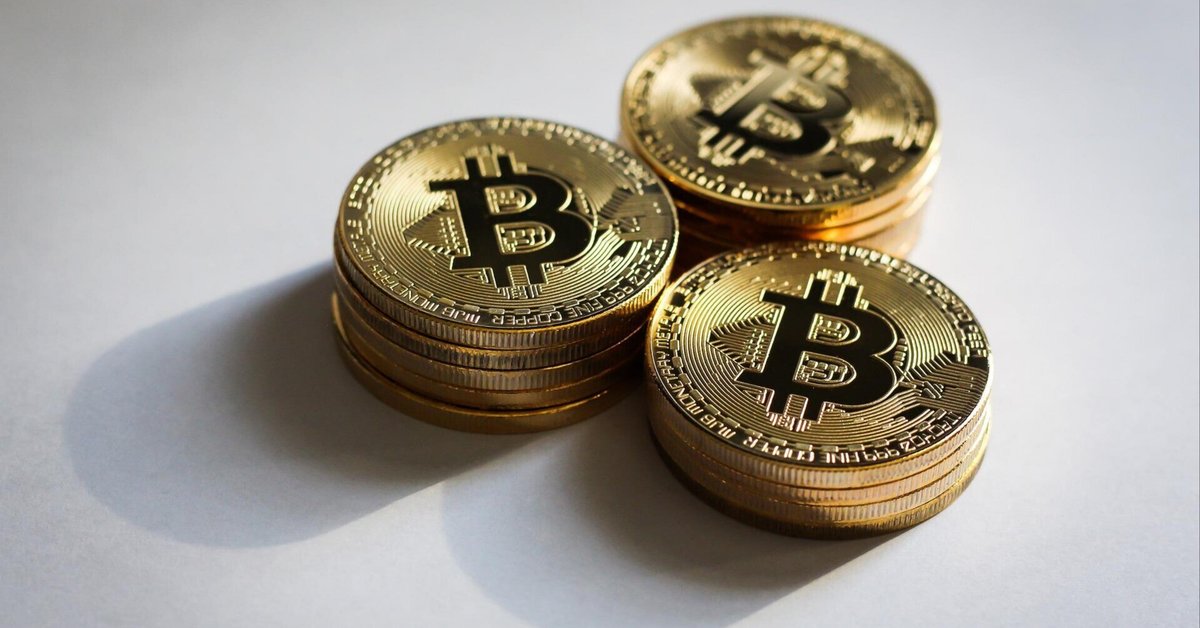
FreeBSD ✖️ truffle でブロックチェーン演習
目標
仮想マシン(FreeBSD)で truffle を使って ERC20 トークンを作成するコードを用意します。それを、ホストマシンで動いている Ganache にデプロイします。
手順
まずは、truffle を使えるか試してみる。
[root@vitothon /usr/local/etc]# pkg search npm
npm-8.13.0 Node package manager
npm-node14-8.13.0 Node package manager
npm-node16-8.13.0 Node package manager
npm-node18-8.13.0 Node package manager
[root@vitothon /usr/local/etc]# pkg install npm-node16-8.13.0
[root@vitothon /usr/local/etc]# npm -v
8.13.0
[root@vitothon /usr/local/etc]# node -v
v16.15.1
[root@vitothon /usr/local/etc]# npm install -g npm@8.14.0
[root@vitothon /usr/local/etc]# npm search truffle
NAME | DESCRIPTION | AUTHOR | DATE | VERSION | KEYWORDS
truffle | Truffle - Simple… | =sukanyaparash… | 2022-07-15 | 5.5.22 |
...
[root@vitothon /usr/local/etc]# npm install -g truffle
[root@vitothon /home/shuma]# mkdir Fikacoin
[root@vitothon /home/shuma]# cd Fikacoin/
[root@vitothon /home/shuma/Fikacoin]# truffle init
[root@vitothon /home/shuma/Fikacoin]# ls
contracts migrations test truffle-config.js
[root@vitothon /home/shuma/Fikacoin]# npm init -f
[root@vitothon /home/shuma/Fikacoin]# npm install zeppelin-solidity --save
サンプルトークン contracts/Fikacoin.sol 作る。
pragma solidity ^0.8.4;
import "zeppelin-solidity/contracts/token/ERC20/StandardToken.sol";
contract Fikacoin is StandardToken {
string public name = "Fikacoin";
string public symbol = "FKC";
uint public decimals = 18;
function Fikacoin(uint initialSupply) public {
totalSupply_ = initialSupply;
balances[msg.sender] = initialSupply;
}
}
コンパイルしてみる。
[root@vitothon /home/shuma/Fikacoin]# truffle compile
Compiling your contracts...
===========================
✓ Fetching solc version list from solc-bin. Attempt #1
✓ Downloading compiler. Attempt #1.
> Compiling ./contracts/Fikacoin.sol
> Compiling ./contracts/Migrations.sol
> Compiling zeppelin-solidity/contracts/token/ERC20/StandardToken.sol
> Compilation warnings encountered:
Warning: SPDX license identifier not provided in source file. Before publishing, consider adding a comment containing "SPDX-License-Identifier: <SPDX-License>" to each source file. Use "SPDX-License-Identifier: UNLICENSED" for non-open-source code. Please see <https://spdx.org> for more information.
--> project:/contracts/Fikacoin.sol
CompileError: ParserError: Source file requires different compiler version (current compiler is 0.8.15+commit.e14f2714.Emscripten.clang) - note that nightly builds are considered to be strictly less than the released version
--> zeppelin-solidity/contracts/token/ERC20/StandardToken.sol:1:1:
|
1 | pragma solidity ^0.4.24;
| ^^^^^^^^^^^^^^^^^^^^^^^^
Error: Truffle is currently using solc 0.8.15, but one or more of your contracts specify "pragma solidity ^0.4.24".
Please update your truffle config or pragma statement(s).
(See <https://trufflesuite.com/docs/truffle/reference/configuration#compiler-configuration> for information on
configuring Truffle to use a specific solc compiler version.)
Compilation failed. See above.
at /usr/local/lib/node_modules/truffle/build/webpack:/packages/compile-solidity/dist/run.js:64:1
at Generator.next (<anonymous>)
at fulfilled (/usr/local/lib/node_modules/truffle/build/webpack:/packages/compile-solidity/dist/run.js:4:43)
Truffle v5.5.22 (core: 5.5.22)
Node v16.15.1
バージョンの違いで、コンパイルに失敗する。zeppelin-solidity が使えないかも。代わりに @openzeppelin/contracts を使ってみる。
[root@vitothon /home/shuma/Fikacoin]# npm install @openzeppelin/contracts --save
contracts/Fikacoin.sol を以下のように修正。
pragma solidity ^0.8.4;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract Fikacoin is ERC20 {
constructor(uint256 initialSupply) ERC20("Fikacoin", "FKC") {
_mint(msg.sender, initialSupply);
}
}
もう一度コンパイルしてみる。
[root@vitothon /home/shuma/Fikacoin]# truffle compile
Compiling your contracts...
===========================
> Compiling ./contracts/Fikacoin.sol
> Compiling ./contracts/Migrations.sol
> Compiling @openzeppelin/contracts/token/ERC20/ERC20.sol
> Compiling @openzeppelin/contracts/token/ERC20/IERC20.sol
> Compiling @openzeppelin/contracts/token/ERC20/extensions/IERC20Metadata.sol
> Compiling @openzeppelin/contracts/utils/Context.sol
> Compilation warnings encountered:
Warning: SPDX license identifier not provided in source file. Before publishing, consider adding a comment containing "SPDX-License-Identifier: <SPDX-License>" to each source file. Use "SPDX-License-Identifier: UNLICENSED" for non-open-source code. Please see <https://spdx.org> for more information.
--> project:/contracts/Fikacoin.sol
> Artifacts written to /usr/home/shuma/Fikacoin/build/contracts
> Compiled successfully using:
- solc: 0.8.15+commit.e14f2714.Emscripten.clang
成功した。次にマイグレーションファイル migrations/2_deploy_myToken.js を作成する。
const Fikacoin = artifacts.require('./Fikacoin.sol')
module.exports = (deployer) => {
const initialSupply = 100000000
deployer.deploy(Fikacoin, initialSupply)
}
とりあえず、ホストマシンの Ganache で確認するために truffle-config.js を修正する。
# ホストマシンでネットワーク状況を確認
user$ ifconfig
...
vboxnet0: flags=8943<UP,BROADCAST,RUNNING,PROMISC,SIMPLEX,MULTICAST> mtu 1500
ether 0a:00:27:00:00:00
inet 192.168.56.1 netmask 0xffffff00 broadcast 192.168.56.255
# FreeBSD の truffle-config.js を修正する
networks: {
development: {
host: "192.168.56.1", // Localhost (default: none)
port: 8545, // Standard Ethereum port (default: none)
network_id: "*", // Any network (default: none)
},
}
ホストマシンの Ganache のワークスペースは以下のように設定。
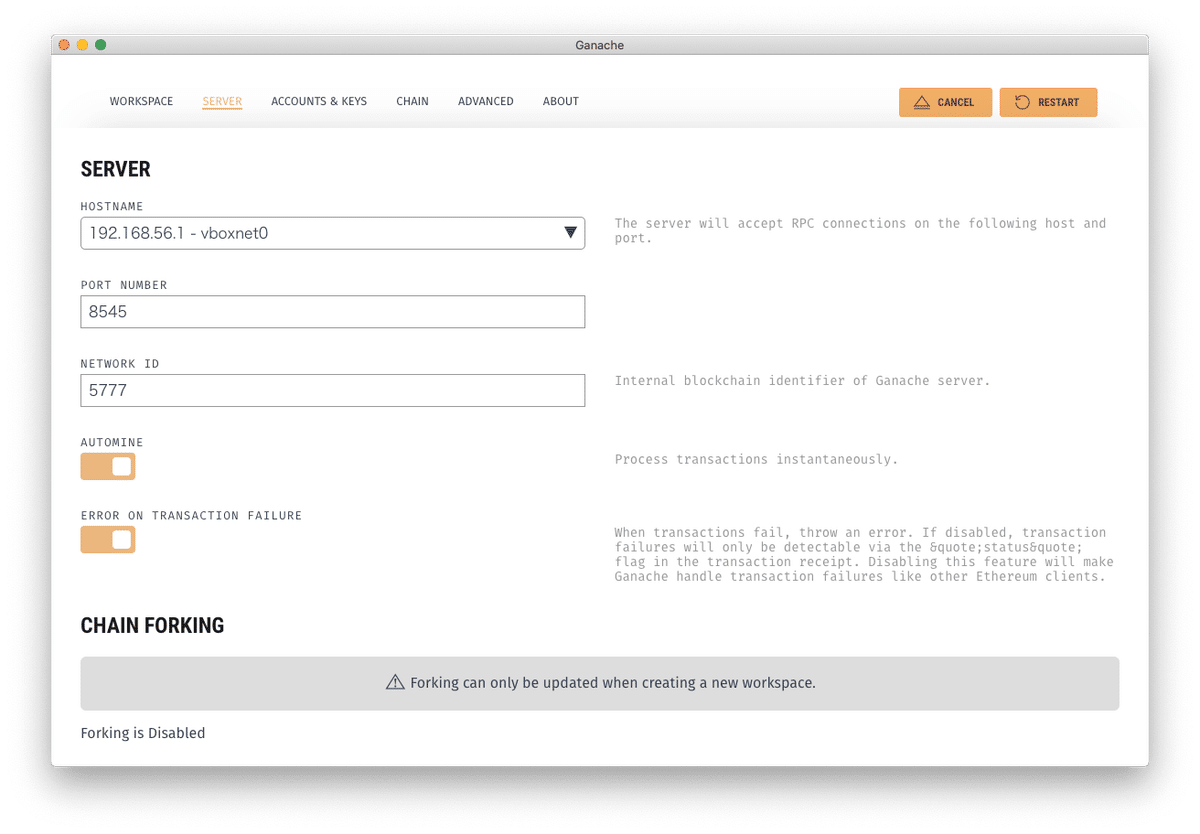
deploy してみる。
[root@vitothon /home/shuma/Fikacoin]# truffle deploy
This version of µWS is not compatible with your Node.js build:
Error: Cannot find module './uws_freebsd_x64_93.node'
Falling back to a NodeJS implementation; performance may be degraded.
Compiling your contracts...
===========================
> Everything is up to date, there is nothing to compile.
Starting migrations...
======================
> Network name: 'development'
> Network id: 5777
> Block gas limit: 67219750000 (0xfa69bfc70)
2_deploy_myToken.js
===================
Deploying 'Fikacoin'
--------------------
> transaction hash: 0x8aa71197246a8072b3ea0aecf245209fea5c4e2850c175380601b1bfbd3cf8e8
> Blocks: 0 Seconds: 0
> contract address: 0x13476AD53e77909F0059890bBF13E1D4fe880105
> block number: 3
> block timestamp: 1658060225
> account: 0x1c064ff51B520343c99be874279795306fBd4684
> balance: 99.97060556
> gas used: 1178355 (0x11faf3)
> gas price: 20 gwei
> value sent: 0 ETH
> total cost: 0.0235671 ETH
> Saving migration to chain.
> Saving artifacts
-------------------------------------
> Total cost: 0.0235671 ETH
Summary
=======
> Total deployments: 1
> Final cost: 0.0235671 ETH
成功!
次回
FreeBSD の jails でそれぞれブロックチェーンのノードを動かして、プライベートチェーンを動かす。
この記事が気に入ったらサポートをしてみませんか?