Selenium Grid とPythonで並列処理テスト
概要
Selenium GridとPythonのコンテナを作成して並列実行のテストをする。
やったこと
Docker環境の準備
ディレクトリ構成は以下
.
│ docker-compose.yml
│
└─python
│ Dockerfile
│ requirements.txt
│
├─bin
│ test-serenium.py
│
└─inout
Dockerfile
FROM python:3.9
WORKDIR /usr/src/app
ARG passwd
RUN groupadd -g 61000 batch
RUN useradd -u 61001 -g 61000 -m \
-p $(perl -e 'print crypt(${passwd}, "\$6\$salt03")') \
-s /bin/bash pybatch
USER pybatch
COPY requirements.txt ./
RUN pip install --no-cache-dir -r requirements.txt
RUN mkdir ~/bin
requirements.txt
selenium==4.1.3
docker-compose.yml
version: "3"
services:
chrome1:
image: selenium/node-chrome:4.1.4-20220427
shm_size: 2gb
depends_on:
- selenium-hub
environment:
- SE_EVENT_BUS_HOST=selenium-hub
- SE_EVENT_BUS_PUBLISH_PORT=4442
- SE_EVENT_BUS_SUBSCRIBE_PORT=4443
chrome2:
image: selenium/node-chrome:4.1.4-20220427
shm_size: 2gb
depends_on:
- selenium-hub
environment:
- SE_EVENT_BUS_HOST=selenium-hub
- SE_EVENT_BUS_PUBLISH_PORT=4442
- SE_EVENT_BUS_SUBSCRIBE_PORT=4443
chrome3:
image: selenium/node-chrome:4.1.4-20220427
shm_size: 2gb
depends_on:
- selenium-hub
environment:
- SE_EVENT_BUS_HOST=selenium-hub
- SE_EVENT_BUS_PUBLISH_PORT=4442
- SE_EVENT_BUS_SUBSCRIBE_PORT=4443
selenium-hub:
image: selenium/hub:4.1.4-20220427
container_name: selenium-hub
ports:
- "4442:4442"
- "4443:4443"
- "4444:4444"
python-container:
build:
context: ./python
args:
passwd: passwd
image: selenium-python
container_name: selenium-python-container
working_dir: "/home/pybatch/python"
tty: true
volumes:
- ./python:/home/pybatch/python
テスト実行
テストプログラム
yahooのトップ画面をスクショするだけのプログラム。
3並列で実行するプログラムをいかに載せている。並列数を変えるときはmax_workersを変更する。
from selenium import webdriver
from concurrent import futures
def test(i):
screen_shot_file_path = f'/home/pybatch/python/inout/screen_shot{i}.png'
with webdriver.Remote(
command_executor=f'http://selenium-hub:4444/wd/hub',
options=webdriver.ChromeOptions(),
) as driver:
driver.get('https://www.yahoo.co.jp/')
print(i, driver.current_url)
# スクリーンショットを取って保存
driver.save_screenshot(screen_shot_file_path)
future_list = []
with futures.ThreadPoolExecutor(max_workers=3) as executor:
for i in range(10):
future = executor.submit(test, i)
future_list.append(future)
_ = futures.as_completed(fs=future_list)
実行前
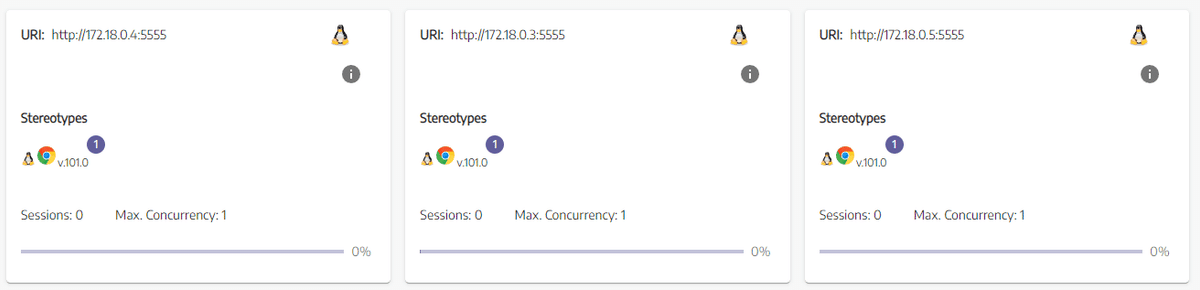
2並列で実行した場合
2ノードが使用されていることがわかる。
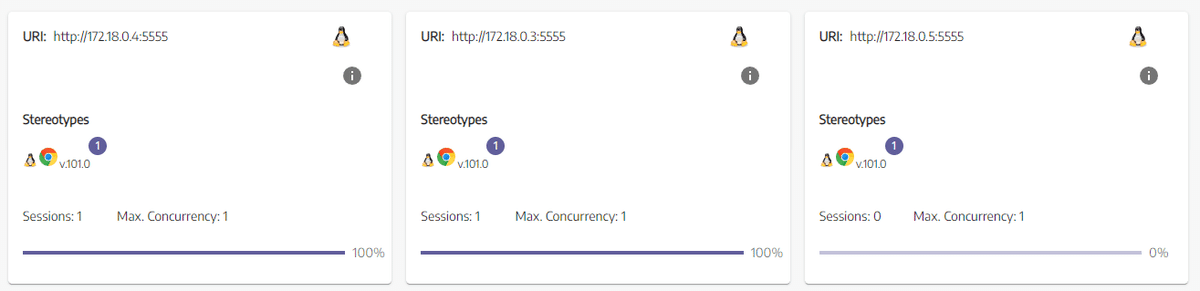
3並列で実行した場合
3ノードが使用されていることがわかる。
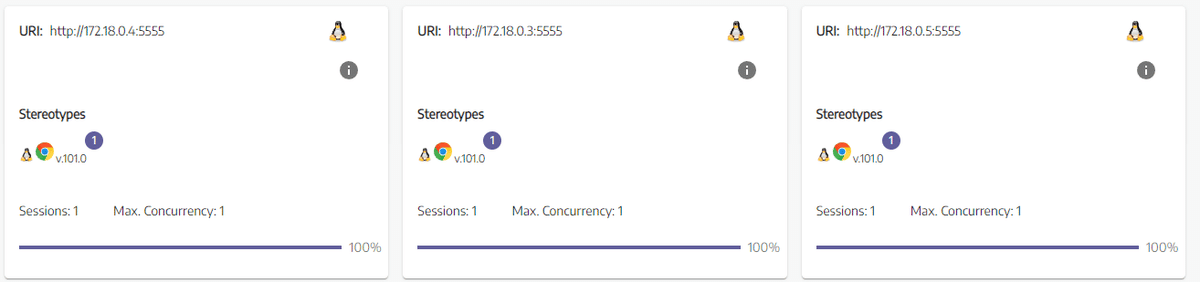
3並列で実行してラスト1つの処理が残った場面
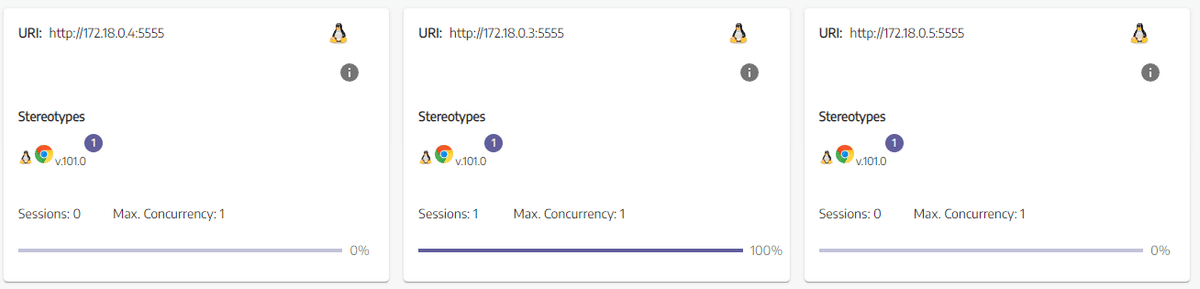
まとめ
特に意識せずSeleniumを動かすテストコードを書いて実行したら勝手にSelenium Gridが実行先を振り分けてくれてすごい便利でした。
この記事が気に入ったらサポートをしてみませんか?