-1.amazonaws.com/0123456789/samplemodule-queue"
kms_data_key_reuse_period_seconds = 300
max_message_size = 262144
message_retention_seconds = 345600
name = "samplemodule-queue"
receive_wait_time_seconds = 0
sqs_managed_sse_enabled = true
tags_all = {
"environment" = "samplemodule"
}
url = "https://sqs.ap-northeast-1.amazonaws.com/0123456789/samplemodule-queue"
visibility_timeout_seconds = 30
}
リソース削除
cdktf destroy
この記事が気に入ったらサポートをしてみませんか?